A Road Trip to Understanding JavaScript SDK

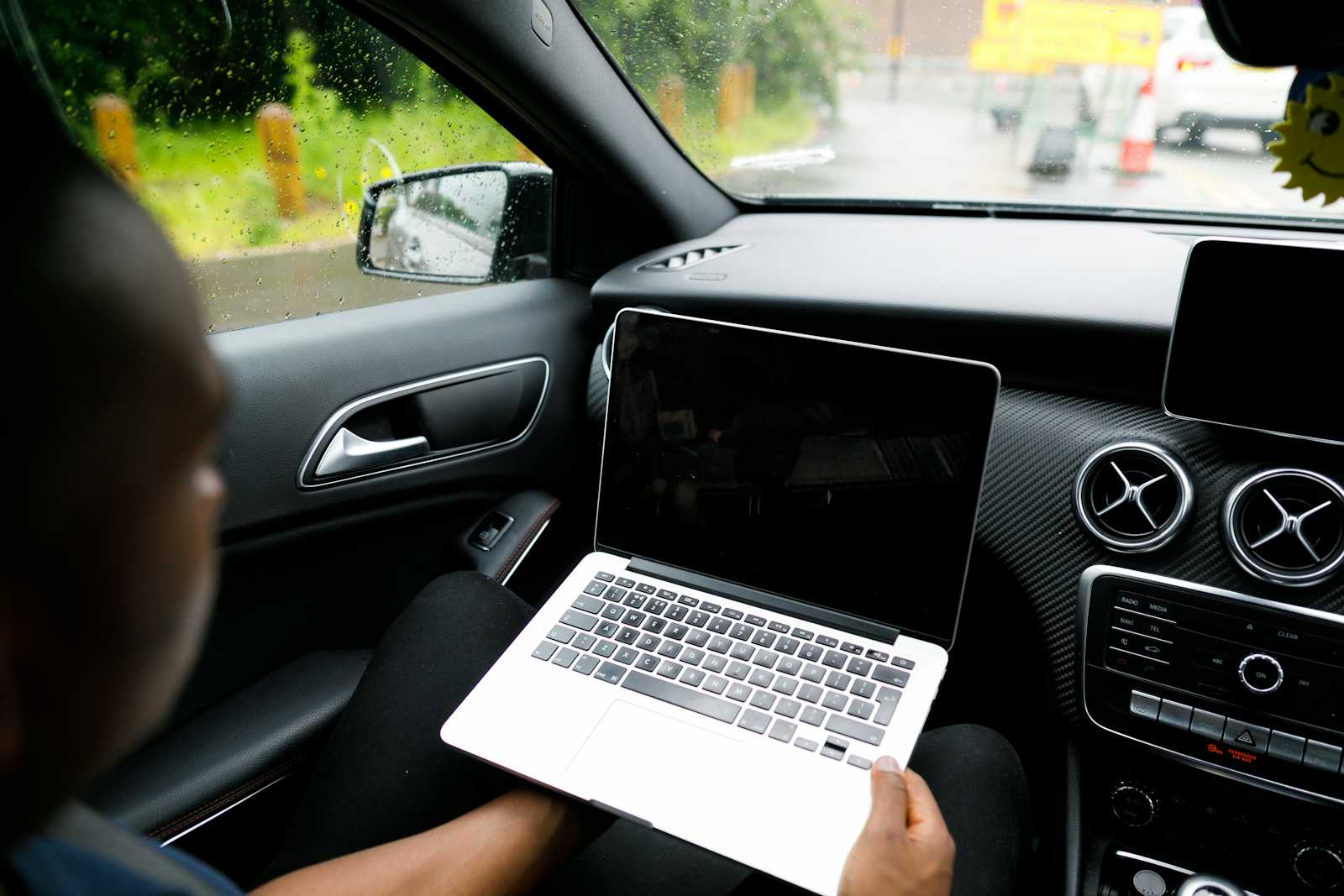
Yo man! New to tech, right? Not to worry, I got you. We have all been there. Just have the beginner's curiosity, and you are good to go. Let's take a road trip to some parts of JavaScript.
What is JavaScript?
Just like a house cannot stand and be called a house without proper planning and architecture, a website or web application cannot be developed without the right tools and languages.
We can't talk about JavaScript without discussing the foundations that make JavaScript valuable. Building a website consists of two important structures:
HTML - Interpreted as Hypertext Markup Language, HTML is the root file or skeletal part of the website. Just like building a house, you lay the foundation and erect it with blocks. Similarly, when developing a website, HTML elements represent the blocks used to build its structure.
CSS - Known as Cascading Style Sheets, CSS is the styling, beautifying, or aesthetics of the website. Just like you can't build a house without plastering, painting, and fixing windows and doors to make it homely, you can't build a website using just HTML without making it presentable for its users.
Just like a house built with blocks and beautified with nice aesthetics, the user of the house needs to have the ability to open and close the doors and windows, run the taps for water to flow, and switch on and off the lighting system for regulation. Although our website has been built, beautified, and is ready for use, it is static. We need our users to enjoy interacting with our website, and to do this, we need the help of JavaScript.
