Automating Ad Campaigns with Open AI and Python-Django
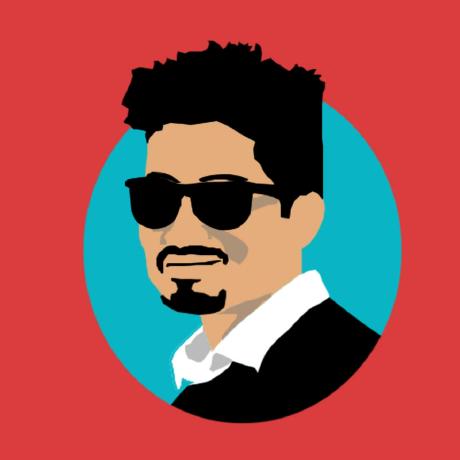
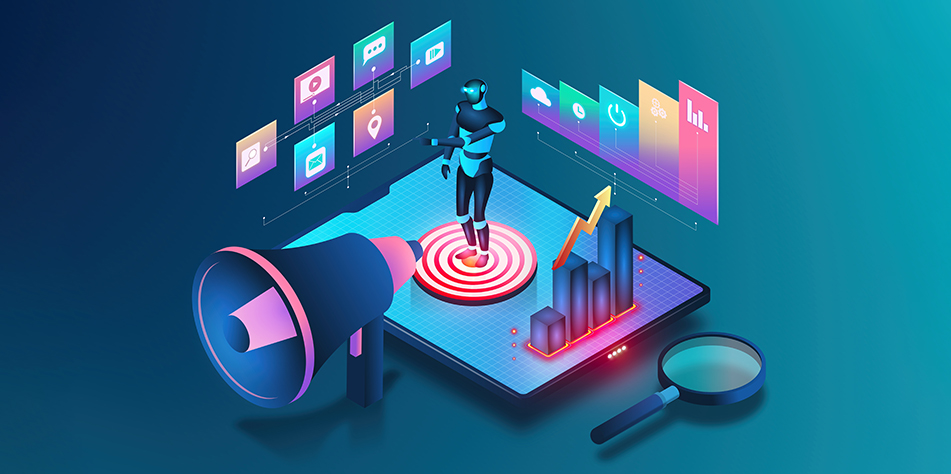
Outline:
Introduction
Purpose of the blog post
Importance of integrating OpenAI API into Ad Tech products
Brief overview of what will be covered
Understanding the Integration of OpenAI API in Ad Tech
Overview of OpenAI API
Benefits of using OpenAI for ad campaigns
Case studies and examples of successful integrations
Streamlining and Automating Ad Campaigns
How OpenAI API can help streamline ad campaigns
Automating ad creation and optimization
Ensuring character limit compliance for Google and Facebook
Sample Best Performing Ad Titles and Descriptions in Different Tones
List of tones: Persuasive, Witty, Professional, Friendly, Urgent
Examples of ad titles and descriptions for each tone
Technical Implementation: Using Python, Django, DRF, and PostgreSQL
Setting up the development environment
Creating the Django project and app
Configuring Django REST Framework (DRF)
Setting up PostgreSQL
Fetching Best Performing Ad Campaigns
Integrating with Google BigQuery, Google Ads, and Facebook Ads
Creating an API to fetch ad data
Storing and managing ad data in PostgreSQL
Using OpenAI GPT-3.5 Turbo for Ad Content Generation
Creating a prompt to fetch ad titles and descriptions
Integrating OpenAI API with the Django app
Parsing the OpenAI API response in Python
Building the API to Return Ad Content
Designing the API endpoint
Implementing the API to fetch and return ad content
Testing the API
Conclusion
Recap of the integration process
Benefits of using OpenAI in ad tech
Future possibilities and enhancements
Appendix
- Resources and references
1. Introduction
Integrating advanced AI technologies into ad tech products is revolutionizing how we approach digital marketing. By leveraging the power of OpenAI's API, businesses can streamline, automate, and enhance their ad campaigns across platforms like Google and Facebook. This blog post will guide you through a detailed tutorial on how to integrate OpenAI API into your ad tech product, ensuring your ads are compelling, effective, and within the character limits of each platform.
