Database Indexing - Why it's matter?

Table of contents
- Improve database with proper indexing
- 1. Analyze Your Queries
- 2. Identify Candidates for Indexing
- 3. Use the Right Type of Index
- 4. Avoid Over-Indexing
- 5. Use Indexes for Full-Text Search
- 6. Regular Maintenance
- Monitoring and Tuning Indexes
- Implementation in Laravel
- Example: Improving Indexing Strategy
- 1. Indexing Key Columns
- 2. Improving Full-Text Search
- 3. Optimizing Join Operations
- 4. Caching Frequent Queries
- 5. Regular Maintenance
- Example Scenario: Improving Product Retrieval
- Conclusion
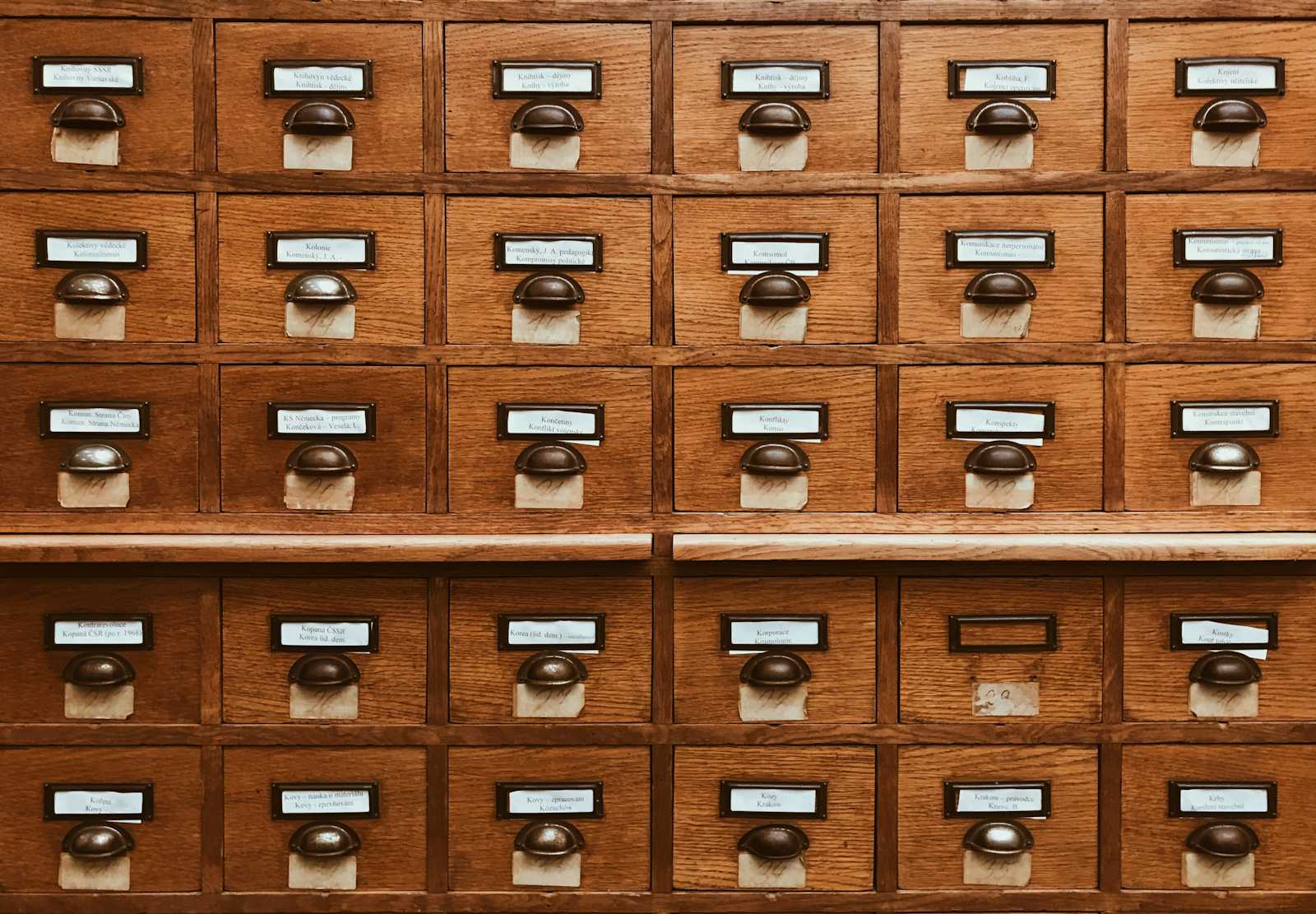
Database indexing is crucial in Laravel application development for several reasons:
Performance Improvement:
Faster Query Execution: Indexes allow the database to find and retrieve specific rows much faster than it could without them. This significantly reduces the time it takes to execute queries, especially on large datasets.
