Effectively Using useEffect Hook

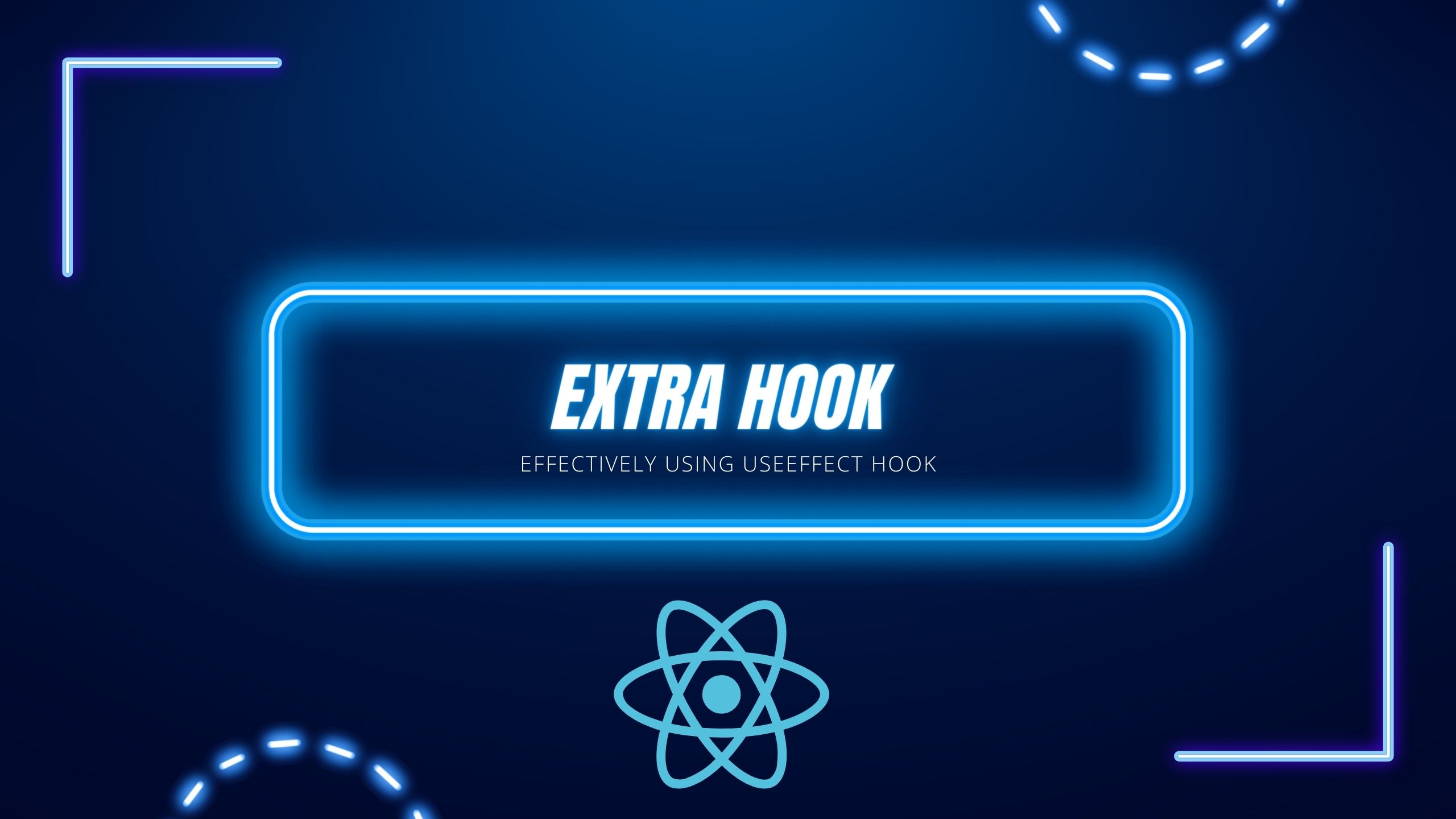
Managing Side Effects
The primary role of the useEffect hook is to manage side effects within React applications efficiently. Side effects include tasks such as fetching data from an API, directly manipulating the DOM, and setting up subscriptions. By encapsulating these effects within useEffect, developers ensure that these operations are performed at the right moments in the component lifecycle, leading to cleaner and more predictable code.
