Guide to State Management in Flutter with GetX || Part - 1
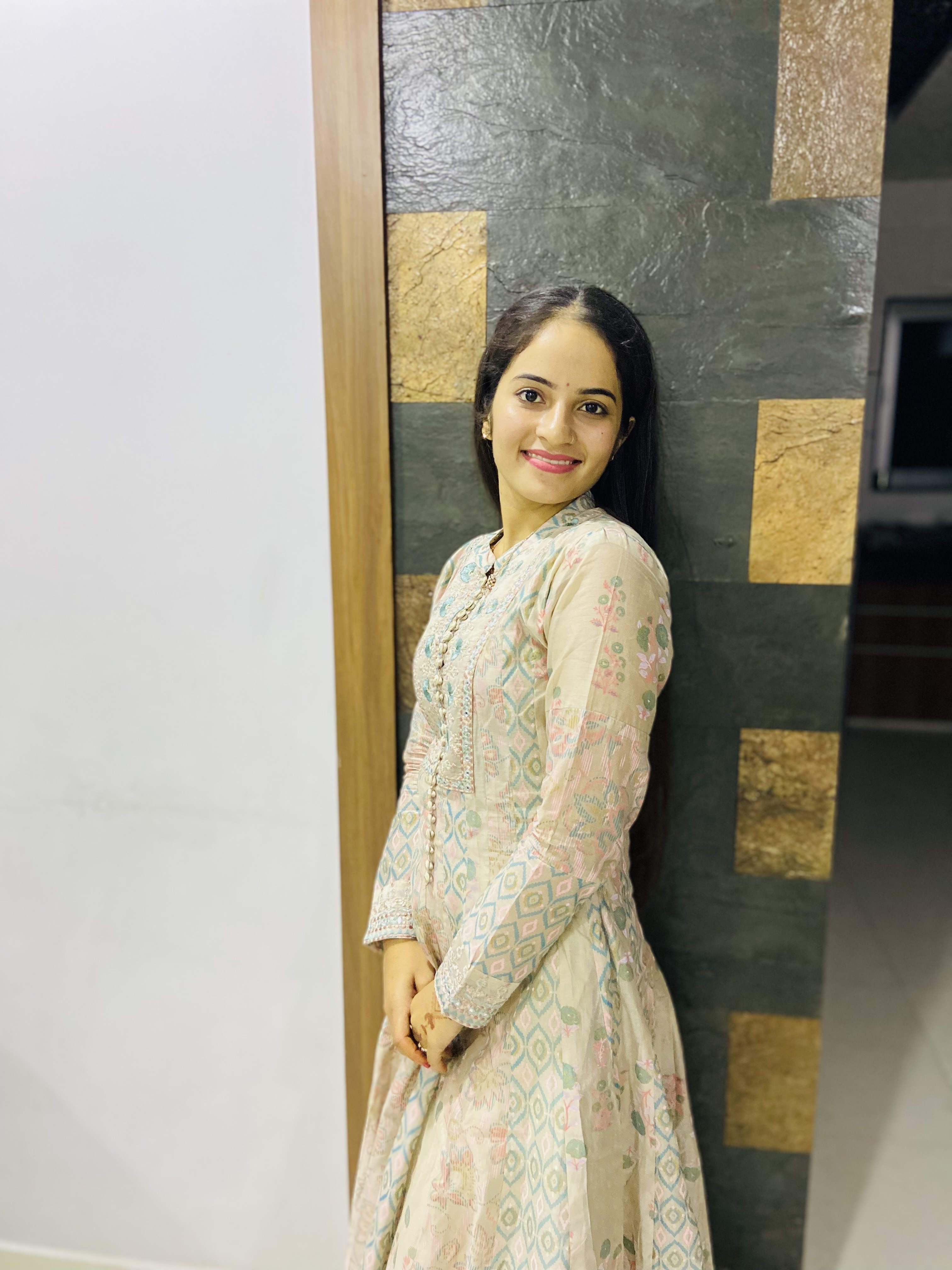
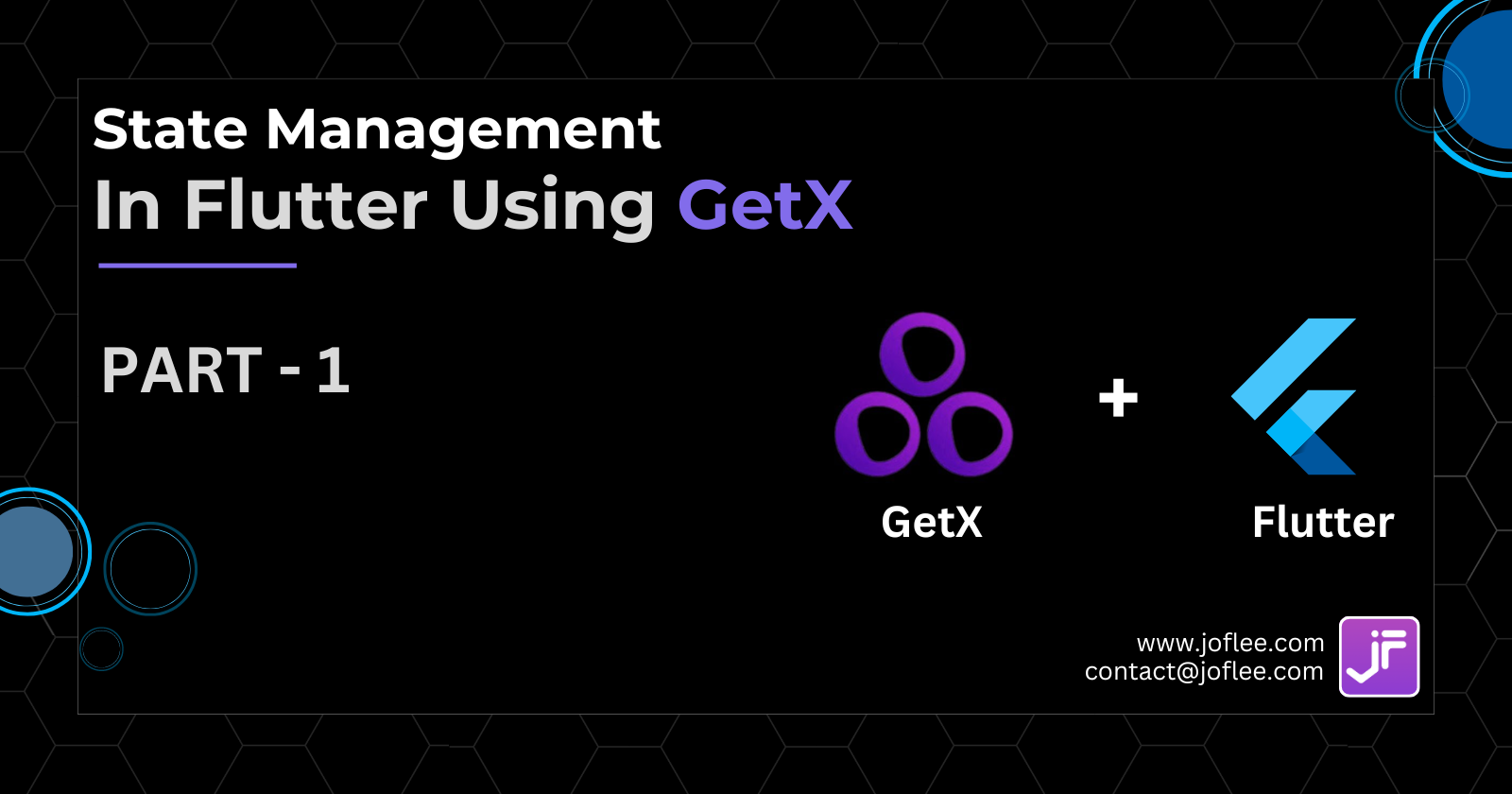
Let's dive into GetX, a lightweight and powerful state management library for Flutter. Designed for simplicity and efficiency, GetX helps manage app state and provides real-time updates to the user interface. This guide will walk you through using GetX for state management, making your Flutter development experience smoother and more productive.
What is GetX?
GetX is not just a state management library; it’s a micro-framework that combines route management and dependency injection.
It aims to deliver an excellent development experience with minimal overhead, making it an ideal choice for Flutter apps.
Three pillars of GetX:
Performance: Minimal memory and resource consumption.
Productivity: Intuitive syntax and straightforward tools save development time.
Organisation: Decouples business logic from view and presentation logic without needing context for navigation or stateful widgets.
State Management with GetX:
GetX allows you to manage states efficiently without context.
You can create reactive state variables using
Rx
classes (e.g.,RxInt
,RxString
).Example:
final count = 0.obs;
Update the state:
count.value++;
Routing with GetX:
Define routes without context:
Get.toNamed('/details');
Use named routes in your app:
GetMaterialApp( initialRoute: '/', getPages: [ GetPage(name: '/', page: () => HomeScreen()), GetPage(name: '/details', page: () => DetailsScreen()), ], )
Dependency Injection:
GetX provides a simple way to inject dependencies using
Get.put
orGet.lazyPut
.Example:
Get.put(ApiService());
Access the service anywhere in your app:
final apiService = Get.find<ApiService>();
Other Features:
Snack bar and Dialogs: Easily show snack bar messages or dialogs.
