Routing in React with React Router

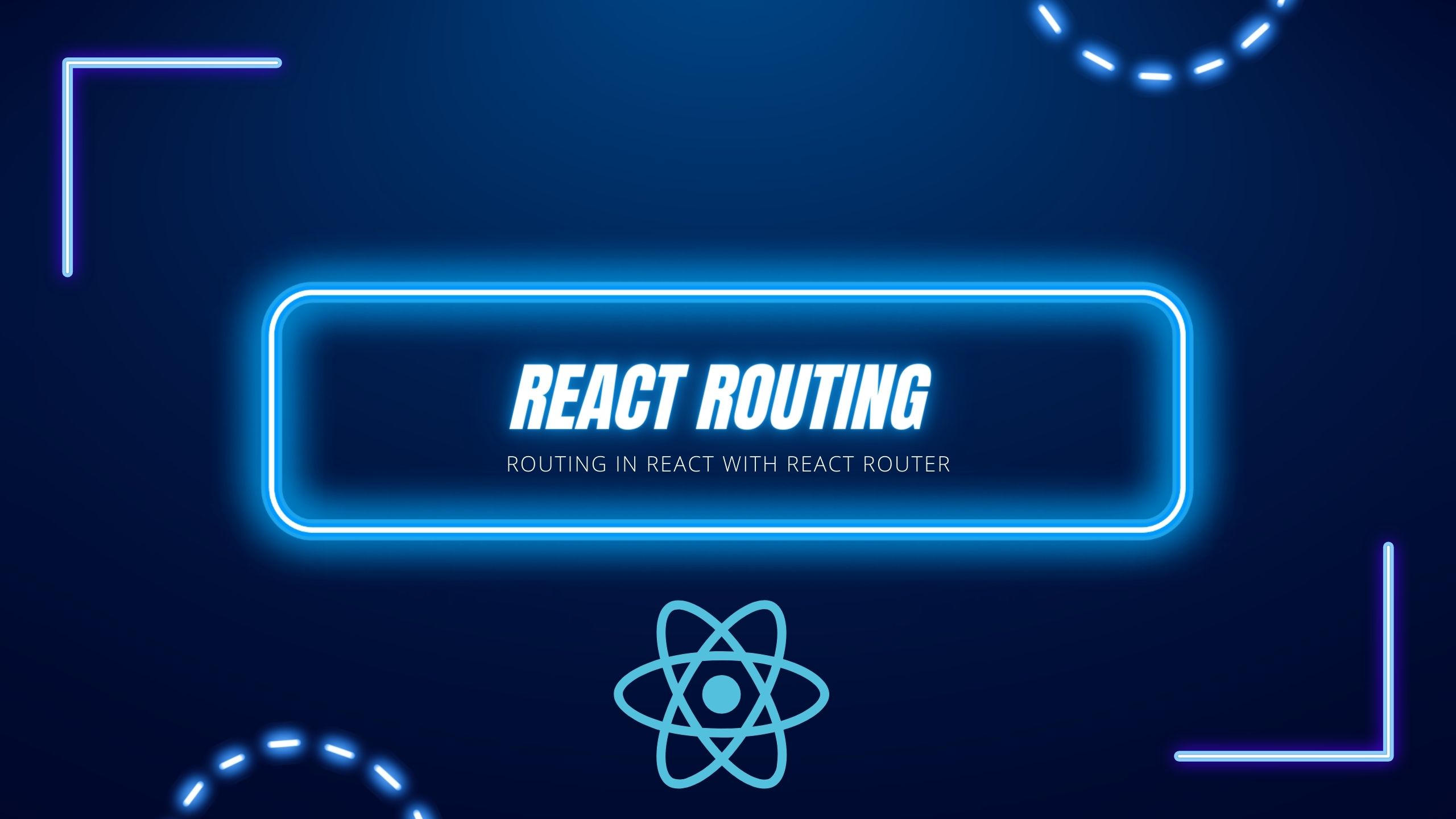
Introduction to React Router
React Router is an essential tool for managing navigation in modern web applications. It facilitates seamless transitions and enhances user experience in single-page applications (SPAs). Understanding the role and setup of React Router is crucial for any React developer aiming to build sophisticated, user-friendly web apps.
