Embed Report Server Designer in Your Blazor WebAssembly App with Bold Reports
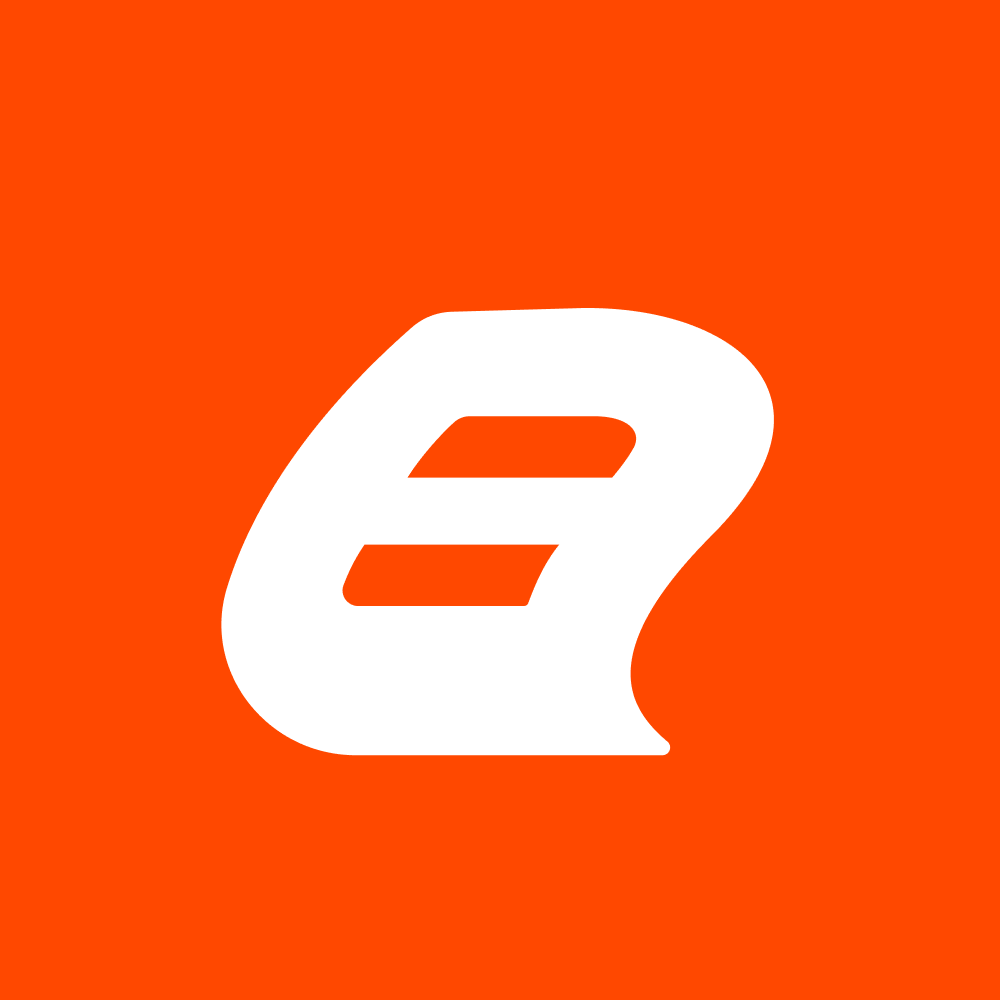
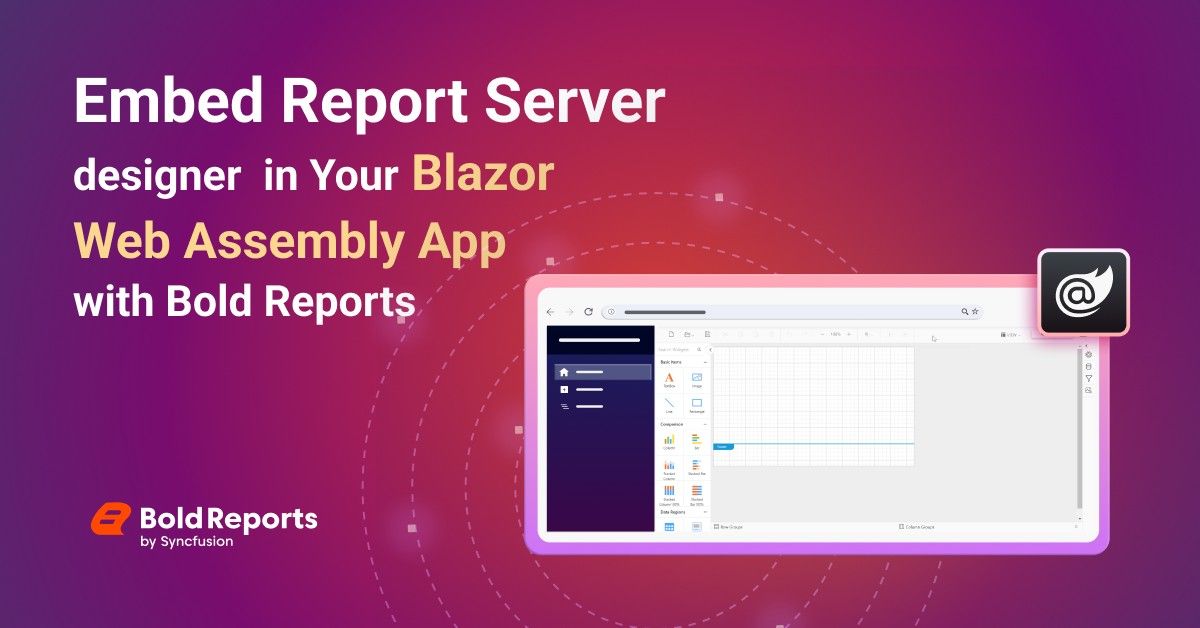
Embedding reporting features directly into web applications has become essential for businesses seeking actionable insights. Bold Reports offers a robust solution for integrating a report server designer into Blazor WebAssembly apps. This step-by-step guide will walk you through the process, letting your users create and customize reports directly within the app.
Prerequisites
Ensure that your development environment includes the following:
.NET 8.0 SDK or higher
Create Blazor WebAssembly Application
To create a new Blazor WebAssembly server application, open a command prompt, navigate to the directory where you want to create the project using the cd command, and then run the following command:
dotnet new blazorwasm -n ReportDesignerApp
A new Blazor WebAssembly application named ReportDesignerApp will be created.
Adding Scripts and Themes for Blazor Report Designer
Include the following CDN links with the boldreports-interop.js file in the head section of wwwroot\index.html to integrate the JavaScript reporting controls into your Blazor application.
<link href="https://cdn.boldreports.com/6.1.34/content/material/bold.reports.all.min.css" rel="stylesheet" />
<link href="https://cdn.boldreports.com/6.1.34/content/material/bold.reportdesigner.min.css" rel="stylesheet" />
<link href="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.37.0/codemirror.min.css" rel="stylesheet" />
<link href="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.37.0/addon/hint/show-hint.min.css" rel="stylesheet" />
<script src="https://cdn.boldreports.com/external/jquery-1.10.2.min.js" type="text/javascript"></script>
<script src="https://cdn.boldreports.com/external/jsrender.min.js" type="text/javascript"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.37.0/codemirror.min.js" type="text/javascript"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.37.0/addon/hint/show-hint.min.js" type="text/javascript"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.37.0/addon/hint/sql-hint.min.js" type="text/javascript"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.37.0/mode/sql/sql.min.js" type="text/javascript"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.37.0/mode/vb/vb.min.js" type="text/javascript"></script>
<!--Used to render the gauge item. Add this script only if your report contains the gauge report item. -->
