How to create toggle sidebar that is smooth af
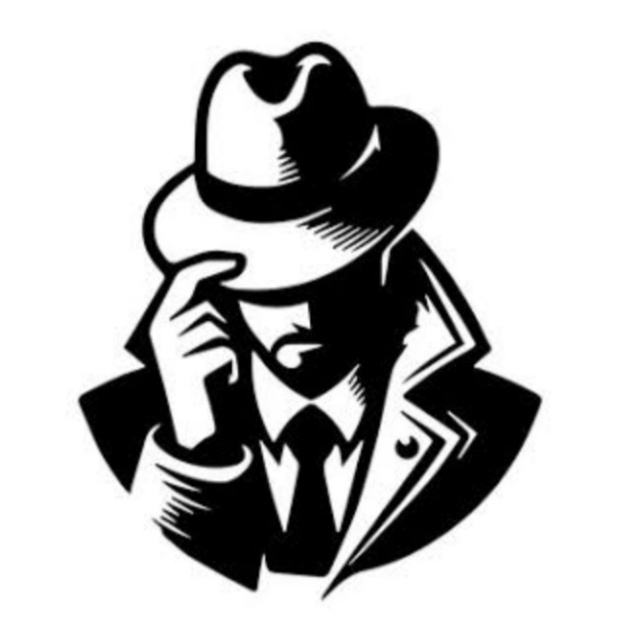
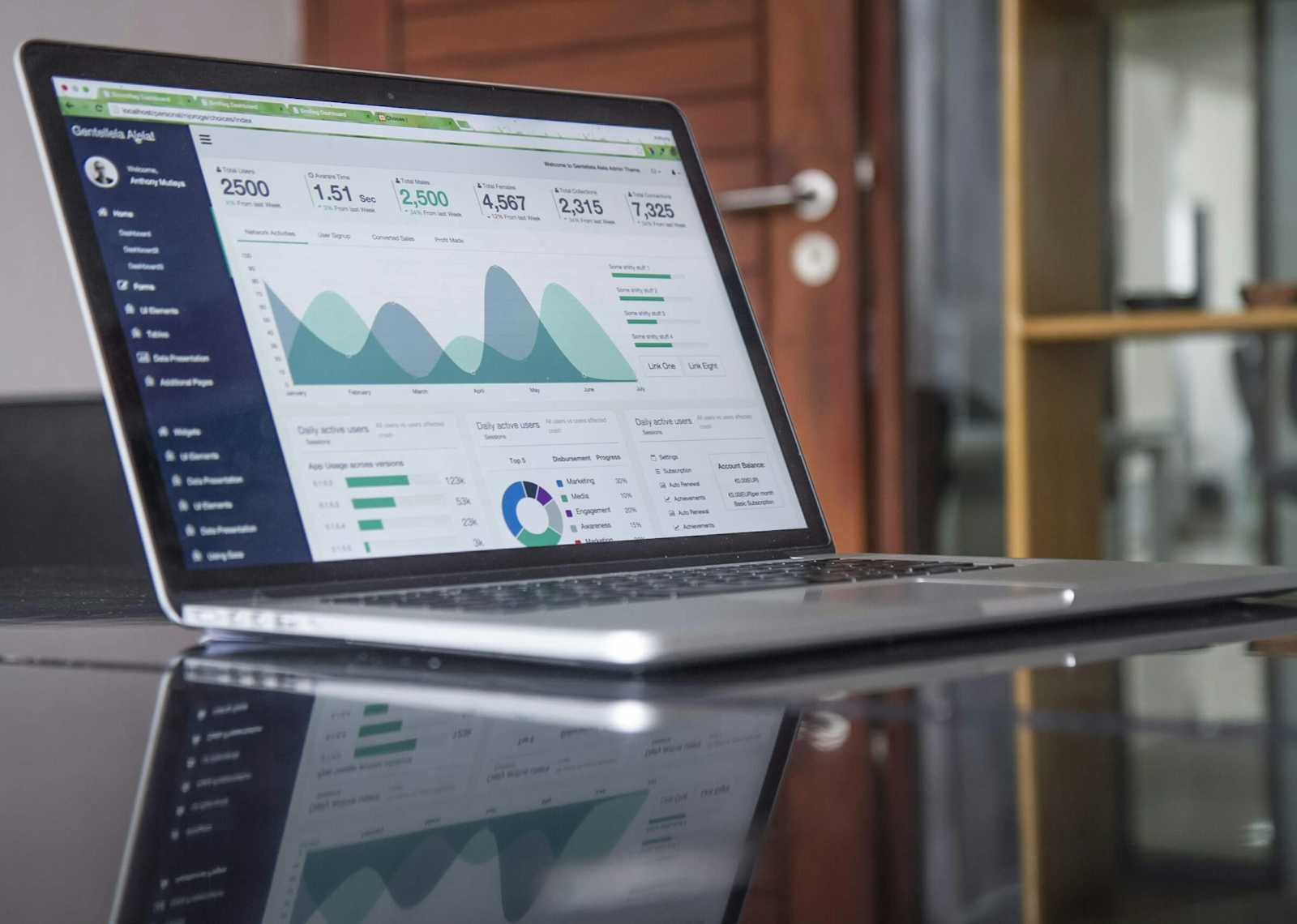
Explanation is given after the code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Interactive Sidebar Example</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
display: flex;
}
.sidebar {
width: 250px;
height: 100%;
background-color: #f0f0f0;
position: fixed;
left: -250px;
top: 0;
transition: left 0.3s ease;
z-index: 1000; /* Ensure sidebar is on top */
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
padding: 20px;
}
.sidebar.open {
left: 0;
}
.sidebar h2 {
color: #333;
text-align: center;
margin-bottom: 20px;
}
.sidebar .close-btn {
position: absolute;
top: 10px;
right: 10px;
cursor: pointer;
font-size: 20px;
color: #666;
}
.sidebar ul {
list-style-type: none;
padding: 0;
}
.sidebar ul li {
margin-bottom: 10px;
}
.sidebar ul li a {
text-decoration: none;
color: #333;
display: block;
padding: 5px;
transition: background-color 0.3s ease;
}
.sidebar ul li a:hover {
background-color: #ddd;
}
.content {
flex: 1;
padding: 20px;
}
</style>
</head>
<body>
<div class="sidebar" id="sidebar">
<span class="close-btn" onclick="closeSidebar()">×</span>
<h2>Sidebar</h2>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Contact</a></li>
</ul>
</div>
<div class="content">
<h2>Main Content Area</h2>
<p>This is the main content area. Content goes here.</p>
</div>
<button onclick="toggleSidebar()">Toggle Sidebar</button>
<script>
const sidebar = document.getElementById('sidebar');
function toggleSidebar() {
sidebar.classList.toggle('open');
}
function closeSidebar() {
sidebar.classList.remove('open');
}
// Close sidebar if clicked outside
window.addEventListener('click', function(event) {
