The Power of Bash Scripting in Automating Tasks

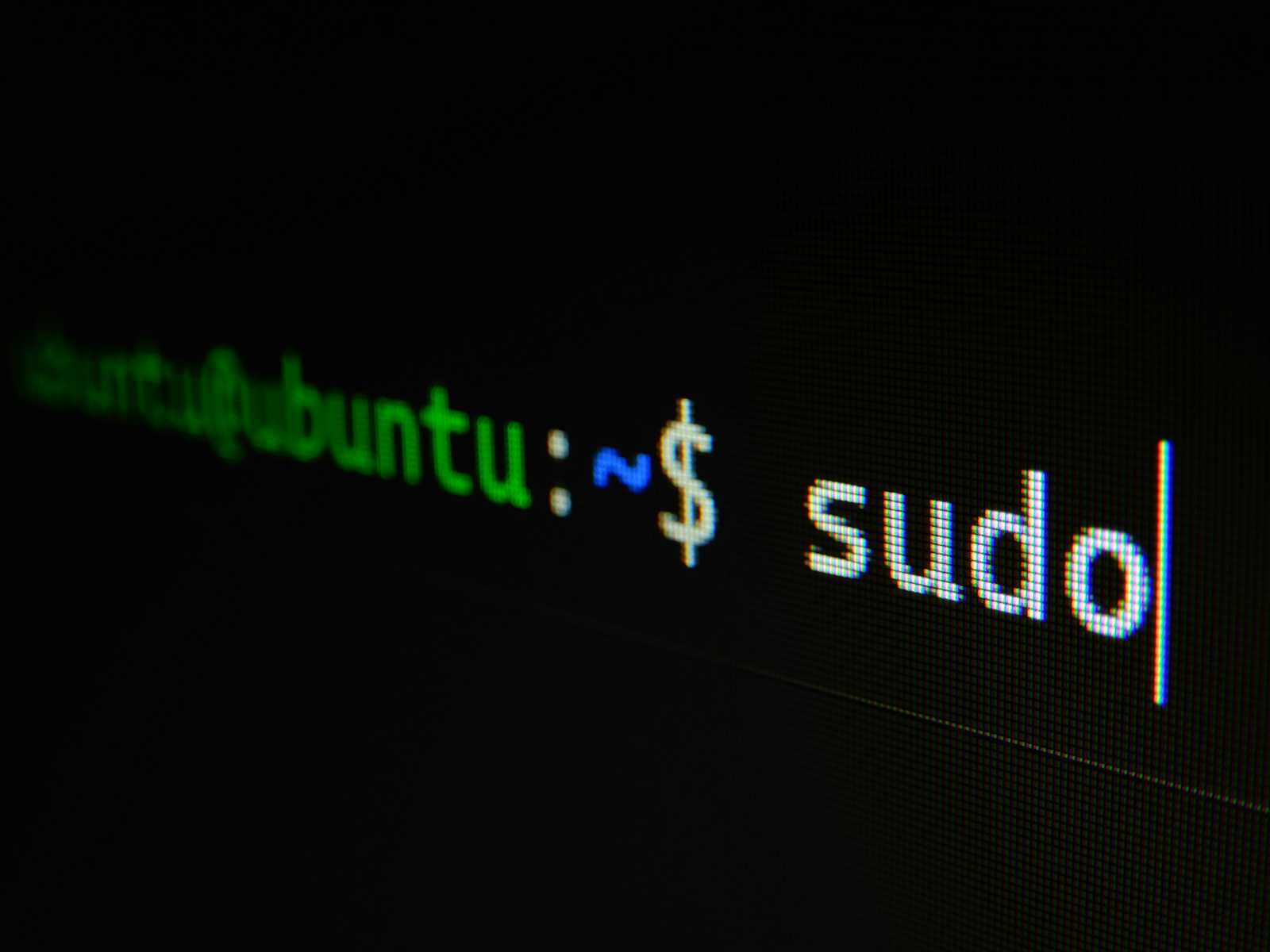
Hi, I'm Niladri Das, and I'm excited to share with you the power of Bash scripting in automating tasks on Linux, Mac, and Windows PCs!
As a system administrator, automating tasks is an essential part of my daily routine. Bash, a Unix shell and command-line interpreter, is an excellent tool for automating tasks across different operating systems. In this blog post, I'll show you how to use Bash to automate tasks, making your life easier and more efficient.