<script src="https://cdn.boldreports.com/6.1.34/scripts/common/ej2-base.min.js"></script>
<script src="https://cdn.boldreports.com/6.1.34/scripts/common/ej2-data.min.js"></script>
<script src="https://cdn.boldreports.com/6.1.34/scripts/common/ej2-pdf-export.min.js"></script>
<script src="https://cdn.boldreports.com/6.1.34/scripts/common/ej2-svg-base.min.js"></script>
<script src="https://cdn.boldreports.com/6.1.34/scripts/data-visualization/ej2-lineargauge.min.js"></script>
<script src="https://cdn.boldreports.com/6.1.34/scripts/data-visualization/ej2-circulargauge.min.js"></script>
<!--Used to render the map item. Add this script only if your report contains the map report item.-->
<script src="https://cdn.boldreports.com/6.1.34/scripts/data-visualization/ej2-maps.min.js"></script>
<script src="https://cdn.boldreports.com/6.1.34/scripts/common/bold.reports.common.min.js"></script>
<script src="https://cdn.boldreports.com/6.1.34/scripts/common/bold.reports.widgets.min.js"></script>
<script src="https://cdn.boldreports.com/6.1.34/scripts/common/bold.report-designer-widgets.min.js"></script>
<!--Used to render the chart item. Add this script only if your report contains the chart report item.-->
<script src="https://cdn.boldreports.com/6.1.34/scripts/data-visualization/ej.chart.min.js"></script>
<!-- Report Designer component script-->
<script src="https://cdn.boldreports.com/6.1.34/scripts/bold.report-designer.min.js"></script>
<script src="https://cdn.boldreports.com/6.1.34/scripts/bold.report-designer.min.js"></script>
<!-- Blazor interop file -->
<script src="~/scripts/boldreports-interop.js"></script>
Report Server Configuration to Render the Report Designer
To embed the Report Designer from your report server and perform API actions with the server, you need to provide the necessary information from your report server:
serviceUrl: This API requires information about the report server reporting service.
reportServerUrl: This API requires information about the report server reporting server.
serviceAuthorizationToken: A security token if your reports require login. You can refer to the documentation to see how to generate a token within an application.
Depending on your report server type, you can follow one of the procedures below:
Initialize the Report Server Designer
To set up the Report Server Designer with essential parameters, you’ll need to incorporate the Bold Reports Report Designer control by generating an interop file:
Create a new class file named cs in the Data folder. This file will store properties to configure the Report Designer.
