Step-by-Step Jest Testing Library for React Developers
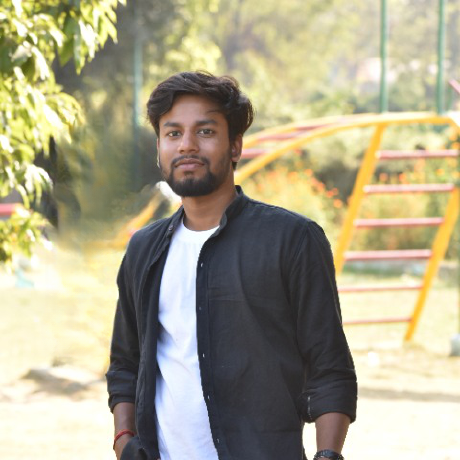
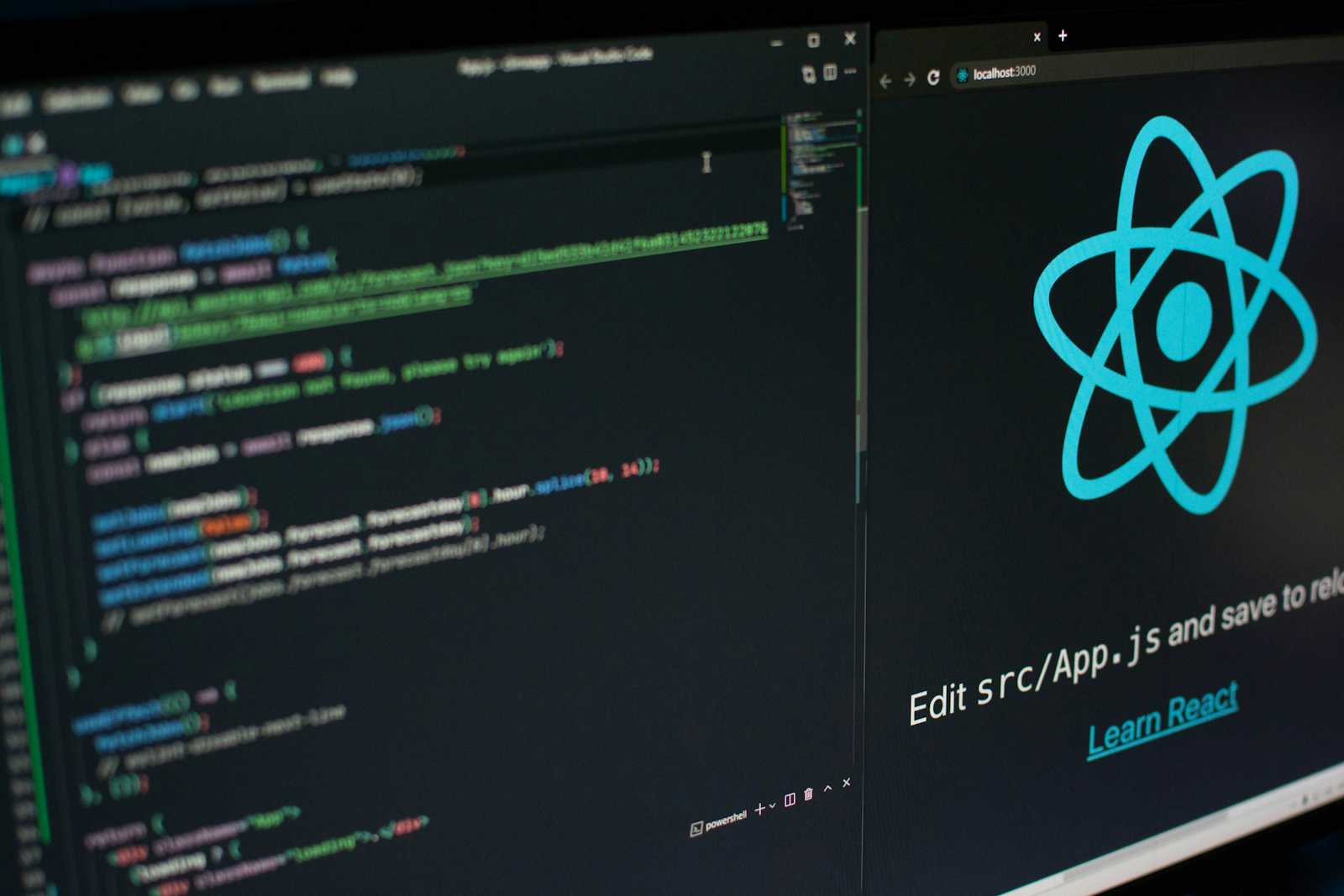
There are mainly 3 Types of testing that developers do.
Unit Testing: Testing individual units or components.
Integrated Testing: Testing between two units or component
E — 2 — E Testing: Test Start to end complete project.
Where do we focus most?
i. Mostly we focus on Unit and Integrated Testing.
ii. Also we try to cover some E-2-E testing topics.
iii. React developer needs to do component testing mostly that comes under unit testing.
What Should Do Testing
Important for developers
Dev Testing High in Demand
Interview Question covered
Code on git
Learning from scratch.
