A Recent Backend Problem That I Had To Solve
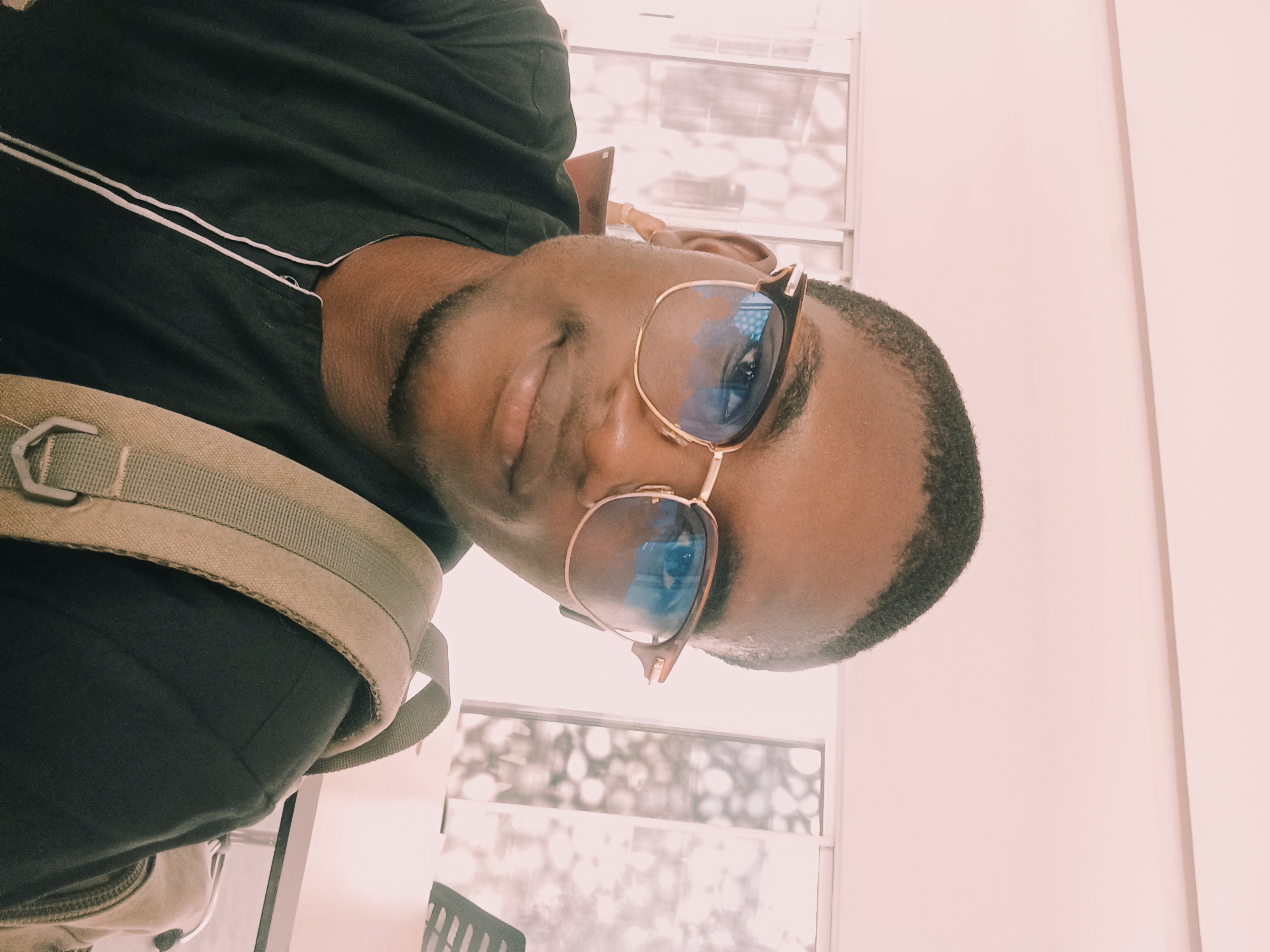
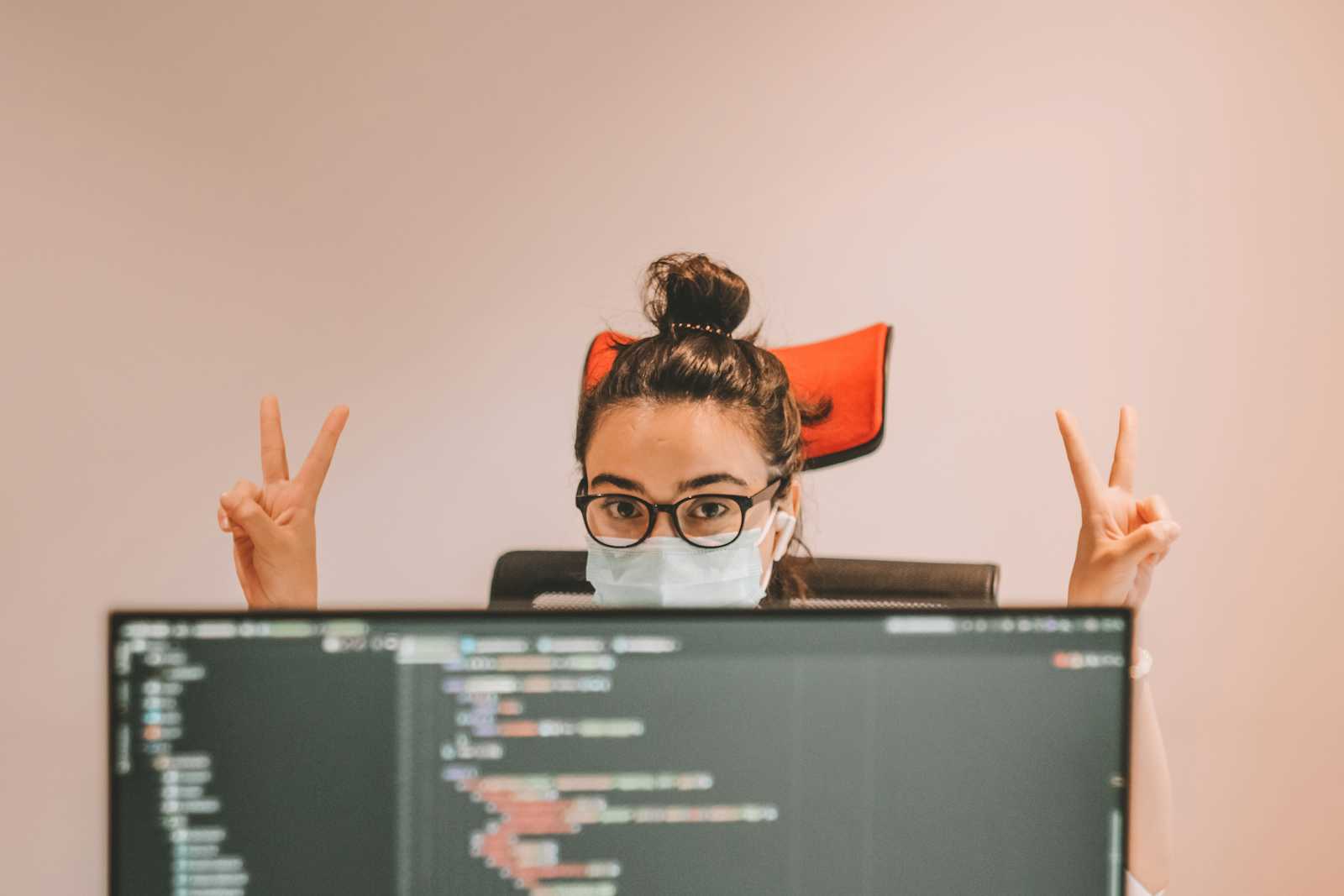
Let us assume that you are working on a backend project with NodeJS and this project has lots of API endpoints that need strict validation on each of the routes. How best would you validate these endpoints and still return a user-friendly error to the end user?
Today, I wish to share this problem with you and the solution that I came up with to tackle this problem.
In the era of backend development, there is a saying that goes like this "Never trust user input". Well, this is true only for the reason that a single unsanitized input from a user can compromise your entire system and that is the reason why you should guard your API endpoints with strict validation rules using a nice validation library.
What is a Validation Library?
A validation library is a module or package that accepts data and a set of rules, designed to sanitize, compare, and ensure that the data submitted is in the required format.
So this means that when interacting with a system that accepts user input, there is a library or module that helps to check if you provided the correct email address, and phone number, or even uploaded the right documents.
There are so many validation libraries on the internet now, but I would only focus on octavalidate, which appears to be my solution to this problem.
Introducing Octavalidate For NodeJS
I started this project in 2022 because I enjoyed watching people try to bypass systems that claim to offer the best protection and I know this also positively impacted my love for cybersecurity.
Octavalidate started with the native javascript environment (javascript that runs on the browser) and this offered maximum protection to any website it was integrated into because it had a rich set of validation rules and offered customizable input attributes.
