Daily Hack #day84 - Automating Patch Management
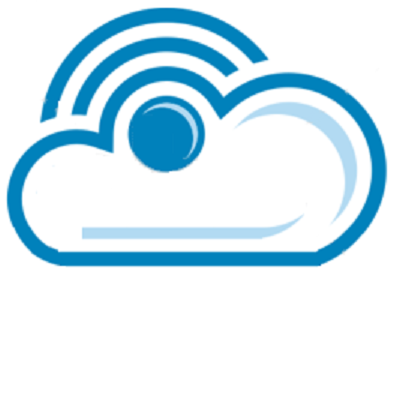
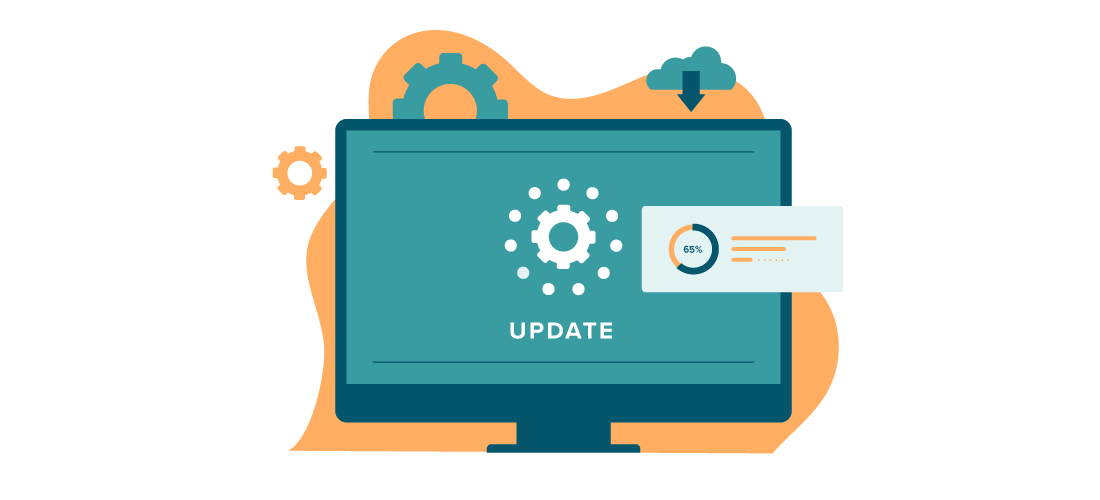
Below is an example of a shell script that automates the patch management process on a Linux system using the apt
package manager (commonly used in Debian-based systems like Ubuntu). This script will update the package list, upgrade all installed packages, and log the actions taken.
#!/bin/bash
# Define log file
LOG_FILE="/var/log/patch_management.log"
# Function to log messages with timestamp
log_message() {
echo "$(date +'%Y-%m-%d %H:%M:%S') - $1" | tee -a $LOG_FILE
}
# Update package list
log_message "Starting package list update"
sudo apt update >> $LOG_FILE 2>&1
if [ $? -ne 0 ]; then
log_message "Package list update failed"
exit 1
else
log_message "Package list updated successfully"
fi
# Upgrade all packages
log_message "Starting package upgrade"
sudo apt upgrade -y >> $LOG_FILE 2>&1
if [ $? -ne 0 ]; then
log_message "Package upgrade failed"
exit 1
else
log_message "Packages upgraded successfully"
fi
# Clean up unused packages
log_message "Starting package cleanup"
sudo apt autoremove -y >> $LOG_FILE 2>&1
if [ $? -ne 0 ]; then
log_message "Package cleanup failed"
exit 1
else
log_message "Package cleanup completed successfully"
fi
log_message "Patch management completed successfully"
exit 0
Steps Explained
Log File: The script logs its actions to
/var/log/patch_management.log
.Logging Function:
log_message
function logs messages with a timestamp.Updating Package List:
sudo apt update
updates the list of available packages and their versions.Logs the success or failure of the update process.
Upgrading Packages:
sudo apt upgrade -y
upgrades all installed packages to their latest versions.Logs the success or failure of the upgrade process.
Cleaning Up:
sudo apt autoremove -y
removes unused packages that were automatically installed to satisfy dependencies for other packages and are no longer needed.Logs the success or failure of the cleanup process.
Error Handling:
- If any step fails, the script logs an error message and exits with a non-zero status.
Running the Script
Make the script executable:
chmod +x patch_management.sh
Run the script:
./patch_management.sh
Scheduling with Cron
To automate this script to run periodically, you can add it to the cron jobs:
Edit the crontab:
crontab -e
Add a cron job to run the script daily at 2 AM:
0 2 * * * /path/to/patch_management.sh
Make sure to replace /path/to/patch_management.sh
with the actual path to your script.
This script provides a basic example of automated patch management. You can further enhance it by adding email notifications, handling more specific error conditions, or extending it for use with other package managers like yum
for Red Hat-based systems.
Subscribe to my newsletter
Read articles from Cloud Tuned directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
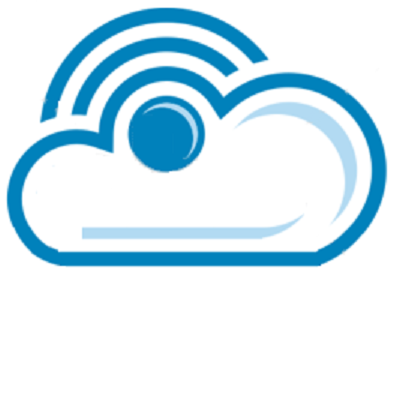