Common JavaScript Errors and How to Fix Them
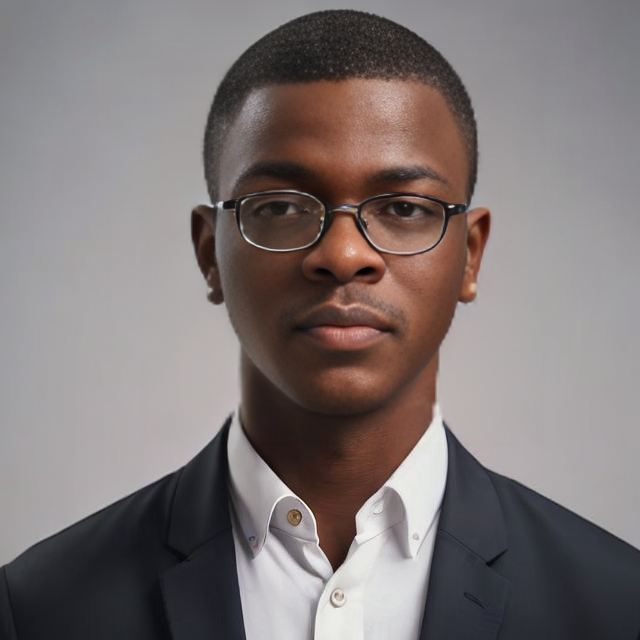
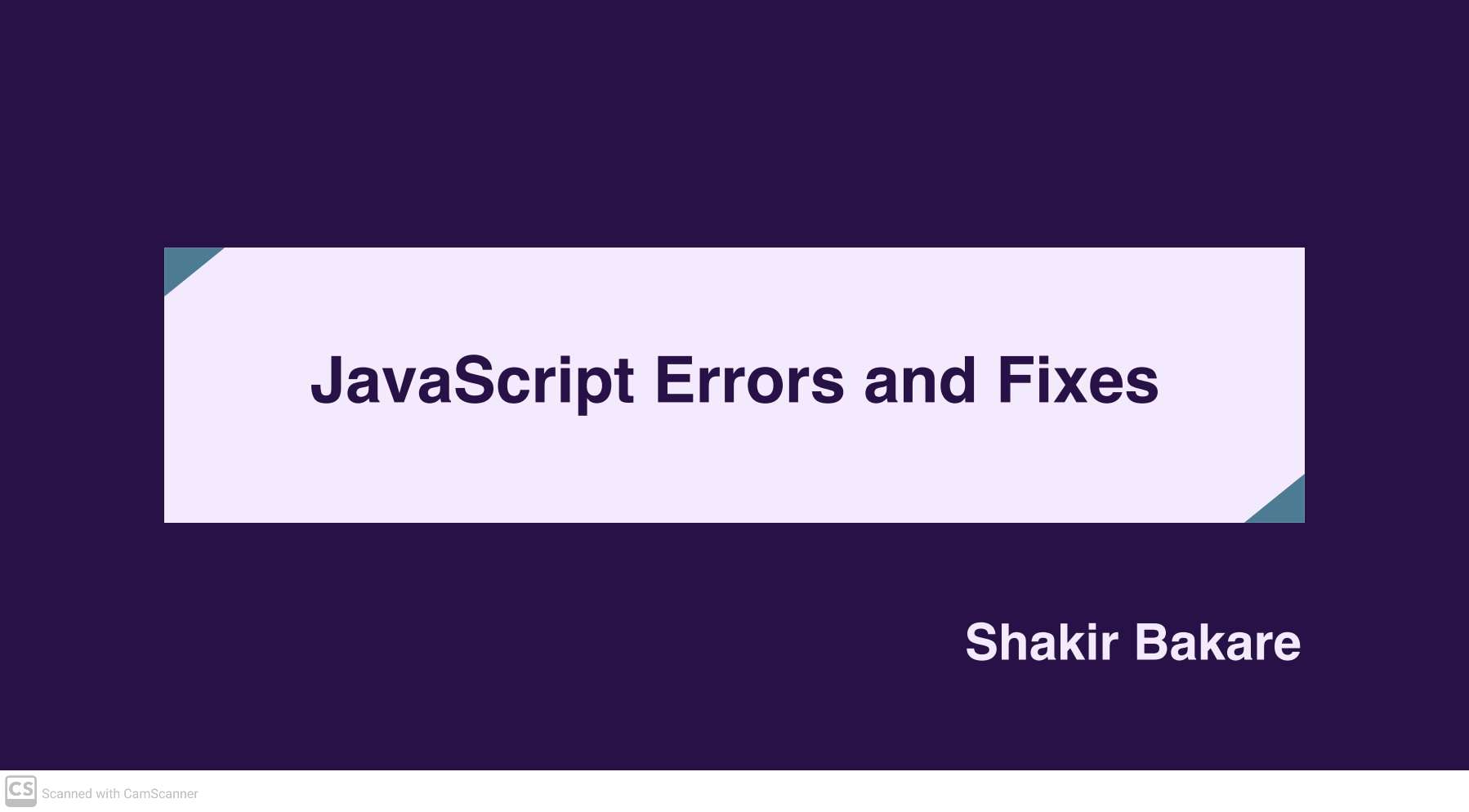
Errors can be frustrating and time-consuming to debug. Understanding common JavaScript errors and knowing how to fix them is crucial for efficient coding and maintaining robust applications. In this article, I will discuss some of the most frequent JavaScript errors, such as ReferenceError
, SyntaxError
, TypeError
, and RangeError
, and provide practical tips on how to prevent and resolve them.
