Enhancing Network Security: A Comprehensive Guide for Developers

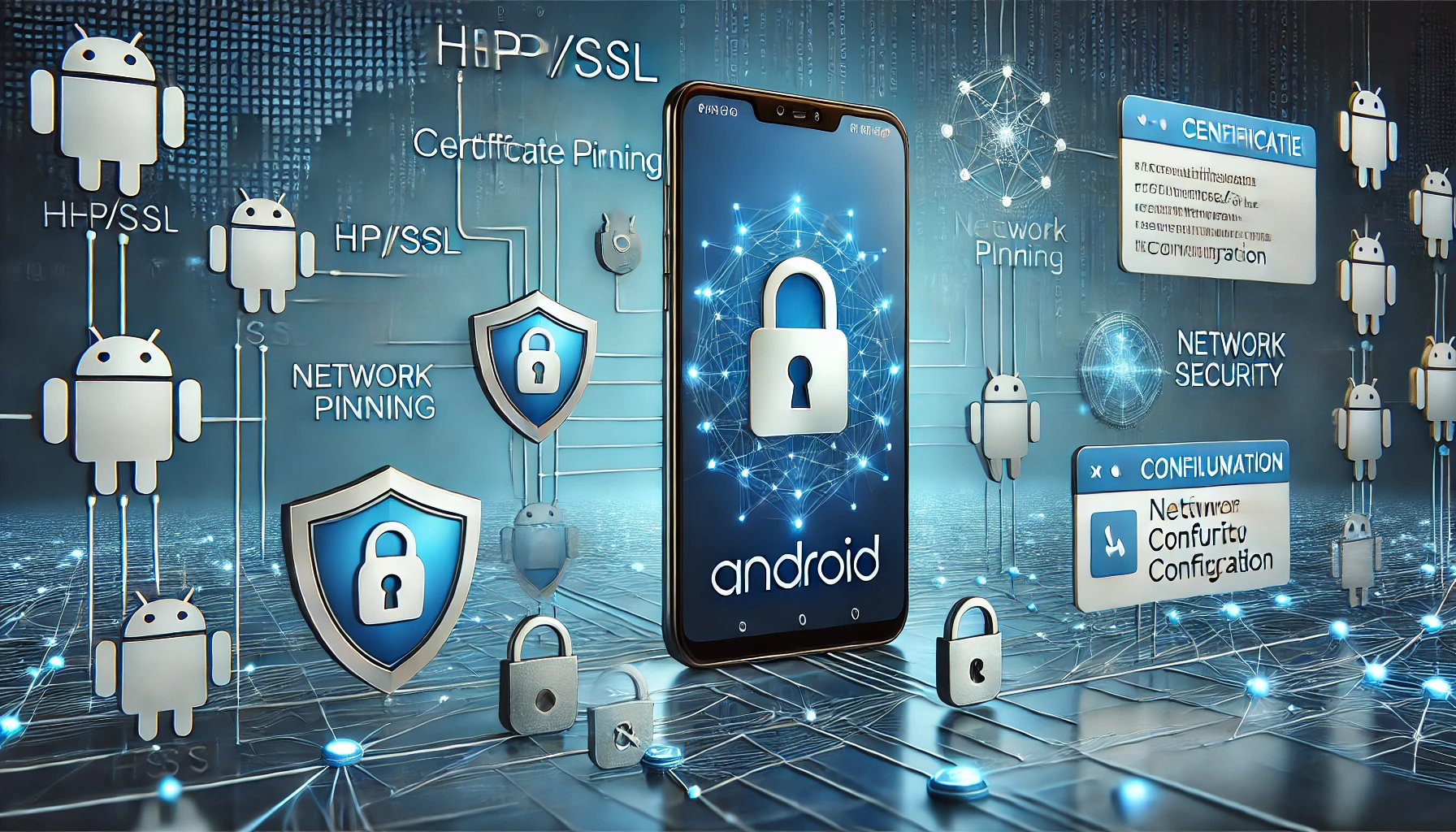
Hello, fellow developers! 👋 Today, we’re diving deep into the crucial world of network security. Ensuring secure communication in our apps is a top priority, and we'll explore some practical and advanced steps you can take to achieve this. So, let’s get started! 🚀
Secure Communication with HTTPS and SSL/TLS
What is HTTPS and SSL/TLS?
HTTPS (HyperText Transfer Protocol Secure) is an extension of HTTP and uses SSL/TLS (Secure Sockets Layer/Transport Layer Security) to encrypt data transmitted over the internet. This ensures that the data exchanged between a client and a server is secure and cannot be intercepted by third parties.
How to Implement HTTPS in Your App
Server Configuration:
Ensure your server supports SSL/TLS.
Obtain an SSL certificate from a trusted Certificate Authority (CA) like Let's Encrypt, Comodo, or DigiCert.
Install the SSL certificate on your server.
Client Configuration:
Ensure all API endpoints use HTTPS.
Configure your network libraries (e.g., OkHttp, Retrofit) to use HTTPS.
