Daily Hack #day86 - JUnit 5 @MethodSource
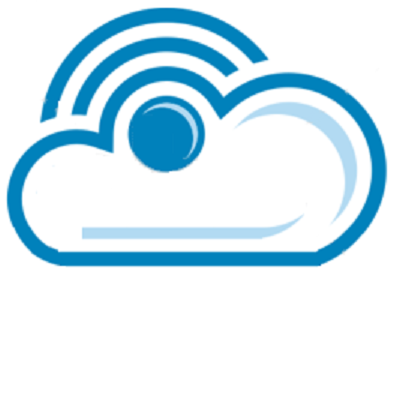
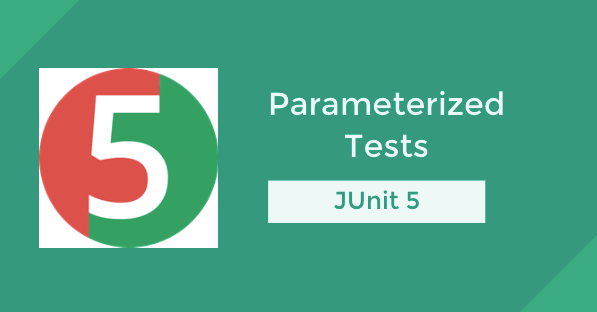
MethodSource in JUnit 5
@MethodSource
is an annotation in JUnit 5 that allows for parameterized tests using a method as a source of arguments. This method is particularly useful for generating complex test data or reusing common data sets across multiple tests.
Key Features:
Dynamic Data Generation: The method specified by
@MethodSource
can dynamically generate a stream of arguments, enabling more flexible and comprehensive testing scenarios.Reusability: Test data can be generated in one place and reused across multiple test methods, promoting cleaner and more maintainable code.
Compatibility with Various Data Types: The source method can return a stream, collection, array, or iterable of arguments.
Usage:
Defining the Source Method:
The source method must be static.
It should return a
Stream
,Collection
,Iterable
, or an array of arguments.
Annotating the Test Method:
- Use
@MethodSource
to specify the source method.
- Use
Example:
import static org.junit.jupiter.api.Assertions.assertTrue;
import java.util.stream.Stream;
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.MethodSource;
public class MethodSourceExampleTest {
// Source method that provides test data
static Stream<String> stringProvider() {
return Stream.of("apple", "banana", "cherry");
}
@ParameterizedTest
@MethodSource("stringProvider")
void testWithStringProvider(String argument) {
// Test logic
assertTrue(argument.length() > 0);
}
}
In this example:
stringProvider()
is a static method that returns aStream<String>
.testWithStringProvider(String argument)
is a parameterized test method that will be executed three times, once for each string in the stream ("apple"
,"banana"
,"cherry"
).
Benefits:
Flexibility:
@MethodSource
allows for generating complex and varied test data sets, improving test coverage.Readability: Test methods can be more readable and concise by separating data generation from test logic.
Maintainability: Centralized data generation methods can simplify maintenance, especially when test data needs to be updated or extended.
@MethodSource
is a powerful feature in JUnit 5 for parameterized testing, enabling more dynamic and reusable test setups.
Subscribe to my newsletter
Read articles from Cloud Tuned directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
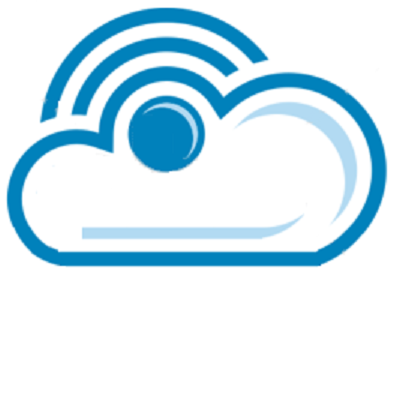