Run the First React Test
Install React app
Check Testing Library
Run test case
Check Output
Interview Questions
Jest Already comes default in the React app.
npx create-react-app app-name
sum.js
const sum = (a, b) => {
return a + b;
}
export default sum;
sum.test.js
import sum from "./sum";
test('should be sum', () => {
// expect(sum(10, 10)).toBe(20)
let a = 10;
let b = 20;
let output = 30
expect(sum(a, b)).toBe(output);
});
test('sum case 2', () => {
// expect(sum(10, 10)).toBe(20)
let a = 100;
let b = 200;
let output = 300
expect(sum(a, b)).toBe(output);
})j
OUTPUT
Test Suites: 1 passed, 1 total
Tests: 2 passed, 2 total
Snapshots: 0 total
Time: 7.38 s
Ran all test suites related to changed files.
Testing
As a developer, our primary goal is to build software that works
To ensure our software works, we test the application
We check if our software works as expected
Manual Testing
An individual will open the website, interact with the website and ensure
everything works as intended
If a new feature is released, you repeat the same steps
You may have to test not only the new feature but also the existing features
Drawbacks:
1. Time consuming
2. Complex repetitive tasks has a risk of human error
3. You may not get a chance to test all the features you should
Automated Testing
Automated tests are programs that automate the task of testing your software
Write code to test the software code
Additional effort required when you develop a feature
Advantages
1. Not time consuming
2. Reliable, consistent and not error prone
3. Easy to identify and fix features that break tests
4. Gives confidence when shipping software
Course structure
Jest and React Testing Library
Fundamentals of writing a test
Test components with user interactions
Test components wrapped in a provider
Test components with mocking
Static analysis testing
Jest vs RTL
Jest
Jest is a JavaScript testing framework
- Jest is a test runner that finds tests, runs the tests, determines whether the tests passed or failed and reports it back in a human readable manner
RTL
JavaScript testing utility that provides virtual DOM for testing React components
React Testing Library provides a virtual DOM which we can use to interact with and verify the behavior of a react component
Testing Library is infect a family of packages which helps test UI components
The core library is called DOM Testing library and RTL is simply a wrapper around
this core library to test React applications in an easier way
Types of Tests
Unit tests
Integration tests
E2E tests
Unit tests
Focus is on testing the individual building blocks of an application such as a class or a function or a component
Each unit or building block is tested in isolation, independent of other units
Dependencies are mockedRun in a short amount of time and make it very easy to pinpoint failures
Relatively easier to write and maintain
Integration tests
Focus is on testing a combination of units and ensuring they work together
Take longer than unit tests
E2E tests
Focus is on testing the entire application flow and ensuring it works as designed
from start to finishInvolves in a real UI, a real backend database, real services etc
Take the longest as they cover the most amount of code
Have a cost implication as you interact with real APIs that may charge based on the number of requests
Testing pyramid
RTL Philosophy
The more your tests resemble the way your software is used, the more confidence they can give you."
Tests we are going to learn to write in this series strike a balance between unit tests in the sense they are at a component level and easy to write and maintain and E2E tests in the sense they resemble the way a user would interact with the component
With React Testing Library, we are not concerned about the implementation details of a component
Instead we are testing how the component behaves when a user interacts with it
RTL will not care if you add 4+4 or 5+3 to display the number 8
Types of tests and RTL
Unit tests
Integration tests
E2E tests
RTL strikes a balance between unit and E2E tests which is what we will be learning in the rest of the series
code:
const getFullName
=
(fname, lname) => {
return ${fname} ${lname}
}
const actualFullName = getFullName('Bruce', 'Wayne')
const expectedFullName = 'BruceWayne'
if(actualFullName !== expectedFullName) {
}
throw new Error(`${actualFullName} is not equal to ${expectedFullName}`)
});
Test
test(name, fn, timeout)
The first argument is the test name used to identify the test
The second argument is a function that contains the expectations to test
The third argument is timeout which is an optional argument for specifying how long to wait before aborting the test. The default timeout value is 5 seconds.
import React from 'react';
import { render, screen } from '@testing-library/
react';
import App from './App';
test('renders learn react link', () => {
render(<App />);
const linkElement = screen.getByText(/learn
react/i);
});
expect(linkElement).toBeInTheDocument();
});
Test Driven Development (TDD)
Test driven development is a software development process where you write tests before writing the software code
Once the tests have been written, you then write the code to ensure the tests pass
Create tests that verify the functionality of a specific feature
Write software code that will run the tests successfully when re-executed
Refactor the code for optimization while ensuring the tests continue to pass
Also called red-green testing as all tests go from a red failed state to a green passed state
Note: I will add more content to this page related to ReactJS Testing with Jest Testing library. To get up to date you can bookmark this page or follow me.
Subscribe to my newsletter
Read articles from Suraj Choudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
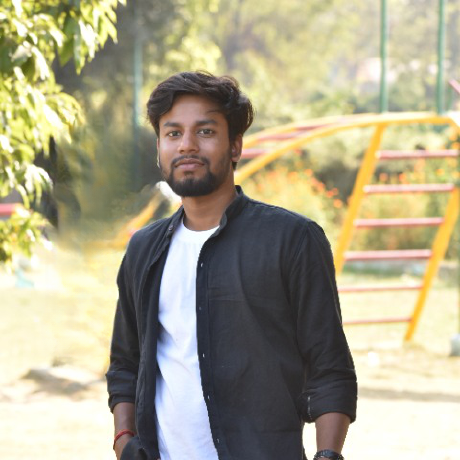
Suraj Choudhary
Suraj Choudhary
Who is a highly motivated and results-oriented Software Engineer with a passion for crafting robust and user-friendly web applications. Possesses strong expertise in both backend development and full-stack development, leveraging a diverse skillset to design, develop, and deploy scalable software solutions.