Here's an excerpt of this library from CSS scripts that I enjoy reading;
OctaValidate is an open-source, feature-rich form validation JavaScript library that makes it easy to validate form input against built-in rules, regular expressions, and input attributes. It can help you validate the HTML forms in your application to increase user engagement by providing grouped errors, it can also help you make your forms manageable and consistent across many devices and browsers.
This was posted on the 4th of February, 2023 and today I wish to officially announce that this validation library fully supports the NodeJS environment ๐.
Well, the library was there for NodeJS 2 years ago, but the truth is that it didn't give me the confidence that I needed then to present it to my peers and even boast of a great and quality source code, and after so many procrastination, I was able to refactor the source code into what I consider a better version of the package today and this took me just 1 week.
The library is also there for ReactJs, and this hasn't been updated yet to support anything React. So, yes you can say I am procrastinating again ๐
How to Use Octavalidate in Your NodeJS Environment
One thing I love to do when working on a project is to make sure that everything is both user-friendly and developer-friendly.
Developer friendly in the sense that I use comments in my source codes to explain what a particular module or function does and I try to use libraries that don't give much of a headache during integration.
Today, I can assure you that this library fully supports the NodeJS environment and can be used to validate both file and non-file form inputs.
To use this library in your project, you just need to import it, define and assign validation rules to it, and then proceed to validate your payload.
Now, that's a very good way to summarize how easy it is to integrate this library into your project.
Consider the sample code below;
//import library
const Octavalidate = require("octavalidate-nodejs");
//create new validation instance
const octavalidate = new Octavalidate("ROUTE_IDENTIFIER");
//get validation methods
const { createValidator, validate, getError, getErrors } = octavalidate;
//define validation rules
const validationRules = {
username: {
required: true,
type: "string",
pattern: /^[a-zA-Z][a-zA-Z0-9-_]+$/,
},
};
//assign validation rules
createValidator(validationRules);
//validate your JSON payload
//true means validation passed
//false means validation failed
const validationResponse = validate(req.body);
//return single error
console.log(getError());
//return all errors
console.log(getErrors());
Just merely looking at the code above, you will immediately understand that we are trying to validate the form input username
with validation rules that read;
Your username is required
Your username must be of type string
Your username must contain alphanumeric characters and may include underscores or hyphens
Now, let me explain in detail how you can use Octavalidate in your NodeJS environment.
After importing the library, you just have to create validation rules.
//define validation rules
const validationRules = {
username: {
required: true,
type: "string",
pattern: /^[a-zA-Z][a-zA-Z0-9-_]+$/,
},
};
Once you have created your validation rules, you need to assign them to the createValidator
method from the script so that the script will validate these rules and separate files from non-file inputs in its logical way.
//import library
const Octavalidate = require("octavalidate-nodejs");
//create new validation instance
const octavalidate = new Octavalidate("ROUTE_IDENTIFIER");
//get validation methods
const { createValidator, validate, getError, getErrors } = octavalidate;
//define validation rules
const validationRules = {
username: {
required: true,
type: "string",
patten: /^[a-zA-Z][a-zA-Z0-9-_]+$/,
},
};
//assign validation rules
createValidator(validationRules);
After assigning these rules, you are ready to validate the payload that would contain these form inputs. In the code below, I am trying to validate the req.body
payload, so this is what I would do;
//import library
const Octavalidate = require("octavalidate-nodejs");
//create new validation instance
const octavalidate = new Octavalidate("ROUTE_IDENTIFIER");
//get validation methods
const { createValidator, validate, getError, getErrors } = octavalidate;
//define validation rules
const validationRules = {
username: {
required: true,
type: "string",
pattern: /^[a-zA-Z][a-zA-Z0-9-_]+$/,
},
};
//assign validation rules
createValidator(validationRules);
//validate request body
if(validate(req.body)){
//you are good to go
}else{
//return error
}
The return data type of this method is boolean
and this means that the value could either be true
or false
true
means that the validation passedfalse
means that the validation failed
So this means that whenever you receive true
as the validation response, you are good to go, and when you receive false
, you should return a friendly error message to the end user ....Psst, let your validation rules be the judge here ๐ซข
How easy was that? Pretty clear and concise right?
Rounding up
Currently, I am part of an intensive 6-month internship program at HNG and there is no greater reason why I joined this internship program other than to upskill myself and have more experience under my sleeves and this article happens to be my first task - wonderful.
I wanted to try out taking on both frontend and backend but I guess I have to stick to one because I have some other activities currently using up most of my time like education.
