Getting Started with Actix for Rust Developers - A Crow's Perspective (Part 2)
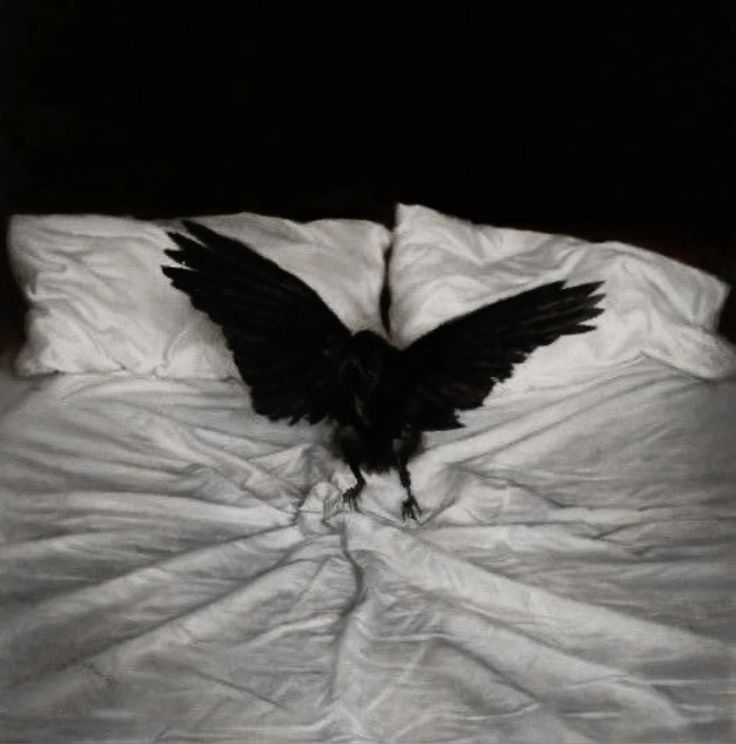
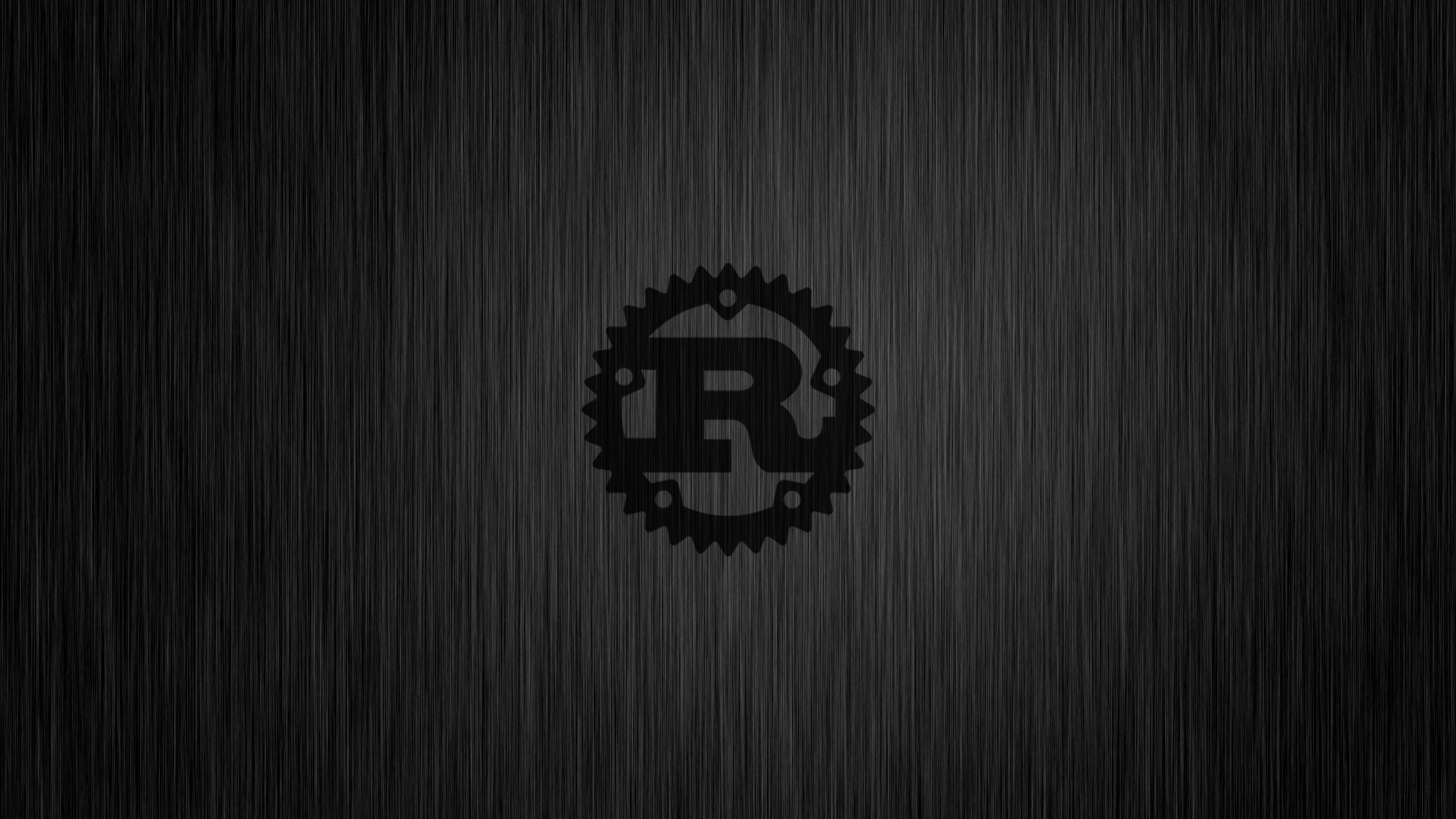
Welcome back, feathered friends! In Part 1, we covered the basics of setting up an Actix web server, handling requests and responses, using middleware, and managing errors. In this part, we'll dive deeper into more advanced features and best practices to help you build more robust and scalable applications.
