Mastering LeetCode: Solving the "Product of Array Except Self" Problem
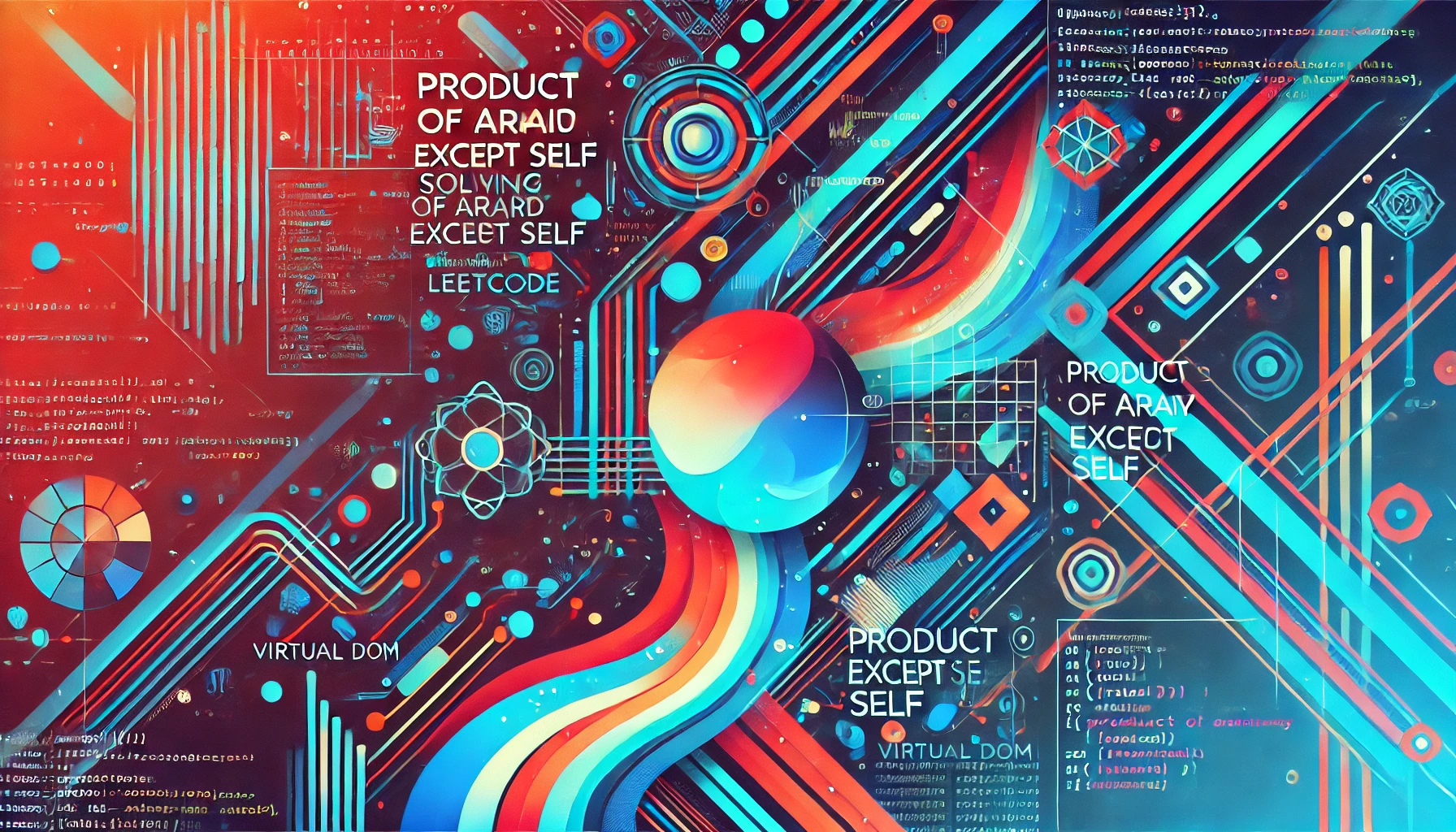
Introduction
Solving algorithmic problems is a critical skill for any aspiring software engineer, and LeetCode offers a treasure trove of challenges to hone this skill. One such problem is the "Product of Array Except Self," a classic problem that tests your ability to manipulate arrays and optimize code performance.
In this article, we will explore two efficient approaches to solve this problem, ensuring a deep understanding of the underlying concepts.
