Getting Started with Actix for Rust Developers - A Crow's Perspective (Part 1)
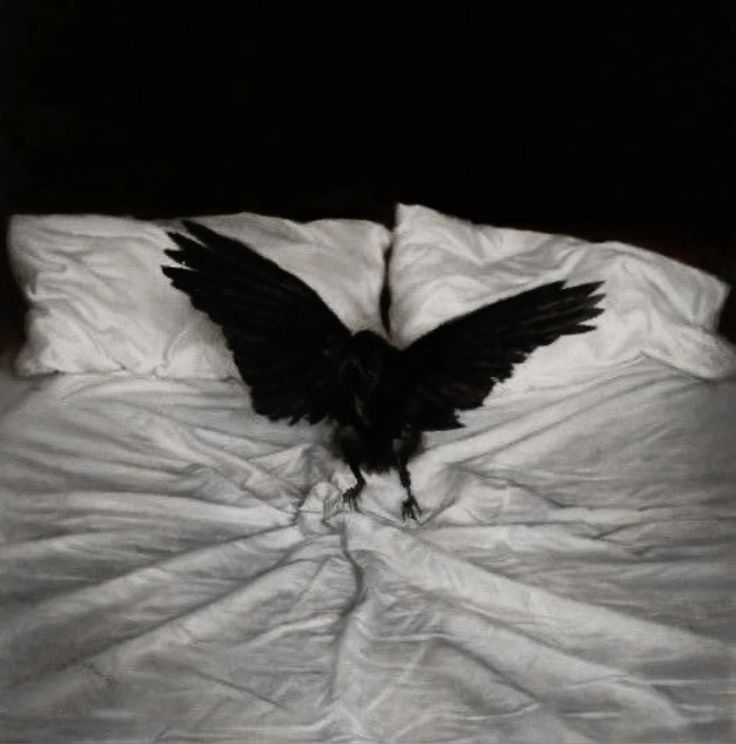
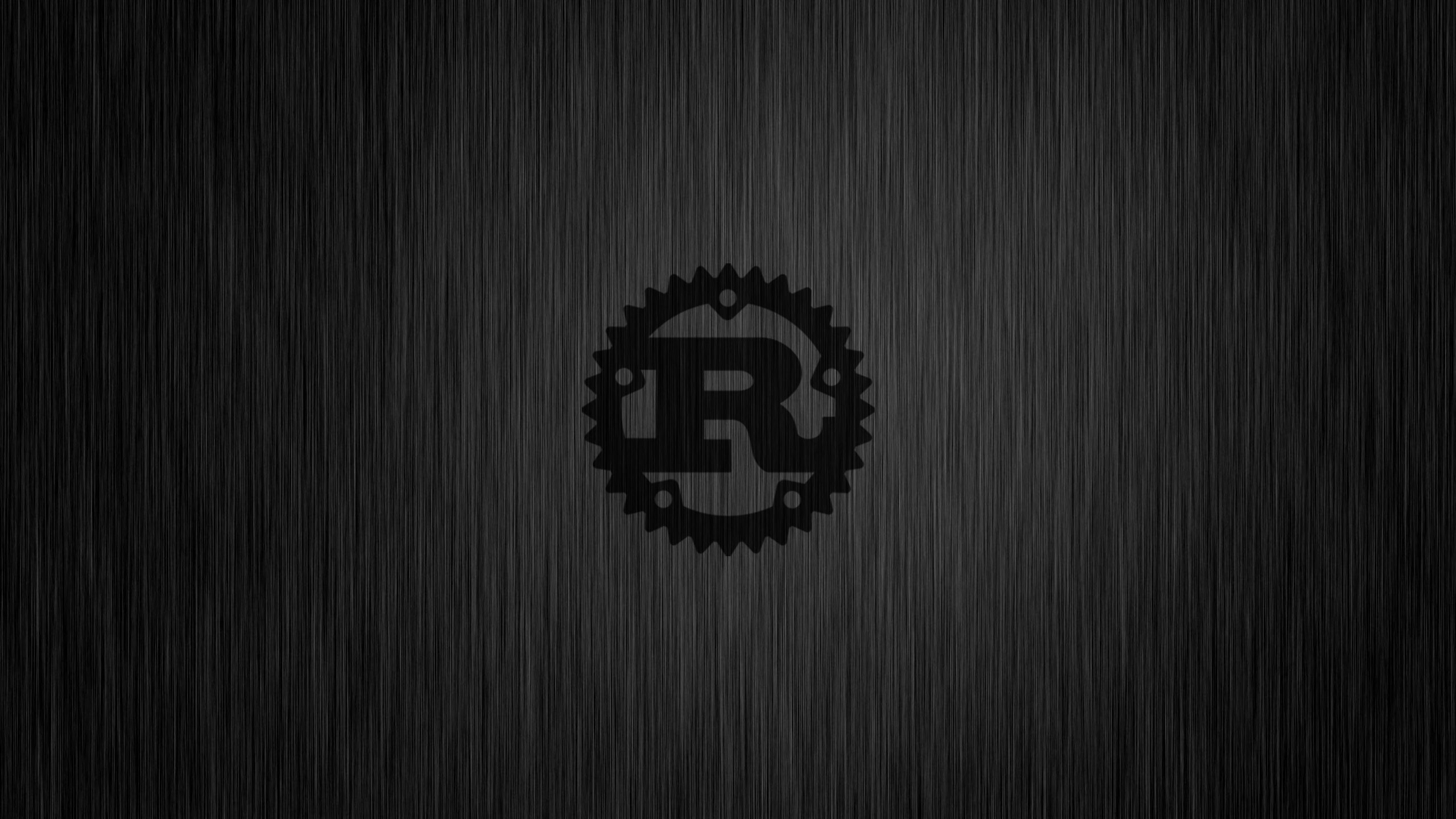
Greetings, fellow Rustaceans! It's your favorite feathered friend here, CrowWrites, swooping down to deliver some juicy tidbits on Actix. If you're looking to spread your wings and soar into the skies of asynchronous web development with Rust, you've landed at the right perch. Let’s dive into the worm-filled world of Actix, and I'll show you how to caw-reate a simple web server. Ready? Let's get flocking!
Table of Contents
Introduction to Actix
Setting Up Your Nest (Project)
Building Your First Nest (Web Server)
Pecking at Requests and Responses
Feathering Your Nest with Middleware
Handling Errors like a Wise Old Crow
Flying High with Advanced Features
Conclusion
1. Introduction to Actix
Actix is like the shiny object in the web framework nest—it's powerful, high-performing, and perfect for asynchronous applications. Built on Rust's robust type system and async capabilities, Actix Web allows us to build scalable web servers that can handle a murder of requests (that's a group of crows, by the way).
Why Choose Actix?
Performance: Actix is known for its blazing speed, often outperforming other web frameworks in benchmarks.
Scalability: Actix can handle a high number of concurrent connections, making it ideal for modern web applications.
Flexibility: With a rich set of features, Actix can be used for anything from simple APIs to complex, real-time applications.
Type Safety: Leveraging Rust's type system, Actix ensures that many errors are caught at compile time, leading to more robust applications.
2. Setting Up Your Nest (Project)
Before we start caw-ing our way through code, ensure you have Rust and Cargo installed. If not, fly over to rustup.rs and grab them.
Create a new Cargo project with a quick flap of your wings:
shCopy codecargo new actix-web-demo
cd actix-web-demo
Now, add Actix Web to your Cargo.toml
to get those feathers in place:
tomlCopy code[dependencies]
actix-web = "4"
Run cargo build
to download and compile the dependencies. You're now ready to start building!