Table of Contents
Advanced State Management
Working with Databases
WebSockets in Depth
Testing Your Actix Applications
Deployment Best Practices
Conclusion
1. Advanced State Management
In Part 1, we touched on state management using Mutex
. However, there are more advanced techniques for handling state, especially in a multi-threaded environment.
Using Arc
and RwLock
For read-heavy workloads, consider using Arc
and RwLock
:
rustCopy codeuse actix_web::{web, App, HttpServer, Responder};
use std::sync::{Arc, RwLock};
struct AppState {
counter: RwLock<i32>,
}
async fn increment(data: web::Data<Arc<AppState>>) -> impl Responder {
let mut counter = data.counter.write().unwrap();
*counter += 1;
format!("Counter: {}", counter)
}
async fn get_counter(data: web::Data<Arc<AppState>>) -> impl Responder {
let counter = data.counter.read().unwrap();
format!("Counter: {}", counter)
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
let data = web::Data::new(Arc::new(AppState {
counter: RwLock::new(0),
}));
HttpServer::new(move || {
App::new()
.app_data(data.clone())
.route("/increment", web::get().to(increment))
.route("/counter", web::get().to(get_counter))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
Explanation:
Arc:
Arc
(Atomic Reference Counted) ensures that the state can be shared across multiple threads.RwLock:
RwLock
allows multiple readers or one writer at a time, improving concurrency for read-heavy workloads.
2. Working with Databases
Actix integrates seamlessly with various databases. Here, we’ll use Diesel, a powerful ORM for Rust.
Setting Up Diesel
Add Diesel to your Cargo.toml
:
tomlCopy code[dependencies]
diesel = { version = "1.4.8", features = ["postgres"] }
dotenv = "0.15"
actix-web = "4"
actix-rt = "2.4"
Run the following commands to set up Diesel:
shCopy codecargo install diesel_cli --no-default-features --features postgres
diesel setup
Creating a Model
Define your database schema in src/
schema.rs
:
rustCopy codetable! {
users (id) {
id -> Int4,
name -> Varchar,
email -> Varchar,
}
}
Create a model in src/
models.rs
:
rustCopy codeuse super::schema::users;
use diesel::prelude::*;
#[derive(Queryable, Insertable)]
#[table_name = "users"]
struct User {
id: i32,
name: String,
email: String,
}
Using Diesel in Actix
Set up a simple Actix Web server to interact with the database:
rustCopy codeuse actix_web::{web, App, HttpServer, HttpResponse, Responder};
use diesel::prelude::*;
use diesel::pg::PgConnection;
use dotenv::dotenv;
use std::env;
mod models;
mod schema;
use models::User;
pub fn establish_connection() -> PgConnection {
dotenv().ok();
let database_url = env::var("DATABASE_URL").expect("DATABASE_URL must be set");
PgConnection::establish(&database_url).expect(&format!("Error connecting to {}", database_url))
}
async fn get_users() -> impl Responder {
use schema::users::dsl::*;
let connection = establish_connection();
let results = users.load::<User>(&connection).expect("Error loading users");
HttpResponse::Ok().json(results)
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.route("/users", web::get().to(get_users))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
Explanation:
Database Connection: The
establish_connection
function sets up a connection to the PostgreSQL database using environment variables.Route Setup: The
/users
route fetches and returns all users from the database in JSON format.
3. WebSockets in Depth
WebSockets enable real-time communication in web applications. Actix provides robust support for WebSockets, allowing you to build interactive applications easily.