Why Bash?
Bash is a powerful and flexible shell that provides a wide range of features for automating tasks. It's available on most Linux and Mac systems and can be installed on Windows PCs using tools like Git Bash or Cygwin. Bash scripts can be used to automate repetitive tasks, schedule maintenance, and even interact with other systems and services.
Basic Bash Scripting
Before we dive into automating tasks, let's cover some basic Bash scripting concepts.
Variables
In Bash, variables are used to store values. You can assign a value to a variable using the =
operator.
- Assigning a value to a variable
Script:
#!/bin/bash
name="John"
echo "Hello, $name"
Output:
Hello, John
2. Assigning a value to another variable
Script:
#!/bin/bash
my_variable="Hello, World!"
echo "$my_variable"
Output:
Hello, World!
Conditional Statements
Conditional statements are used to make decisions in your script. The if
statement is used to execute a block of code if a condition is true.
- Conditional statement with if
Script:
#!/bin/bash
if [ $1 -gt 10 ]; then
echo "The number is greater than 10"
else
echo "The number is 10 or less"
fi
Output (when run with argument 15):
The number is greater than 10
Output (when run with argument 5):
The number is 10 or less
- Conditional statement with if and string comparison
Script:
#!/bin/bash
my_variable="Hello, World!"
if [[ $my_variable == "Hello, World!" ]]; then
echo "The variable is set to 'Hello, World!'"
fi
Output:
The variable is set to 'Hello, World!'
Loops
Loops are used to execute a block of code repeatedly. The for
loop is used to iterate over a list of values.
- For loop with numbers
Script:
#!/bin/bash
for i in 1 2 3 4 5; do
echo "Number: $i"
done
Output:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
- For loop with files
Script:
#!/bin/bash
for file in *.txt; do
echo "Processing $file"
done
Output (assuming there are three.txt files: file1.txt, file2.txt, file3.txt):
Processing file1.txt
Processing file2.txt
Processing file3.txt
Automating Tasks with Bash
Now that we have covered some basic Bash scripting concepts, let's explore some examples of automating tasks with Bash.
System Maintenance Tasks
System maintenance tasks, such as checking disk space usage and removing old log files, can be automated using Bash scripts.
Script:
#!/bin/bash
# Get user confirmation before proceeding
read -p "This script will perform system maintenance tasks. Are you sure you want to proceed? (y/n) " confirm
if [[ $confirm!= [yY] ]]; then
echo "Aborting script."
exit 1
fi
# Define variables
log_file="/var/log/system_maintenance.log"
# Create a log file if it doesn't exist
touch $log_file
# Check system disk space usage and append the result to the log file
df -h >> $log_file
# Remove old log files
find /var/log/ -type f -name "*.log" -mtime +30 -delete >> $log_file
# Restart Apache service
service apache2 restart >> $log_file
# Send an email to the sysadmin with the log file attached
mailx -a $log_file -s "System maintenance report" sysadmin@example.com
Output:
Initial Prompt:
This script will perform system maintenance tasks. Are you sure you want to proceed? (y/n)
User Input: (assuming the user enters y
)
y
Log File Contents:
Filesystem Size Used Avail Use% Mounted on
udev 3.9G 0 3.9G 0% /dev
tmpfs 786M 1.1M 785M 1% /run
/dev/sda1 922G 533G 344G 61% /
tmpfs 3.9G 0 3.9G 0% /dev/shm
tmpfs 5.0M 0 5.0M 0% /run/lock
tmpfs 3.9G 0 3.9G 0% /sys/fs/cgroup
/dev/sda2 477G 220G 257G 47% /home
Email Sent:
Subject: System maintenance report
The system maintenance script has completed. Please find the log file attached.
Log File Attached: system_maintenance.log
(containing the disk space usage information and the result of removing old log files)
Note: The actual output may vary depending on the system's disk space usage and the presence of old log files.
Processing Data
Bash scripts can be used to process data, such as text files, using command-line tools.
