How to Create a Blog Using Angular: Step-by-Step Guide

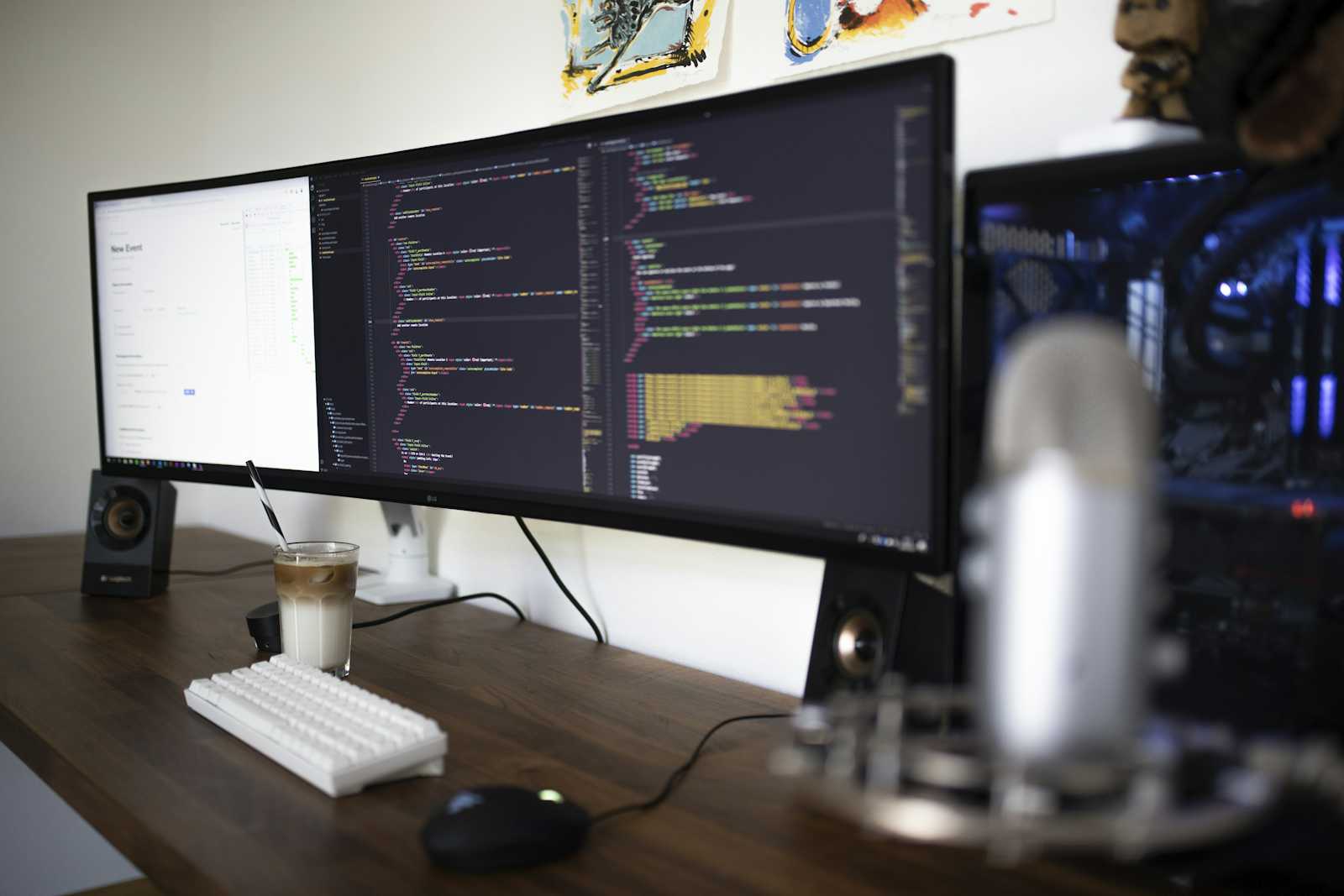
As a Software Engineer and Founder/CEO of a company, I've had the opportunity to work with various front-end frameworks, and I must say that Angular is one of my favourites. In this article, I'll guide you through the process of creating a blog using Angular, and explain why I prefer it over other frameworks.
Why Angular?
So, why did I choose Angular for this project? Well, there are several reasons. Firstly, Angular is a mature and widely used framework, which means there's a large community of developers who contribute to it and provide support. This is especially important when you're working on a complex project and need help troubleshooting issues.
Another reason I chose Angular is its robustness and scalability. Angular is designed to handle complex, enterprise-level applications, which makes it perfect for building a blog that can handle a large amount of traffic. Additionally, Angular's modular architecture makes it easy to break down the application into smaller, manageable components, which makes it easier to maintain and update.
Finally, I chose Angular because of its strong typing system and built-in support for dependency injection. These features make it easier to write clean, modular code that's easy to understand and maintain.