2. Understanding the Integration of OpenAI API in Ad Tech
OpenAI's API offers robust natural language processing capabilities that can generate high-quality text content. Integrating this API into ad tech products can significantly enhance ad copy creation, ensuring it is tailored to the target audience and optimized for performance. This integration not only saves time but also improves the effectiveness of ad campaigns by automating repetitive tasks and providing data-driven insights.
Benefits of using OpenAI API:
Automation: Automatically generate ad copy that adheres to platform guidelines.
Optimization: Continuously refine ad content based on performance metrics.
Personalization: Create personalized ad experiences at scale.
3. Streamlining and Automating Ad Campaigns
OpenAI's API can help streamline ad campaigns by automating the generation of ad titles and descriptions. It ensures that the content complies with the character limits for platforms like Google and Facebook, making the ad creation process more efficient and less error-prone.
Key advantages include:
Time Efficiency: Reduce the time spent on creating and revising ad copy.
Consistency: Maintain a consistent tone and style across all ads.
Scalability: Easily scale ad creation to match campaign needs.
4. Sample Best Performing Ad Titles and Descriptions in Different Tones
To demonstrate the power of OpenAI, here are examples of ad titles and descriptions tailored to different tones:
Persuasive:
Title: "Unlock Your Potential with Our Premium Courses"
Description: "Join thousands of learners who have transformed their careers. Enroll now and get a 20% discount!"
Witty:
Title: "Boost Your Brainpower – No Magic Wand Needed!"
Description: "Our courses are spellbindingly good! Enroll today and start your magical learning journey."
Professional:
Title: "Advance Your Career with Expert-Led Courses"
Description: "Gain in-demand skills from industry leaders. Enroll now to elevate your professional profile."
Friendly:
Title: "Learn Something New Today – We've Got Your Back!"
Description: "Join our community of learners and enjoy fun, interactive courses. Sign up now for a special offer!"
Urgent:
Title: "Last Chance! Enroll Now and Save Big"
Description: "Don't miss out on our limited-time discount. Sign up today and start learning immediately!"
5. Technical Implementation: Using Python, Django, DRF, and PostgreSQL
Setting up the Development Environment:
Install Python and virtualenv:
sudo apt update sudo apt install python3 python3-venv python3-pip
Create and activate a virtual environment:
python3 -m venv myenv source myenv/bin/activate
Install Django and Django REST Framework:
pip install django djangorestframework psycopg2-binary
Create a new Django project and app:
django-admin startproject adtech_project cd adtech_project django-admin startapp ads
Configuring Django REST Framework (DRF): Add rest_framework
to your INSTALLED_APPS
in settings.py
:
INSTALLED_APPS = [
...
'rest_framework',
'ads',
]
Setting up PostgreSQL:
Install PostgreSQL:
sudo apt install postgresql postgresql-contrib
Create a database and user:
CREATE DATABASE adtech_db; CREATE USER adtech_user WITH PASSWORD 'yourpassword'; ALTER ROLE adtech_user SET client_encoding TO 'utf8'; ALTER ROLE adtech_user SET default_transaction_isolation TO 'read committed'; ALTER ROLE adtech_user SET timezone TO 'UTC'; GRANT ALL PRIVILEGES ON DATABASE adtech_db TO adtech_user;
Configure Django to use PostgreSQL: In
settings.py
, update theDATABASES
setting:DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'adtech_db', 'USER': 'adtech_user', 'PASSWORD': 'yourpassword', 'HOST': 'localhost', 'PORT': '', } }
6. Fetching Best Performing Ad Campaigns
Integrating with Google BigQuery, Google Ads, and Facebook Ads: To fetch ad performance data, you will need to set up API connections with Google BigQuery, Google Ads, and Facebook Ads.
