Restructuring a Laravel Project with Inertia.js, TypeScript, and React: A Case Study
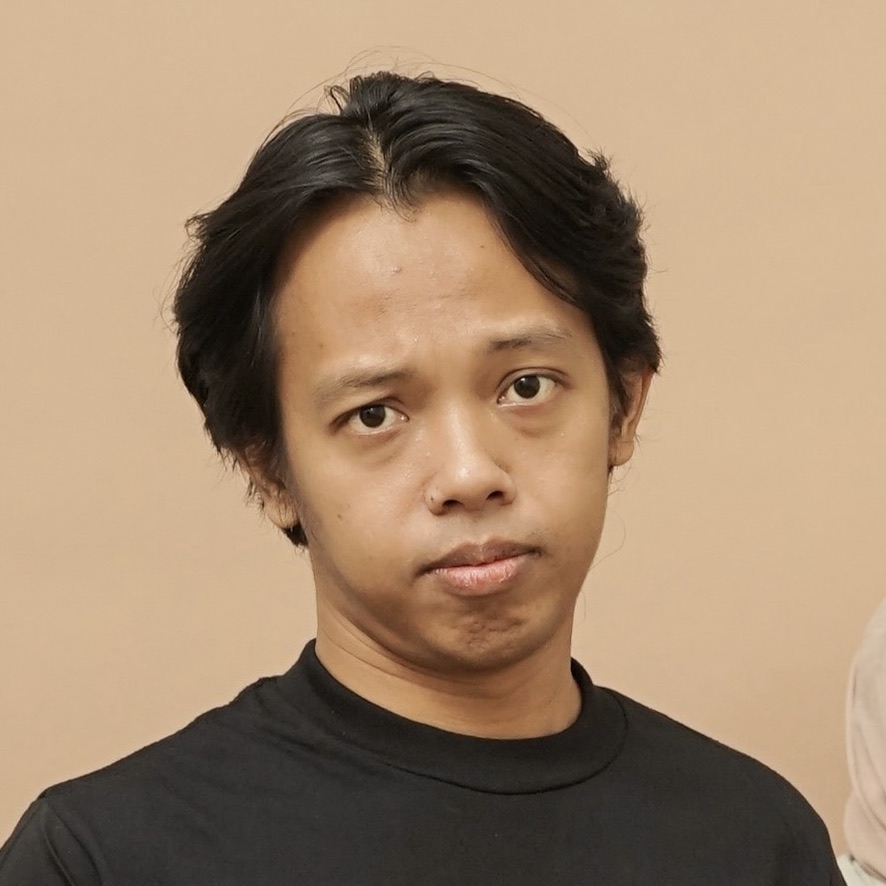
In modern web development, creating a seamless and efficient workflow for handling web requests is crucial for both developers and users. Recently, I undertook a significant restructuring of a Laravel project that integrates Inertia.js, TypeScript, and React. The primary goal was to streamline web requests, making it easier to manage and navigate components and views. Here’s a detailed look into the changes made and the reasoning behind them.
Key Changes and Their Impact
Controller Modifications: The controllers responsible for rendering views were modified to reflect the new directory structure. Here's a specific example of how rendering logic was updated:
Before:
return Inertia::render('Profile/Edit', [ 'mustVerifyEmail' => $request->user() instanceof MustVerifyEmail, 'status' => session('status'), ]);
After:
return Inertia::render('profile/page', [ 'mustVerifyEmail' => $request->user() instanceof MustVerifyEmail, 'status' => session('status'), ]);
This change ensures that the controller directly maps routes to the new view structure, making the application logic cleaner and more intuitive.
Component Renaming and Relocation:
BreezeComponents Directory: Starter components such as
ApplicationLogo
,Checkbox
,DangerButton
, and others were moved fromresources/js/Components
toresources/BreezeComponents
. This renaming not only organizes the components better but also makes it clear which components are part of the Breeze scaffolding.Layouts Relocation: Similar to components, starter layout files were moved to
resources/BreezeLayouts
, which helps in distinguishing between initial layouts and those developed further for the application.
Script and Type Definitions Organization:
- Scripts were moved from
resources/js
toresources/scripts
, and type definitions followed the same pattern. This separation aids in better organization and clearer understanding of the project's structure, especially for developers new to the project.
- Scripts were moved from
Views and Pages Reorganization:
Views Directory: The pages directory within
resources/js/Pages
was moved toresources/views/pages
. This change is significant as it aligns the directory structure with the traditional Laravel view directory, making it intuitive for Laravel developers.Specific Page Mapping: Files were renamed to reflect their specific routes. For instance,
resources/views/pages/auth/login.tsx
corresponds directly to the/login
route, andresources/views/pages/profile/page.tsx
corresponds to/profile
. This clear mapping between routes and their components simplifies the navigation and enhances code readability.
New Component and Layout Organization:
- New Components and Layouts: While starter components and layouts are housed in
resources/BreezeComponents
andresources/BreezeLayouts
, new components and layouts developed for the application will be placed inresources/views/components
andresources/views/layouts
. This ensures a clear separation between initial scaffolding and custom development, promoting better organization and scalability.
