Daily Hack #day85 - Redis Backup and Recovery
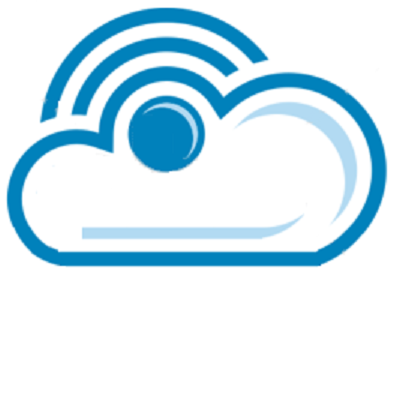
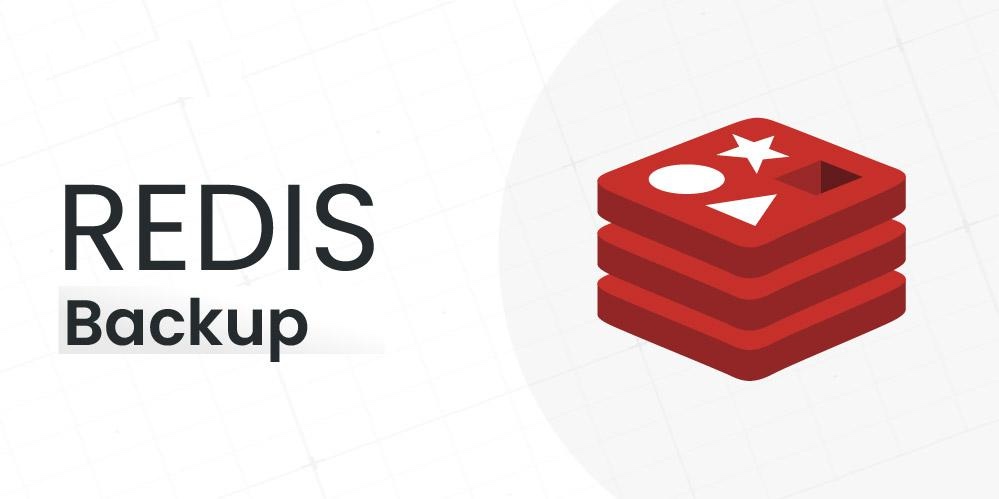
The shell script below automates the backup and recovery process for a Redis database. This script will create a backup of the Redis database and allow you to restore it from the backup.
Redis Backup and Recovery Script
#!/bin/bash
# Configuration
REDIS_CLI="/usr/bin/redis-cli"
BACKUP_DIR="/var/backups/redis"
TIMESTAMP=$(date +'%Y%m%d%H%M%S')
BACKUP_FILE="$BACKUP_DIR/redis_backup_$TIMESTAMP.rdb"
LOG_FILE="/var/log/redis_backup_recovery.log"
# Function to log messages
log_message() {
echo "$(date +'%Y-%m-%d %H:%M:%S') - $1" | tee -a $LOG_FILE
}
# Function to create a backup
backup_redis() {
log_message "Starting Redis backup"
# Create backup directory if it doesn't exist
mkdir -p $BACKUP_DIR
# Trigger Redis to create a backup
$REDIS_CLI SAVE
# Check if the SAVE command was successful
if [ $? -ne 0 ]; then
log_message "Failed to trigger Redis backup"
exit 1
fi
# Copy the dump file to the backup directory with a timestamp
cp /var/lib/redis/dump.rdb $BACKUP_FILE
# Verify the backup file was created successfully
if [ $? -eq 0 ]; then
log_message "Redis backup created successfully at $BACKUP_FILE"
else
log_message "Failed to create Redis backup file"
exit 1
fi
}
# Function to restore from a backup
restore_redis() {
BACKUP_TO_RESTORE=$1
if [ ! -f $BACKUP_TO_RESTORE ]; then
log_message "Backup file $BACKUP_TO_RESTORE does not exist"
exit 1
fi
log_message "Starting Redis restore from $BACKUP_TO_RESTORE"
# Stop Redis server
systemctl stop redis
# Check if the Redis server stopped successfully
if [ $? -ne 0 ]; then
log_message "Failed to stop Redis server"
exit 1
fi
# Copy the backup file to the Redis data directory
cp $BACKUP_TO_RESTORE /var/lib/redis/dump.rdb
# Verify the backup file was copied successfully
if [ $? -eq 0 ]; then
log_message "Backup file copied to Redis data directory"
else
log_message "Failed to copy backup file"
exit 1
fi
# Start Redis server
systemctl start redis
# Check if the Redis server started successfully
if [ $? -eq 0 ]; then
log_message "Redis restored successfully from $BACKUP_TO_RESTORE"
else
log_message "Failed to start Redis server"
exit 1
fi
}
# Main script logic
case $1 in
backup)
backup_redis
;;
restore)
if [ -z "$2" ]; then
echo "Usage: $0 restore <backup_file>"
exit 1
fi
restore_redis $2
;;
*)
echo "Usage: $0 {backup|restore <backup_file>}"
exit 1
;;
esac
exit 0
Explaining the script steps
Configuration:
REDIS_CLI
: Path to theredis-cli
executable.BACKUP_DIR
: Directory where backups will be stored.TIMESTAMP
: Current timestamp to uniquely name backup files.BACKUP_FILE
: Full path to the backup file.LOG_FILE
: Log file to record backup and restore operations.
Logging Function:
log_message()
: Logs messages with timestamps to the log file.
Backup Function:
backup_redis()
: Creates a backup of the Redis database.Triggers the Redis
SAVE
command to create a backup.Copies the
dump.rdb
file to the backup directory with a timestamp.
Restore Function:
restore_redis()
: Restores the Redis database from a specified backup file.Stops the Redis server.
Copies the specified backup file to the Redis data directory.
Starts the Redis server.
Main Script Logic:
Uses a
case
statement to handlebackup
andrestore
commands.backup
: Calls thebackup_redis
function.restore
: Calls therestore_redis
function with the specified backup file.
Usage
Make the script executable:
chmod +x redis_backup_recovery.sh
Run the backup command:
./redis_backup_recovery.sh backup
Run the restore command:
./redis_backup_recovery.sh restore /path/to/backup_file
Scheduling with Cron
To automate the backup process, you can schedule the script using cron:
Edit the crontab:
crontab -e
Add a cron job to run the script daily at 2 AM:
0 2 * * * /path/to/redis_backup_recovery.sh backup
Make sure to replace /path/to/redis_backup_recovery.sh
with the actual path to your script.
This script automates the process of backing up and restoring a Redis database, providing a reliable way to manage Redis data.
Subscribe to my newsletter
Read articles from Cloud Tuned directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
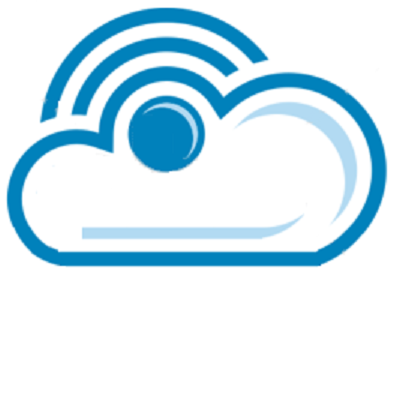