Javascript Strict Mode Explained: How to Write Secure and Efficient Code
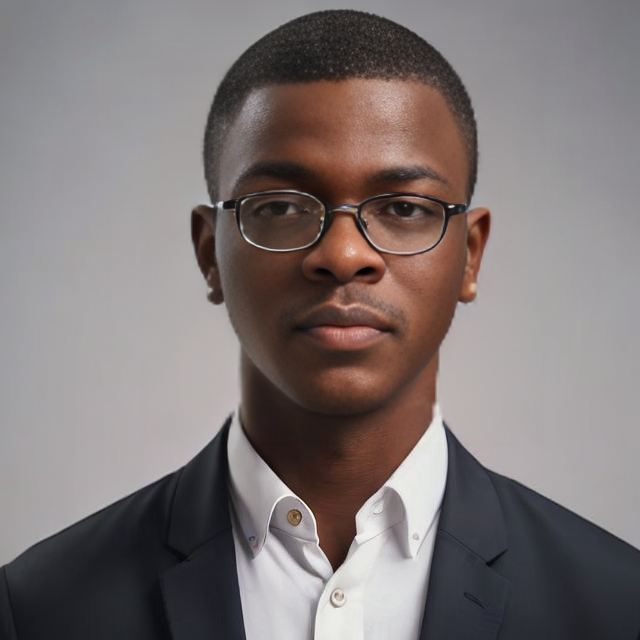
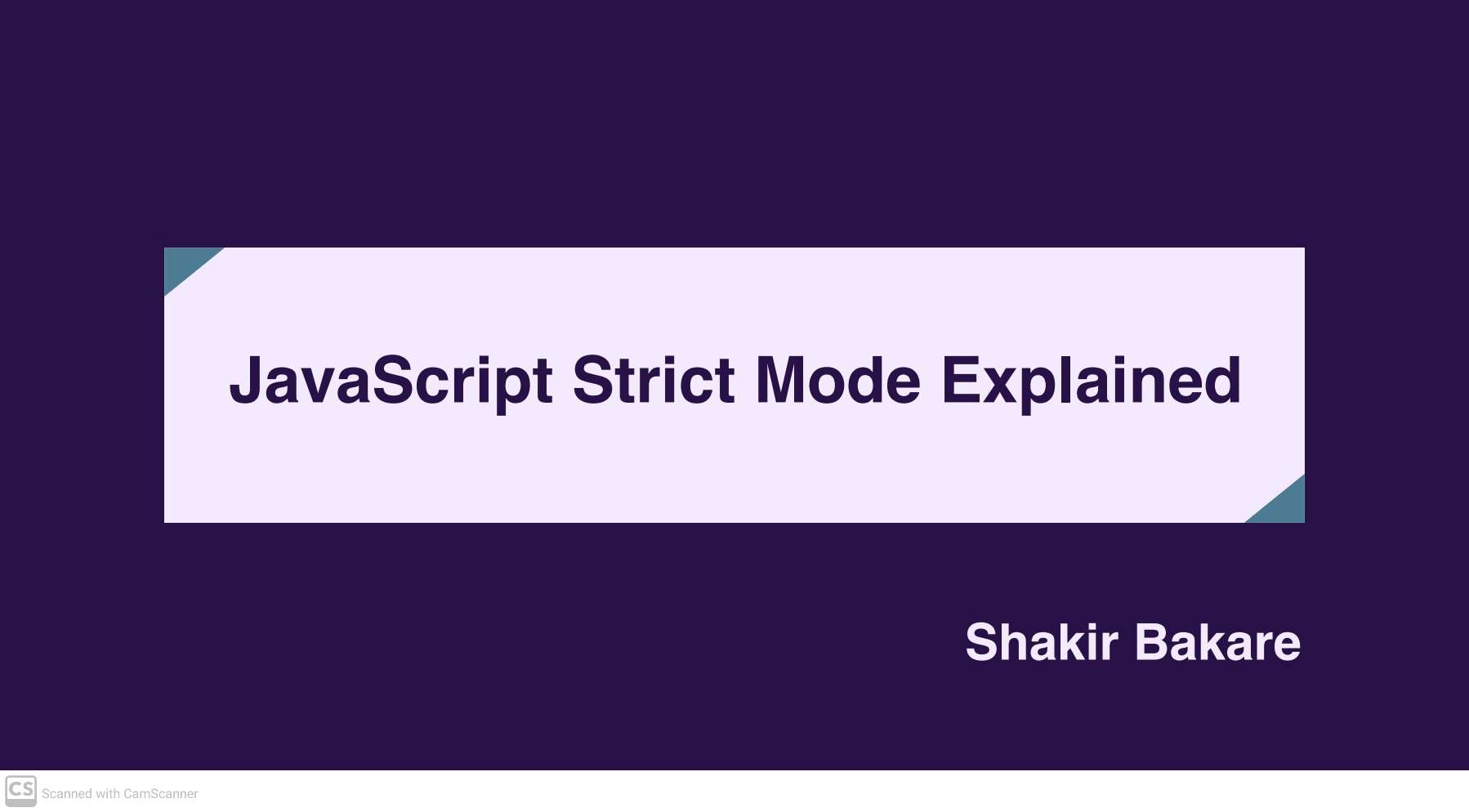
In the constantly changing world of web development, writing secure and efficient code is crucial. JavaScript, one of the most popular programming languages, offers a feature called "strict mode" to help developers with this. Introduced in ECMAScript 5 (ES5), strict mode enforces stricter parsing and error handling rules, making it easier to catch common mistakes and potential bugs. In this article, I will help you understand what strict mode is, explain how to use it, and highlights its many benefits, ultimately helping you write more robust and maintainable code.
