How to Use Git from Shell for Your GitLab Coding Projects

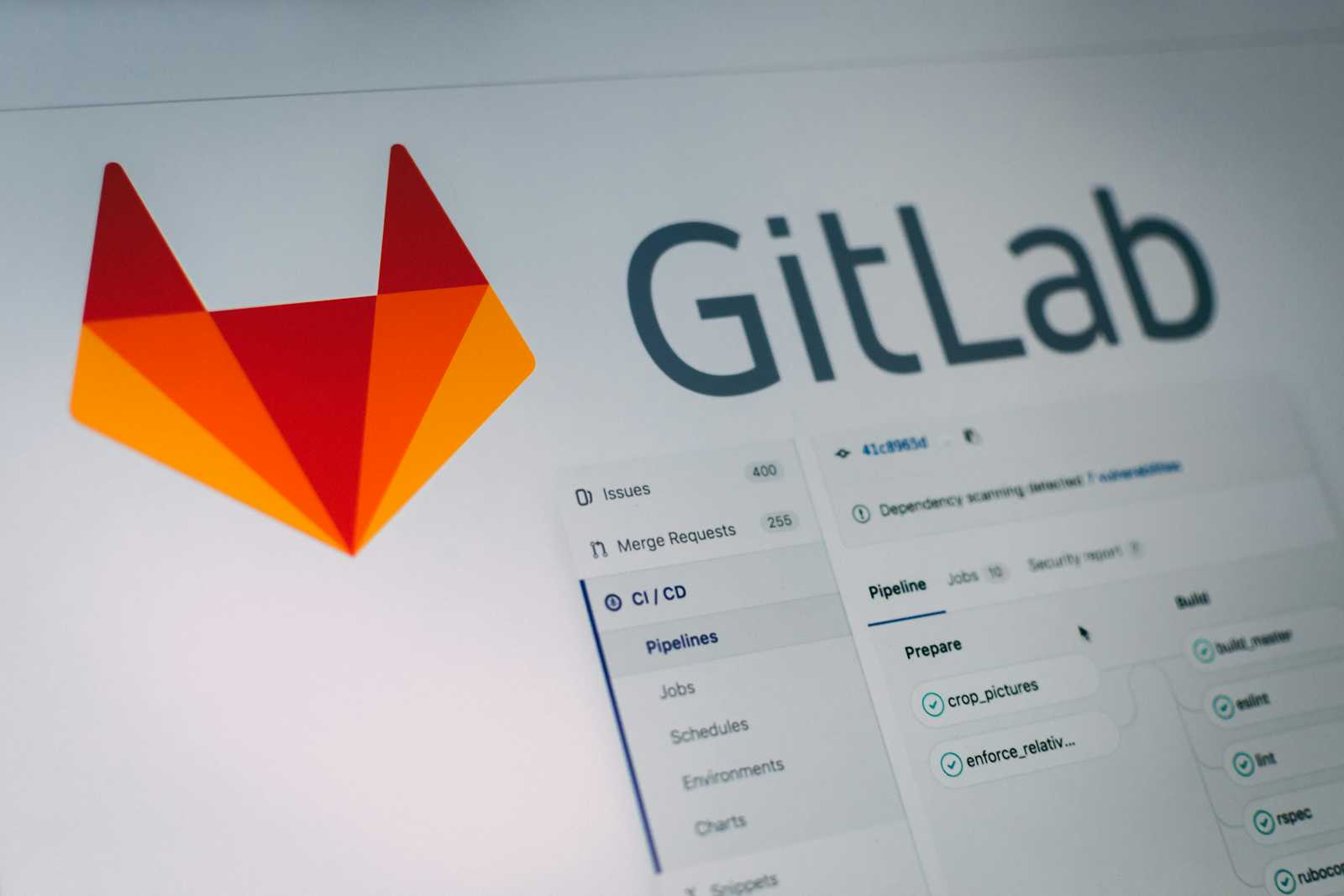
GitLab is a versatile platform for collaborative Git repositories, supporting open-source and private development projects. With robust features like issue tracking, wikis, and CI/CD pipelines, GitLab empowers teams to collaborate effectively and deliver exceptional software solutions.
In this advanced tutorial, we will guide you through creating a GitLab account, setting up a Node.js project using Express Generator, pushing your project to GitLab, and automating deployment using Git from the terminal along with GitLab's CI/CD pipelines. Each step includes practical examples and clear explanations to ensure comprehensive understanding and useful application.
Creating a GitLab Account
- Verify Your Email Address: Complete the registration on GitLab.com and activate your account by clicking the confirmation link sent to your email.
Setting up the Node.js Project
Install and Use Express Generator: Express Generator is a tool that simplifies the creation of Node.js applications using the Express framework. Install it globally to use it for creating a new project:
$ npm install -g express-generator
This command installs Express Generator globally on your system.