Efficient Data Retrieval: With indexes, the database can quickly locate the data without scanning the entire table, resulting in quicker response times for SELECT queries.
Optimized Resource Usage:
Reduced CPU and Memory Usage: Since indexes make data retrieval more efficient, they help reduce the load on the CPU and memory. This optimization is crucial for maintaining the overall performance of the application, particularly under heavy load.
Lower I/O Operations: Indexes decrease the number of disk I/O operations required to retrieve data, which is beneficial for improving performance in environments where disk speed is a bottleneck.
Enhanced User Experience:
Responsive Applications: Fast data retrieval directly impacts the responsiveness of an application. Users experience quicker load times and smoother interactions, which is essential for maintaining user satisfaction and engagement.
Scalability: Efficient indexing allows an application to scale better. As the dataset grows, well-indexed databases can handle increased traffic and larger volumes of data without significant performance degradation.
Supporting Complex Queries:
Efficient Sorting and Filtering: Indexes are beneficial for operations that involve sorting and filtering data. They help optimize ORDER BY and WHERE clauses, making these operations more efficient.
Joins Optimization: Indexes can significantly speed up JOIN operations by quickly locating the matching rows in related tables, thus improving the performance of complex queries involving multiple tables.
Best Practices and Maintenance:
Index Management: Laravel’s Eloquent ORM provides methods to create and manage indexes easily. Properly managing indexes (adding, updating, or removing them as needed) ensures the database remains optimized.
Avoiding Over-Indexing: While indexes are beneficial, having too many can lead to performance issues during INSERT, UPDATE, and DELETE operations. It’s important to balance the number of indexes to maintain optimal performance for both read and write operations.
Improve database with proper indexing
Improving your database with proper indexing involves understanding the specific needs of your application and the queries that are being executed most frequently. Here are steps and best practices to guide you through the process:
1. Analyze Your Queries
Identify the queries that are run most frequently and those that have the highest impact on performance. Use tools and techniques like query logs, profiling, and monitoring tools to gather this information.
2. Identify Candidates for Indexing
Focus on the following types of columns when considering which to index:
Primary Keys: These are often indexed automatically by the database.
Foreign Keys: Indexing foreign keys can speed up joins and improve referential integrity checks.
Columns in WHERE Clauses: Columns frequently used in WHERE clauses can benefit significantly from indexing.
Columns in JOIN Conditions: Columns that are used in JOIN operations.
Columns in ORDER BY and GROUP BY Clauses: Indexing these can speed up sorting and grouping operations.
Columns used in Aggregate Functions: Such as COUNT(), AVG(), SUM().
3. Use the Right Type of Index
Single-Column Index: Simple and useful for columns that are often queried alone.
Composite Index: Useful for queries that filter or sort by multiple columns. The order of columns in the index should match the order of columns in your queries.
Unique Index: Ensures that all values in the index are unique. This is automatically created for primary keys.
4. Avoid Over-Indexing
While indexes can speed up read operations, they can slow down write operations (INSERT, UPDATE, DELETE). Avoid creating unnecessary indexes that won’t be used frequently.
5. Use Indexes for Full-Text Search
For columns that store large text values and need full-text search capabilities, consider using full-text indexes.
6. Regular Maintenance
Monitor and Analyze Index Usage: Regularly check which indexes are being used and which are not. Remove unused indexes.
Rebuild and Reorganize Indexes: Over time, indexes can become fragmented. Periodically rebuild or reorganize them to maintain performance.
Monitoring and Tuning Indexes
Use Database-Specific Tools:
MySQL:
EXPLAIN
,SHOW INDEXES
, and the slow query log.PostgreSQL:
EXPLAIN ANALYZE
,pg_stat_user_indexes
, andpg_stat_all_indexes
.
Use Laravel Debugging Tools:
Laravel Telescope: Provides insights into queries and their execution times.
Laravel Debugbar: Offers a comprehensive view of the executed queries during a request.
Implementation in Laravel
In Laravel, you can create indexes using migrations, which makes the process straightforward and maintains consistency in your database schema. Here are some examples:
Creating an Index:
Schema::table('users', function (Blueprint $table) { $table->index('email'); });
Creating a Unique Index:
Schema::table('users', function (Blueprint $table) { $table->unique('email'); });
Creating a Composite Index:
Schema::table('users', function (Blueprint $table) { $table->index(['first_name', 'last_name']); }); // another example Schema::table('orders', function (Blueprint $table) { $table->index(['user_id', 'product_id']); });
Dropping an Index
Schema::table('users', function (Blueprint $table) { $table->dropIndex(['email']); // drops index 'users_email_index' });
Full-Text Index (if supported by your database)
Schema::table('posts', function (Blueprint $table) { $table->fullText('content'); });
Example: Improving Indexing Strategy
Consider we have an e-commerce application, Now let's make some improvement of this application Database.
