Dissecting JavaScript II
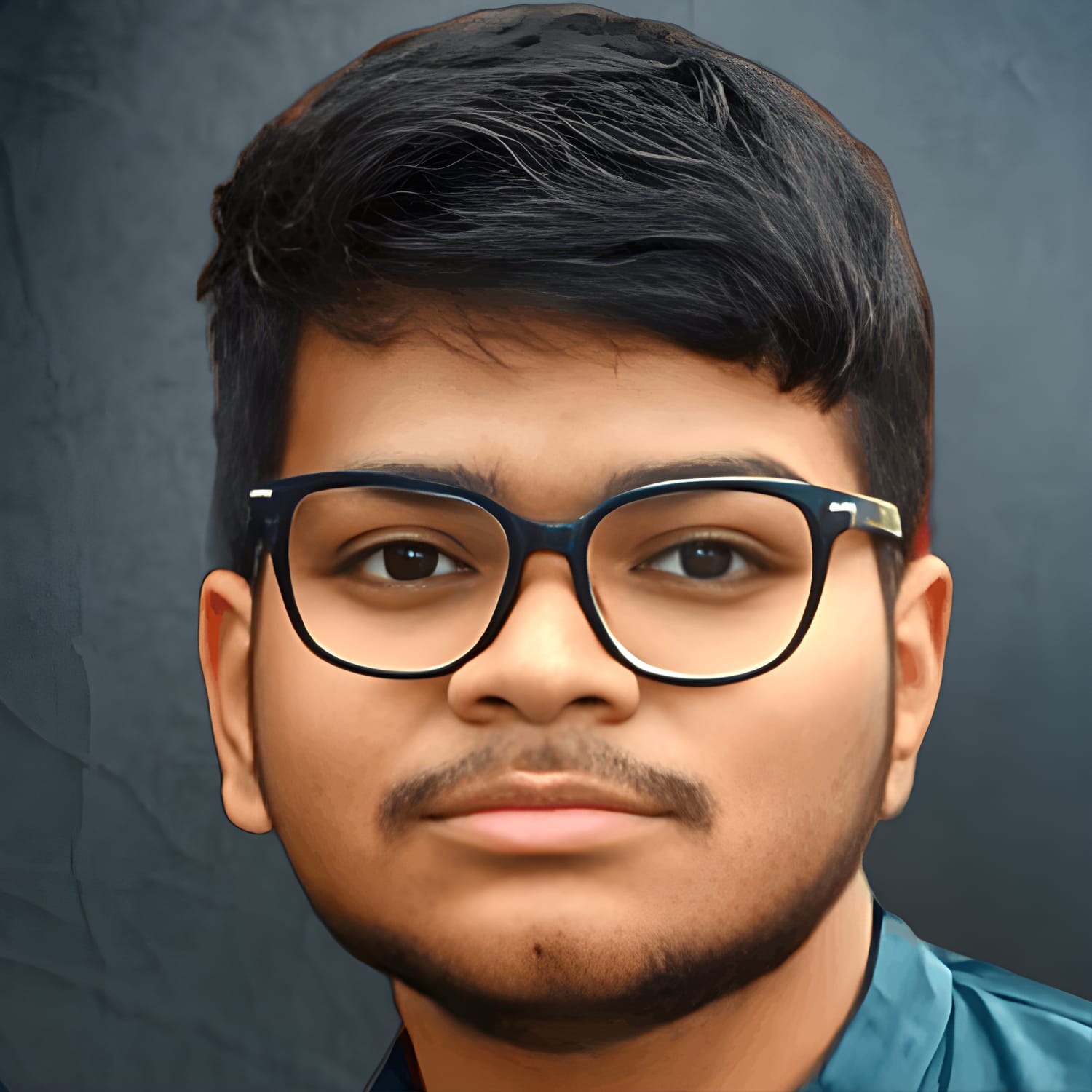
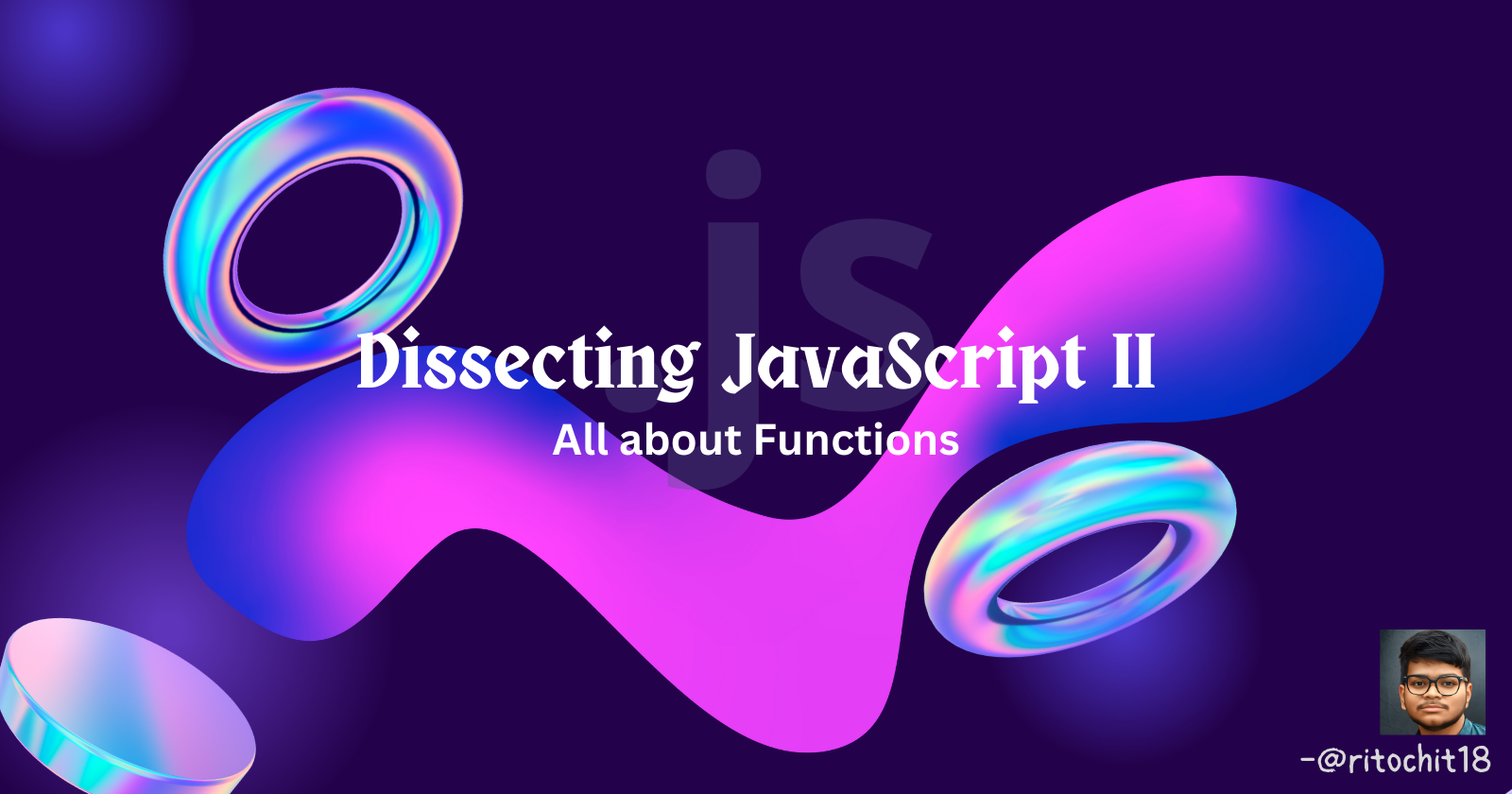
Introduction
In the last part, we have discussed about the internal workings of JavaScript & some deeper concepts of JavaScript in a much simpler way, in case you haven't checked it yet. Here is the link to that, if you are a beginner trying to learn from this blog, I would rather suggest you to go through that article once before starting it. This blog contains all about functions in JavaScript, different array methods, and some related topics along with terminologies.
ctrl + Shift + I
(For Windows) Cmd + Option + I
(For Mac) to open the console tab.Functions
Functions are reusable blocks of code, designed to perform a specific task. They allow us programmers to follow the DRY(don't Repeat Yourself!) principle of coding. Functions make our code modulated, organized & maintainable. Typically, a function takes input(Parameters), run some predefined logic on them, and returns an output.
Take a look at a basic greeting function in JavaScript:-
function Greet(user){
console.log(`Hello, ${user}`);
}
Greet("Ritochit");
// Output: Hello, Ritochit
Function Statement
A function statement in JavaScript is a named function with specified parameters and a block of code to execute whenever the function is called. The above greeting function is an example of a simple function Statement.
It is also referred to as Function Declaration.
Function Expression
A function, which is assigned to a variable is a Function Expression. In function expression, functions can be used as values. For example,
let func = function(){
console.log("func function is called");
}
func();
// Output: func function is called
If a function expression is hoisted it will give a Reference error i.e. in the memory creation phase the function expression will be treated as a variable.
