The Complete Transformers Library Guide: Harnessing Pre-Trained Model Power

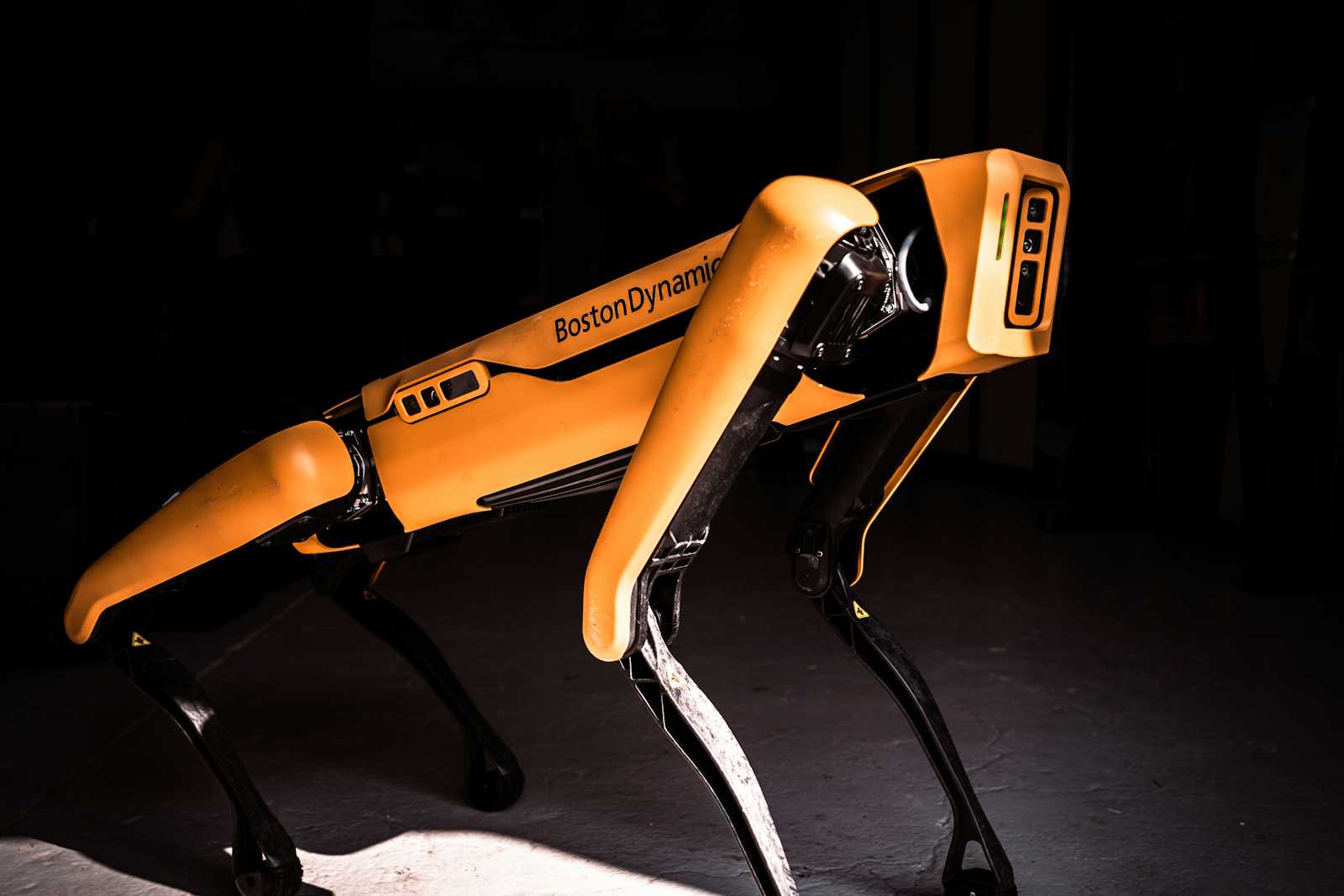
The transformers library is a game-changer in the world of natural language processing (NLP). With its vast collection of pre-trained models, it has revolutionized the way we approach NLP tasks. In this comprehensive guide, we'll delve into the world of transformers and explore how to harness their power to achieve state-of-the-art results in various NLP applications.
What are Transformers?
Transformers are a type of neural network architecture introduced in the paper "Attention is All You Need" by Vaswani et al. in 2017. They're primarily designed for sequence-to-sequence tasks, such as machine translation, but have since been adapted for a wide range of NLP applications.
The Transformers Library
The transformers library is a Python package developed by Hugging Face that provides a unified interface for using pre-trained models. It's an incredible resource that allows developers to easily access and utilize the power of transformers in their projects.