Enhancing Application Performance
Proper use of useEffect can significantly enhance application performance. It allows developers to control when and how side effects are executed, reducing unnecessary operations and preventing performance bottlenecks. For instance, by specifying dependencies, useEffect can be configured to run only when certain state variables change, avoiding redundant operations and keeping the application responsive.
Basic Usage of useEffect
Setting Up useEffect
Syntax and Structure
The syntax of useEffect is straightforward yet powerful. It takes two arguments: a function that contains the side effect logic and an optional dependency array. The function executes the side effect, while the dependency array determines when the effect should re-run.
useEffect(() => {
// Side effect logic
}, [dependencies]);
Example: Logging to the Console
A simple use case for useEffect is logging to the console whenever a component mounts or updates. This can be useful for debugging purposes.
import React, { useEffect } from 'react';
function LoggerComponent() {
useEffect(() => {
console.log('Component mounted or updated');
});
return <div>Check the console</div>;
}
Dependency Array Explained
Role of the Dependency Array
The dependency array is crucial for optimizing useEffect. It controls when the effect should run by specifying which state or props the effect depends on. If any value in this array changes, the effect will re-run. If the array is empty, the effect runs only once after the initial render.
Examples of Different Dependency Scenarios
No dependency array: The effect runs after every render.
Empty dependency array: The effect runs only once, after the initial render.
Specific dependencies: The effect runs only when specified values change.
useEffect(() => {
// Effect runs only once
}, []);
useEffect(() => {
// Effect runs when 'count' changes
}, [count]);
Cleaning Up with useEffect
Why Cleanup is Necessary
Cleanup is essential to avoid memory leaks and ensure that side effects do not persist beyond their intended lifecycle. This is particularly important for effects like subscriptions and timers, which need to be explicitly stopped or cleared.
Example: Clearing Timers and Subscriptions
import React, { useEffect } from 'react';
function TimerComponent() {
useEffect(() => {
const timer = setInterval(() => {
console.log('Timer running');
}, 1000);
return () => clearInterval(timer); // Cleanup
}, []);
return <div>Timer is running, check the console</div>;
}
Advanced useEffect Techniques
Conditional Execution of useEffect
Implementing Conditional Logic
Conditional logic within useEffect allows for more precise control over when effects should run. By incorporating conditions within the effect function or the dependency array, developers can fine-tune the execution to match specific criteria.
Example: Fetching Data Conditionally
import React, { useState, useEffect } from 'react';
function DataFetcher({ userId }) {
const [data, setData] = useState(null);
useEffect(() => {
if (userId) {
fetch(`https://api.example.com/user/${userId}`)
.then(response => response.json())
.then(data => setData(data));
}
}, [userId]);
return data ? <div>{data.name}</div> : <div>Loading...</div>;
}
Multiple useEffect Hooks
Using Multiple useEffect Calls in a Component
Using multiple useEffect hooks in a single component can help separate concerns and organize side effects more cleanly. Each useEffect can focus on a specific effect, making the code more modular and easier to maintain.
Example: Separating Concerns in useEffect
import React, { useEffect, useState } from 'react';
function MultiEffectComponent() {
const [data, setData] = useState(null);
const [isOnline, setIsOnline] = useState(false);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
useEffect(() => {
function handleStatusChange(status) {
setIsOnline(status.isOnline);
}
window.addEventListener('online', () => handleStatusChange({ isOnline: true }));
window.addEventListener('offline', () => handleStatusChange({ isOnline: false }));
return () => {
window.removeEventListener('online', handleStatusChange);
window.removeEventListener('offline', handleStatusChange);
};
}, []);
return (
<div>
<p>Data: {data}</p>
<p>Status: {isOnline ? 'Online' : 'Offline'}</p>
</div>
);
}
Using useEffect with Async/Await
Handling Asynchronous Operations
Handling asynchronous operations with useEffect requires attention to promise handling and potential cleanup to avoid memory leaks or unexpected behavior. Using async/await within useEffect can simplify dealing with asynchronous code.