- New Components and Layouts: While starter components and layouts are housed in
Configuration Adjustments: Along with structural changes, the configuration files were also updated to reflect the new directory structure:
tailwind.config.js:
content: [ './vendor/laravel/framework/src/Illuminate/Pagination/resources/views/*.blade.php', './storage/framework/views/*.php', './resources/views/**/*.blade.php', './resources/**/*.tsx', './resources/**/*.ts', ],
tsconfig.json:
"paths": { "~/*": ["./resources/*"], "ziggy-js": ["./vendor/tightenco/ziggy"] }, "include": ["resources/**/*.ts", "resources/**/*.tsx", "resources/**/*.d.ts"]
vite.config.js:
export default defineConfig({ resolve: { alias: { '~': '/resources', }, }, plugins: [ laravel({ input: ['resources/scripts/app.tsx', 'resources/scripts/ssr.tsx'], refresh: true, }), ], });
Highlighting the Streamlined Web Requests
One of the most significant advantages of this restructuring is the streamlined handling of web requests. Here’s how the new structure enhances the development process:
Intuitive Routing: With the new structure, it’s immediately clear which component corresponds to a specific route. For example, a request to
/profile
will directly render the component located atresources/views/pages/profile/page.tsx
. This direct mapping reduces the cognitive load on developers, making it easier to trace and debug routes.Enhanced Maintainability: By organizing components, layouts, and scripts into clearly defined directories, the project becomes more maintainable. Developers can quickly locate the files they need to modify without wading through unrelated code.
Separation of Concerns: The separation between starter components/layouts and custom components/layouts enforces a clear separation of concerns. This modular approach promotes better code practices and easier updates in the future.
Ease for New Developers: The clear and intuitive structure makes it easier for new developers to join the project and get up to speed quickly. By having a well-organized codebase, early programmers can understand the project's layout and start contributing more effectively without a steep learning curve.
Conclusion
The restructuring of this Laravel project with Inertia.js, TypeScript, and React exemplifies how thoughtful organization and clear mapping of components to routes can greatly enhance a project's maintainability and developer experience. By aligning the directory structure with intuitive routing and separating concerns effectively, we’ve created a more streamlined and efficient workflow for handling web requests. This approach not only benefits current development efforts but also lays a solid foundation for future scalability and enhancements.
As we continue to refine our development practices, this case study serves as a valuable example of how structural changes can lead to significant improvements in project management and code quality. Whether you’re working on a new project or maintaining an existing one, consider how these principles can be applied to streamline your own web development processes.
Subscribe to my newsletter
Read articles from Qisthi Ramadhani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
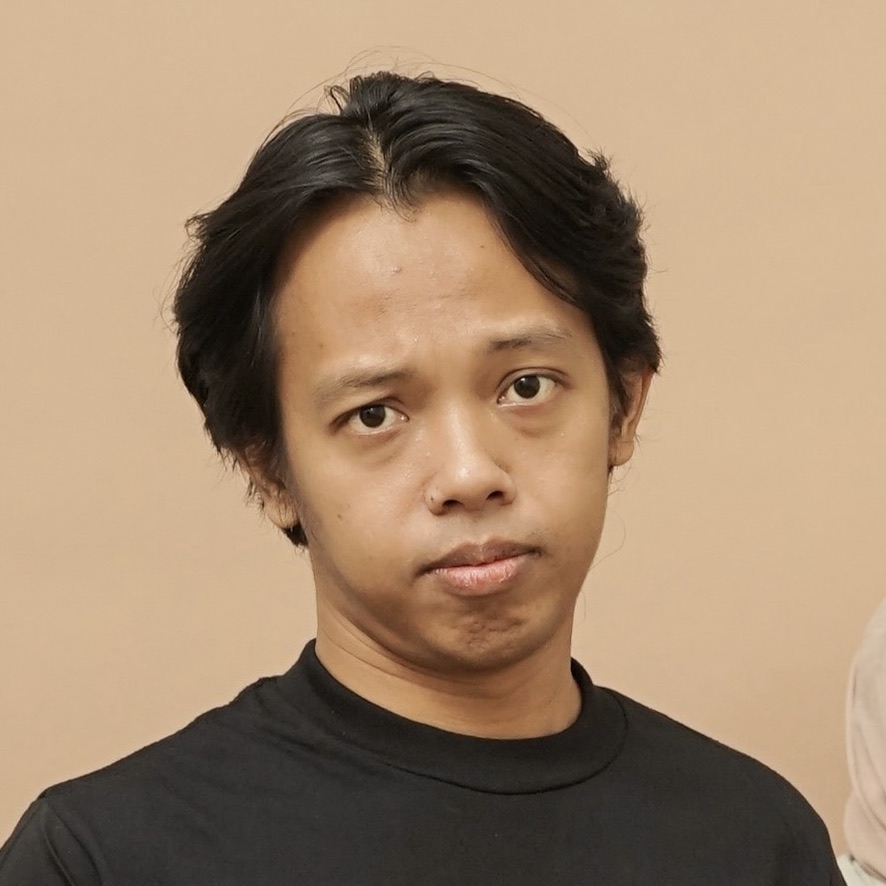
Qisthi Ramadhani
Qisthi Ramadhani
Meet Your Go-To Full Stack Developer: Driven by Passion to Forge Flawless Web Experiences