Here’s a basic example using OkHttp:
kotlinCopy codeval client = OkHttpClient.Builder()
.sslSocketFactory(sslSocketFactory, trustManager)
.hostnameVerifier { hostname, session ->
HttpsURLConnection.getDefaultHostnameVerifier().verify("yourserver.com", session)
}
.build()
Advantages of Using HTTPS and SSL/TLS
Encryption: Protects data from eavesdroppers.
Data Integrity: Ensures data is not tampered with during transmission.
Authentication: Verifies the identity of the communicating parties.
Implementing Certificate Pinning
What is Certificate Pinning?
Certificate pinning is a technique that helps prevent man-in-the-middle attacks by hardcoding the server’s certificate or public key into the client application. This way, even if a CA is compromised, the app will only trust the pinned certificate.
How to Implement Certificate Pinning
Using OkHttp in Android:
Extract the Public Key Hash:
Obtain your server’s SSL certificate.
Extract the public key hash using a tool like OpenSSL.
bashCopy codeopenssl x509 -in yourserver_cert.pem -pubkey -noout | \
openssl rsa -pubin -outform der | \
openssl dgst -sha256 -binary | base64
Configure Certificate Pinning in OkHttp:
kotlinCopy codeval certificatePinner = CertificatePinner.Builder() .add("yourserver.com", "sha256/AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA=") .build() val client = OkHttpClient.Builder() .certificatePinner(certificatePinner) .build()
Advantages of Certificate Pinning
Enhanced Security: Protects against compromised CAs.
Prevent Man-in-the-Middle Attacks: Ensures the client connects to the intended server.
Using Network Security Configuration
What is Network Security Configuration?
Network Security Configuration is a feature in Android that allows developers to configure network security settings in a declarative way using an XML file. This simplifies the management of security configurations.
How to Use Network Security Configuration
Create the Configuration File:
- Create a
network_security_config.xml
file in theres/xml
directory.
- Create a
xmlCopy code<network-security-config>
<domain-config cleartextTrafficPermitted="false">
<domain includeSubdomains="true">yourserver.com</domain>
<pin-set expiration="2024-06-27">
<pin digest="SHA-256">AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA=</pin>
</pin-set>
</domain-config>
</network-security-config>
Reference the Configuration in Your Manifest:
- Add the following to your
AndroidManifest.xml
:
- Add the following to your
xmlCopy code<application
android:networkSecurityConfig="@xml/network_security_config"
... >
</application>
Advantages of Network Security Configuration
Ease of Management: Centralizes network security settings.
Flexibility: Supports cleartext traffic policies, certificate pinning, and more.
Declarative Approach: Reduces code complexity and potential errors.
Handling Insecure Network Connections
Why Handle Insecure Connections?
Despite your best efforts, users might still encounter insecure networks. Handling these connections gracefully is essential to maintaining security and providing a good user experience.
Detecting Insecure Connections
To detect insecure connections, you need to check if the connection is using HTTP instead of HTTPS. Here’s a basic example of how you can do this in an Android app:
kotlinCopy codeval url = URL("http://example.com")
val urlConnection = url.openConnection() as HttpURLConnection
if (urlConnection.url.protocol == "http") {
// Alert the user about insecure connection
showInsecureConnectionAlert()
} else {
// Proceed with secure connection
urlConnection.connect()
}
Handling Insecure Connections Gracefully
Alert the User:
Inform users when they are on an insecure connection.
Provide options to proceed with caution or switch to a secure network.
Example:
kotlinCopy codefun showInsecureConnectionAlert() {
AlertDialog.Builder(this)
.setTitle("Insecure Connection")
.setMessage("You are about to connect over an insecure connection. This may expose your data to third parties. Do you want to proceed?")
.setPositiveButton("Proceed") { dialog, which ->
// User chose to proceed
}
.setNegativeButton("Cancel") { dialog, which ->
// User chose to cancel
}
.show()
}
Fallback to Secure Alternatives:
- If the connection is insecure, try to provide secure alternatives such as connecting through a VPN or using a different network.
kotlinCopy codeif (isNetworkInsecure()) {
// Suggest using a VPN or switching to a different network
suggestSecureNetworkOptions()
}
Disable Certain Features:
- Disable features that require secure connections when an insecure connection is detected.
kotlinCopy codeif (isNetworkInsecure()) {
disableSensitiveFeatures()
}
Advantages of Handling Insecure Connections
User Awareness: Educates users about the risks of insecure connections.
Enhanced Security: Provides an additional layer of security by preventing accidental use of insecure networks.
Improved User Experience: Ensures users are informed and can make informed decisions about their security.
Wrapping Up
Securing network communications in your app is not just a best practice; it's a necessity. By using HTTPS and SSL/TLS, implementing certificate pinning, leveraging Network Security Configuration, and handling insecure connections, you can significantly enhance your app's security. 🛡️
Remember, security is an ongoing process. Regularly review and update your security practices to stay ahead of potential threats. Happy coding, and stay secure! ✌️
Subscribe to my newsletter
Read articles from Pratik Mhatre directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