if (!event.target.closest('.sidebar') && !event.target.matches('button')) {
closeSidebar();
}
});
</script>
</body>
</html>
Explanation
HTML and CSS
The sidebar (
<div class="sidebar">
) is initially positioned off-screen (left: -250px;
) and slides into view when the.open
class is applied (left: 0;
).A close button (
<span class="close-btn" onclick="closeSidebar()">×</span>
) is positioned at the top right of the sidebar to close it when clicked.The main content area (
<div class="content">
) occupies the remaining space usingflex: 1;
.
JavaScript
toggleSidebar()
function toggles the.open
class on the sidebar, allowing it to slide in and out of view.closeSidebar()
function removes the.open
class from the sidebar, closing it.window.addEventListener('click', function(event) {...})
listens for clicks anywhere in the window. If the click is outside the sidebar and not on the toggle button (button
), it closes the sidebar.
Toggle Button
- A simple toggle button (
<button onclick="toggleSidebar()">Toggle Sidebar</button>
) is included at the end of the document to demonstrate opening and closing the sidebar. In a real application, you might triggertoggleSidebar()
based on user interaction or screen size.
Conclusion
This example provides an interactive sidebar that can be toggled open and closed using a button and a close button within the sidebar itself. It also automatically closes when clicking outside of it. Adjust the styles and functionality further to suit your specific design requirements and application needs.
Subscribe to my newsletter
Read articles from Maneeshwar Karne directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
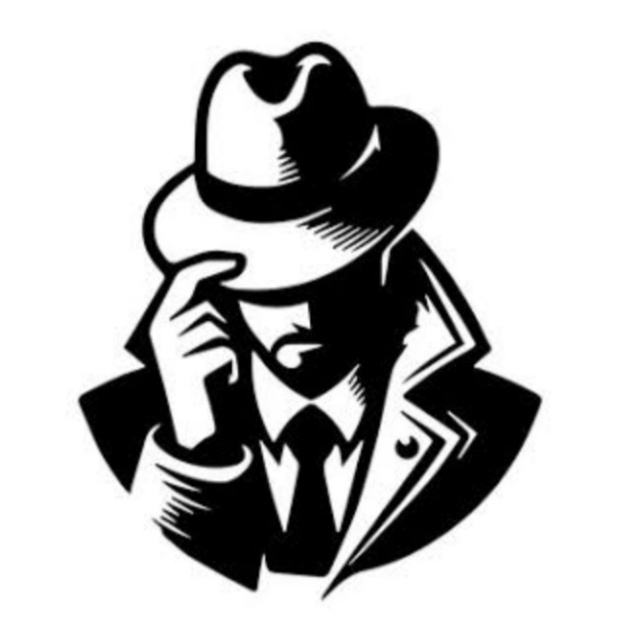
Maneeshwar Karne
Maneeshwar Karne
Learning web devlelopment