Day 3: Beginning My Animation Journey with GSAP

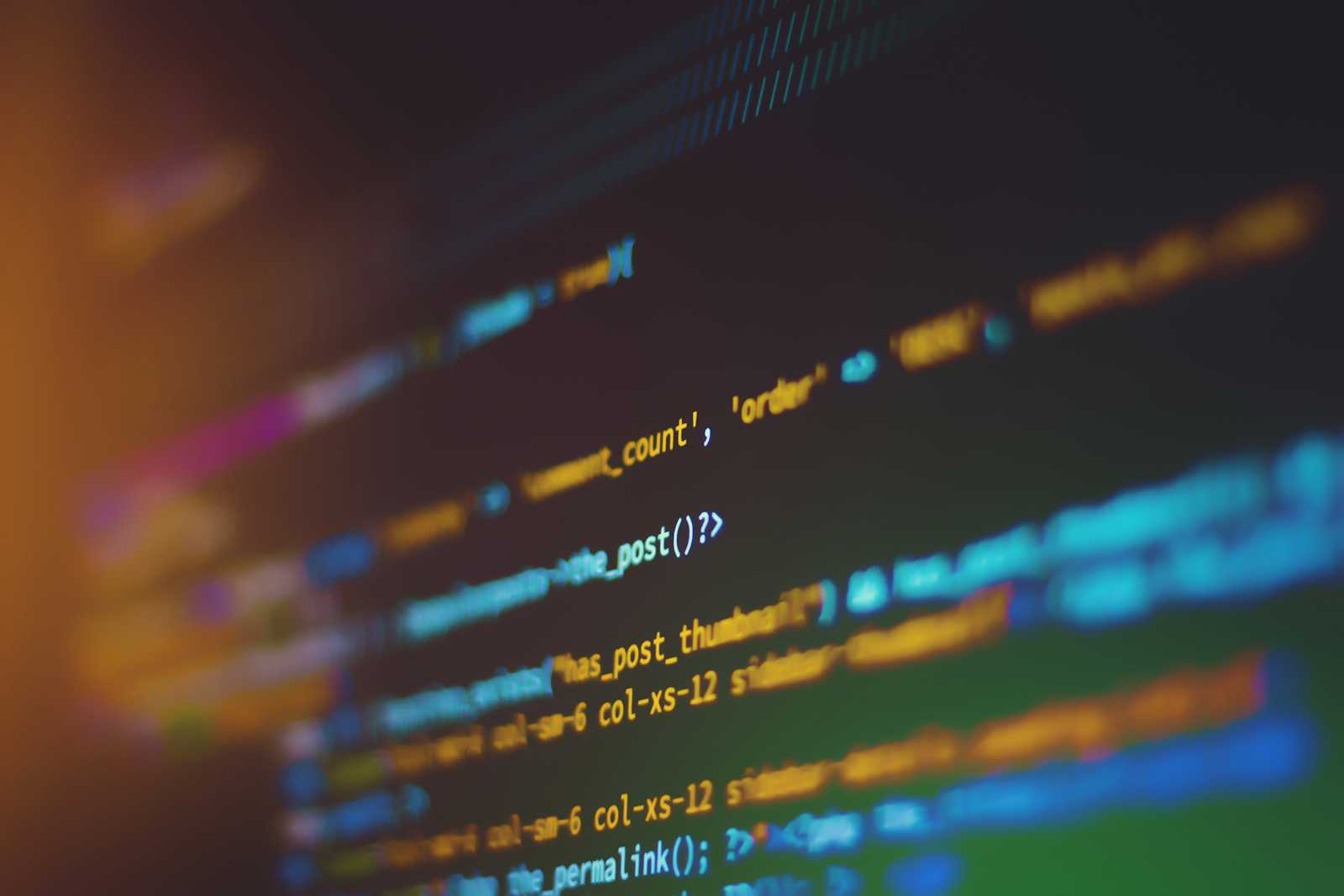
Today, I learned how to create a captivating text animation using GSAP. This animation makes text appear as if it's coming from the middle of the screen, adding a dynamic touch to headings. Here's a simple explanation of how I achieved this effect:
Breaking Down the Text
First, I split the text into individual characters and wrapped them in <span>
elements. This allowed me to animate each character separately.
//HTML Code
<body>
<h1>Saksham</h1>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.12.5/gsap.min.js"
integrity="sha512-7eHRwcbYkK4d9g/6tD/mhkf++eoTHwpNM9woBxtPUBWm67zeAfFC+HrdoE2GanKeocly/VxeLvIqwvCdk7qScg=="
crossorigin="anonymous"
referrerpolicy="no-referrer"
></script>
<script src="/text-animation/script.js"></script>
</body>
//Css Code
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: Arial;
}
html, body {
height: 100%;
width: 100%;
}
body{
background-color: #111;
}
#page1, #page2, #page3{
height: 100vh;
width: 100%;
padding: 5vw 0;
}
#move{
background-color: #D9FF06;
display: flex;
padding: 3vw 0;
overflow: hidden;
}
.marque img {
height: 80px;
}
.marque{
flex-shrink: 0;
display: flex;
align-items: center;
gap: 3vw;
padding: 0 1.5vw;
}
/* //make aall later capital */
.marque h1{
font-size: 70px;
text-transform: uppercase;
}
//Javascript
function breakText() {
let h1 = document.querySelector("h1");
let h1text = h1.textContent;
let splited = h1text.split("");
let halfValue = Math.floor(splited.length / 2);
console.log(halfValue);
let clutter = "";
splited.forEach(function (elem, idx) {
if (idx < halfValue) {
clutter += `<span class="a">${elem}</span>`;
} else {
clutter += `<span class="b">${elem}</span>`;
}
});
h1.innerHTML = clutter;
}
breakText();
Select the Heading: I selected the
<h1>
element and got its text content.Split the Text: I split the text into individual characters.
Wrap Characters in Spans: I wrapped each character in a
<span>
, assigning classes "a" and "b" to the first and second halves, respectively.Update the Heading: I replaced the original text with the new HTML structure.
Animating the Text with GSAP
Next, I used GSAP to animate the characters, making them move up and fade in from the bottom.
gsap.from("h1 .a", {
y: 50,
duration: 0.6,
delay: 0.5,
stagger: 0.15,
opacity: 0,
});
gsap.from("h1 .b", {
y: 50,
duration: 0.6,
delay: 0.5,
stagger: -0.15,
opacity: 0,
});
Animate First Half: The characters in the first half (
.a
) move up by 50 pixels, fade in over 0.6 seconds, start after a 0.5-second delay, and stagger their animations by 0.15 seconds.Animate Second Half: The characters in the second half (
.b
) have the same animation properties but with a negative stagger value, making them animate in the opposite order.
Conclusion
Today's lesson was about creating engaging text animations with GSAP. By splitting the text into individual characters and animating them with different timings, I achieved a dynamic effect that makes headings more visually appealing. This technique can be used to add a lively touch to any website, enhancing user experience with smooth and captivating animations.
Subscribe to my newsletter
Read articles from Saksham basnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saksham basnet
Saksham basnet
I am a Frontend Developer From Nepal ꔪ