JavaScript is referred to as a scripting or programming language that allows you to implement simple or complex features on a static web page. The ability for a user to display timely content updates, interactive maps, animated 2D/3D graphics, and scrolling video jukeboxes is a result of JavaScript.
What is JavaScript SDK?
To understand what a JavaScript SDK is, let's take a look at this scenario:
Mrs. Hammit is a young mother of two boys who tries her best to juggle her career and being a good mother. She barely has time for herself as she tries to please her boss and take good care of her home. From Monday to Saturday, she has tons of targets to meet at work and most times brings the leftover work home. On one of those busy days, she overheard her two boys talking about how they would love to eat a home-cooked chicken soup made by their mom.
In order to satisfy the desires of her boys, she decided to make the chicken soup for them. She barely has time for herself, so instead of buying a live chicken and going through the process of butchering, defeathering, and cutting the chicken, she chose to stop by a store not too far from her workplace to get the service of an already butchered, defeathered, and cut chicken. She got home and used the processed chicken to prepare the chicken soup her boys craved, and they were very happy.
Just like the store Mrs. Hammit visited to get the already processed chicken, a JavaScript SDK is like a library offered by a company for easier integration of their API.
What is an SDK?
SDKs, which is the short form of Software Development Kits, consist of inbuilt functions, methods, documentation, and components that help beginners, intermediate, and senior engineers alike throughout the software development process. An SDK can be visualized as a toolbox containing all the things a developer needs when they run a program. This includes external plugins, packages, testing dependencies, debuggers, compilers, and many more.
A JavaScript SDK is a set of libraries and tools that simplifies the process of building applications using JavaScript.
How does a JavaScript SDK work?
Include it in your project by installing it via npm (node.js) or in your HTML file.
Initialize the SDK by using the necessary configuration options.
Use the API in your project by calling it and customizing it to your desired outcome.
Building a Demo with JavaScript SDK
To give you a practical understanding, let's look at an example of using the Cloudinary JavaScript SDK.
Step 1: Install SDK
If you're using npm, you can install the Cloudinary SDK by running:
npm install @cloudinary/widget
Step 2: Initialize the SDK
In your JavaScript file, initialize Cloudinary upload widget with your personalized configuration settings:
import { createUploadWidget } from '@cloudinary/widget';
// Configure the Cloudinary Upload Widget
const cloudName = 'YOUR_CLOUD_NAME'; // Replace with your Cloudinary cloud name
const uploadPreset = 'YOUR_UPLOAD_PRESET'; // Replace with your Cloudinary upload preset
const myWidget = createUploadWidget(
{
cloudName: cloudName,
uploadPreset: uploadPreset
},
(error, result) => {
if (!error && result && result.event === "success") {
console.log("Uploaded image info: ", result.info);
document.getElementById("image").src = result.info.secure_url;
}
}
);
Step 3: Use the API
Now you can use Cloudinary services in your project. For example, add a button to open the widget and an image element to display the uploaded image. Then, add an event listener to the button to open the widget when clicked.
<!DOCTYPE html>
<html>
<head>
<title>Cloudinary Upload Widget Demo</title>
</head>
<body>
<!-- Button to trigger the upload widget -->
<button id="upload_widget_opener">Upload Image</button>
<!-- Image element to display the uploaded image -->
<img id="image" src="" alt="Uploaded Image" />
<script type="module">
import { createUploadWidget } from '@cloudinary/widget';
// Configure the Cloudinary Upload Widget
const cloudName = 'YOUR_CLOUD_NAME'; // Replace with your Cloudinary cloud name
const uploadPreset = 'YOUR_UPLOAD_PRESET'; // Replace with your Cloudinary upload preset
const myWidget = createUploadWidget(
{
cloudName: cloudName,
uploadPreset: uploadPreset
},
(error, result) => {
if (!error && result && result.event === "success") {
console.log("Uploaded image info: ", result.info);
document.getElementById("image").src = result.info.secure_url;
}
}
);
// Add event listener to the upload button to open the widget
document.getElementById("upload_widget_opener").addEventListener("click", () => {
myWidget.open();
}, false);
</script>
</body>
</html>
Other Popular JavaScript SDKs
Here are a few popular JavaScript SDKs that are widely used in web development:
Firebase SDK: Provides tools for real-time databases, authentication, and cloud functions.
Stripe SDK: Simplifies integrating payment processing into your website or app.
Cloudinary SDK: Offers tools for image and video management, including uploads, transformations, and optimizations.
Leveraging these SDKs, developers can save time and effort, focusing more on building features rather than dealing with low-level implementation details.
Subscribe to my newsletter
Read articles from Toluwanimi Esther directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Toluwanimi Esther
Toluwanimi Esther
Toluwanimi is a TECH VALUE provider. She is available to put her own quota into providing quality value into lives, businesses and the economy globally using Technology.