Internationalisation (i18n): Localise your app with GetX.
Bindings: Manage controller lifecycles.
Reactive Workers: Execute background tasks reactively.
Example of using Reactive state Variables with GetX:
Setting Up GetX: First, make sure you’ve added the
get
package to yourpubspec.yaml
file:dependencies: flutter: sdk: flutter get: ^4.6.6
Create a Controller: Create a controller class that extends
GetxController
. This controller will manage our state:import 'package:get/get.dart'; class CounterController extends GetxController { final count = 0.obs; // Observable state variable void increment() { count.value++; // Automatically triggers UI updates } void decrement() { count.value--; } }
Use the Controller in Your Widget: In your widget, use the
GetBuilder
widget to listen to changes in thecount
variable:import 'package:flutter/material.dart'; import 'package:get/get.dart'; class CounterScreen extends StatelessWidget { final CounterController _controller = Get.put(CounterController()); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Counter App')), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text('Count:', style: TextStyle(fontSize: 24)), GetBuilder<CounterController>( builder: (controller) => Text( '${controller.count}', // Display the Number style: TextStyle(fontSize: 36), ), ), SizedBox(height: 16), Row( mainAxisAlignment: MainAxisAlignment.center, children: [ ElevatedButton( onPressed: _controller.increment, child: Text('Increment'), ), SizedBox(width: 16), ElevatedButton( onPressed: _controller.decrement, child: Text('Decrement'), ), ], ), ], ), ), ); } }
Run Your App: Run your app, and you’ll see the counter value updating as you press the “Increment” and “Decrement” buttons.
Learn more about dependency injection with GetX:
Certainly! Let’s dive into dependency injection (DI) with GetX in Flutter. Dependency injection is a technique that allows you to manage and provide dependencies (such as services, repositories, or other objects) to different parts of your app without tightly coupling them. GetX makes DI straightforward and efficient.
Why Use Dependency Injection?
Decoupling: DI helps decouple your app’s components, making them more modular and easier to maintain.
Testability: By injecting dependencies, you can easily mock or replace them during testing.
Reusability: Reusable services can be injected into multiple parts of your app.
How Does GetX Handle Dependency Injection?
GetX uses a simple and intuitive approach:
Get.put<T>(T instance)
: Registers an instance of typeT
globally. You can access it anywhere usingGet.find<T>()
.Get.lazyPut<T>(() => T())
: Lazily creates an instance ofT
when it is first accessed.Get.delete<T>()
: Removes the instance of typeT
.
Example: Injecting an API Service
Let’s say you have an
ApiService
class that handles network requests. Here’s how you’d use DI with GetX://1). Create your ApiService class class ApiService { Future<String> fetchData() async { // Fetch data from an API return 'Data from API'; } } // 2). Inject ApiService globally final apiService = Get.put(ApiService()); // 3). Use it in your widget class MyWidget extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Dependency Injection')), body: Center( child: ElevatedButton( onPressed: () async { final data = await apiService.fetchData(); print('Received data: $data'); }, child: Text('Fetch Data'), ), ), ); } }
Lazy Initialisation If you want to create the
ApiService
only when needed (lazy initialisation), useGet.lazyPut
:final apiService = Get.lazyPut(() => ApiService());
Scoped Dependencies You can also create scoped dependencies for specific parts of your app (e.g., per screen or feature). Use
Get.create
:final myScopedService = Get.create(() => MyScopedService());
Clean Up Don’t forget to clean up when your widget or screen is disposed:
@override void dispose() { Get.delete<ApiService>(); super.dispose(); }
That’s the essence of dependency injection with GetX! It keeps your code organised, testable, and maintainable. Feel free to explore more features like reactive state management and routing with GetX.
Advantages of using GetX over other state management libraries:
Certainly! Let’s explore the advantages of using GetX for state management in Flutter compared to other libraries:
Lightweight and Minimal Boilerplate:
GetX is incredibly lightweight, which means less overhead in your app.
It requires minimal boilerplate code, making development faster and more efficient.
Reactive State Management:
GetX provides reactive state management using
Rx
classes (e.g.,RxInt
,RxString
).Changes to state variables automatically trigger UI updates without using
setState
.
Dependency Injection (DI):
GetX integrates DI seamlessly. You can inject dependencies globally or lazily.
DI improves code organisation, testability, and reusability.
Routing and Navigation:
GetX combines state management and routing.
Define named routes easily and navigate without context.
Performance Optimisation:
GetX optimises memory usage and performance.