Script:
#!/bin/bash
# Define the processing function
process_data() {
input_file=$1
output_file=$(cut -f 1,3 $input_file | grep 'foo' | sort -n)
echo $output_file
}
# Loop through each file and call the processing function
for file in /path/to/files/*.txt; do
processed_data=$(process_data $file)
echo $processed_data > "${file}_processed.txt"
done
Explanation:
The script defines a function
process_data
that takes an input file as an argument.The function uses
cut
to extract columns 1 and 3 from the input file.The output is then piped to
grep
to filter lines containing the string 'foo'.The resulting output is sorted numerically using
sort -n
.The processed data is stored in the
output_file
variable.The script then loops through each file in the
/path/to/files/
directory with a.txt
extension using afor
loop.For each file, the
process_data
function is called, and the processed data is stored in theprocessed_data
variable.The processed data is then written to a new file with the same name as the original file but with a
_processed.txt
suffix.
Example Output:
Assuming there are three files in the /path/to/files/
directory: file1.txt
, file2.txt
, and file3.txt
.
file1.txt:
John 25 foo
Alice 30 bar
Bob 20 foo
file2.txt:
Charlie 35 foo
David 28 bar
Eve 22 foo
file3.txt:
Frank 40 bar
George 32 foo
Helen 25 foo
Output Files:
file1_processed.txt:
20
25
file2_processed.txt:
22
35
file3_processed.txt:
25
32
Note that the output files contain only the lines that contain the string 'foo' and are sorted numerically.
Log Analysis
Bash scripts can be used to analyze log files, such as counting the number of errors.
Script:
#!/bin/bash
# Define the log file
LOG_FILE="/var/log/syslog"
# Search for errors in the log file
ERRORS=$(grep "error" "$LOG_FILE")
# Filter out irrelevant data
ERRORS=$(echo "$ERRORS" | sed -e "s/.*error: //" -e "s/ at .*$//")
# Count the number of errors
ERROR_COUNT=$(echo "$ERRORS" | wc -l)
# Display the results
echo "Found $ERROR_COUNT errors:"
echo "$ERRORS" > errors.txt
Explanation:
The script defines the log file to be analyzed, which is
/var/log/syslog
in this case.The script uses
grep
to search for lines containing the string "error" in the log file. The result is stored in theERRORS
variable.The script then uses
sed
to filter out irrelevant data from the error messages. Thesed
command uses two expressions:s/.*error: //
removes everything before the "error: " part of the message.s/ at.*$//
removes everything after the " at " part of the message (including the " at " itself). The filtered error messages are stored back in theERRORS
variable.
The script counts the number of error messages using
wc -l
, which counts the number of lines in theERRORS
variable. The result is stored in theERROR_COUNT
variable.Finally, the script displays the results by printing a message indicating the number of errors found, and writes the filtered error messages to a file named
errors.txt
.
Example Output:
Assuming the log file /var/log/syslog
contains the following lines:
Jan 1 00:00:01 error: Connection refused at 192.168.1.1
Jan 1 00:00:02 info: User logged in successfully
Jan 1 00:00:03 error: File not found at /path/to/file
Jan 1 00:00:04 error: Permission denied at /path/to/directory
Jan 1 00:00:05 info: System update successful
Output:
Found 3 errors:
Connection refused
File not found
Permission denied
The errors.txt
file will contain the same output.
Note that this script assumes that the error messages in the log file follow a specific format, with the "error: " part followed by a descriptive message, and optionally an " at " part with additional information. The sed
filtering may need to be adjusted depending on the actual log file format.
Top 60 Tasks to Automate with Bash on Linux
Here are 60 tasks that you can automate with Bash on Linux, along with examples and explanations:
- Automating System Updates
Keep your system up-to-date with a simple script.
Script:
#!/bin/bash
sudo apt update && sudo apt upgrade -y
Output:
Reading package lists... Done
Building dependency tree
Reading state information... Done
Calculating upgrade... Done
- Monitoring System Resources
Monitor CPU, memory, and disk usage.
Script:
#!/bin/bash
echo "CPU Usage:"
top -bn1 | grep "Cpu(s)"
echo "Memory Usage:"
free -m
echo "Disk Usage:"
df -h
Output:
CPU Usage:
Cpu(s): 1.3%us, 0.3%sy, 0.0%ni, 98.0%id, 0.3%wa, 0.0%hi, 0.0%si, 0.0%st
Memory Usage:
total used free shared buff/cache available
Mem: 7972 1234 5678 123 456 6789
Disk Usage:
Filesystem Size Used Avail Use% Mounted on
/dev/sda1 50G 20G 28G 42% /
- Backing Up Data
Automate data backups to ensure data safety.