3. Building Your First Nest (Web Server)
In your src/main.rs
, set up a basic Actix Web server. This will be your new nest:
rustCopy codeuse actix_web::{web, App, HttpServer, Responder};
async fn greet() -> impl Responder {
"Caw! Welcome to my web server!"
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.route("/", web::get().to(greet))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
Explanation:
Imports: The
use
statements bring necessary Actix Web components into scope.Async Function: The
greet
function is defined as asynchronous and returns a responder with a simple text message.Main Function: The main function sets up and runs the HTTP server:
HttpServer::new(|| { ... })
: Creates a new server instance.App::new()
: Creates a new Actix Web application..route("/", web::get().to(greet))
: Defines a route that maps the root URL to thegreet
function..bind("127.0.0.1:8080")?
: Binds the server to the specified address and port..run().await
: Runs the server asynchronously.
To run the server, use:
shCopy codecargo run
Hop over to http://127.0.0.1:8080
in your browser to see the greeting.
4. Pecking at Requests and Responses
Actix makes it easy to handle different types of requests and return various types of responses. Here's how you can handle JSON requests and responses:
rustCopy codeuse actix_web::{web, App, HttpResponse, HttpServer, Responder};
use serde::{Deserialize, Serialize};
#[derive(Deserialize)]
struct Info {
name: String,
}
#[derive(Serialize)]
struct Greet {
message: String,
}
async fn greet(info: web::Json<Info>) -> impl Responder {
HttpResponse::Ok().json(Greet {
message: format!("Caw! Hello, {}!", info.name),
})
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.route("/greet", web::post().to(greet))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
Explanation:
Imports: The necessary Actix Web and Serde components are imported.
Data Structures:
Info
: A struct for deserializing incoming JSON payloads.Greet
: A struct for serializing outgoing JSON responses.
Greet Function: The
greet
function accepts a JSON payload, constructs a greeting message, and responds with a JSON object.Route Setup: The
/greet
route is defined to accept POST requests, mapped to thegreet
function.
This approach leverages Rust's powerful type system and Serde's serialization/deserialization capabilities to handle JSON data effortlessly.
5. Feathering Your Nest with Middleware
Middleware in Actix allows you to modify requests and responses. Let's add some logging, like a crow keeping track of shiny objects:
rustCopy codeuse actix_web::{web, App, HttpServer, middleware::Logger, Responder};
async fn greet() -> impl Responder {
"Caw! Welcome to my web server!"
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
env_logger::init();
HttpServer::new(|| {
App::new()
.wrap(Logger::default())
.route("/", web::get().to(greet))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
Explanation:
- Logger Middleware: The
Logger
middleware is added to the application using.wrap(Logger::default())
. This middleware logs each incoming request, providing valuable insights for debugging and monitoring.
6. Handling Errors like a Wise Old Crow
Actix provides a flexible way to handle errors. Here's how to return custom error responses:
rustCopy codeuse actix_web::{web, App, HttpResponse, HttpServer, Result};
async fn index() -> Result<&'static str> {
Ok("Caw! Hello, World!")
}
async fn error() -> Result<&'static str> {
Err(actix_web::error::ErrorBadRequest("Caw! Bad Request"))
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.route("/", web::get().to(index))
.route("/error", web::get().to(error))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
Explanation:
Result Type: The handler functions return
Result
, allowing them to return either a successful response or an error.Error Handling: The
error
function demonstrates how to return a custom error response usingactix_web::error::ErrorBadRequest
.