Advanced WebSocket Example
rustCopy codeuse actix::{Actor, StreamHandler, Handler, Message};
use actix_web::{web, App, HttpRequest, HttpServer, Responder, Error};
use actix_web_actors::ws;
struct MyWebSocket {
count: usize,
}
impl Actor for MyWebSocket {
type Context = ws::WebsocketContext<Self>;
}
impl StreamHandler<Result<ws::Message, ws::ProtocolError>> for MyWebSocket {
fn handle(&mut self, msg: Result<ws::Message, ws::ProtocolError>, ctx: &mut Self::Context) {
match msg {
Ok(ws::Message::Text(text)) => {
self.count += 1;
ctx.text(format!("Message {}: {}", self.count, text))
}
Ok(ws::Message::Ping(msg)) => ctx.pong(&msg),
_ => (),
}
}
}
async fn websocket(req: HttpRequest, stream: web::Payload) -> Result<impl Responder, Error> {
ws::start(MyWebSocket { count: 0 }, &req, stream)
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.route("/ws/", web::get().to(websocket))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
Explanation:
Stateful Actor: The
MyWebSocket
struct now includes acount
field to track the number of messages.StreamHandler Implementation: The
handle
function processes different types of WebSocket messages, incrementing the count and responding with the count and message text.
4. Testing Your Actix Applications
Testing is crucial for building reliable applications. Actix supports testing through integration tests and unit tests.
Integration Tests
Create a test file tests/integration_
test.rs
:
rustCopy codeuse actix_web::{test, App, web, HttpResponse};
async fn greet() -> HttpResponse {
HttpResponse::Ok().body("Caw! Welcome to my web server!")
}
#[actix_web::test]
async fn test_greet() {
let app = test::init_service(App::new().route("/", web::get().to(greet))).await;
let req = test::TestRequest::with_uri("/").to_request();
let resp = test::call_service(&app, req).await;
assert!(resp.status().is_success());
}
Explanation:
Test Service:
test::init_service
initializes the Actix application for testing.Test Request:
test::TestRequest
creates a test request.Call Service:
test::call_service
sends the test request to the application.Assertions:
assert!
verifies the response status.
5. Deployment Best Practices
Deploying Actix applications requires some best practices to ensure performance and reliability.
Building for Release
Always build your application in release mode for optimized performance:
shCopy codecargo build --release
Using a Reverse Proxy
Deploy your Actix application behind a reverse proxy like Nginx or Apache to handle SSL termination, load balancing, and more.
6. Conclusion
By now, you should have a solid understanding of Actix's capabilities and how to use it to build scalable, high-performance web applications in Rust. From setting up your project and handling requests to managing state, working with databases, implementing WebSockets, testing, and deploying, Actix offers a comprehensive toolkit for web development.
Keep experimenting, and don't be afraid to explore new features and patterns. The Rust ecosystem is constantly evolving, and there’s always something new to learn.
Happy coding, and may your code be as robust as a crow's nest in a storm!
That's it for now, fellow Rustaceans. Keep those beaks sharp, and may your code always be cleaner than a freshly preened feather. Caw-caw for now!
Subscribe to my newsletter
Read articles from Kawshall directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
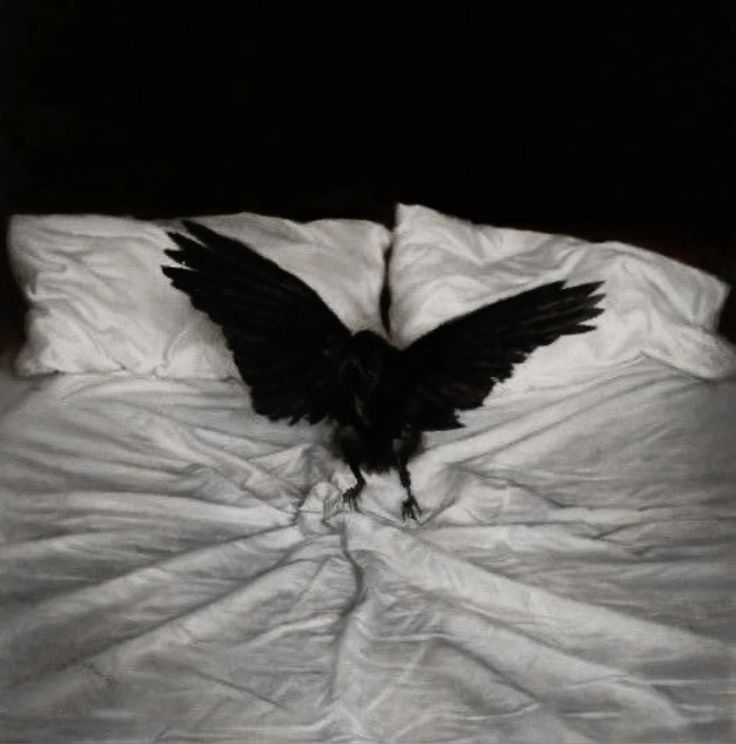
Kawshall
Kawshall
i sleep and write, with the obvious {kaw kaw}'s