The Toast Whisperer: Mastering Notifications with React Toastify
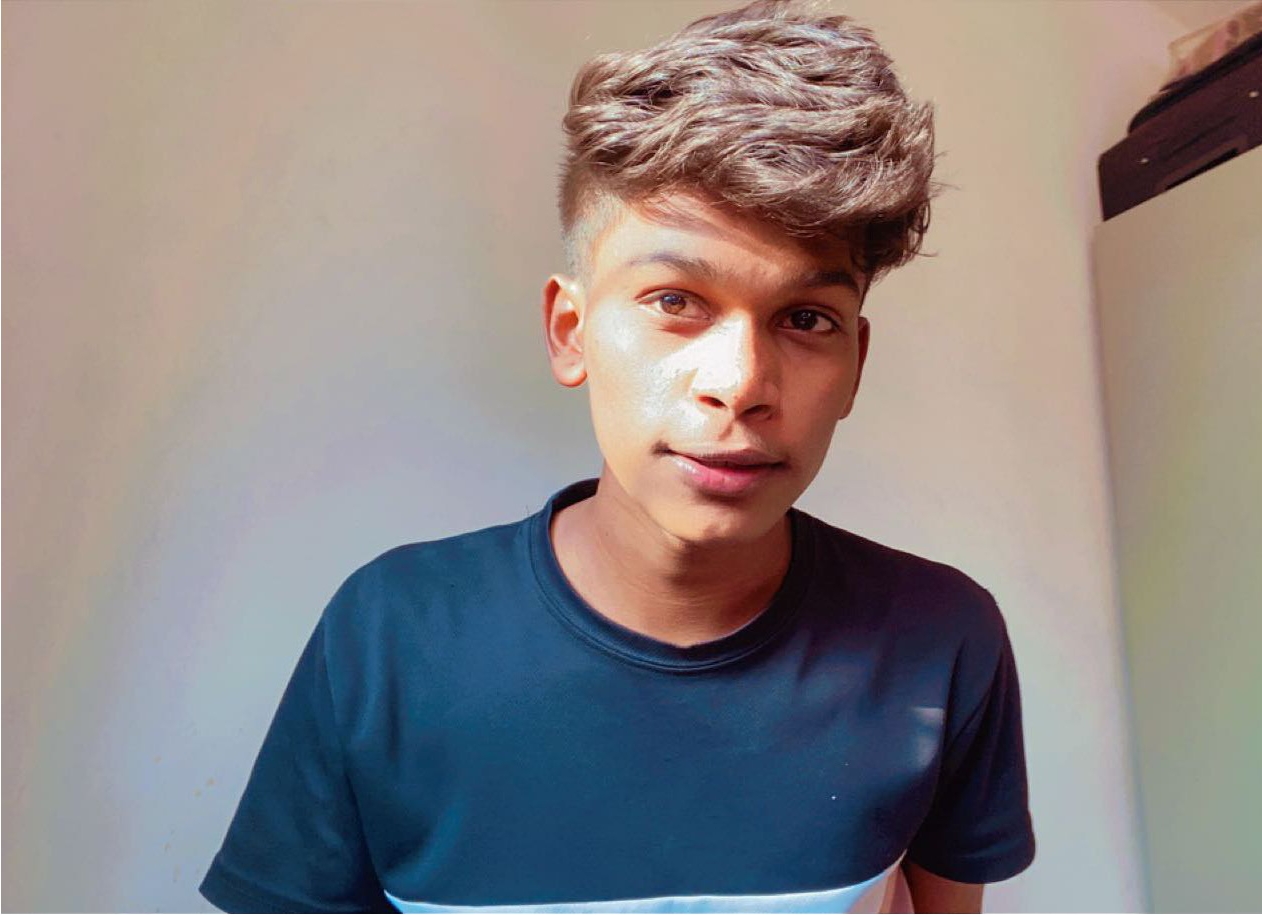
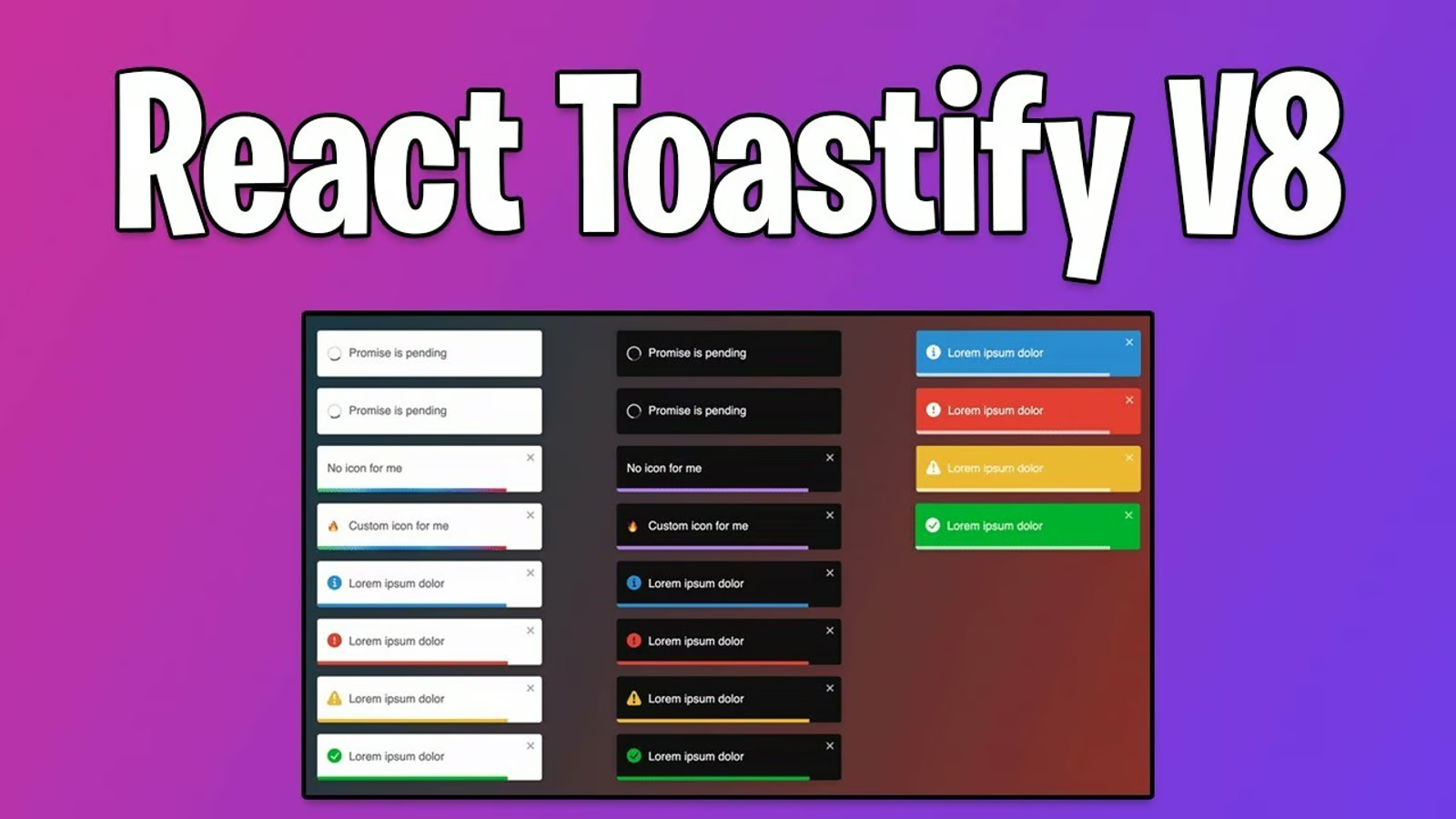
Introduction
Once upon a time, in the bustling land of Web Development, there was a developer named Alex. Alex was on a quest to find the perfect way to notify users of important events and updates on their web application. Traditional methods were cumbersome, unappealing, and often neglected by users. Then, Alex discovered the magical library known as React Toastify. Join Alex on a journey to master the art of user notifications with this powerful tool. 🍞✨
The Problem with Traditional Notifications
In the dark ages of web development, notifications were often intrusive, breaking the flow of user interaction. Alert boxes would pop up, demanding attention, while users would scramble to dismiss them just to get back to their tasks. These notifications were like unwanted guests at a party—annoying and disruptive. 🚨
Alex knew there had to be a better way, a method that would inform users without interrupting their experience. The search for a more elegant solution began.
