Ultimate Guide to Mastering LINQ in C#
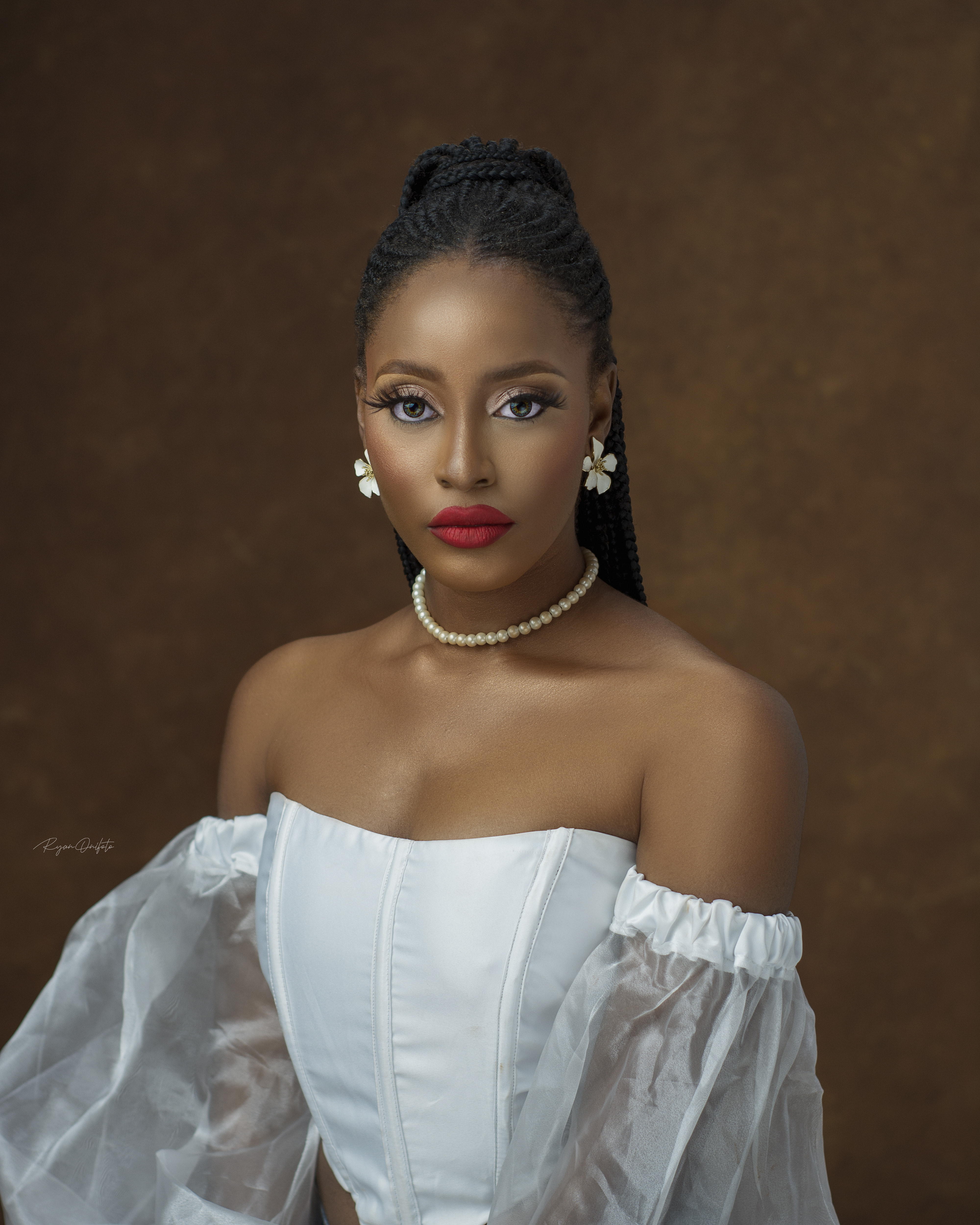
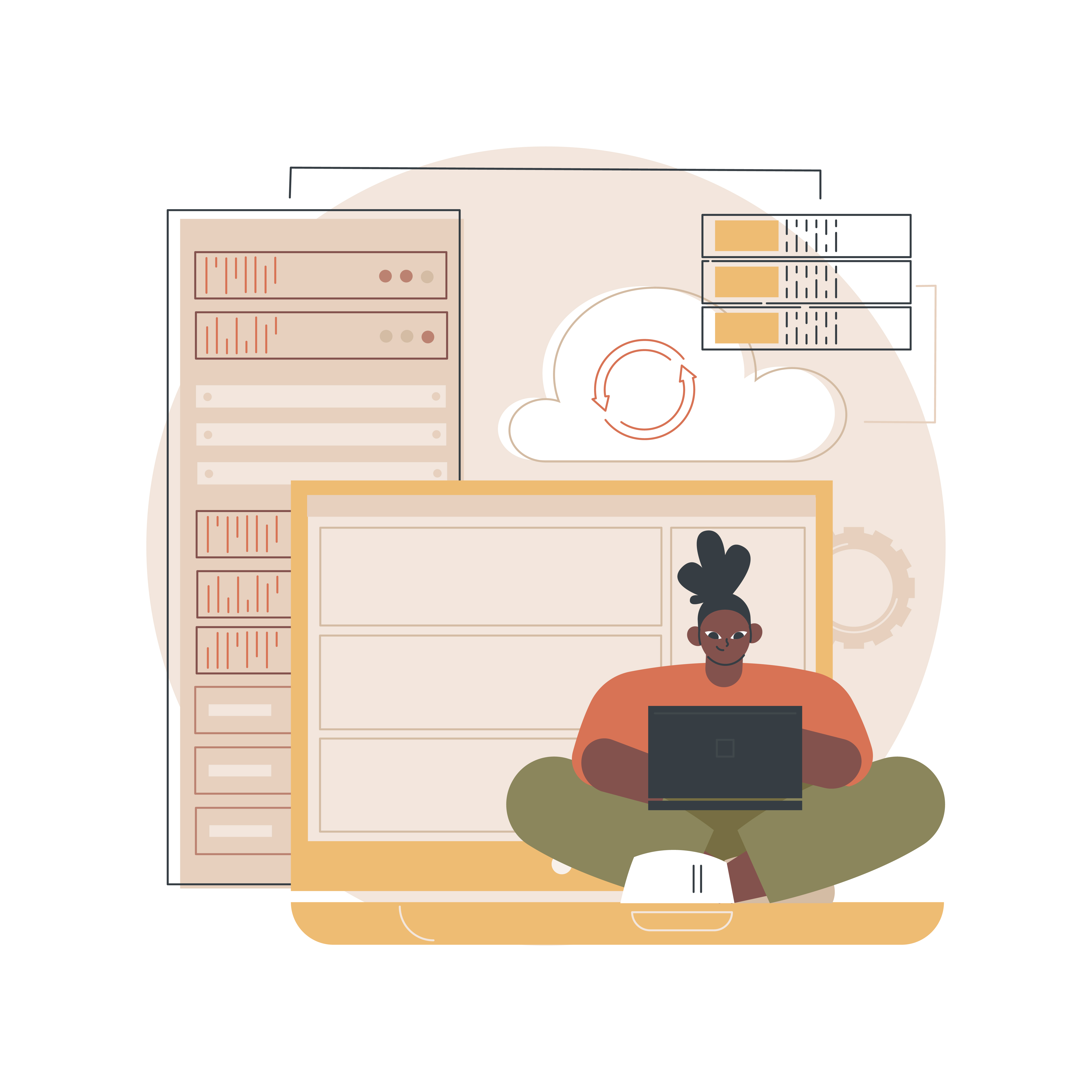
Welcome to the second article in the "Mastering C#" series. In this article, we will explore LINQ (Language Integrated Query), a powerful feature in .NET. LINQ allows you to write queries directly in C# and Visual Basic (.NET) using familiar syntax. This unified approach makes it easy to query various data sources, such as object collections, databases, and XML documents.
Pre-requisites
To fully understand and benefit from this article, readers should have the following prerequisites:
Basic Understanding of C#:
- Familiarity with the basic concepts of C#, such as variables (placeholders for data), data types (like numbers and text), loops (repeating actions), and conditionals (making decisions in code).
Object-Oriented Programming (OOP) Concepts:
- Knowledge of fundamental OOP principles like classes (blueprints for objects), objects (instances of classes), inheritance (creating new classes from existing ones), and polymorphism (using a method in different ways).
Basic Knowledge of .NET Framework:
- An understanding of the .NET ecosystem and how C# fits into it.
Familiarity with Collections:
- Awareness of common collection types in C#, such as arrays (ordered lists), lists (dynamic arrays), and dictionaries (key-value pairs), and basic operations on them.