The Importance of Routing in Single Page Applications
Understanding SPAs
Single Page Applications (SPAs) have revolutionized web development by loading a single HTML page and dynamically updating content as the user interacts with the app. This approach eliminates the need for page reloads, providing a faster and more fluid user experience. SPAs rely heavily on JavaScript to manage the state and render components, making routing a central aspect of their architecture.
Role of Routing in SPAs
In SPAs, routing is the mechanism that enables navigation between different views or components without triggering a full page reload. By managing the application’s state and URL in sync, routing ensures that users can navigate through the app as if they were browsing multiple pages. This seamless navigation is critical for maintaining a coherent and responsive user interface.
Overview of React Router
What is React Router?
React Router is a standard library for routing in React applications. It enables developers to define multiple routes in an application, linking URLs to specific components. React Router abstracts the complexities of navigation and URL management, providing a simple and declarative approach to implement routing in SPAs.
Benefits of Using React Router
React Router offers several advantages:
Declarative Routing: Simplifies route definitions through JSX.
Dynamic Routing: Adjusts to changes in the app’s state and URL.
Nested Routes: Supports nested route structures for complex UIs.
Code Splitting: Enhances performance by loading only the necessary components.
Browser and Hash History: Supports both history modes for compatibility and flexibility.
Setting Up React Router in a React Project
Prerequisites for Using React Router
Required Tools and Libraries
To use React Router, you need:
Node.js and npm (or yarn) for package management.
A React project set up using Create React App or a custom configuration.
Basic Understanding of React
A fundamental understanding of React concepts such as components, state, and props is necessary. Familiarity with ES6 syntax and JavaScript modules will also be beneficial.
Installing React Router
Step-by-Step Installation Guide
Install React Router: Run
npm install react-router-dom
to add React Router to your project.Set Up BrowserRouter: Import
BrowserRouter
in your main application file (e.g.,index.js
) and wrap yourApp
component with it.
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter } from 'react-router-dom';
import App from './App';
ReactDOM.render(
<BrowserRouter>
<App />
</BrowserRouter>,
document.getElementById('root')
);
Verifying Installation
Ensure React Router is correctly installed by defining a basic route and checking if it renders the corresponding component.
Basic Routing Concepts
Defining Routes
What Are Routes?
Routes define the relationship between URLs and the components rendered by the application. Each route specifies a path and the component to be displayed when the URL matches that path.
How to Define Routes in React Router
Use the Route
component to define routes. The path
prop specifies the URL, and the component
prop specifies the component to render.
import { Route, Switch } from 'react-router-dom';
const App = () => (
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
);
Navigating Between Routes
Using the Link Component
The Link
component creates navigable links that users can click to navigate between routes without a full page reload.
import { Link } from 'react-router-dom';
const Navigation = () => (
<nav>
<Link to="/">Home</Link>
<Link to="/about">About</Link>
</nav>
);
Programmatic Navigation with useHistory
The useHistory
hook allows for programmatic navigation within a component.
import { useHistory } from 'react-router-dom';
const NavigateButton = () => {
let history = useHistory();
const handleClick = () => {
history.push('/about');
};
return <button onClick={handleClick}>Go to About</button>;
};
Route Parameters and URL Parameters
Understanding Route Parameters
Route parameters are dynamic segments in a URL that can be used to pass data to components. They are defined using the colon syntax in the route path.
<Route path="/user/:id" component={UserProfile} />
Extracting and Using Parameters
The useParams
hook retrieves route parameters, enabling their use within a component.
import { useParams } from 'react-router-dom';
const UserProfile = () => {
let { id } = useParams();
return <div>User ID: {id}</div>;
};
Advanced Routing Techniques
Nested Routes
Creating Nested Routes
Nested routes allow for hierarchical navigation structures, where routes can be defined within other routes.
const Dashboard = () => (
<Switch>
<Route path="/dashboard/overview" component={Overview} />
<Route path="/dashboard/settings" component={Settings} />
</Switch>
);
Benefits of Nested Routing
Nested routing supports modular component structures and improves code organization by keeping related routes and components together.