Setting Up Angular on a Local Machine
To get started with Angular, you'll need to set it up on your local machine. Here's a step-by-step guide on how to do it:
Windows
Install Node.js from the official website: https://nodejs.org/en/download/
Install Angular CLI using npm:
npm install -g @angular/cli
Create a new Angular project:
ng new my-blog
Install the necessary dependencies:
npm install
Start the development server:
ng serve
MacOS/Linux
Install Node.js using Homebrew:
brew install node
Install Angular CLI using npm:
npm install -g @angular/cli
Create a new Angular project:
ng new my-blog
Install the necessary dependencies:
npm install
Start the development server:
ng serve
IDEs
There are several IDEs that you can use to develop Angular applications. Here are some popular ones:
Visual Studio Code (VS Code)
IntelliJ IDEA
WebStorm
Sublime Text
Micro-Project: Building a Blog with Angular
As the Founder/CEO of a company, I want to create a blog that showcases our company's expertise in Angular development. Here's a micro-project that demonstrates how to build a blog with Angular:
Step 1: Create a Markdown File for the Post
First, let's create a markdown file in src/content/my-first-post.md
for our first post.
---
title: My First Post
slug: my-first-post
---
Hello World
Step 2: Define an Interface for the Data
Next, let's define an interface for the shape of the data returned from the front matter. For this example, the interface is placed in the src/app/models/post.ts
file.
export interface BlogPost {
title: string;
slug: string;
content: string;
}
Step 3: Create a Page Component for the Blog
Now, let's create a page component for the blog. This page serves as the parent layout for listing the blog posts and displaying an individual blog post. Create the page component file in src/app/pages/blog.page.ts
.
import { Component } from '@angular/core';
import { RouterOutlet } from '@angular/router';
@Component({
standalone: true,
imports: [RouterOutlet],
template: `
<h1>My Blog</h1>
<router-outlet />
`,
})
export default class BlogPage {}
Step 4: Create a Page Component for Displaying a Blog Post
Finally, let's create a page component for displaying a blog post. Define a page component in src/app/pages/blog/[slug].page.ts
.
import { MarkdownComponent, injectContent } from '@analogjs/content';
import { AsyncPipe, NgIf } from '@angular/common';
import { Component } from '@angular/core';
import { BlogPost } from 'rc/app/models/post';
@Component({
standalone: true,
imports: [MarkdownComponent, NgIf, AsyncPipe],
template: `
<div *ngIf="post$ | async as post">
<h2>{{ post.attributes.title }}</h2>
<analog-markdown [content]="post.content" />
</div>
`,
})
export default class BlogPostPage {
post$ = injectContent<BlogPost>();
}
Advanced Topics
Now that we've covered the basics of creating a blog with Angular, let's dive into some advanced topics:
Angular Universal: Angular Universal is a technology that allows you to render your Angular application on the server. This can improve SEO and provide faster page loads.
Angular Service Worker: Angular Service Worker is a technology that allows you to cache your application's resources and provide offline support.
Angular Material: Angular Material is a library of UI components that can be used to build responsive and accessible applications.
ChatGPT Prompts for Bloggers
Now that we have created our blog, let's explore some ChatGPT prompts for bloggers. These prompts can help you come up with ideas for your blog posts, write your scripts for YouTube videos, and even generate social media content.
ChatGPT Prompts to Come Up With Ideas
1. Prompt: I run a blog aimed at [AUDIENCE] about [TOPIC]. Come up with 10 ideas that I could write about on my blog. For each idea, suggest 3 different titles.
2. Prompt: I want to start a blog. I’m interested in [TOPIC]. Give me 5 topic areas and 10 specific blog post ideas for each.
ChatGPT Prompts for Social Media Content
22. Prompt: Give me [X] tweets to promote my blog post [TITLE]. Each tweet should offer a valuable tip or idea as well as linking to the post.
23. Prompt: Give me [X] LinkedIn posts to share insights from my latest [PRODUCT TYPE], titled [TITLE]. The [PRODUCT TYPE] covers [BRIEF DESCRIPTION].
24. Prompt: I run a blog about [TOPIC]. Come up with [X] ideas for photos I could post on Instagram, with [X] choices of captions for each, to promote my blog. I want to attract an audience of [AUDIENCE].
ChatGPT Prompts for YouTube Videos
25. Prompt: Come up with [X] ideas for short YouTube videos that fit with my topic of [TOPIC]. All the ideas should be suitable for an audience of [AUDIENCE].
26. Prompt: Write a script for a short YouTube video on [TITLE/TOPIC]. Include a call to action at the end to [DO SOMETHING].
27. Prompt: Outline a [X] minute YouTube video on [TITLE], split into [NUMBER] sections.
Additional ChatGPT Prompts
28. Prompt: I want to create a lead magnet to attract subscribers to my blog. Give me [X] ideas for lead magnets that would appeal to an audience of [AUDIENCE].
29. Prompt: I want to create a course to teach [TOPIC]. Give me [X] ideas for course modules, with [X] lessons in each module.
30. Prompt: I want to create a podcast about [TOPIC]. Give me [X] ideas for podcast episodes, with [X] guests for each episode.
Conclusion
In this article, we've created a blog using Angular and explored some ChatGPT prompts for bloggers. We've covered the basics of creating a markdown file, defining an interface for the data, creating a page component for the blog, and creating a page component for displaying a blog post. We've also explored some advanced topics, such as Angular Universal, Angular Service Worker, and Angular Material.
I hope this article has helped create a blog with Angular and use ChatGPT prompts for bloggers. Happy blogging!
"Success is not final, failure is not fatal: It is the courage to continue that count." - Winston Churchill
Subscribe to my newsletter
Read articles from Niladri Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