Example: Fetching Data from an API
import React, { useState, useEffect } from 'react';
function AsyncDataFetcher() {
const [data, setData] = useState(null);
useEffect(() => {
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
}
fetchData();
}, []);
return data ? <div>{data.name}</div> : <div>Loading...</div>;
}
Common Patterns and Practices
Data Fetching with useEffect
Best Practices for Fetching Data
When fetching data with useEffect, it's essential to handle loading states, errors, and cleanup properly. This ensures that the component remains responsive and free of bugs.
Example: Displaying Data from an API
import React, { useState, useEffect } from 'react';
function DataComponent() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(result => {
setData(result);
setLoading(false);
})
.catch(error => {
setError(error);
setLoading(false);
});
}, []);
if (loading) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
return <div>{data.name}</div>;
}
useEffect for Event Listeners
Adding and Removing Event Listeners
Managing event listeners with useEffect ensures they are set up and torn down correctly, preventing memory leaks and ensuring the component behaves as expected.
Example: Handling Window Resize Events
import React, { useState, useEffect } from 'react';
function ResizeComponent() {
const [windowWidth, setWindowWidth] = useState(window.innerWidth);
useEffect(() => {
function handleResize() {
setWindowWidth(window.innerWidth);
}
window.addEventListener('resize', handleResize);
return () => {
window.removeEventListener('resize', handleResize);
};
}, []);
return <div>Window width: {windowWidth}</div>;
}
useEffect for Subscriptions
Managing Subscriptions Effectively
Subscriptions to data streams or other sources should be handled carefully to avoid leaks. useEffect's cleanup function is ideal for unsubscribing when the component unmounts.
Example: WebSocket Connections
import React, { useState, useEffect } from 'react';
function WebSocketComponent() {
const [messages, setMessages] = useState([]);
useEffect(() => {
const socket = new WebSocket('ws://example.com/socket');
socket.onmessage = (event) => {
setMessages(prevMessages => [...prevMessages, event.data]);
};
return () => {
socket.close();
};
}, []);
return (
<div>
{messages.map((msg, index) => (
<p key={index}>{msg}</p>
))}
</div>
);
}
Performance Considerations
Optimizing useEffect Usage
Minimizing Unnecessary Re-renders
To optimize performance, ensure that useEffect dependencies are accurate and avoid re-renders when not needed. This prevents performance issues in larger applications.
Example: Using Memoization with useEffect
import React, { useState, useEffect, useMemo } from 'react';
function OptimizedComponent({ userId }) {
const [data, setData] = useState(null);
const fetchData = useMemo(() => {
return async () => {
const response = await fetch(`https://api.example.com/user/${userId}`);
const result = await response.json();
setData(result);
};
}, [userId]);
useEffect(() => {
fetchData();
}, [fetchData]);
return data ? <div>{data.name}</div> : <div>Loading...</div>;
}
Debouncing and Throttling in use
Effect
Techniques for Performance Optimization
Debouncing and throttling are techniques used to limit the frequency of function execution, which is crucial for optimizing performance in certain scenarios, such as input handling or scrolling.
Example: Implementing Debounce Logic
import React, { useState, useEffect } from 'react';
function DebouncedInput() {
const [value, setValue] = useState('');
const [debouncedValue, setDebouncedValue] = useState(value);
useEffect(() => {
const handler = setTimeout(() => {
setDebouncedValue(value);
}, 500);
return () => {
clearTimeout(handler);
};
}, [value]);
return (
<div>
<input value={value} onChange={(e) => setValue(e.target.value)} />
<p>Debounced value: {debouncedValue}</p>
</div>
);
}
Debugging useEffect Issues
Common Bugs and Solutions
Identifying Typical useEffect Problems
Common issues with useEffect include infinite loops, stale closures, and incorrect dependencies. Identifying and resolving these problems is key to robust application performance.