Dynamic Routing
Implementing Dynamic Routes
Dynamic routes enable components to render based on the URL and route parameters, supporting flexible and context-aware navigation.
<Route path="/products/:productId" component={ProductDetail} />
Use Cases for Dynamic Routing
Dynamic routing is useful for applications with resource-based navigation, such as e-commerce sites displaying product details based on the product ID in the URL.
Redirects and Navigation Guards
Setting Up Redirects
Redirects automatically navigate users from one route to another, useful for route changes or authentication flows.
import { Redirect } from 'react-router-dom';
<Route path="/old-path">
<Redirect to="/new-path" />
</Route>
Protecting Routes with Navigation Guards
Navigation guards restrict access to certain routes based on conditions such as user authentication.
const PrivateRoute = ({ component: Component, ...rest }) => (
<Route {...rest} render={(props) => (
isAuthenticated
? <Component {...props} />
: <Redirect to="/login" />
)} />
);
Handling 404 Errors and Fallbacks
Configuring a 404 Route
Setting Up a Catch-All Route
A catch-all route handles undefined URLs, guiding users to a 404 page.
<Route component={NotFound} />
Custom 404 Pages
Custom 404 pages enhance user experience by providing helpful navigation links and a friendly message.
Fallback UI Components
Using Suspense and Error Boundaries
Suspense and Error Boundaries handle loading states and errors gracefully, enhancing the robustness of the app.
import { Suspense } from 'react';
const App = () => (
<Suspense fallback={<div>Loading...</div>}>
<Routes />
</Suspense>
);
Creating User-Friendly Fallbacks
User-friendly fallbacks provide meaningful feedback during loading or error states, maintaining a positive user experience.
Optimizing Performance with Code Splitting
Introduction to Code Splitting
What is Code Splitting?
Code splitting breaks down the application’s code into smaller bundles, loading only the necessary code for a particular route.
Benefits of Code Splitting
Code splitting improves performance by reducing initial load times and optimizing resource usage.
Implementing Code Splitting with React Router
Using React.lazy and Suspense
React.lazy and Suspense simplify the implementation of code splitting by dynamically loading components.
const LazyComponent = React.lazy(() => import('./LazyComponent'));
const App = () => (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
Best Practices for Code Splitting
Organize code splitting to balance between smaller bundles and maintainable code structure, avoiding excessive fragmentation.
Managing State with React Router
Passing State Through Routes
Using the Link Component's state Prop
The state prop of the Link component passes state directly through navigation, useful for passing non-URL data.
<Link to={{ pathname: '/details', state: { fromDashboard: true } }}>Details</Link>
Accessing Passed State in Components
Access the passed state within the component using the useLocation hook.
import { useLocation } from 'react-router-dom';
const Details = () => {
let location = useLocation();
return <div>{location.state.fromDashboard}</div>;
};
Integrating React Router with State Management Libraries
Using React Router with Redux
Combine React Router with Redux to manage application state and navigation seamlessly.
import { connect } from 'react-redux';
import { push } from 'connected-react-router';
const mapDispatchToProps = (dispatch) => ({
navigate: (path) => dispatch(push(path))
});
Combining React Router with Context API
Utilize the Context API to share state and navigation logic across the application.
const AuthContext = React.createContext();
const App = () => (
<AuthContext.Provider value={auth}>
<Routes />
</AuthContext.Provider>
);
Animations
and Transitions in React Router
Adding Basic Route Transitions
Implementing Simple Transitions
Simple transitions enhance user experience by animating route changes.
import { CSSTransition, TransitionGroup } from 'react-transition-group';
const App = () => (
<TransitionGroup>
<CSSTransition key={location.key} classNames="fade" timeout={300}>
<Switch location={location}>
<Route path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
</CSSTransition>
</TransitionGroup>
);
Libraries for Route Animations
Libraries like React Transition Group and Framer Motion provide powerful tools for creating sophisticated animations.
Advanced Animations with React Transition Group
Setting Up React Transition Group
React Transition Group offers advanced features for controlling the lifecycle of animations and transitions.