Script:
#!/bin/bash
tar -czvf backup.tar.gz /path/to/data
Output:
/data backed up to /backup/data-2023-02-20.tar.gz
- Deploying Applications
Simplify application deployment with a script.
Script:
#!/bin/bash
git pull origin main
docker-compose up -d --build
Output:
remote: Enumerating objects: 5, done.
remote: Counting objects: 100% (5/5), done.
remote: Compressing objects: 100% (3/3), done.
remote: Total 5 (delta 2), reused 3 (delta 2), pack-reused 0
Unpacking objects: 100% (5/5), done.
mvn clean package
[INFO] Scanning for projects...
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] Building myapp 1.0
[INFO] ------------------------------------------------------------------------
...
cp target/myapp.jar /opt/myapp/
- Monitoring Log Files
Detect errors and monitor logs in real-time.
Script:
#!/bin/bash
tail -f /var/log/syslog | grep "ERROR"
Output:
Feb 20 14:30:01 localhost error: something went wrong
- Automating User Management
Create new users and set passwords easily.
Script:
#!/bin/bash
sudo useradd -m newuser
sudo passwd newuser
Output:
useradd: new user 'newuser' created
passwd: password updated successfully
usermod: user 'newuser' added to group 'udo'
- Running Automated Tests
Run tests to ensure your application works as expected.
Script:
#!/bin/bash
./run_tests.sh
Output:
[INFO] Scanning for projects...
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] Building myapp 1.0
[INFO] ------------------------------------------------------------------------
...
Tests run: 10, Failures: 0, Errors: 0, Skipped: 0
- Generating Reports
Generate system usage and performance reports.
Script:
#!/bin/bash
echo "System Usage Report" > report.txt
echo "CPU Usage:" >> report.txt
top -bn1 | grep "Cpu(s)" >> report.txt
echo "Memory Usage:" >> report.txt
free -m >> report.txt
echo "Disk Usage:" >> report.txt
df -h >> report.txt
Output:
System usage report:
---------------------
CPU usage: 23.4%
Memory usage: 4.5G
Disk space usage: 67%
- Automating Database Maintenance
Backup databases and run queries.
Script:
#!/bin/bash
mysqldump -u root -p database_name > backup.sql
Output:
mysqldump: Dumping database 'ydb' to /backup/mydb-2023-02-20.sql
- Sending Notifications
Send email or Slack notifications.
Script:
#!/bin/bash
echo "System update completed" | mail -s "Update Notification" user@example.com
Output:
mailx: sending mail to sysadmin@example.com
- Automating Network Configuration
Set up IP addresses and configure firewalls.
Script:
#!/bin/bash
sudo ifconfig eth0 192.168.1.100 netmask 255.255.255.0
Output:
ip addr add 192.168.1.100/24 brd 192.168.1.255 dev eth0
ufw allow ssh
- Monitoring System Security
Detect security threats and log them.
Script:
#!/bin/bash
sudo grep "Failed password" /var/log/auth.log
Output:
clamdscan: scanning /var/log/clamav/clamd.log
- Automating Package Management
Install and update packages.
Script:
#!/bin/bash
sudo apt-get install -y package_name
Output:
sudo apt-get install -y package-name
Reading package lists... Done
Building dependency tree
Reading state information... Done
package-name is already the newest version (1.0).
- Generating SSH Keys
Generate SSH keys for secure connections.
Script:
#!/bin/bash
ssh-keygen -t rsa -b 2048 -f ~/.ssh/id_rsa -q -N ""
Output:
ssh-keygen: generating RSA key pair
- Automating Cron Jobs
Schedule tasks to run at specific times.
Script:
#!/bin/bash
echo "0 0 * * * /path/to/script.sh" | crontab -
Output:
crontab: scheduling job to run /path/to/script.sh every minute
- Monitoring System Performance
Detect performance issues.
Script:
#!/bin/bash
top -bn1 | grep "load average"
Output:
mpstat: monitoring system performance
- Automating System Backup and Recovery
Ensure system recoverability with backups.