Installing the Transformers Library
To get started with the transformers library, you'll need to install it using pip:
pip install transformers
Loading Pre-Trained Models
One of the most significant advantages of the transformers library is its vast collection of pre-trained models. These models have been trained on massive datasets and can be fine-tuned for specific tasks. To load a pre-trained model, you can use the AutoModel
class:
from transformers import AutoModel
model = AutoModel.from_pretrained("bert-base-uncased")
This code loads the BERT-base-uncased model, which is a popular choice for many NLP tasks.
Tokenization
Tokenization is a crucial step in NLP pipelines. The transformers library provides the AutoTokenizer
class, which can be used to tokenize input text:
from transformers import AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased")
inputs = tokenizer("Hello world!", return_tensors="pt")
This code tokenizes the input text "Hello world!" using the BERT-base-uncased tokenizer.
Sentiment Analysis
Sentiment analysis is a common NLP task that involves determining the sentiment of a piece of text. The transformers library provides a simple way to perform sentiment analysis using the pipeline
function:
from transformers import pipeline
classifier = pipeline('sentiment-analysis')
result = classifier('We are very happy to introduce pipeline to the transformers repository.')
print(result)
This code performs sentiment analysis on the input text and outputs the sentiment score.
Text Generation
Text generation is another popular NLP task that involves generating text based on a given prompt. The transformers library provides the pipeline
function for text generation:
from transformers import pipeline
generator = pipeline('text-generation')
result = generator('This is a sample input text', max_length=150)
print(result)
This code generates text based on the input prompt, with a maximum length of 150 tokens.
Hugging Face Transformers in Action
Hugging Face provides a wide range of pre-trained models and a simple interface for using them. Let's take a look at an example of generating text using GPT-2:
import torch
import transformers
from transformers import GPT2Tokenizer
from transformers import GPT2LMHeadModel
tokenizer = GPT2Tokenizer.from_pretrained("gpt2")
model = GPT2LMHeadModel.from_pretrained("gpt2", pad_token_id=tokenizer.eos_token_id)
input_string = "Yesterday I spent several hours in the library, studying"
input_tokens = tokenizer.encode(input_string, return_tensors="pt")
output_greedy = model.generate(input_tokens, max_length=256)
output_string = tokenizer.decode(output_greedy[0], skip_special_tokens=True)
print(f"Input sequence: {input_string}")
print(f"Output sequence: {output_string}")
This code generates text based on the input prompt, using the GPT-2 model.
Vertex AI and OpenAI
Vertex AI and OpenAI are two prominent organizations that have made significant contributions to the development of transformers. Vertex AI provides a platform for building, deploying, and managing machine learning models, including transformers. OpenAI, on the other hand, is a research organization that has developed several popular transformer models, including GPT-3.
Beam Search and Repetition Penalty
When generating text, it's often useful to use beam search to explore multiple possible text branches. The transformers library provides a simple way to integrate beam search and repetition penalty:
output_beam = model.generate(input_tokens, max_length=64, num_beams=32, no_repeat_ngram_size=2, early_stopping=True)
This code generates text based on the input prompt, using beam search and repetition penalty.
Conclusion
The transformers library is an incredibly powerful tool for NLP tasks. With its vast collection of pre-trained models and simple interface, it's easy to get started with transformers and achieve state-of-the-art results. In this guide, we've covered the basics of the transformers library and explored its applications in sentiment analysis, text generation, named entity recognition, question answering, and language translation. We've also taken a look at Hugging Face, Vertex AI, and OpenAI, and explored the use of beam search and repetition penalty. Whether you're a seasoned NLP practitioner or just starting out, the transformers library is an essential tool to have in your toolkit.
Further Reading
Get Started with the Transformers Library Today!
With this comprehensive guide, you're ready to start exploring the world of transformers and achieving state-of-the-art results in your NLP projects. Remember to install the transformers library using pip and start experimenting with its vast collection of pre-trained models. Happy coding!
Additional Details on Transformers
Transformers are a type of neural network architecture that use self-attention mechanisms to process input sequences.
The transformers library provides a unified interface for using pre-trained models, making it easy to get started with transformers.
Pre-trained models can be fine-tuned for specific tasks, such as sentiment analysis, text generation, named entity recognition, question answering, and language translation.
Hugging Face is a leading provider of pre-trained models and a simple interface for using them.
Vertex AI is a platform for building, deploying, and managing machine learning models, including transformers.
OpenAI is a research organization that has developed several popular transformer models, including GPT-3.
Beam search and repetition penalty can be used to generate text based on input prompts.
Additional Resources
Additional Code Examples
Subscribe to my newsletter
Read articles from Niladri Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