What is Strict Mode?
Strict mode is a way to opt into a restricted variant of JavaScript, which can help you write more secure and efficient code. It was introduced in ECMAScript 5 (ES5) and can be applied to an entire script or individual functions.
How to Use Strict Mode
You can use strict mode for an entire script, or you can use it for functions.
For an entire script
Add "use strict";
at the beginning of the file.
"use strict";
// before your JavaScript codes
For a function
Add "use strict";
at the beginning of the function body.
function myFunction() {
"use strict";
// function code here
}
Advantage of Strict Mode
Strict mode makes it easier to catch common coding errors and bugs by throwing errors where the default behavior would have silently failed or produced unintended results. For instance, assigning values to undeclared variables results in an error in strict mode.
Without strict mode, if you forget to declare a variable with var, let, or const, JavaScript creates a global variable, which can lead to hard-to-track bugs. Strict mode eliminates this issue by ensuring that variables are properly declared.
In strict mode,
this
remains undefined in functions that are called as simple functions (rather than as methods). This helps prevent unexpected behavior when working withthis
.In non-strict mode, you can accidentally use the same name for multiple function parameters, which can lead to confusing behavior. Strict mode throws an error if duplicate parameter names are detected, promoting cleaner code.
Strict mode catches common programming mistakes, such as assigning to non-writable properties, assigning to getter-only properties, and deleting undeletable properties. This helps beginners learn better coding practices.
Strict mode ensures that your code is compatible with future versions of JavaScript by disallowing features that are likely to be deprecated. This helps in writing code that adheres to the latest standards.
By disallowing certain actions that are generally considered unsafe, such as using eval() to execute strings of code, strict mode helps enhance the security of your scripts.
Using strict mode encourages beginners to adopt best practices from the start, such as proper variable declarations and avoiding the use of potentially harmful constructs. This leads to better quality and more maintainable code.
It disables features that are considered problematic or error-prone. For example, strict mode does not let you use
with
andeval
in ways that can cause issues.
Conclusion
JavaScript strict mode is a powerful tool that helps developers write more secure, efficient, and maintainable code. By enforcing stricter parsing and error handling rules, it catches common mistakes and potential bugs early in the development process. Whether applied to an entire script or individual functions, strict mode promotes best practices, enhances code security, and ensures compatibility with future versions of JavaScript. Adopting strict mode in your projects can lead to cleaner, more robust code, ultimately improving the overall quality of your web applications.
Thank you for reading, and if you found this article helpful, please like, share, and leave your feedback.
Lets connect
Subscribe to my newsletter
Read articles from Shakir Bakare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
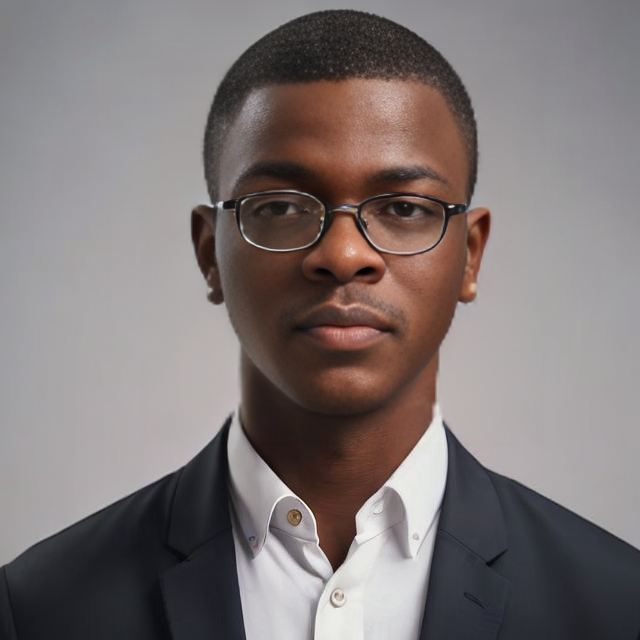
Shakir Bakare
Shakir Bakare
I'm a passionate frontend web developer with a love for creating beautiful and responsive web applications. With a strong foundation in HTML, CSS, JavaScript, and frameworks like React and Vue.js, I strive to bring innovative ideas to life. When I'm not coding, I enjoy sharing my knowledge through technical writing. I believe in the power of clear and concise documentation, and I write articles and tutorials to help others on their coding journey. Let's connect and create something amazing together!