Discovering React Toastify: A Game Changer
One day, while exploring the enchanted realms of NPM, Alex stumbled upon React Toastify. It was a revelation—a lightweight, customizable library designed specifically for creating beautiful, non-intrusive notifications. It promised to make notifications a delightful experience for users, seamlessly blending into the application without causing disruption. 🌟
Why React Toastify?
Simplicity: Easy to set up and use, even for beginners.
Customization: Highly customizable to fit the look and feel of any application.
Responsiveness: Works flawlessly on both desktop and mobile devices.
Accessibility: Built with accessibility in mind, ensuring all users can benefit from notifications.
Alex was intrigued and decided to give React Toastify a try.
Setting Up Your First Toast
The first step in Alex’s journey was to set up a simple toast notification. Armed with React Toastify, Alex dove into the code.
Installation
To get started, Alex installed the library using NPM:
npm install react-toastify
Next, Alex imported the necessary components and styles in the main application file:
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
Creating a Toast
With the library set up, Alex created a basic toast notification:
const notify = () => toast("This is a simple toast notification!");
function App() {
return (
<div>
<button onClick={notify}>Show Toast</button>
<ToastContainer />
</div>
);
}
With a click of a button, a beautiful toast notification appeared on the screen, unobtrusively delivering its message. Alex was thrilled. 🎉
Customizing Your Toasts: A Personal Touch
Alex realized that while the default toast was nice, it could be even better with some personalization. React Toastify provided numerous options to customize the appearance and behavior of toasts.
Custom Styles
Alex added custom styles to make the toast fit the application’s theme:
const customToast = () => toast("Customized toast notification!", {
position: "top-center",
autoClose: 5000,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined,
style: {
background: 'linear-gradient(to right, #00b09b, #96c93d)',
color: '#fff'
}
});
Adding Icons
To make the notifications more engaging, Alex added icons:
import { toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import { FaCheckCircle } from 'react-icons/fa';
const successToast = () => toast.success(<div><FaCheckCircle /> Success!</div>, {
position: toast.POSITION.TOP_RIGHT,
autoClose: 3000,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined,
});
Advanced Features: Taking Toasts to the Next Level
As Alex delved deeper, the true power of React Toastify became apparent. The library offered advanced features that could turn any notification into a masterpiece.
Custom Components
Alex created custom toast components to deliver richer content:
const CustomToast = ({ closeToast }) => (
<div>
<h4>Custom Toast</h4>
<button onClick={closeToast}>Close</button>
</div>
);
const showCustomToast = () => toast(<CustomToast />, {
position: toast.POSITION.BOTTOM_LEFT
});
Themed Toasts
By using different themes, Alex made notifications blend seamlessly with different sections of the app:
toast.configure({
theme: "dark",
position: "bottom-right",
autoClose: 5000,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined,
});
Real-World Applications
Armed with the knowledge of React Toastify, Alex applied it to various real-world scenarios, enhancing the user experience across the application.
User Feedback
Providing immediate feedback on user actions, such as form submissions or data saves, made the app feel responsive and engaging.
const handleSubmit = () => {
// Save data
toast.success("Form submitted successfully!");
}
Error Notifications
Alerting users to errors without disrupting their workflow helped maintain a smooth experience.
const handleError = () => {
// Handle error
toast.error("An error occurred. Please try again.");
}
Informational Updates
Keeping users informed about ongoing processes or system statuses without breaking their focus became effortless.
const handleInfo = () => {
// Inform user
toast.info("Your data is being processed.");
}
Challenges and Considerations
While React Toastify brought numerous benefits, Alex encountered a few challenges:
Overuse
Too many notifications can overwhelm users. Alex learned to use toasts judiciously, ensuring they added value without becoming a nuisance.
Performance
For large-scale applications, performance optimization was key. Alex ensured that toasts were lightweight and did not impact the app’s responsiveness.
Accessibility
Ensuring all users, including those with disabilities, could benefit from notifications was paramount. React Toastify’s built-in accessibility features were a great help, but Alex also added custom ARIA attributes where needed.
Conclusion
With React Toastify, Alex transformed the way notifications were handled in their application. No longer were notifications a dreaded interruption; they became a seamless part of the user experience.