Google BigQuery:
Install Google Cloud library:
pip install google-cloud-bigquery
Authenticate and fetch data:
from google.cloud import bigquery client = bigquery.Client() query = """ SELECT ad_title, ad_description, performance_metric FROM `your_project.your_dataset.your_table` ORDER BY performance_metric DESC LIMIT 1 """ query_job = client.query(query) results = query_job.result() for row in results: print(row.ad_title, row.ad_description, row.performance_metric)
Google Ads and Facebook Ads:
- Follow similar steps to authenticate and fetch data using their respective APIs.
Storing and Managing Ad Data in PostgreSQL:
Create models in Django for storing ad data:
from django.db import models class Ad(models.Model): title = models.CharField(max_length=255) description = models.TextField() platform = models.CharField(max_length=50) performance_metric = models.FloatField() created_at = models.DateTimeField(auto_now_add=True)
Run migrations to create the database schema:
python manage.py makemigrations python manage.py migrate
7. Using OpenAI GPT-3.5 Turbo for Ad Content Generation
Creating a Prompt to Fetch Ad Titles and Descriptions: To generate ad content, create a prompt that specifies the required tone and character limits.
Integrating OpenAI API with Django:
Install OpenAI library:
pip install openai
Generate ad content using OpenAI:
import openai openai.api_key = 'your_openai_api_key' def generate_ad_content(tone, character_limit): response = openai.Completion.create( engine="text-davinci-003", prompt=f"Generate an ad title and description with a {tone} tone within {character_limit} characters.", max_tokens=100 ) return response.choices[0].text.strip()
Parsing the OpenAI API Response in Python: The response from OpenAI will be in JSON format. Parse it to extract the ad title and description.
import json
def parse_openai_response(response):
data = json.loads(response)
title = data['title']
description = data['description']
return title, description
8. Building the API to Return Ad Content
Designing the API Endpoint: Create an endpoint to fetch and return ad content.
Implementing the API:
Define a view in Django:
from rest_framework.views import APIView from rest_framework.response import Response from rest_framework import status class AdContentView(APIView): def get(self, request, tone, character_limit): ad_content = generate_ad_content(tone, character_limit) title, description = parse_openai_response(ad_content) return Response({"title": title, "description": description}, status=status.HTTP_200_OK)
Add the URL pattern:
from django.urls import path from .views import AdContentView urlpatterns = [ path('ad-content/<str:tone>/<int:character_limit>/', AdContentView.as_view(), name='ad_content'), ]
Test the API: Use tools like Postman or curl to test the API endpoint.
9. Conclusion
By integrating OpenAI's API into your ad tech product, you can streamline and enhance your ad campaigns, ensuring they are effective and compliant with platform guidelines. This tutorial has covered the technical implementation in detail, demonstrating how to create a powerful tool for automated ad content generation.
10. Appendix
Resources and References:
By following this guide, you'll be well-equipped to harness the power of AI in your ad tech product, driving better results and maximizing efficiency.
For further help, visit AhmadWKhan.com
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
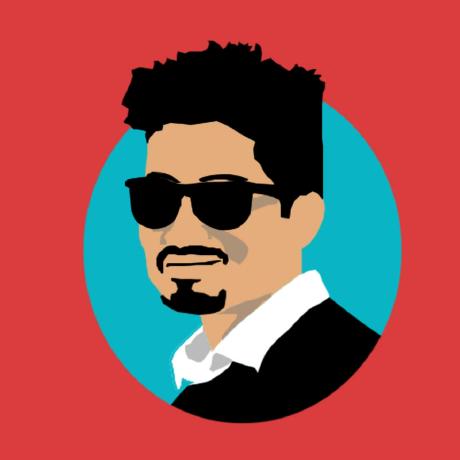