Deploy a Node.js Project on Vercel: Shell and Git Instructions

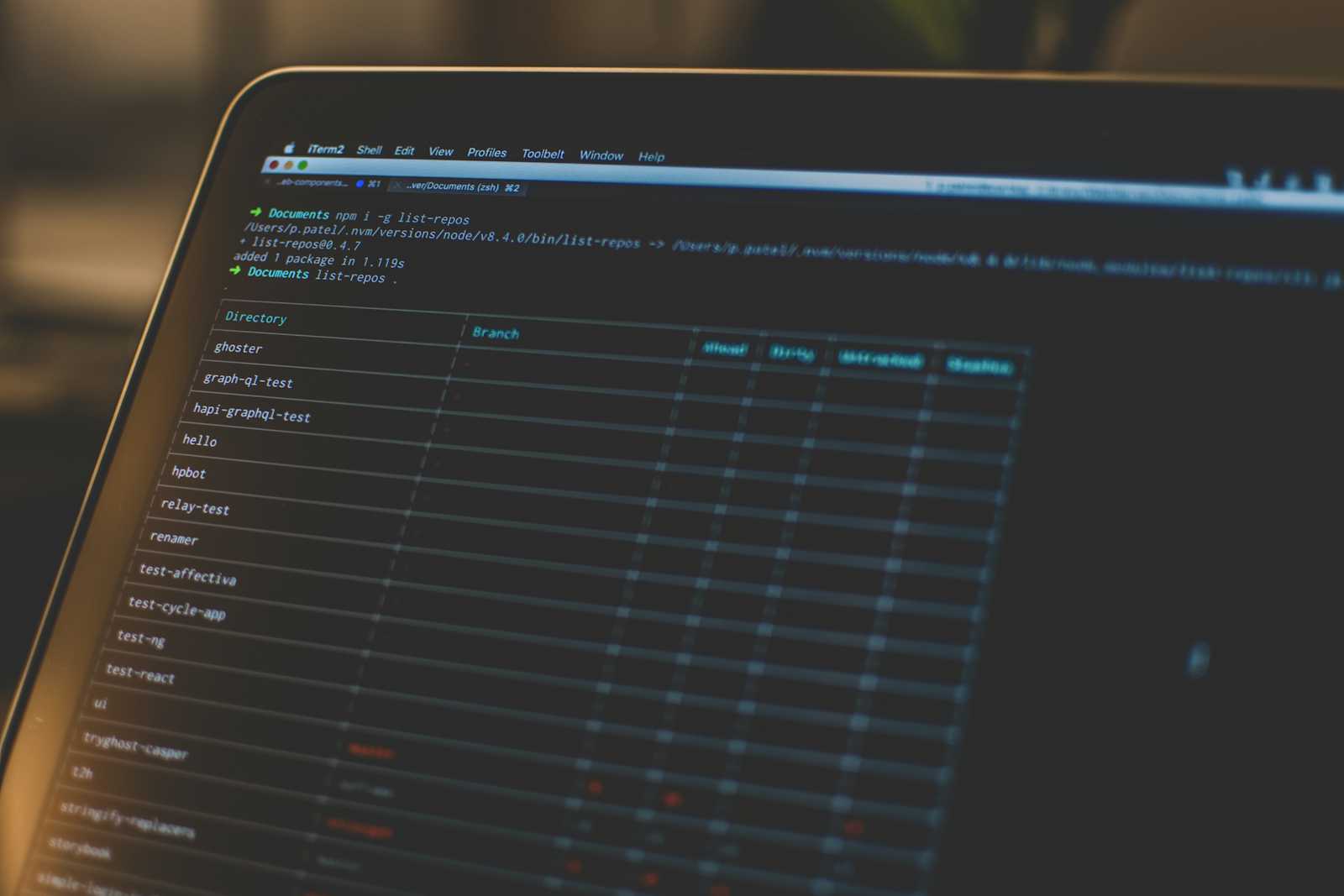
Vercel is a cloud platform that allows you to deploy and manage applications. In this tutorial, we will cover how to deploy a Node.js project to Vercel using Git from the terminal. We will also explore the benefits of using Vercel and how it can improve your development workflow.
Benefits of Using Vercel
Vercel is a popular choice for deploying applications because of its ease of use, scalability, and performance. Here are some benefits of using Vercel:
Easy Deployment: Vercel makes it easy to deploy your application with a single command. You don't need to worry about setting up servers or configuring infrastructure.