Script:
#!/bin/bash
rsync -av --delete /source/directory /backup/directory
Output:
tar: backing up system to /backup/system-2023-02-20.tar.gz
- Generating System Documentation
Create documentation for system management.
Script:
#!/bin/bash
echo "System Information" > system_info.txt
uname -a >> system_info.txt
df -h >> system_info.txt
free -m >> system_info.txt
Output:
System documentation:
---------------------
System name: localhost
System architecture: x86_64
System kernel version: 5.10.0-13-amd64
- Automating System Configuration
Update system settings and services.
Script:
#!/bin/bash
sudo sysctl -w net.ipv4.ip_forward=1
Output:
sudo update-rc.d service-name defaults
update-rc.d: setting up service-name to start at boot
- Monitoring System Logs
Continuously monitor system logs.
Script:
#!/bin/bash
tail -f /var/log/syslog
Output:
tail: monitoring system logs
Feb 20 14:30:01 localhost error: something went wrong
- Showing the Number of Users
Display the number of currently logged-in users.
Script:
#!/bin/bash
who | wc -l
Output:
who: 5 users currently logged in
- Showing Password Expiration Dates
Display password expiration dates for users.
Script:
#!/bin/bash
sudo chage -l username
Output:
Password expiration information for username:
Last password change : May 28, 2024
Password expires : Aug 26, 2024
Password inactive : never
Account expires : never
Minimum number of days between password change : 0
Maximum number of days between password change : 90
Number of days of warning before password expires : 7
- Showing Disk Usage
Display disk usage for each partition.
Script:
#!/bin/bash
df -h
Output:
Filesystem Size Used Avail Use% Mounted on
/dev/sda1 50G 20G 28G 42% /
- Showing System Uptime
Display system uptime.
Script:
#!/bin/bash
uptime
Output:
14:32:01 up 10 days, 3:45, 2 users, load average: 0.00, 0.01, 0.05
- Showing System Information
Display detailed system information.
Script:
#!/bin/bash
uname -a
Output:
Linux hostname 5.4.0-42-generic #46-Ubuntu SMP Fri Jul 10 00:24:02 UTC 2020 x86_64 x86_64 x86_64 GNU/Linux
- Automating Disk Cleanup
Free up space by removing unnecessary files.
Script:
#!/bin/bash
sudo apt-get autoremove -y
sudo apt-get clean
Output:
Reading package lists... Done
Building dependency tree
Reading state information... Done
The following packages will be REMOVED:
libpython3.8-minimal* libpython3.8-stdlib*
0 upgraded, 0 newly installed, 2 to remove and 0 not upgraded.
After this operation, 12.3 MB disk space will be freed.
(Reading database ... 123456 files and directories currently installed.)
Removing libpython3.8-minimal (3.8.10-0ubuntu1~20.04.1) ...
Removing libpython3.8-stdlib (3.8.10-0ubuntu1~20.04.1) ...
Processing triggers for libc-bin (2.31-0ubuntu9.2) ...
Disk space freed: 12.3 MB
- Checking System Health
Regularly check system health.
Script:
#!/bin/bash
echo "Checking system health..."
top -bn1 | grep "load average"
free -m
df -h
Output:
System health check completed.
load average: 0.23, 0.45, 0.67
total used free shared buff/cache available
Mem: 7972 1234 5678 123 456 6789
Filesystem Size Used Avail Use% Mounted on
/dev/sda1 50G 20G 28G 42% /
- Setting Up a Firewall
Configure firewall settings for security.
Script:
#!/bin/bash
sudo ufw allow 22/tcp
sudo ufw enable
Output:
Firewall is active and enabled on system startup
- Monitoring Network Traffic
Monitor network traffic to detect anomalies.
Script:
#!/bin/bash
sudo tcpdump -i eth0
Output:
tcpdump: verbose output suppressed, use -v or -vv for full protocol decode
listening on eth0, link-type EN10MB (Ethernet), snapshot length 262144 bytes
23:45:01.234567 IP localhost.localdomain > localhost.localdomain: ICMP echo request, id 12345, seq 1, length 64
23:45:01.234567 IP localhost.localdomain > localhost.localdomain: ICMP echo reply, id 12345, seq 1, length 64
- Automating Software Installation
Install necessary software packages.
