JavaScript Polyfills Explained: Part 1

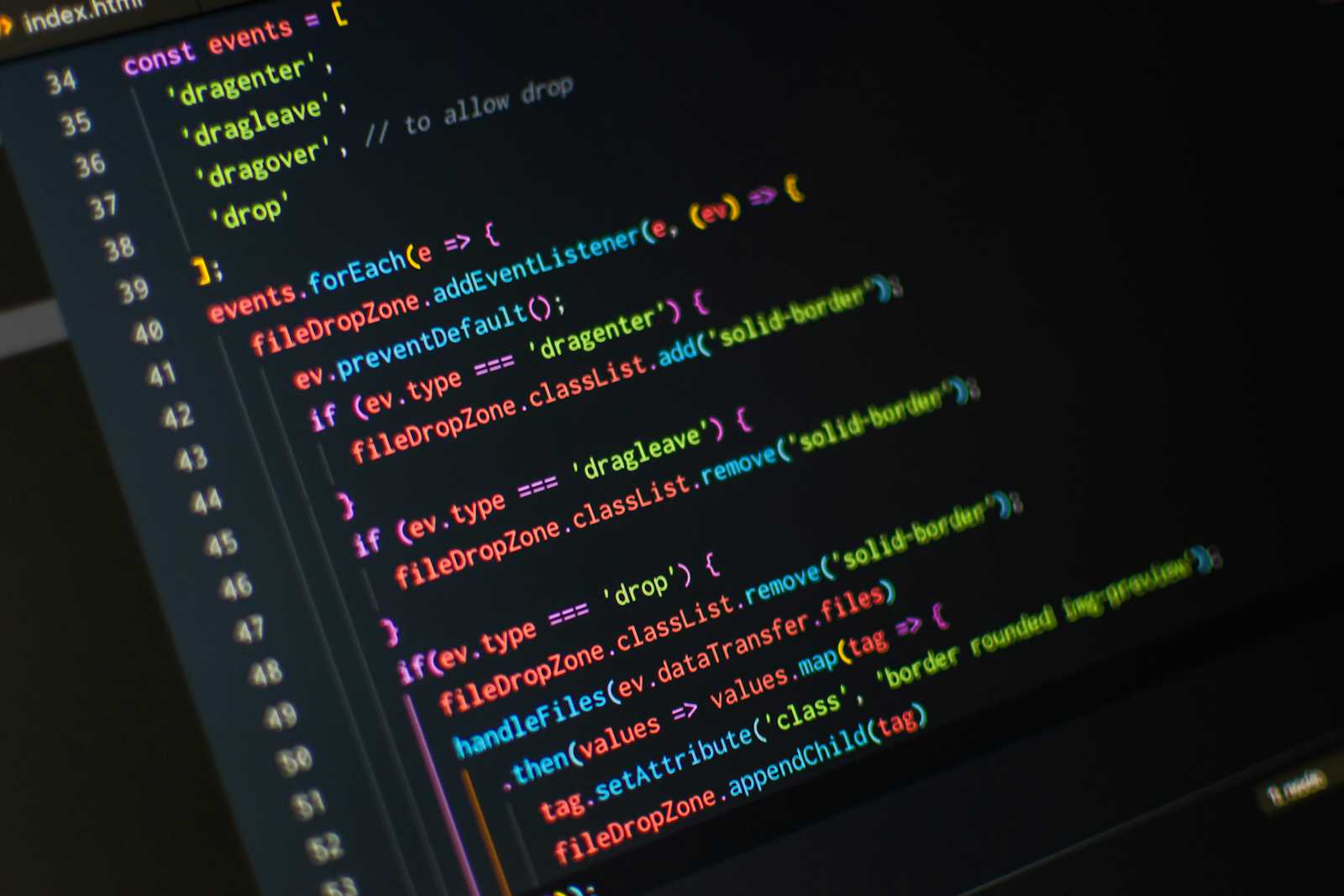
Polyfill is a piece of code (usually in JS🌍) that is used to support modern functionality in older browser versions that don’t natively support these modern functions.
In other words, some older browser versions may not support these modern ES6 features. So you may need to write your version for some of these functions or something similar.
You (certainly) need not write these pieces of code, but it is good to know about the internals of these modern JS functions for better understanding.
Below are the polyfill codes for some frequently used Array methods :
forEach
map
filter
find
reduce
flat
forEach()
let arr = [1, 2, 3, 4, 5];
console.log("<\============= forEach Polyfill ======================/>")
Array.prototype.myForEach = function(cb) {
for (let i = 0; i < this.length; i++) {
cb(this[i], i);
}
}
arr.myForEach((el, index) => {
console.log(index + " ==> " + el * 2)
})
map()
let arr = [1, 2, 3, 4, 5];
console.log("<\============= map Polyfill ======================/>")
Array.prototype.myMap = function(cb) {
let output = [];
for(let i = 0; i < this.length; i++){
output.push(cb(this[i], i));
}
return output
}
let modifiedArr = arr.myMap((el, index) => {
return 2*el + 1;
});
console.log(modifiedArr);
filter()
let arr = [1, 2, 3, 4, 5];
console.log("<\============= filter Polyfill ======================/>")
Array.prototype.myFilter = function(cb) {
let output = [];
for(let i = 0; i < this.length; i++){
if(cb(this[i])){
output.push(this[i]);
}
}
return output;
}
let filteredArray = arr.myFilter((el) => el%2 === 0);
console.log(filteredArray);
find()
let arr = [1, 2, 3, 4, 5];
console.log("<\============= find Polyfill ======================/>")
Array.prototype.myFind = function(cb) {
for(let i = 0; i < this.length; i++){
if(cb(this[i])) return this[i];
}
}
let validResult = arr.myFind((el) => el > 3);
console.log(validResult);
reduce()
let arr = [1, 2, 3, 4, 5];
console.log("<\============= reduce Polyfill ======================/>")
Array.prototype.myReduce = function(cb, initialValue) {
let aggregate = initialValue ? initialValue : this[0];
for(let i = 1; i < this.length; i++){
aggregate = cb(aggregate, this[i]);
}
return aggregate
}
let result = arr.myReduce((accumulator, el) => accumulator*el);
console.log(result);
flat()
let arr1 = [1,2,[3,4,[5]],[6],[[7]],8,[[[9]]]];;
console.log("<\============= flat Polyfill ======================/>")
Array.prototype.myFlat = function(depth = 1){
let output = [];
const flatArr = function(arr, level){
if(!Array.isArray(arr) || level === 0){
return arr;
}
let output = [];
for(let i = 0; i < arr.length; i++){
if(Array.isArray(arr[i])){
output = [...output, ...flatArr(arr[i], level - 1)];
}else{
output.push(arr[i]);
}
}
return output;
}
for(let i = 0; i < this.length; i++){
if(Array.isArray(this[i])){
output = [...output, ...flatArr(this[i], depth - 1)];
}else{
output.push(this[i]);
}
}
return output;
}
console.log(arr1.myFlat());
The next part will give an idea of the internals of Promises and Object methods.
Feel free to point out any improvements/suggestions.
Stay tuned.
References
Subscribe to my newsletter
Read articles from Mayank Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