Scalability: Vercel automatically scales your application to handle traffic spikes, so you don't need to worry about your application crashing under heavy load.
Performance: Vercel uses a global content delivery network (CDN) to distribute your application, which means that your users will experience fast load times regardless of their location.
Security: Vercel provides automatic SSL encryption and supports HTTP/2, which means that your application is secure and fast.
Collaboration: Vercel makes it easy to collaborate with team members by providing a simple way to manage access and permissions.
Creating a New Project
In your terminal, create a new directory and initialize your project:
mkdir new-express-project
cd new-express-project
npm init -y
These commands create a new project directory and a package.json
file with default settings.
Installing Express.js
Add Express to your project:
npm install express
Setting up your Express.js App
Create a directory named /api
. Inside /api
, create a file named index.ts
. This will be your main server file.
Initializing an Express.js Server
Edit your index.ts
with the following code to set up a basic Express.js server:
const express = require("express");
const app = express();
app.get("/", (req, res) => res.send("Express on Vercel"));
app.listen(3000, () => console.log("Server ready on port 3000."));
module.exports = app;
This code will:
Define the base route
/
Return
Express on Vercel
when it’s calledAnd start the Express.js server running on port
3000
Configuring your Project for Vercel
By using a vercel.json
file, you can control how Vercel configures your application.
For our application, we’re going to tell Vercel to route all incoming requests to our /api
folder.
To configure this, create a vercel.json
file in the root of your project and add the following code:
{
"version": 2,
"rewrites": [{ "source": "/(.*)", "destination": "/api" }]
}
Running your Application Locally
You can replicate the Vercel deployed environment locally by using the Vercel CLI. This allows you to test how your application will run as if it were running on Vercel, even before you deploy.
To get started, you need to install the Vercel CLI by running the following command in your terminal:
npm install -g vercel
Next, login to Vercel to authorize the Vercel CLI to run commands on your Vercel account.
Now you use the local development command, which will also execute the vercel.json
configuration you created above.
Running vercel dev
will ask you some questions. You can answer however you like, but the defaults will suffice. This process will also create a new Vercel Project, but don’t worry, this will not make your Express.js app publicly accessible yet.
Deploying to Vercel
To deploy your application to Vercel, run the following command in your terminal:
vercel deploy
This command will deploy your application to Vercel.
Linking your Git Repository to Vercel
To link your Git repository to Vercel, run the following command in your terminal:
vercel link
This command will link your Git repository to Vercel.
Setting up Automatic Deployments
To set up automatic deployments, you need to configure your Git repository to deploy to Vercel on each push.
To do this, run the following command in your terminal:
vercel deploy --prod
This command will set up automatic deployments to Vercel on each push to your Git repository.
Benefits of Automatic Deployments
Automatic deployments provide several benefits:
Efficiency: Automatic deployments save time and effort by eliminating the need to manually deploy your application.
Consistency: Automatic deployments ensure that your application is deployed consistently every time.
Quality: Automatic deployments reduce the risk of errors and improve the quality of your application.
Collaboration: Automatic deployments make it easy to collaborate with team members by providing a simple way to manage access and permissions.
Conclusion
In this tutorial, we covered how to deploy a Node.js project to Vercel using Git from the terminal. We also explored the benefits of using Vercel and how it can improve your development workflow.
Vercel is a powerful cloud platform that makes it easy to deploy and manage applications. By using Vercel, you can focus on writing code and let Vercel handle the infrastructure.
If you're looking for a simple and efficient way to deploy your Node.js project, Vercel is an excellent choice. With its ease of use, scalability, and performance, Vercel is a popular choice for developers and teams of all sizes.
So what are you waiting for? Give Vercel a try and see how it can improve your development workflow.
Here are the commands used in this tutorial:
mkdir new-express-project
cd new-express-project
npm init -y
npm install express
mkdir api
cd api
touch index.ts
touch vercel.json
vercel dev
vercel deploy
vercel link
vercel deploy --prod
Frequently Asked Questions
Q: What is Vercel?
A: Vercel is a cloud platform that allows you to deploy and manage applications.
Q: How do I deploy a Node.js project to Vercel using Git from the terminal?
A: You can deploy a Node.js project to Vercel using Git from the terminal by following the steps outlined in this tutorial.
Q: What are the benefits of using Vercel?
A: Vercel provides several benefits, including easy deployment, scalability, performance, security, and collaboration.