Problem Statement
The problem statement is the following:
Given an integer array
nums
, return an arrayanswer
such thatanswer[i]
is equal to the product of all the elements ofnums
exceptnums[i]
.The product of any prefix or suffix of
nums
is guaranteed to fit in a 32-bit integer.You must write an algorithm that runs in
O(n)
time and without using the division operation.Example 1:
Input: nums = [1,2,3,4] Output: [24,12,8,6]
Example 2:
Input: nums = [-1,1,0,-3,3] Output: [0,0,9,0,0]
Approach Overview
The key challenge in this problem is to compute the product of all elements except the current one without using division and in linear time. We will discuss two different approaches, each with its unique implementation and advantages:
Using Left and Right Product Arrays
Using Prefix and Suffix Products with Single Array
Detailed Approaches
1. Using Left and Right Product Arrays
Explanation: This approach involves creating two auxiliary arrays, one for the product of elements to the left of each index and one for the product of elements to the right.
Step-by-Step Breakdown:
Initialize two arrays,
left_products
andright_products
, both of sizen
.Compute the products of all elements to the left of each index.
Compute the products of all elements to the right of each index.
Multiply the corresponding elements of
left_products
andright_products
to get the final result.
Code Implementation:
from typing import List
def product_except_self(nums: List[int]) -> List[int]:
n = len(nums)
left_products = [1] * n
right_products = [1] * n
answer = [1] * n
# Calculate left products
for i in range(1, n):
left_products[i] = left_products[i - 1] * nums[i - 1]
# Calculate right products
for i in range(n - 2, -1, -1):
right_products[i] = right_products[i + 1] * nums[i + 1]
# Calculate the answer array
for i in range(n):
answer[i] = left_products[i] * right_products[i]
return answer
# Example usage
print(product_except_self([1, 2, 3, 4])) # Output: [24, 12, 8, 6]
print(product_except_self([-1, 1, 0, -3, 3])) # Output: [0, 0, 9, 0, 0]
Pros and Cons:
Pros: Simple to understand and implement.
Cons: Uses extra space for the two auxiliary arrays.
2. Using Prefix and Suffix Products with Single Array
Explanation: This method optimizes space by calculating the prefix and suffix products directly in the result array.
Step-by-Step Breakdown:
Initialize the result array with 1s.
Compute the prefix products and store them in the result array.
Compute the suffix products and multiply them directly into the result array.
Code Implementation:
from typing import List
def product_except_self(nums: List[int]) -> List[int]:
n = len(nums)
answer = [1] * n
# Calculate prefix products and store in answer
prefix_product = 1
for i in range(n):
answer[i] = prefix_product
prefix_product *= nums[i]
# Calculate suffix products and multiply with prefix products in answer
suffix_product = 1
for i in range(n - 1, -1, -1):
answer[i] *= suffix_product
suffix_product *= nums[i]
return answer
# Example usage
print(product_except_self([1, 2, 3, 4])) # Output: [24, 12, 8, 6]
print(product_except_self([-1, 1, 0, -3, 3])) # Output: [0, 0, 9, 0, 0]
Pros and Cons:
Pros: Optimized space usage.
Cons: Slightly more complex than the previous method.
Comparison of Approaches
Time Complexity Analysis: Both methods run in O(n) time, making them efficient for large input sizes.
Space Complexity Analysis:
Using Left and Right Product Arrays: O(n) extra space.
Using Prefix and Suffix Products with Single Array: O(1) extra space.
Situational Use Cases:
Left and Right Product Arrays: Useful for clear, step-by-step understanding.
Single Array Approach: Best for optimizing space.
Practical Applications
Understanding and implementing this problem has practical applications in various real-world scenarios:
Data Transformation: Efficiently computing transformed views of data without modifying the original dataset.
Financial Calculations: Calculating financial metrics that require excluding specific values.
Parallel Processing: Distributing tasks where certain computations need to exclude specific elements.
Moreover, mastering such problems enhances your problem-solving skills, making you better prepared for coding interviews and algorithm-intensive tasks.
Conclusion
The "Product of Array Except Self" problem is an excellent example of how thoughtful algorithm design can lead to efficient and elegant solutions. By exploring multiple approaches, we've seen how to tackle the problem from different angles, optimizing both time and space complexity.
Keep practicing these techniques to sharpen your skills and prepare for your next coding interview challenge!
Related Articles
Other Medium LeetCode Problems:
Subscribe to my newsletter
Read articles from Sean Coughlin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Sean Coughlin
Sean Coughlin
Software Engineer