By mastering the art of toast notifications, Alex became the Toast Whisperer, guiding users through the enchanted land of Web Development with elegance and finesse. 🌟🍞
Embrace the power of React Toastify, and let your notifications elevate your application to new heights.
Subscribe to my newsletter
Read articles from Aditya Dhaygude directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
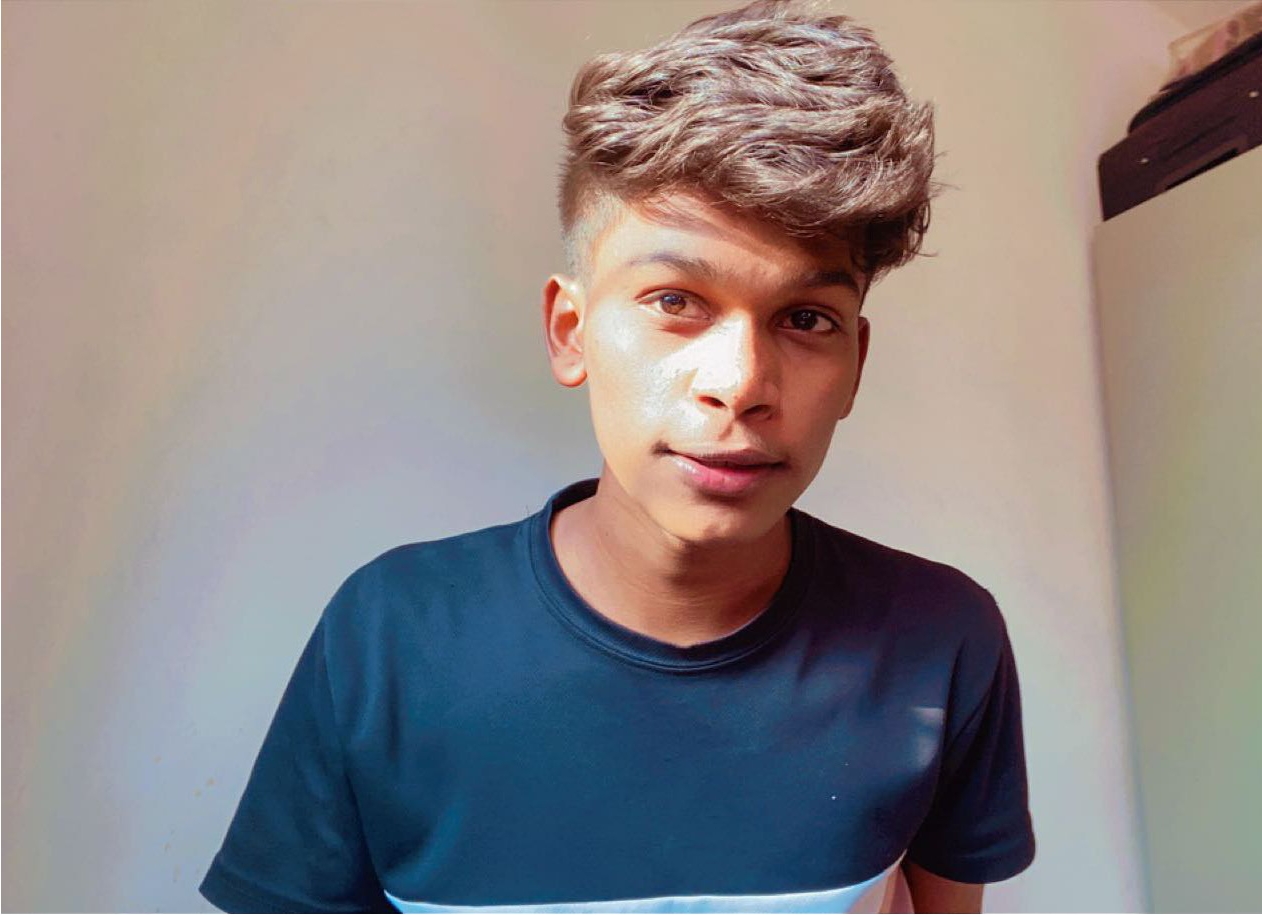
Aditya Dhaygude
Aditya Dhaygude
i Build Stuff