namespace BlazorReportingTools.Data { public class RenderReportDesigner { public string? ServiceURL { get; set; } public string? ServerURL { get; set; } public string? AuthorizationToken { get; set; } } }
Next, create a folder named scripts inside the wwwroot Within this folder, create a file named boldreports-interop.js. Add the following code to ensure the proper functioning of the Bold Report Designer, setting up the reportPath, reportServiceurl, reportServerUrl, and serviceAuthorizationToken.
// Interop file to render the Bold Report Designer component with properties. window.BoldReports = { RenderDesigner: function (elementID, reportDesignerOptions) { $("#" + elementID).boldReportDesigner({ serviceUrl: reportDesignerOptions.serviceURL, reportServerUrl: reportDesignerOptions.serverURL, serviceAuthorizationToken: reportDesignerOptions.authorizationToken }); } }
Embed Blazor Report Designer in a Razor Page
Open the Pages/home.razor file and add the following code snippet. This code integrates the IJSRuntime and invokes the JavaScript interop with the Report Server Designer URL specified in the Index.razor file, enabling you to view reports.
@page "/"
@using Microsoft.JSInterop
@using Microsoft.AspNetCore.Components
@inject IJSRuntime JSRuntime
@using BlazorReportingTools.Data;
<div id="report-designer" style="width: 100%;height: 950px"></div>
@code {
// Reportdesigner options
RenderReportDesigner designerOptions = new RenderReportDesigner();
// Used to render the Bold Report designer component in Blazor page.
public async void RenderReportDesigner()
{
designerOptions.serviceUrl = "http://{{Portno}}/reporting/reportservice/api/Designer";
designerOptions.reportServerUrl = "http://{{Portno}}/reporting/api/site/site";
designerOptions.serviceAuthorizationToken = "bearer token";
await JSRuntime.InvokeVoidAsync("BoldReports.RenderDesigner", "report-designer", designerOptions);
}
// Initial rendering of Bold Report designer
protected override void OnAfterRender(bool firstRender)
{
RenderReportDesigner();
}
}
Methods | Description |
RenderReportDesigner | Renders the Report Server Designer component in a Blazor page. |
OnAfterRender | Initializes the Report Designer by calling the method RenderReportDesigner that we created. |
Run the Blazor WebAssembly Application
To run your Blazor application and view the report, open the command prompt and navigate to the root directory of your Blazor Server project. Then, execute the following command:
dotnet run
Once your application is running, open the local host URL in your browser.