1. ReferenceError
Happens when a variable is not found. This can be because of one of the following reasons:
ReferenceError
happens in the example because x
is not declared. You must declare a variable before you can use it.
let x = 9;
console.log(x)
// Outputs 9.
- You try to access a variable outside its scope.
function sampleFunc() {
let y = 6;
}
console.log(y);
// ReferenceError: y is not defined
The y
variable is declared inside the sampleFunc
block, not outside it. But console.log
tries to access the y
variable outside the scope. That's why the ReferenceError
happens.
- You write a variable name wrongly.
Example:
let sampleVariable = 29;
console.log(sampelVariable);
// ReferenceError: sampelVariable is not defined
ReferenceError
happens in the example because there's a typo in the sampleVariable
name. sampelVariable
is used with console.log
instead of sampleVariable
. Do you understand? “sampel” instead of “sample".
How to prevent ReferenceError
Make sure you declare variables and objects properly before using them.
Make sure you write variables name correctly.
Make sure variables are accessible within the correct scope.
2. SyntaxError
Happens when the JavaScript engine sees a code that doesn't follow the JavaScript’s syntax rules. You can get SyntaxError
because of one of the following reasons:
- You forget to add a parenthesis to a function.
function sampleFunc(){
let x = 5;
}
sampleFunc();
The sampleFunc
function does not have a closing parenthesis. The example will have a SyntaxError
.
You will also get a SyntaxError
if you forget to add a brace (opening or closing {}) in your code.
You repeat a variable name.
Your code is missing an important property. For example, the increment sign.
How to prevent SyntaxError
Double check your code for missing or additional operators like the plus sign (
+
).Make sure your variables have unique names.
Make sure your code does not miss any important property (parenthesis, brace, and the like)
3. TypeError
Happens when an operation is performed on a value of an inappropriate type. This can be because of one of the following reasons:
- You call a non-function as a function.
let x = 29;
x();
// TypeError: x is not function.
- You use the wrong operators with the wrong type.
let x = "string" - 1;
// TypeError: invalid arithmetic operation.
- You pass an incorrect argument to a function.
var numbers = [1, 2, 3];
numbers.reduce(5);
// TypeError: 5 is not a function
(reduce expects a function as its argument)
How to prevent TypeError
Make sure you give functions correct arguments.
Use linting tools like ESLint to catch potential type errors during development.
Be sure you don't use a variable as a function.
4. RangeError
Happens when a value is not within the set or allowed range for values.
Reasons:
- You create an array with an invalid length.
let arr = new Array(-1);
// RangeError: Invalid array length
let arr2 = new Array(2**32);
// RangeError: Invalid array length
Certain functions expect arguments to be within a specific range. For example, some mathematical functions or methods dealing with strings may throw a
RangeError
when the arguments are outside the allowed range.You change the length property of an existing array to an invalid value.
let arr = [];
arr.length = -1;
// RangeError: Invalid array length
arr.length = 2**32;
// RangeError: Invalid array length
How to prevent RangeError
Validate input values before using them in functions or methods that expect specific ranges.
Ensure the length of arrays is within the valid range (0 to (2^{32} - 1)).
You try to access a variable you haven't declared.
Example:
console.log(x);
// ReferenceError: x is not defined
Conclusion
Understanding and addressing common JavaScript errors such as ReferenceError
, SyntaxError
, TypeError
, and RangeError
is essential for developing robust and efficient applications. By familiarizing yourself with the causes and solutions for these errors, you can significantly reduce debugging time and improve your coding skills. Implementing best practices, such as proper variable declaration, scope management, and using linting tools, will help you prevent these errors and create more reliable JavaScript applications. Keep honing your skills and stay updated with the latest JavaScript developments to continue writing clean and error-free code.
Thank you for reading, and if you found this article helpful, please like, share, and leave your feedback.
Lets connect
Subscribe to my newsletter
Read articles from Shakir Bakare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
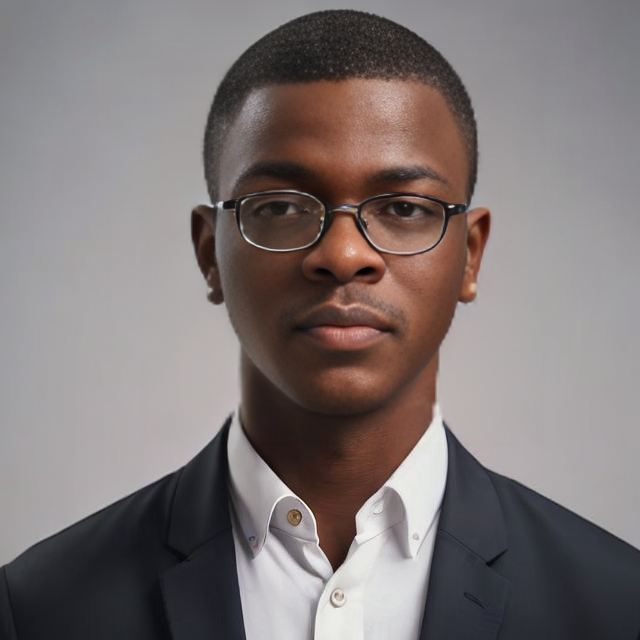
Shakir Bakare
Shakir Bakare
I'm a passionate frontend web developer with a love for creating beautiful and responsive web applications. With a strong foundation in HTML, CSS, JavaScript, and frameworks like React and Vue.js, I strive to bring innovative ideas to life. When I'm not coding, I enjoy sharing my knowledge through technical writing. I believe in the power of clear and concise documentation, and I write articles and tutorials to help others on their coding journey. Let's connect and create something amazing together!