IDE Experience:
- Experience using an Integrated Development Environment (IDE) like Visual Studio or Visual Studio Code for writing and debugging C# code.
Introduction to SQL (Optional):
- Basic knowledge of SQL (Structured Query Language) can be helpful, as LINQ queries resemble SQL syntax.
Table of Contents
Introduction to LINQ and its advantages
Getting Started with LINQ
Common LINQ queries and operations
Advanced LINQ techniques and best practices
Debugging and Testing LINQ Queries
Introduction to LINQ and Its Advantages
What is LINQ?
LINQ, which stands for Language Integrated Query, is a feature in .NET that allows you to write queries directly within your programming languages, like C# and Visual Basic. Think of it as a way to ask your code to find and manipulate data, similar to how you might use SQL to query a database, but in a much more integrated and intuitive way.
History and Evolution of LINQ
LINQ was introduced by Microsoft in 2007 as part of the .NET Framework 3.5. The goal was to make it easier for developers to work with data from different sources, such as databases, XML documents, and collections of objects. Before LINQ, working with these data sources often required learning different query languages and APIs. LINQ unified these approaches, making it simpler and more efficient to query data.
Key Advantages of Using LINQ
Consistency:
- LINQ provides a consistent way to query various data sources, whether you’re working with objects in memory, databases, or XML files.
Readability:
- LINQ queries are written in a syntax that is easy to read and understand, which makes the code more maintainable.
Integration:
- Because LINQ is integrated into C# and Visual Basic, it allows you to write queries directly within your code, without needing to learn separate query languages.
Productivity:
- LINQ can reduce the amount of code you need to write, making your development process faster and less error-prone.
Strong Typing:
- LINQ queries are checked at compile-time, which means errors can be caught early in the development process.
LINQ Providers
LINQ works with various data sources through different providers. Each provider allows LINQ to query a specific type of data source:
LINQ to Objects:
- This is used to query in-memory collections, such as arrays, lists, and other .NET collections. For example, you can use LINQ to find all the even numbers in an array of integers.
int[] numbers = { 1, 2, 3, 4, 5 };
var evenNumbers = from number in numbers
where number % 2 == 0
select number;
LINQ to SQL:
- This allows you to query SQL databases using LINQ syntax. It translates LINQ queries into SQL queries that can be executed on a database.
using (var context = new DataContext())
{
var query = from customer in context.Customers
where customer.City == "Seattle"
select customer;
}
LINQ to XML:
- This is used to query and manipulate XML documents using LINQ. It simplifies the process of working with XML data.
XDocument doc = XDocument.Load("data.xml");
var query = from element in doc.Descendants("Customer")
where (string)element.Element("City") == "Seattle"
select element;
LINQ in ASP.NET:
- This part of LINQ in ASP.NET applications enables seamless interaction with diverse data sources such as databases, XML files, and in-memory collections. It streamlines data retrieval and manipulation within web applications.
public ActionResult Index()
{
using (var context = new MyDbContext())
{
var products = from product in context.Products
where product.Category == "Electronics"
select product;
return View(products.ToList());
}
}
LINQ to Entities:
- This is part of the Entity Framework and allows you to work with relational data as domain-specific objects. It’s used to query databases using LINQ syntax in a way that integrates closely with your application’s data model.
using (var context = new MyDbContext())
{
var query = from order in context.Orders
where order.OrderDate >= new DateTime(2023, 1, 1)
select order;
}
Getting Started with LINQ
Setting Up Your Environment
Before you can start using LINQ in your C# projects, you need to set up your development environment. Here’s how you can do it:
Install Visual Studio:
- Download and install Visual Studio Community Edition from the official website. It’s free and includes all the tools you need to get started with C# and LINQ.
Create a New Project:
Open Visual Studio.
Select "Create a new project."
Choose "Console App (.NET Core)" or "Console App (.NET Framework)" depending on your preference, and click "Next."
Name your project and choose a location to save it, then click "Create."
Now, your environment is ready for you to start writing C# code with LINQ.
Basic Syntax and Structure
LINQ (Language Integrated Query) allows you to write queries directly in C#. Here are the basics of LINQ syntax and structure:
Data Source:
- LINQ queries operate on data sources like arrays, lists, or any collection that implements
IEnumerable<T>
.
- LINQ queries operate on data sources like arrays, lists, or any collection that implements
Query Creation:
- A LINQ query is created using a specific syntax that looks similar to SQL.
Query Execution:
- The query is executed to retrieve the results.
