Comprehensive Guide to Advanced YAML
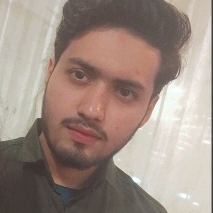
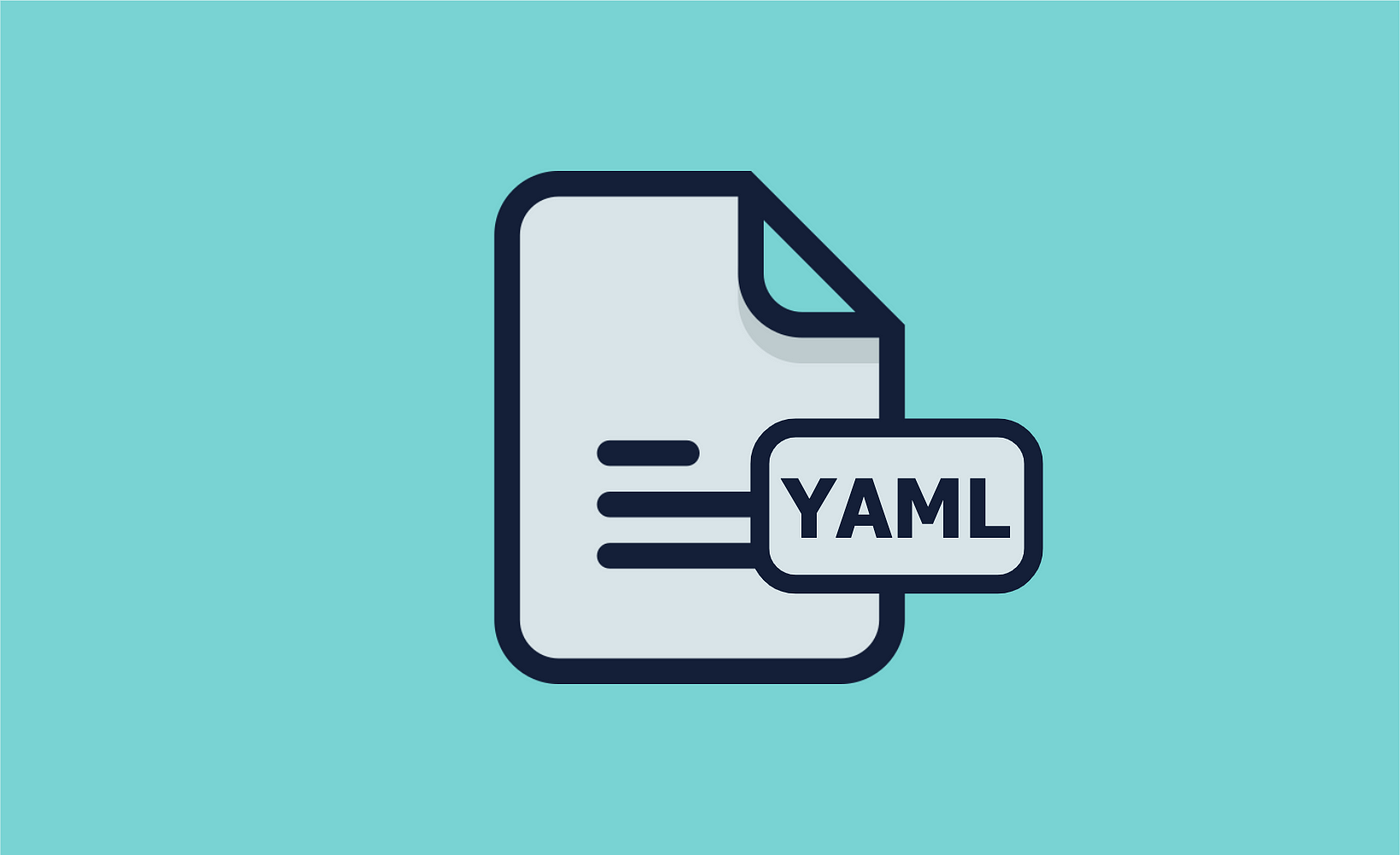
Here is an advanced tutorial on YAML, covering more complex structures and features:
1. Introduction to YAML
YAML (YAML Ain't Markup Language) is a human-readable data serialization standard. It can be used with all programming languages and is often used for writing configuration files.