Q: What is automatic deployment?
A: Automatic deployment is a feature that allows you to configure your Git repository to deploy to Vercel on each push.
Q: Why should I use automatic deployment?
A: Automatic deployment provides several benefits, including efficiency, consistency, quality, and collaboration.
Q: How do I set up automatic deployments?
A: You can set up automatic deployments by running the vercel deploy --prod
command in your terminal.
Q: How do I link my Git repository to Vercel?
A: You can link your Git repository to Vercel by running the vercel link
command in your terminal.
Q: How do I replicate the Vercel deployed environment locally?
A: You can replicate the Vercel deployed environment locally by using the Vercel CLI.
Q: How do I configure my project for Vercel?
A: You can configure your project for Vercel by using a vercel.json
file.
Q: How do I initialize an Express.js server?
A: You can initialize an Express.js server by creating a file named index.ts
in the /api
directory and adding the code outlined in this tutorial.
Q: How do I install Express.js?
A: You can install Express.js by running the npm install express
command in your terminal.
Q: How do I create a new project?
A: You can create a new project by running the mkdir new-express-project
and cd new-express-project
commands in your terminal, followed by the npm init -y
command.
Q: What is a content delivery network (CDN)?
A: A content delivery network (CDN) is a distributed network of servers that delivers content to users based on their geographic location.
Q: What is HTTP/2?
A: HTTP/2 is a major revision of the HTTP network protocol used by the World Wide Web.
Q: What is SSL encryption?
A: SSL encryption is a security protocol that encrypts data transmitted over the internet.
Q: What is a global content delivery network (CDN)?
A: A global content delivery network (CDN) is a distributed network of servers that delivers content to users based on their geographic location, regardless of their location.
Q: What is a single command deployment?
A: A single command deployment is a deployment process that requires only one command to deploy your application.
Q: What is a production-ready version of my project?
A: Aproduction-ready version of your project is a version that is ready to be deployed to a production environment.
Q: What is a development environment?
A: A development environment is a environment where you develop and test your application.
Q: What is a production environment?
A: A production environment is a environment where your application is deployed and accessible to users.
Q: What is a staging environment?
A: A staging environment is a environment where you test and validate your application before deploying it to a production environment.
Q: What is a testing environment?
A: A testing environment is a environment where you test and validate your application.
Q: What is a continuous integration and continuous deployment (CI/CD) pipeline?
A: A continuous integration and continuous deployment (CI/CD) pipeline is a automated process that builds, tests, and deploys your application.
Q: What is a Git repository?
A: A Git repository is a version control system that allows you to track changes to your code.
Q: What is a Vercel project?
A: A Vercel project is a project that is deployed to Vercel.
Q: What is a Vercel account?
A: A Vercel account is an account that allows you to access Vercel services.
Q: How do I login to Vercel?
A: You can login to Vercel by running the vercel login
command in your terminal.
Q: How do I create a new Vercel project?
A: You can create a new Vercel project by running the vercel create
command in your terminal.
Q: How do I deploy a Vercel project?
A: You can deploy a Vercel project by running the vercel deploy
command in your terminal.
Q: How do I link a Vercel project to a Git repository?
A: You can link a Vercel project to a Git repository by running the vercel link
command in your terminal.
Q: How do I set up automatic deployments for a Vercel project?
A: You can set up automatic deployments for a Vercel project by running the vercel deploy --prod
command in your terminal.
Q: How do I replicate the Vercel deployed environment locally for a Vercel project?
A: You can replicate the Vercel deployed environment locally for a Vercel project by using the Vercel CLI.
Q: How do I configure a Vercel project for Vercel?
A: You can configure a Vercel project for Vercel by using a vercel.json
file.
Q: How do I initialize an Express.js server for a Vercel project?
A: You can initialize an Express.js server for a Vercel project by creating a file named index.ts
in the /api
directory and adding the code outlined in this tutorial.
Q: How do I install Express.js for a Vercel project?
A: You can install Express.js for a Vercel project by running the npm install express
command in your terminal.
Q: How do I create a new Vercel project with Express.js?
A: You can create a new Vercel project with Express.js by running the mkdir new-express-project
and cd new-express-project
commands in your terminal, followed by the npm init -y
and npm install express
commands.
I hope this tutorial has been helpful in deploying your Node.js project to Vercel using Git from the terminal. If you have any questions or need further assistance, please don't hesitate to ask.
Subscribe to my newsletter
Read articles from Niladri Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