Arrow Function
The arrow function is another way of writing concise function expressions in JavaScript. It was not a part of JavaScript initially but was introduced later in ES6. The main feature is implicit returns for single expressions. Arrow functions do not have their own this
context but they can inherit it lexically from their parents. Thus, though arrow functions are ideal for short functions and callbacks, but they cannot be used as constructors.
Here is an example of an arrow function:
const greet = (user) => {
console.log(`Hello, ${user}`);
}
greet("Ritochit");
// Output: Hello, Ritochit
IIFE (Immediately Invoked Function Expression)
An Immediately Invoked Function Expression (IIFE) is a JavaScript function that runs as soon as it is defined. It is generally used to create a block scope, avoiding variable collisions in the global namespace. The syntax is a normal function statement that is followed by ()
which executes the function immediately while running the code.
(function() { /* code */ })();
(function() {
console.log("Hello Reader, this is IIFE");
})();
// output: Hello Reader, this is IIFE
Higher-Order Functions
A higher-order function is just another JS function but the key is it either accepts another function as an argument or returns another function as an output or maybe both simultaneously. This concept is crucial for functional programming as it enables operations like mapping, filtering, & reduction. These functions help in code reusability and modularity.
function operation(num1, num2, logic) {
let first = logic(num1);
let second = logic(num2);
return first + second;
}
const cube = (n) => {
return n*n*n;
}
const square = (n) => {
return n*n;
}
const ans = operation(2, 3, square);
console.log(`Answer: ${ans}`);
// Output: Answer: 13
String Interpolation
Some of you who are learning JavaScript might be wondering about the console.log(`Answer: ${ans}`);
in the last line of the previous example. It is called string interpolation. It allows us to embed expressions within a string. In this method, literals are enclosed by backticks (`)
and preceded by a dollar sign($)
. This type of literals is called Template literals it's also another feature included in ES6, which is a way to create strings in JavaScript that allows for easier inclusion of variables and expressions within the string. Template literals provide several benefits, such as multi-line strings and string interpolation.
const person = "Ritochit Ghosh";
let age = "19";
console.log(`${person} is ${age} years old`);
// Output: Ritochit Ghosh is 19 years old
Array Methods: Map, Filter, and Reduce
Now, let's discuss the three most used array methods in JavaScript: map
, filter
, and reduce
. These methods are essential for processing and transforming arrays efficiently.
Map
The map function is generally used to transform elements of an array following certain logic. The map
method creates a new array by applying a function to each element of the original array.
const numbers = [1, 2, 3, 4, 5];
const squareOfNumbers = numbers.map(function(num) {
return num * num;
});
console.log(squareOfNumbers);
// Output: [1, 4, 9, 16, 25]
Filter
The filter function is preferably used to filter out some of the elements of an existing array based on a given condition. The filter
method creates a new array with all elements that fulfill a test implemented by the provided function. It is useful when you want to select a subset of elements from an array based on some condition.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(function(num) {
return num % 2 === 0;
});
console.log(evenNumbers);
// Output: [2, 4]
Reduce
The reduce function is used when you need to calculate a single value from an array of values. The reduce
method applies a function against an accumulator and each element in the array to reduce it to a single value. Optionally, you can provide an initial value for the accumulator
. If not provided, the first element of the array is used as the initial accumulator value and iteration starts from the second element.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce(function(accumulator, currentValue) {
return accumulator + currentValue;
}, 0);
console.log(sum);
// Output: 15
These methods promote functional programming practices and help in writing clean, readable, and maintainable code.
Spread Operator
The spread operator (...
) in JavaScript is one of the versatile syntaxes provided by JS which simplifies array manipulation & parameter handling. It is used to expand elements of an array (or iterable) into places where multiple elements are expected. For example, [...array]
spreads out the elements of the array
, making them individual elements of a new array.
const batters = ["Rohit", "Gill", "Virat", "Shreyas", "Pant"];
const allRounders = ["Hardik", "Jadeja"];
const bowlers = ["Bumrah", "Shami", "Kuldeep", "Chahal"];
const team = [...batters, ...allRounders, ...bowlers];
console.log(team);
// Output: [ "Rohit", "Gill", "Virat", "Shreyas", "Pant",
// "Hardik", "Jadeja", "Bumrah", "Shami", "Kuldeep", "Chahal"]
This marks an end to this blog the second of the series Dissecting JavaScript. Here we have learned about functions, different jargon related to them the differences between each of them, String Interpolation, Spread operator, & Higher order functions - map, filter & reduce along with proper examples of each of them.