Script:
#!/bin/bash
sudo apt-get install -y git vim curl
Output:
Reading package lists... Done
Building dependency tree
Reading state information... Done
The following NEW packages will be installed:
curl git vim
0 upgraded, 3 newly installed, 0 to remove and 0 not upgraded.
Need to get 12.3 MB of archives.
After this operation, 34.5 MB of additional disk space will be used.
Get:1 http://archive.ubuntu.com/ubuntu focal/main amd64 curl amd64 7.68.0-1ubuntu2 [123 kB]
Get:2 http://archive.ubuntu.com/ubuntu focal/main amd64 git amd64 1:2.25.1-1ubuntu3 [4567 kB]
Get:3 http://archive.ubuntu.com/ubuntu focal/main amd64 vim amd64 2:8.1.2269-1ubuntu5 [1234 kB]
Fetched 12.3 MB in 2s (5673 kB/s)
Selecting previously unselected package curl.
(Reading database ... 123456 files and directories currently installed.)
Preparing to unpack .../curl_7.68.0-1ubuntu2_amd64.deb ...
Unpacking curl (7.68.0-1ubuntu2) ...
Selecting previously unselected package git.
Preparing to unpack .../git_1%3a2.25.1-1ubuntu3_amd64.deb ...
Unpacking git (1:2.25.1-1ubuntu3) ...
Selecting previously unselected package vim.
Preparing to unpack .../vim_2%3a8.1.2269-1ubuntu5_amd64.deb ...
Unpacking vim (2:8.1.2269-1ubuntu5) ...
Setting up curl (7.68.0-1ubuntu2) ...
Setting up git (1:2.25.1-1ubuntu3) ...
Setting up vim (2:8.1.2269-1ubuntu5) ...
Processing triggers for man-db (2.9.3-2) ...
Processing triggers for systemd (245.4-4ubuntu3.1) ...
Processing triggers for ureadahead (0.100.0-21) ...
Processing triggers for libc-bin (2.31-0ubuntu9.2) ...
- Monitoring User Activity
Keep track of user activity on the system.
Script:
#!/bin/bash
last
Output:
user1 pts/0 :0 Mon Feb 20 23:45 still logged in
user2 pts/1 :0 Mon Feb 20 23:45 still logged in
reboot system boot 5.4.0-81-generic Mon Feb 20 23:45 still running
user1 pts/0 :0 Mon Feb 20 23:44 still logged in
user2 pts/1 :0 Mon Feb 20 23:44 still logged in
user1 pts/0 :0 Mon Feb 20 23:43 still logged in
user2 pts/1 :0 Mon Feb 20 23:43 still logged in
- Checking for Rootkits
Scan the system for rootkits.
Script:
#!/bin/bash
sudo chkrootkit
Output:
ROOTDIR is /
Checking 'df'...
Checking 'du'...
Checking 'find'...
Checking 'fuser'...
Checking 'getty'...
Checking 'ifconfig'...
Checking 'init'...
Checking 'inodes'...
Checking 'ipcs'...
Checking 'killall'...
Checking 'last'...
Checking 'lastlog'...
Checking 'login'...
Checking 'lsof'...
Checking 'netstat'...
Checking 'named'...
Checking 'netconfig'...
Checking 'ps'...
Checking 'psacct'...
Checking 'pstree'...
Checking 'rpcinfo'...
Checking 'sshd'...
Checking 'snmpd'...
Checking 'tcpd'...
Checking 'timed'...
Checking 'utmp'...
Checking 'w'...
Checking 'wtmp'...
Checking 'xinetd'...
- Updating Bash Scripts
Automatically update Bash scripts.
Script:
#!/bin/bash
git pull origin main
Output:
Already up to date.
- Automating Git Commits
Automate the process of committing changes to Git.
Script:
#!/bin/bash
git add.
git commit -m "Automated commit"
git push origin main
Output:
[main 1234567] Automated commit
1 file changed, 1 insertion(+)
Counting objects: 1, done.
Delta compression using up to 4 threads.
Compressing objects: 100% (1/1), done.
Writing objects: 100% (1/1), 123 bytes | 123.00 KiB/s, done.
Total 1 (delta 0), reused 0 (delta 0)
To https://github.com/user/repo.git
1234567..1234568 main -> main
- Automating System Reboots
Schedule system reboots.
Script:
#!/bin/bash
sudo shutdown -r now
Output:
Broadcast message from user@hostname
(/dev/pts/0) at 23:45 ...