This might be the first time I'm writing an article like this but it definitely won't be the last. If you wish to check out octavalidate for the NodeJS environment, please refer to the links below and if you want to contribute to the repo or add any comment to this article, please leave a good message for me below ๐
I appreciate your time.
Subscribe to my newsletter
Read articles from Simon Ugorji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
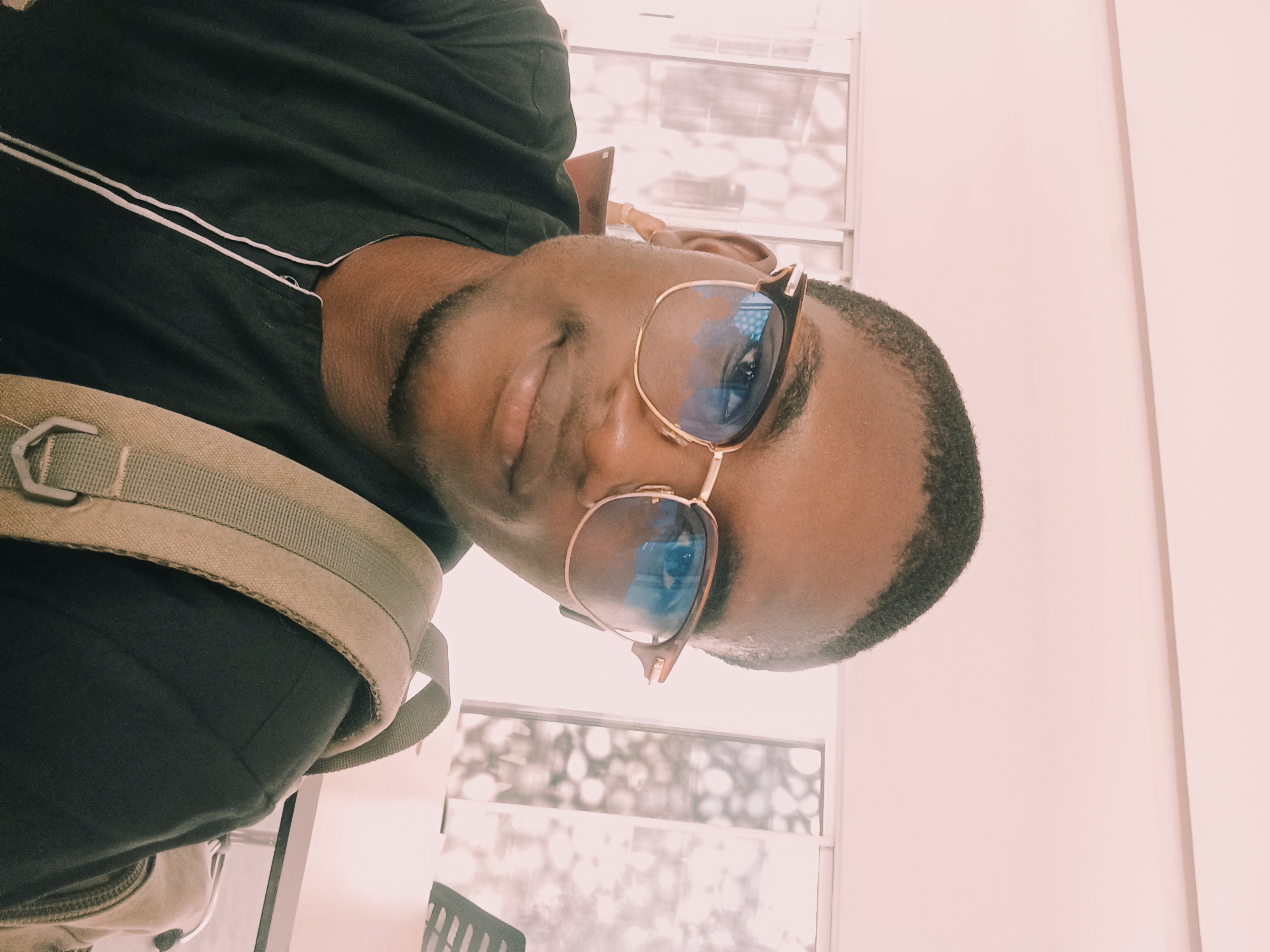
Simon Ugorji
Simon Ugorji
My name is Simon Ugorji and I aspire to become a computer scientist. I officially started my web development journey in the year 2020 as a backend web Developer and I have enjoyed the experience so far. I spend my day coding, learning new topics, and figuring out the next article to share with the world.