Your Blazor WebAssembly application will load, and the report will be rendered and displayed in the Report Designer.
Conclusion
I hope this blog has clarified how to embed the Report Server Designer in your Blazor WebAssembly app with Bold Reports. To delve deeper into the Report Designer’s features, feel free to explore.
Bold Reports offers a free 15-day trial, and you don’t need to provide any credit card details. We encourage you to try it out and share your feedback with us!
Subscribe to my newsletter
Read articles from Bold Reports Team directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
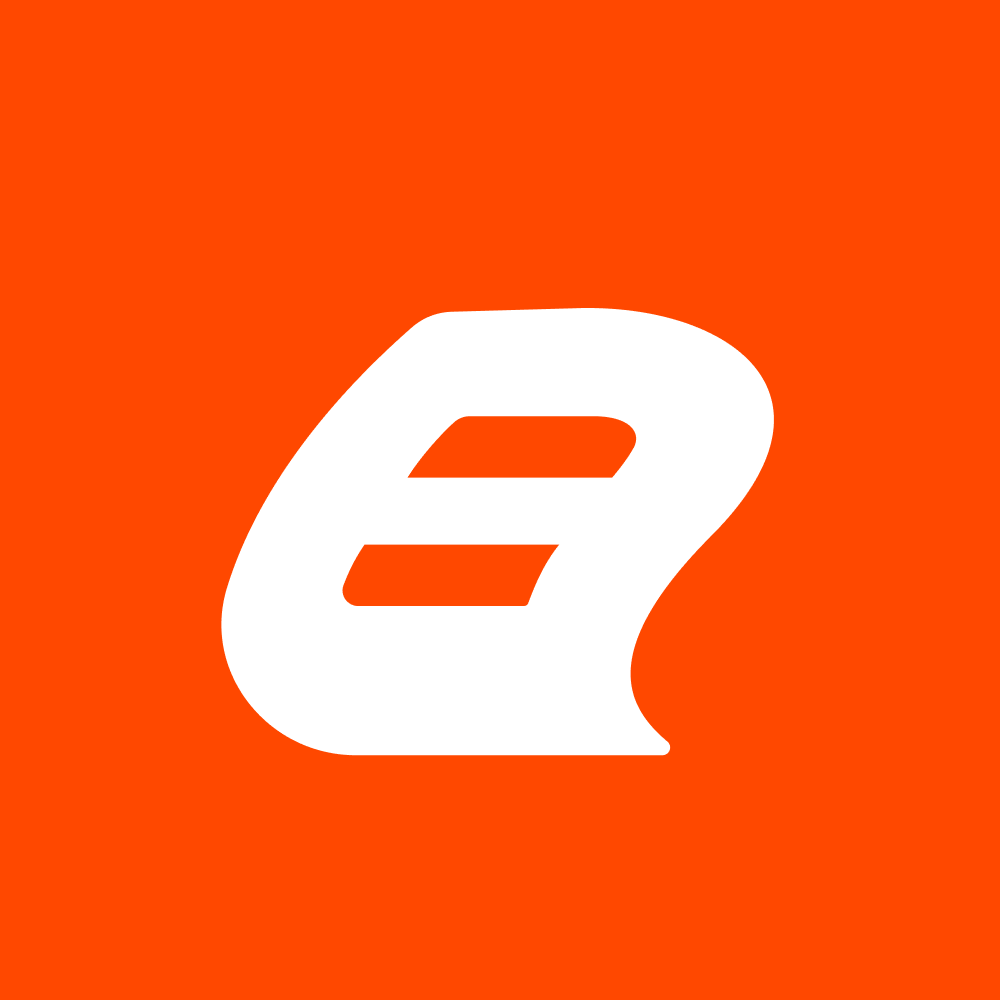