Here’s a simple example to illustrate the syntax:
// Sample data source: an array of numbers
int[] numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
// LINQ query to find even numbers
var evenNumbers = from number in numbers
where number % 2 == 0
select number;
// Execute the query and print the results
foreach (var num in evenNumbers)
{
Console.WriteLine(num);
}
Data Source:
int[] numbers = { 1, 2, 3, ... };
Query Creation:
from number in numbers where number % 2 == 0 select number;
Query Execution: Using
foreach
to iterate through the results.
Writing Your First LINQ Query
Let’s write a simple LINQ query step-by-step.
Create a Data Source:
Start by creating a data source, such as an array or list.string[] names = { "Alice", "Bob", "Charlie", "David" };
Write a LINQ Query:
Create a LINQ query to filter or transform the data. For example, let's find names that start with the letter 'A'.var namesStartingWithA = from name in names where name.StartsWith("A") select name;
from name in names
: Iterate over each element in thenames
array.where name.StartsWith("A")
: Filter names that start with 'A'.select name
: Select the filtered names.
Execute the Query:
Execute the query and display the results.foreach (var name in namesStartingWithA) { Console.WriteLine(name); }
When you run this code, it will output:
Alice
Common LINQ Queries and Operations
Filtering Data
Filtering data in LINQ allows you to select elements that meet specific conditions. The where
clause is used for filtering.
Example:
int[] numbers = { 1, 2, 3, 4, 5 };
var evenNumbers = from number in numbers
where number % 2 == 0
select number;
foreach (var num in evenNumbers)
{
Console.WriteLine(num);
}
Output:
2
4
Sorting Data
Sorting data in LINQ can be done using the orderby
clause.
Example:
string[] names = { "Charlie", "Alice", "Bob" };
var sortedNames = from name in names
orderby name
select name;
foreach (var name in sortedNames)
{
Console.WriteLine(name);
}
Output:
Alice
Bob
Charlie
Projecting Data
Projection refers to selecting specific fields from each element. The select
clause is used for projection.
Example:
var students = new[]
{
new { Name = "Alice", Age = 20 },
new { Name = "Bob", Age = 22 },
new { Name = "Charlie", Age = 23 }
};
var names = from student in students
select student.Name;
foreach (var name in names)
{
Console.WriteLine(name);
}
Output:
Alice
Bob
Charlie
Advanced LINQ Techniques and Best Practices
Joining Data
Joining data is a powerful feature of LINQ, allowing you to combine data from different sources based on a common key.
Example:
var students = new[]
{
new { StudentId = 1, Name = "Alice" },
new { StudentId = 2, Name = "Bob" }
};
var scores = new[]
{
new { StudentId = 1, Score = 90 },
new { StudentId = 2, Score = 85 }
};
var studentScores = from student in students
join score in scores on student.StudentId equals score.StudentId
select new { student.Name, score.Score };
foreach (var item in studentScores)
{
Console.WriteLine($"{item.Name}: {item.Score}");
}
Output:
Alice: 90
Bob: 85
Grouping Data
Grouping data allows you to organize elements into groups based on a common key.
Example:
var students = new[]
{
new { Name = "Alice", Age = 20 },
new { Name = "Bob", Age = 22 },
new { Name = "Charlie", Age = 20 }
};
var groups = from student in students
group student by student.Age into ageGroup
select ageGroup;
foreach (var group in groups)
{
Console.WriteLine($"Age: {group.Key}");
foreach (var student in group)
{
Console.WriteLine($" - {student.Name}");
}
}
Output:
Age: 20
- Alice
- Charlie
Age: 22
- Bob
Debugging and Testing LINQ Queries
Debugging and testing LINQ queries is crucial to ensure their correctness and efficiency. Here are some tips:
Use the Immediate Window:
In Visual Studio, you can use the Immediate Window to test LINQ queries on the fly.Breakpoints:
Set breakpoints to inspect variables and query results at runtime.Unit Tests:
Write unit tests to verify the correctness of your LINQ queries.Profiling Tools:
Use profiling tools to analyze the performance of your LINQ queries and identify any bottlenecks.
Conclusion
In this article, we explored the basics of LINQ, including its history, advantages, and various providers. LINQ offers a consistent and readable way to query different data sources directly within C# and Visual Basic, enhancing productivity and code maintainability.