7. Flying High with Advanced Features
Actix is packed with advanced features that enable you to build complex applications. Here are a few to get you started:
7.1. State Management
You can manage application state using Actix's built-in support:
rustCopy codeuse actix_web::{web, App, HttpServer, Responder};
use std::sync::Mutex;
struct AppState {
counter: Mutex<i32>,
}
async fn increment(data: web::Data<AppState>) -> impl Responder {
let mut counter = data.counter.lock().unwrap();
*counter += 1;
format!("Counter: {}", counter)
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
let data = web::Data::new(AppState {
counter: Mutex::new(0),
});
HttpServer::new(move || {
App::new()
.app_data(data.clone())
.route("/increment", web::get().to(increment))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
Explanation:
AppState: A struct to hold shared state, wrapped in a
Mutex
for thread safety.Data Injection: The state is injected into the application using
web::Data
and accessed in the handler usingweb::Data<AppState>
.
7.2. WebSockets
Actix supports WebSockets for real-time communication:
rustCopy codeuse actix::{Actor, StreamHandler};
use actix_web::{web, App, Error, HttpRequest, HttpServer, Responder};
use actix_web_actors::ws;
struct MyWebSocket;
impl Actor for MyWebSocket {
type Context = ws::WebsocketContext<Self>;
}
impl StreamHandler<Result<ws::Message, ws::ProtocolError>> for MyWebSocket {
fn handle(&mut self, msg: Result<ws::Message, ws::ProtocolError>, ctx: &mut Self::Context) {
if let Ok(ws::Message::Text(text)) = msg {
ctx.text(text);
}
}
}
async fn websocket(req: HttpRequest, stream: web::Payload) -> Result<impl Responder, Error> {
ws::start(MyWebSocket {}, &req, stream)
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.route("/ws/", web::get().to(websocket))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
Explanation:
Actor:
MyWebSocket
struct implementsActor
andStreamHandler
traits from Actix.WebSocket Handler: The
websocket
function starts a WebSocket session usingws::start
.
8. Conclusion
Actix is a shiny framework that leverages Rust's strengths to build high-performance web applications. With this introduction, you're ready to set up a basic Actix project, handle requests and responses, use middleware, and manage errors. As you become more familiar with Actix, you'll find even more features to build complex and scalable applications.
So, spread your wings and start caw-ing with Actix!
Subscribe to my newsletter
Read articles from Kawshall directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
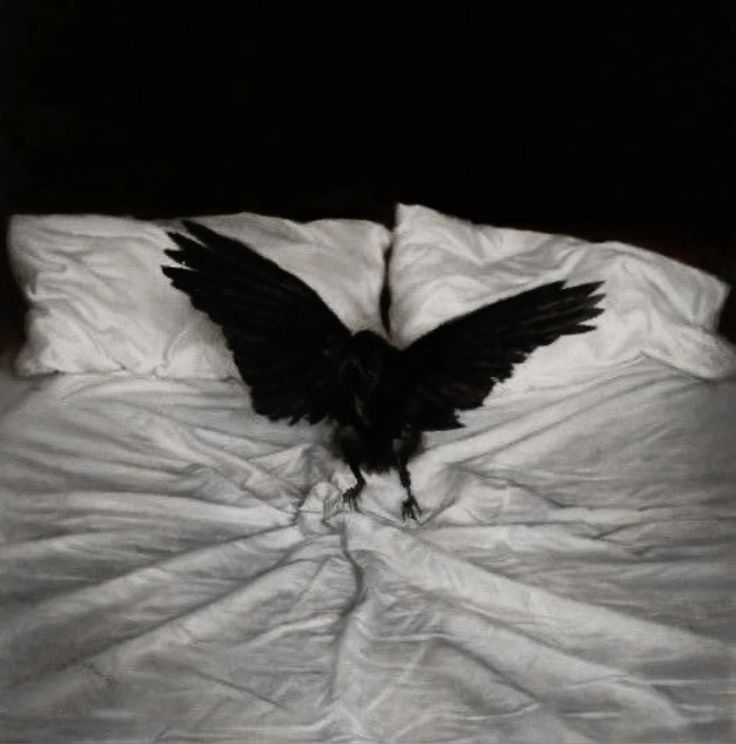
Kawshall
Kawshall
i sleep and write, with the obvious {kaw kaw}'s