2. Basic Syntax Recap
Scalars: Single values like strings, integers, floats, booleans.
Lists: Ordered collections of items.
Dictionaries: Key-value pairs, also called maps or hashes.
3. Advanced Features
3.1. Multi-line Strings
There are two ways to write multi-line strings in YAML:
Literal Block Scalar (
|
): Retains line breaks.Folded Block Scalar (
>
): Folds newlines into spaces.
literal_block: |
This is a
multi-line string
with line breaks.
folded_block: >
This is a
multi-line string
with folded
line breaks.
3.2. Advanced Data Structures
- Nested Maps and Lists:
person:
name: John Doe
age: 30
address:
street: 123 Main St
city: Anytown
zip: 12345
hobbies:
- hiking
- reading
- cooking
- Mixed Content:
mixed:
- name: John
age: 30
- name: Jane
age: 25
hobbies:
- painting
- cycling
3.3. Aliases and Anchors
YAML supports referencing nodes with anchors and aliases. This can help avoid duplication.
defaults: &defaults
adapter: postgres
host: localhost
development:
database: dev_db
<<: *defaults
test:
database: test_db
<<: *defaults
3.4. Merging Nodes
YAML allows merging multiple maps using the merge key (<<
).
base: &base
name: Base
value: 100
extension:
<<: *base
additional: 200
3.5. Complex Keys
YAML lets you use complex keys in maps, including sequences and maps.
?
- name
- age
: 25
3.6. Data Types
YAML supports various data types, including:
Integers:
123
,0o173
,0x7B
Floats:
123.45
,1.23e+2
Booleans:
true
,false
Null:
null
or~
Dates:
2024-06-28
Timestamps:
2024-06-28T14:21:00Z
4. Custom Tags
YAML allows the use of custom tags to denote data types or structures.
custom_tag: !mytag
key: value
5. Using YAML in Code
YAML can be easily parsed and generated in many programming languages using libraries like PyYAML for Python, yaml
for Ruby, and more.
import yaml
data = yaml.safe_load("""
person:
name: John Doe
age: 30
""")
print(data['person']['name']) # Output: John Doe
6. Error Handling and Validation
When working with YAML, it's important to handle errors and validate data structures. Tools and libraries often provide features for validation.
try:
data = yaml.safe_load(yaml_string)
except yaml.YAMLError as exc:
print(f"Error parsing YAML: {exc}")
Conclusion
This advanced tutorial covers the more complex aspects of YAML, including multi-line strings, advanced data structures, aliases, anchors, merging, complex keys, custom tags, and more. Understanding these features can help you use YAML effectively for configuration, data serialization, and other tasks.
Subscribe to my newsletter
Read articles from Mohmmad Saif directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
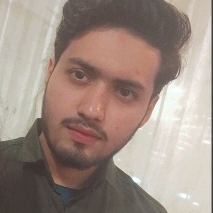
Mohmmad Saif
Mohmmad Saif
👋Hello I am Mohd Saif, passionate technology enthusiast currently pursuing a bachelor of Computer application degree. 🎓Education: I am currently pursuing a bachelor of Computer application degree with the focus on coding at Bareilly University my education journey has equipped me with strong foundation in Computer science and I am eager to apply my knowledge to real word challenges. 💡Passion for technology: I have always been deeply passionate about technology and I am particular drawn to devops with AWS. 🚀Skills: 🔹Linux 🔹Shell scripting 🔹Python 🔹Ansible 🔹Docker 🔹Kubernetes 🔹Jenkins CI/CD 🔹Maven 🔹Git and GitHub ✨Future goals: My goal is to facilitate seamless collaboration between development and operations teams, ensuring faster releases and high-quality software. I am a proactive learner, constantly exploring new DevOps trends and practices