Creating Seamless Page Transitions
Combine React Transition Group with CSS or JavaScript animations to create seamless and engaging page transitions.
Handling Authentication and Authorization
Protecting Routes with Authentication
Implementing Private Routes
Private routes ensure that only authenticated users can access certain parts of the application.
const PrivateRoute = ({ component: Component, ...rest }) => (
<Route {...rest} render={(props) => (
isAuthenticated
? <Component {...props} />
: <Redirect to="/login" />
)} />
);
Redirecting Unauthenticated Users
Redirect unauthenticated users to login pages or other appropriate destinations, ensuring secure access control.
Role-Based Access Control
Setting Up Role-Based Routing
Role-based routing restricts access based on user roles, supporting complex access control scenarios.
const RoleBasedRoute = ({ component: Component, roles, ...rest }) => (
<Route {...rest} render={(props) => (
userHasRequiredRole(roles)
? <Component {...props} />
: <Redirect to="/unauthorized" />
)} />
);
Handling Permissions and Access
Manage permissions and access efficiently by centralizing role definitions and access logic.
Testing React Router Components
Writing Unit Tests for Routes
Tools for Testing React Router
Use testing libraries like Jest and React Testing Library to write unit tests for routes and navigation logic.
import { render } from '@testing-library/react';
import { MemoryRouter } from 'react-router-dom';
test('renders home component', () => {
render(
<MemoryRouter initialEntries={['/']}>
<App />
</MemoryRouter>
);
});
Best Practices for Route Testing
Isolate route tests to ensure they are independent and comprehensive, covering all navigation scenarios.
End-to-End Testing with React Router
Setting Up Cypress or Selenium
Cypress and Selenium offer robust tools for end-to-end testing, simulating user interactions across the entire application.
Writing Comprehensive E2E Tests
Write end-to-end tests to validate the complete user journey, from navigation to state management and component rendering.
Real-World Use Cases and Examples
Building a Blog with React Router
Structuring Routes for a Blog
Define routes for posts, categories, and archives, ensuring intuitive navigation.
const Blog = () => (
<Switch>
<Route exact path="/blog" component={PostList} />
<Route path="/blog/:postId" component={PostDetail} />
</Switch>
);
Managing Content Navigation
Implement pagination and filtering to manage large volumes of content effectively.
Creating a Multi-Step Form
Implementing Step-Based Navigation
Use React Router to create multi-step forms, guiding users through a sequence of steps.
const FormWizard = () => (
<Switch>
<Route path="/step1" component={Step1} />
<Route path="/step2" component={Step2} />
<Route path="/step3" component={Step3} />
</Switch>
);
Handling Form State Across Steps
Persist form state across steps using React state or context, ensuring data integrity.
Developing an E-Commerce Site
Setting Up Product and Category Routes
Define routes for product listings, categories, and individual product pages.
const Shop = () => (
<Switch>
<Route exact path="/shop" component={CategoryList} />
<Route path="/shop/:categoryId" component={ProductList} />
<Route path="/product/:productId" component={ProductDetail} />
</Switch>
);
Managing Shopping Cart Navigation
Implement routes for the shopping cart, checkout process, and order confirmation.
Best Practices and Common Pitfalls
Best Practices for Using React Router
Structuring Your Routes
Organize routes logically, grouping related routes and maintaining a clear structure.
Ensuring SEO Friendliness
Enhance SEO by using descriptive URLs, server-side rendering, and meta tags.
Avoiding Common Mistakes
Common Routing Errors and Fixes
Identify and fix common routing issues, such as mismatched paths and unhandled redirects.
Tips for Debugging Route Issues
Utilize browser dev tools and logging to troubleshoot and resolve routing problems effectively.
Conclusion
Recap of Key Concepts
React Router simplifies navigation in React applications, offering robust features for defining routes, handling dynamic content, and managing navigation state.
Further Reading and Resources
Explore additional resources, including the official React Router documentation, advanced tutorials, and community plugins to enhance your routing skills further.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
R3F | Laravel | Babylon | Three.js | WordPress Performance Enthusiast