Conclusion
In the next part will discuss the remaining topics i.e. callback functions, Callback hell, the need for Promises, async - await syntax, & lastly Browser features and JS engine in detail.
Hope you enjoyed this blog and liked it. Do share your valuable feedback if possible, this will help me to improve myself.
Stay tuned for the last part of the Dissecting JavaScript Series, where we'll conclude our journey of JavaScript programming to mark the start of another journey.
Happy Coding & Stay Safe!
Subscribe to my newsletter
Read articles from Ritochit Ghosh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
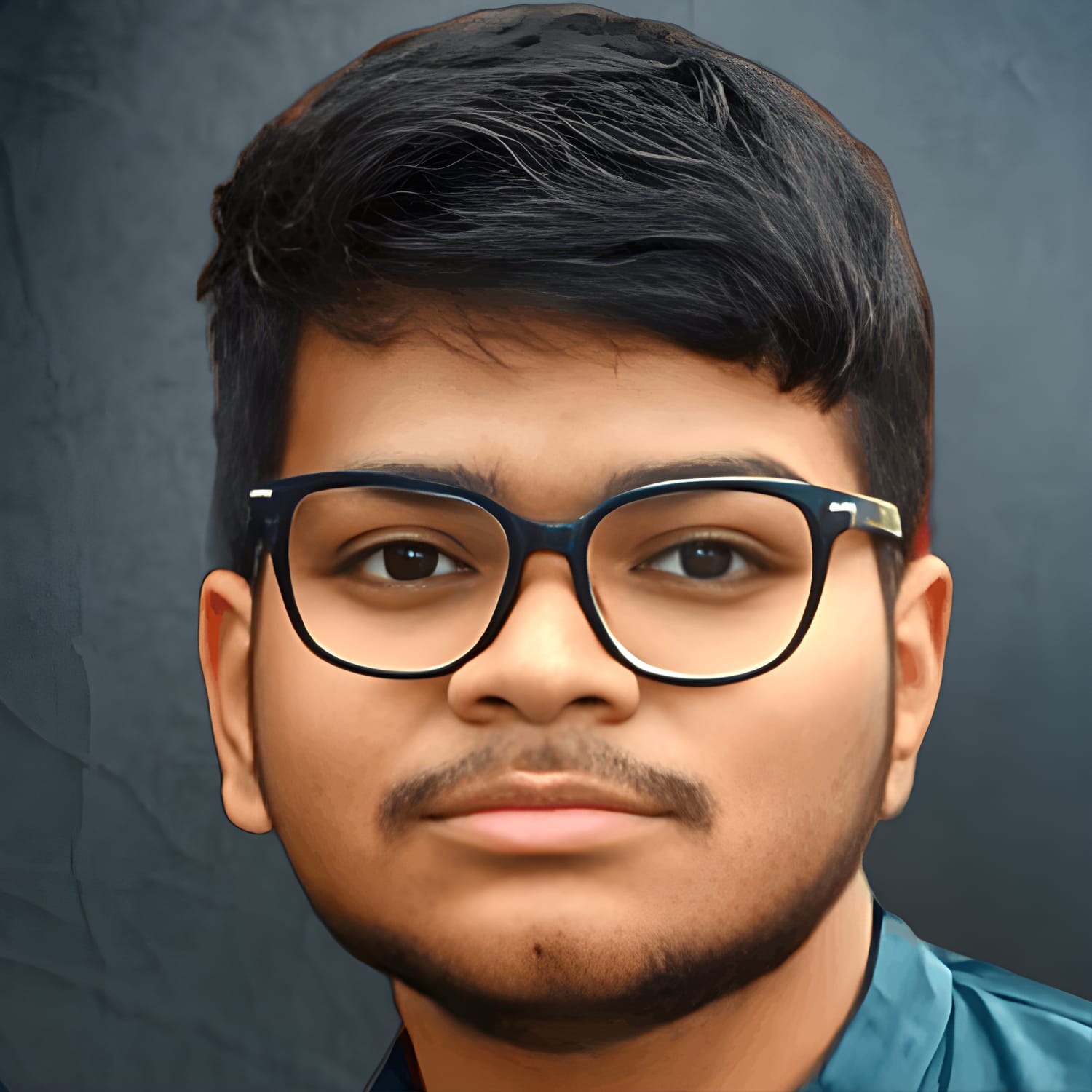
Ritochit Ghosh
Ritochit Ghosh
I am a second-year student pursuing a BTech in CSE here at Maulana Abul Kalam University of Technology. I am a tech enthusiast and an Open-Source advocate, having an interest in web Development and AI/ML. Here I will share my tech journey and talk about different related topics.