Create a New Node.js Project: Generate a new project named
my-node-app
using Express Generator. This command will create a structured project directory with essential files and directories for an Express application:$ express my-node-app
After running this command, you will see the following directory structure created for your project:
my-node-app/ ├── bin/ │ └── www ├── public/ │ ├── images/ │ ├── javascripts/ │ └── stylesheets/ │ └── style.css ├── routes/ │ ├── index.js │ └── users.js ├── views/ │ ├── error.jade │ ├── index.jade │ └── layout.jade ├── app.js └── package.json
Note: Depending on the version of Express Generator, the view files may use
.pug
instead of.jade
.Navigate to the Project Directory: Change into the newly created project directory:
$ cd my-node-app
Now, you're ready to start working on your Node.js project.
Initializing and Using Git
Initialize Git: Start version control for your project by initializing a new Git repository:
bashCopy code$ git init
This command initializes a new Git repository in your project directory, creating a
.git
folder to manage versioning.Add and Commit Changes: Add all files in the project directory to the Git staging area and commit them with a meaningful message:
bashCopy code$ git add . $ git commit -m "Initial commit"
This records the initial state of your project in Git.
Creating and Using a GitLab Repository
Create a GitLab Repository:
- Log in to GitLab.com, navigate to your dashboard, and click on the plus icon to create a new project (
demo-repository
). Choose the visibility level (public or private) and create the repository.
- Log in to GitLab.com, navigate to your dashboard, and click on the plus icon to create a new project (
Add GitLab Repository as Remote:
Link your local Git repository to the newly created GitLab repository by adding it as the remote origin:
$ git remote add origin https://gitlab.com/your-username/demo-repository.git
Replace
your-username
with your actual GitLab username.
Push Changes to GitLab:
Push your committed changes from the local repository to GitLab:
$ git push -u origin main
This command uploads your project files to GitLab under the
main
branch.Troubleshooting Tip: If you encounter authentication errors during the push, ensure your GitLab credentials are correctly configured. You might need to update your Git global configuration with your GitLab username and email:
bashCopy code$ git config --global user.name "Your Name" $ git config --global user.email "your.email@example.com"
Verify your configuration with:
$ git config --global --list
If necessary, update the remote URL for the origin:
$ git remote set-url origin https://gitlab.com/your-username/demo-repository.git
Advanced Topics: Implementing Continuous Integration and Deployment (CI/CD)
Configure CI/CD Pipeline:
Create a
.gitlab-ci.yml
file in the root directory of your project to define the CI/CD pipeline stages and jobs:stages: - build - test - deploy build: stage: build script: - npm install only: - main test: stage: test script: - npm test only: - main deploy: stage: deploy script: - echo "Deploying to production server" # Add deployment commands here only: - main
This configuration file sets up stages for building, testing, and deploying your application, ensuring automated workflows.
Troubleshooting Tip: If the CI/CD pipeline fails, review the pipeline logs on GitLab to identify errors in the build, test, or deployment stages. Common issues include missing dependencies, configuration errors, or access permissions.
Monitoring and Advanced GitLab Features
Monitor CI/CD Pipeline:
- Track the progress and status of your CI/CD pipeline on GitLab to ensure the successful execution of the build, test, and deployment stages.
Collaboration and Code Review:
- Leverage GitLab's merge requests, code reviews, and inline commenting features to collaborate effectively with team members, ensuring code quality and adherence to project standards.
Complete Node.js Project Code (app.js)
Here's the complete code for app.js
, the main entry point of your Node.js application created with Express Generator:
const express = require('express');
const path = require('path');
const cookieParser = require('cookie-parser');
const logger = require('morgan');
const indexRouter = require('./routes/index');
const usersRouter = require('./routes/users');
const app = express();
// view engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'jade');
app.use(logger('dev'));
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
app.use(cookieParser());
app.use(express.static(path.join(__dirname, 'public')));
app.use('/', indexRouter);
app.use('/users', usersRouter);
// catch 404 and forward to error handler
app.use(function(req, res, next) {
next(createError(404));
});
// error handler
app.use(function(err, req, res, next) {
// set locals, only providing error in development
res.locals.message = err.message;
res.locals.error = req.app.get('env') === 'development' ? err : {};
// render the error page
res.status(err.status || 500);
res.render('error');
});
module.exports = app;
This app.js
file sets up a basic Express application with middleware like morgan
for logging, cookie-parser
for parsing cookies, and express.static
for serving static files. It also includes routes defined in index.js
and users.js
.
Conclusion
Congratulations! You've successfully set up a GitLab account, created a Node.js project using Express Generator, integrated Git for version control, automated deployment with GitLab's CI/CD pipelines, and explored advanced GitLab features for collaboration and monitoring. Apply these practices to streamline your development workflows and confidently deliver high-quality software projects using GitLab.
Subscribe to my newsletter
Read articles from Niladri Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