It’s designed to be efficient even in large apps.
Additional Features:
Snackbar and Dialogs: Show snackbar messages or dialogs effortlessly.
Internationalisation (i18n): Localise your app with GetX.
Bindings: Manage controller lifecycles.
Reactive Workers: Execute background tasks reactively.
Community and Documentation:
GetX has an active community and excellent documentation.
You’ll find tutorials, examples, and support readily available.
Now, as we conclude this segment, stay tuned for the second part! We’ll dive deeper into advanced techniques, explore hidden gems, and empower you to create robust and efficient Flutter applications using GetX. Get ready for an exciting continuation! 🚀📝
Subscribe to my newsletter
Read articles from Fenisha Koladiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
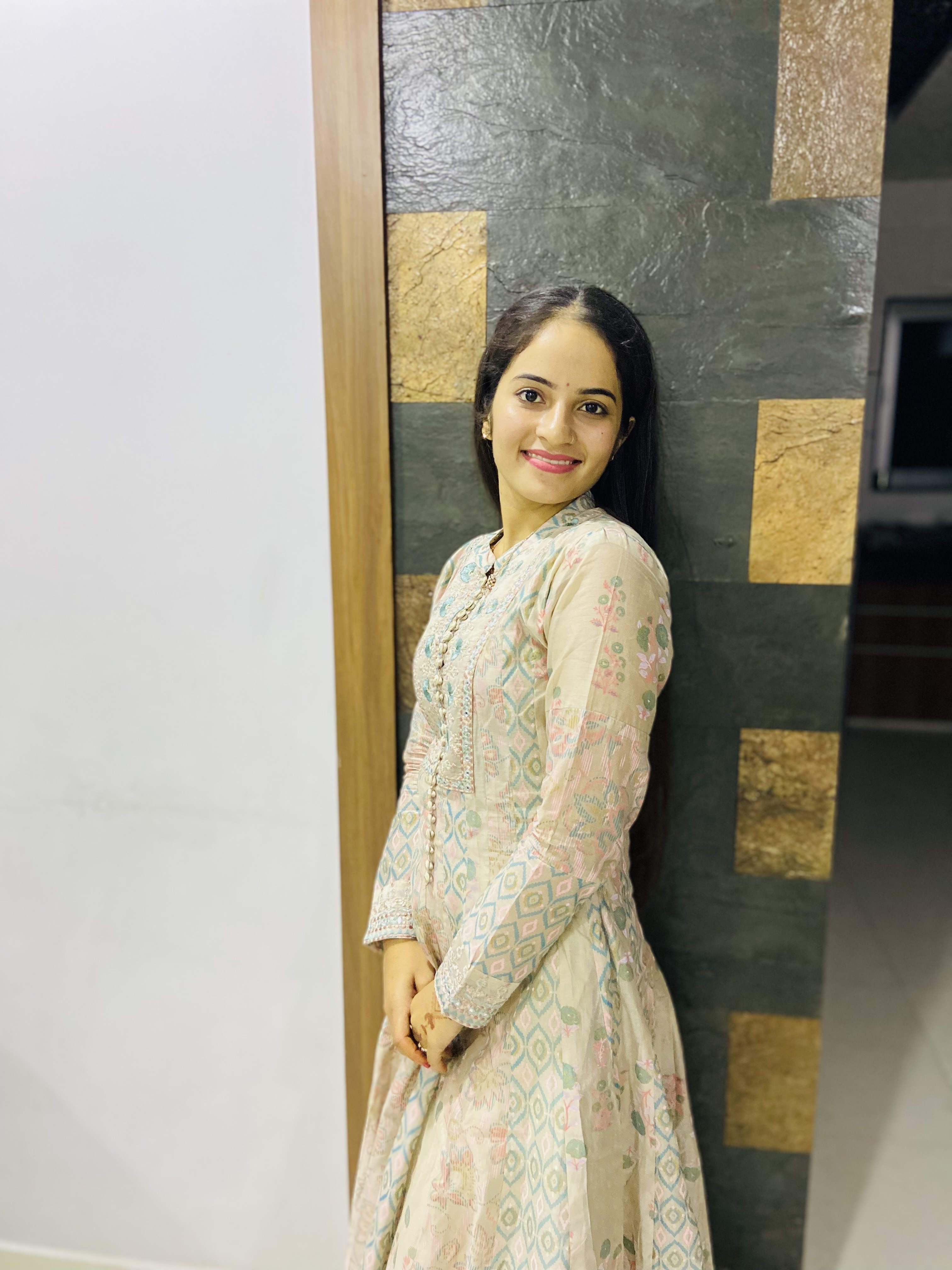