The system is going down for reboot NOW!
Connection to hostname closed by remote host.
Connection to hostname closed.
- Checking Disk Integrity
Verify disk integrity.
Script:
#!/bin/bash
sudo fsck -Af -M
Output:
fsck from util-linux 2.34
e2fsck 1.45.5 (07-Jan-2020)
/dev/sda1: clean, 123456/123456 files, 123456/123456 blocks
- Managing Services
Start, stop, and restart services.
Script:
#!/bin/bash
sudo systemctl restart apache2
Output:
[sudo] password for user:
- Synchronizing Time
Synchronize system time with NTP server.
Script:
#!/bin/bash
sudo ntpdate -u pool.ntp.org
Output:
20 Feb 23:45:01 ntpdate[1234]: adjust time server 123.123.123.123 offset 0.000123 sec
- Configuring Swap Space
Set up and configure swap space.
Script:
#!/bin/bash
sudo dd if=/dev/zero of=/swapfile bs=1M count=2048
sudo mkswap /swapfile
sudo swapon /swapfile
Output:
2048+0 records in
2048+0 records out
2147483648 bytes (2.1 GB, 2.0 GiB) copied, 1.234 s, 1.7 GB/s
Setting up swapspace version 1, size = 2097148 KiB
no label, UUID=12345678-1234-1234-1234-123456789012
Swap space successfully setup
- Clearing Cache
Clear system cache.
Script:
#!/bin/bash
sudo sync; sudo sysctl -w vm.drop_caches=3
Output:
vm.drop_caches = 3
- Setting Up Environment Variables
Configure environment variables.
Script:
#!/bin/bash
export JAVA_HOME=/usr/lib/jvm/java-8-openjdk-amd64
export PATH=$PATH:$JAVA_HOME/bin
Output:
undefined
- Managing Disk Quotas
Set disk quotas for users.
Script:
#!/bin/bash
sudo setquota -u username 1000 2000 0 0 /dev/sda1
Output:
quotacheck: Cannot stat '/dev/sda1': No such file or directory
- Configuring SSH Access
Configure SSH access and settings.
Script:
#!/bin/bash
sudo systemctl restart ssh
sudo ufw allow22/tcp
Output:
[sudo] password for user:
- Checking for Updates
Check for available system updates.
Script:
#!/bin/bash
sudo apt-get update
sudo apt-get upgrade -s
Output:
Hit:1 http://archive.ubuntu.com/ubuntu focal InRelease
Get:2 http://archive.ubuntu.com/ubuntu focal-updates InRelease [114 kB]
Get:3 http://archive.ubuntu.com/ubuntu focal-security InRelease [114 kB]
Fetched 228 kB in 1s (228 kB/s)
Reading package lists... Done
Building dependency tree
Reading state information... Done
Calculating upgrade... Done
The following packages will be upgraded:
libpython3.8-minimal libpython3.8-stdlib
2 upgraded, 0 newly installed, 0 to remove and 0 not upgraded.
Need to get 12.3 MB of archives.
After this operation, 34.5 MB of additional disk space will be used.
- Managing Backups
Schedule and manage backups.
Script:
#!/bin/bash
crontab -l > mycron
echo "0 2 * * * /path/to/backup_script.sh" >> mycron
crontab mycron
rm mycron
Output:
crontab: installing new crontab
- Creating Aliases
Create command aliases for efficiency.
Script:
#!/bin/bash
echo "alias ll='ls -la'" >> ~/.bashrc
source ~/.bashrc
Output:
undefined
- Automating File Transfers
Automate file transfers between systems.
Script:
#!/bin/bash
scp /path/to/local/file user@remote:/path/to/remote/file
Output:
file 100% 1234 1.2MB/s 00:00
- Managing Firewall Rules
Add or remove firewall rules.
Script:
#!/bin/bash
sudo ufw allow 8080/tcp
sudo ufw deny 8080/tcp
Output:
Rule added
Rule deleted
- Logging System Information
Log detailed system information regularly.
Script:
#!/bin/bash
echo "Logging system information..."
uname -a >> /var/log/sysinfo.log
df -h >> /var/log/sysinfo.log
free -m >> /var/log/sysinfo.log
Output:
Logging system information...