Recommended Books, and Tutorials
Books:
"Pro LINQ: Language Integrated Query in C# 2010" by Joe Rattz
"LINQ in Action" by Steve Eichert and Jim Wooley
Tutorials:
Microsoft's Official LINQ Documentation and Tutorials
Pluralsight's LINQ Courses
Congratulations on completing the second article in the "Mastering C#" series! You have learned about LINQ, its advantages, and how to use it to query various data sources. By mastering LINQ, you can write more efficient and readable code, making your development process faster and more enjoyable.
In the next article, we will dive into Unit Testing in C# with xUnit.
Stay tuned and Happy coding!
Subscribe to my newsletter
Read articles from Opaluwa Emidowo-ojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
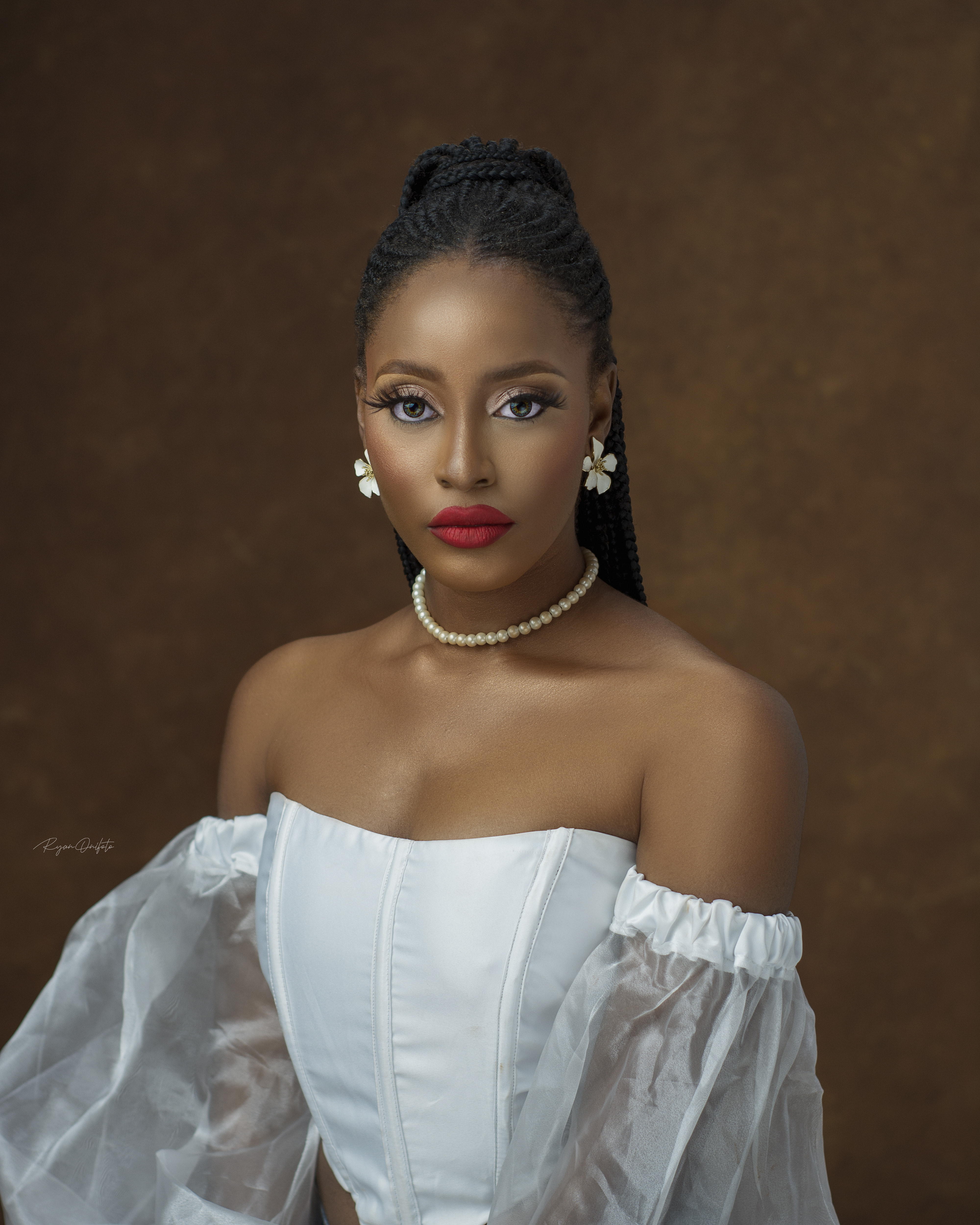