Improving the database for an e-commerce Laravel application involves implementing a well-thought-out indexing strategy and optimizing database design. Here are some practical examples to illustrate this:
1. Indexing Key Columns
Products Table
Query: Retrieve products by category.
SELECT * FROM products WHERE category_id = 1;
Index:
category_id
Schema::table('products', function (Blueprint $table) { $table->index('category_id'); });
Orders Table
Query: Fetch all orders for a specific user, sorted by date.
SELECT * FROM orders WHERE user_id = 1 ORDER BY created_at DESC;
Index: Composite index on
user_id
andcreated_at
Schema::table('orders', function (Blueprint $table) { $table->index(['user_id', 'created_at']); });
Users Table
Query: Find a user by email.
SELECT * FROM users WHERE email = 'example@example.com';
Index:
email
Schema::table('users', function (Blueprint $table) { $table->unique('email'); });
2. Improving Full-Text Search
Products Table
Query: Search products by name or description.
SELECT * FROM products WHERE MATCH(name, description) AGAINST('laptop');
Index: Full-text index on
name
anddescription
Schema::table('products', function (Blueprint $table) { $table->fullText(['name', 'description']); });
3. Optimizing Join Operations
Orders and Products Tables
Query: Retrieve order details with product information.
SELECT orders.*, products.* FROM orders JOIN order_product ON orders.id = order_product.order_id JOIN products ON order_product.product_id = products.id WHERE orders.user_id = 1;
Index: Foreign key indexes on
order_product
tableSchema::table('order_product', function (Blueprint $table) { $table->index('order_id'); $table->index('product_id'); });
4. Caching Frequent Queries
Query: Retrieve a list of featured products.
SELECT * FROM products WHERE is_featured = 1;
Cache: Use Laravel’s caching mechanism to store the result.
$featuredProducts = Cache::remember('featured_products', 3600, function () { return Product::where('is_featured', 1)->get(); });
5. Regular Maintenance
Check how queries are perform: By using
EXPLAIN
, you can gain insights into how MySQL executes your queries and make informed decisions to optimize them.Like we have an query like this, now before the query just put a keyword
EXPLAIN
it will show the result how your query is executed.SELECT * FROM products WHERE category_id = 1 AND price BETWEEN 100 AND 500 AND MATCH(name, description) AGAINST('laptop');
EXPLAIN SELECT * FROM products WHERE category_id = 1 AND price BETWEEN 100 AND 500 AND MATCH(name, description) AGAINST('laptop');
Monitor Index Usage: Regularly check which indexes are being used and drop unused ones.
SHOW INDEX FROM products;
Rebuild Indexes: Periodically rebuild indexes to reduce fragmentation.
OPTIMIZE TABLE products;
Example Scenario: Improving Product Retrieval
Consider a scenario where customers frequently search for products based on various filters such as category, price range, and name. Here's how to optimize it:
Products Table Structure
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('description');
$table->unsignedBigInteger('category_id');
$table->decimal('price', 8, 2);
$table->boolean('is_featured')->default(false);
$table->timestamps();
$table->index('category_id');
$table->index('price');
$table->fullText(['name', 'description']);
});
Sample Query and Indexes
Query: Filter products by category and price, and search by name.
SELECT * FROM products WHERE category_id = 1 AND price BETWEEN 100 AND 500 AND MATCH(name, description) AGAINST('laptop');
With the above indexes, this query will be optimized for filtering by category and price, and searching by product name and description.
Conclusion
In conclusion, proper database indexing is essential for optimizing the performance of Laravel applications. By carefully analyzing query patterns and identifying which columns to index, developers can significantly enhance the speed and efficiency of data retrieval operations. This not only improves application responsiveness but also ensures better resource utilization, leading to a more scalable and robust system. Laravel’s migration tools make it straightforward to implement and manage indexes, allowing for easy adjustments as application requirements evolve.
However, it is crucial to strike a balance to avoid over-indexing, which can degrade performance during write operations. Regular monitoring and maintenance of indexes are necessary to ensure they continue to meet the application's needs effectively. By leveraging database-specific tools and Laravel's debugging utilities, developers can fine-tune their indexing strategy to sustain optimal performance. Ultimately, a well-planned indexing approach can dramatically enhance user experience and support the long-term success of a Laravel application.
To know how indexing works, you may see this article also.
End of this Series.
Subscribe to my newsletter
Read articles from Saiful Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saiful Alam
Saiful Alam
An Expert software engineer in Laravel and React. Creates robust backends and seamless user interfaces. Committed to clean code and efficient project delivery, In-demand for delivering excellent user experiences.