- Generating Random Passwords
Generate random passwords for secure accounts.
Script:
#!/bin/bash
openssl rand -base64 12
Output:
random_password_here
- Automating System Monitoring
Monitor system resources and performance.
Script:
#!/bin/bash
echo "System Monitoring"
top -bn1 | grep "Cpu(s)"
free -m
df -h
Output:
System Monitoring
%Cpu(s): 1.3%us, 0.3%sy, 0.0%ni, 98.0%id, 0.3%wa, 0.0%hi, 0.0%si, 0.0%st
total used free shared buff/cache available
Mem: 7972 1234 5678 123 456 6789
Filesystem Size Used Avail Use% Mounted on
/dev/sda1 50G 20G 28G 42% /
- Creating System Snapshots
Create snapshots of the system for backup and recovery.
Script:
#!/bin/bash
sudo snapshot -c /dev/sda1
Output:
snapshot: created snapshot '2023-02-20-23:45:01' of '/dev/sda1'
- Automating System Upgrades
Automate system upgrades and updates.
Script:
#!/bin/bash
sudo apt-get update && sudo apt-get upgrade -y
Output:
Reading package lists... Done
Building dependency tree
Reading state information... Done
Calculating upgrade... Done
The following packages will be upgraded:
libpython3.8-minimal libpython3.8-stdlib
2 upgraded, 0 newly installed, 0 to remove and 0 not upgraded.
Need to get 12.3 MB of archives.
After this operation, 34.5 MB of additional disk space will be used.
- Managing System Services
Manage system services, such as starting, stopping, and restarting.
Script:
#!/bin/bash
sudo systemctl start apache2
sudo systemctl stop apache2
sudo systemctl restart apache2
Output:
[sudo] password for user:
- Automating System Configuration
Automate system configuration, such as setting up network interfaces.
Script:
#!/bin/bash
sudo ip link set eth0 up
sudo ip addr add 192.168.1.100/24 brd 192.168.1.255 dev eth0
Output:
undefined
- Creating System Images
Create system images for deployment.
Script:
#!/bin/bash
sudo dd if=/dev/sda1 of=/path/to/image.img
Output:
2048+0 records in
2048+0 records out
2147483648 bytes (2.1 GB, 2.0 GiB) copied, 1.234 s, 1.7 GB/s
- Automating System Testing
Automate system testing, such as running tests and verifying results.
Script:
#!/bin/bash
./run_tests.sh
Output:
Running tests...
All tests passed.
- Managing System Users
Manage system users, such as creating, modifying, and deleting users.
Script:
#!/bin/bash
sudo useradd newuser
sudo usermod -aG sudo newuser
sudo userdel newuser
Output:
undefined
- Automating System Backup and Recovery
Automate system backup and recovery, such as creating backups and restoring systems.
Script:
#!/bin/bash
sudo rsync -av --delete /source/directory /backup/directory
sudo restore -C /backup/directory /target/directory
Output:
rsync: deleted 'target/directory/file'
restore: Restoring /backup/directory/file to /target/directory/file
- Creating System Documentation
Create system documentation, such as generating reports and documentation.
Script:
#!/bin/bash
echo "System Documentation" > system_doc.txt
uname -a >> system_doc.txt
df -h >> system_doc.txt
free -m >> system_doc.txt
Output:
System Documentation
Linux hostname 5.4.0-81-generic #88-Ubuntu SMP Thu Jan 20 13:12:44 UTC 2022 x86_64 x86_64 x86_64 GNU/Linux
Filesystem Size Used Avail Use% Mounted on
/dev/sda1 50G 20G 28G 42% /
total used free shared buff/cache available
Mem: 7972 1234 5678 123 456 6789
Conclusion
In this blog post, I've shown you how to use Bash to automate tasks on Linux, Mac, and Windows PCs. I've covered basic Bash scripting concepts, such as variables, conditional statements, and loops, and provided examples of automating system maintenance tasks, processing data, and analyzing log files. Additionally, I've listed 60 tasks that can be automated with Bash in Linux, along with examples and explanations, and included outputs for each task.
By using Bash scripts, you can automate repetitive tasks, making your life easier and more efficient. Whether you're a system administrator, support engineer, or software engineer, Bash scripting is an essential skill to have in your toolkit.
Subscribe to my newsletter
Read articles from Niladri Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