Example: Infinite Loops in useEffect
Infinite loops occur when an effect continually re-runs due to a state change inside the effect, causing another state change and so on.
import React, { useState, useEffect } from 'react';
function InfiniteLoopComponent() {
const [count, setCount] = useState(0);
useEffect(() => {
setCount(count + 1); // This will cause an infinite loop
}, [count]);
return <div>Count: {count}</div>;
}
Tools for Debugging useEffect
Utilizing React DevTools
React DevTools can be invaluable for debugging useEffect issues by providing insights into component renders and dependency changes.
Example: Inspecting Dependency Arrays
Using React DevTools, you can inspect which components are re-rendering and why, helping to pinpoint dependency array issues.
Real-World Applications
useEffect in Form Handling
Managing Form State and Side Effects
Forms often require managing state and side effects, such as validation or API calls. useEffect is ideal for these tasks.
Example: Validating Form Inputs
import React, { useState, useEffect } from 'react';
function FormComponent() {
const [input, setInput] = useState('');
const [isValid, setIsValid] = useState(false);
useEffect(() => {
const validateInput = () => {
setIsValid(input.length > 3);
};
validateInput();
}, [input]);
return (
<div>
<input value={input} onChange={(e) => setInput(e.target.value)} />
<p>{isValid ? 'Valid' : 'Invalid'}</p>
</div>
);
}
useEffect in Authentication
Handling Authentication Flows
Authentication often involves managing tokens, sessions, and side effects related to user state. useEffect simplifies these processes.
Example: Managing Tokens and Sessions
import React, { useState, useEffect } from 'react';
function AuthComponent() {
const [token, setToken] = useState(null);
useEffect(() => {
const storedToken = localStorage.getItem('token');
if (storedToken) {
setToken(storedToken);
}
const handleTokenChange = (newToken) => {
setToken(newToken);
localStorage.setItem('token', newToken);
};
window.addEventListener('tokenChange', handleTokenChange);
return () => {
window.removeEventListener('tokenChange', handleTokenChange);
};
}, []);
return <div>Token: {token}</div>;
}
Best Practices and Tips
Clear and Concise Dependency Arrays
Ensuring Accurate Dependencies
Accurate dependency arrays prevent unnecessary re-renders and ensure that effects only run when intended. Keeping them clear and concise is essential for optimal performance.
Example: Avoiding Unnecessary Dependencies
useEffect(() => {
// Effect logic here
}, [essentialDep1, essentialDep2]); // Only include necessary dependencies
Structuring Components for Better useEffect Management
Organizing Components for Clarity
Well-structured components make managing useEffect simpler and more effective. Separating concerns and breaking down complex logic into smaller, reusable components is key.
Example: Refactoring to Improve Readability
function ParentComponent() {
return (
<div>
<ChildComponent />
<AnotherChildComponent />
</div>
);
}
function ChildComponent() {
useEffect(() => {
// Child-specific effect
}, []);
return <div>Child Component</div>;
}
function AnotherChildComponent() {
useEffect(() => {
// Another child-specific effect
}, []);
return <div>Another Child Component</div>;
}
Avoiding Common Pitfalls
Preventing Common useEffect Mistakes
Common pitfalls with useEffect include stale closures, incorrect dependencies, and overcomplicating logic. Awareness and careful coding can prevent these issues.
Example: Handling State Updates Correctly
import React, { useState, useEffect } from 'react';
function CounterComponent() {
const [count, setCount] = useState(0);
useEffect(() => {
const handleClick = () => {
setCount(prevCount => prevCount + 1); // Use functional update to avoid stale closures
};
document.addEventListener('click', handleClick);
return () => {
document.removeEventListener('click', handleClick);
};
}, []);
return <div>Count: {count}</div>;
}
Conclusion
Recap of Key Concepts
In summary, the useEffect hook is a versatile and powerful tool for managing side effects in React functional components. From basic setup to advanced techniques, mastering useEffect is essential for building robust and performant React applications.
Further Reading and Resources
For further learning, consider exploring the following resources:
Deepening your understanding of useEffect and other hooks will greatly enhance your React development skills and enable you to create more efficient, maintainable applications.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
R3F | Laravel | Babylon | Three.js | WordPress Performance Enthusiast