Enhance Your Java Skills with Advanced Switch Statements
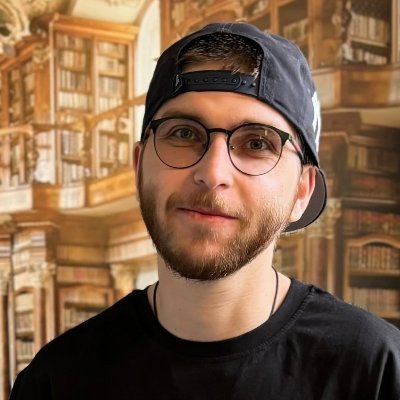
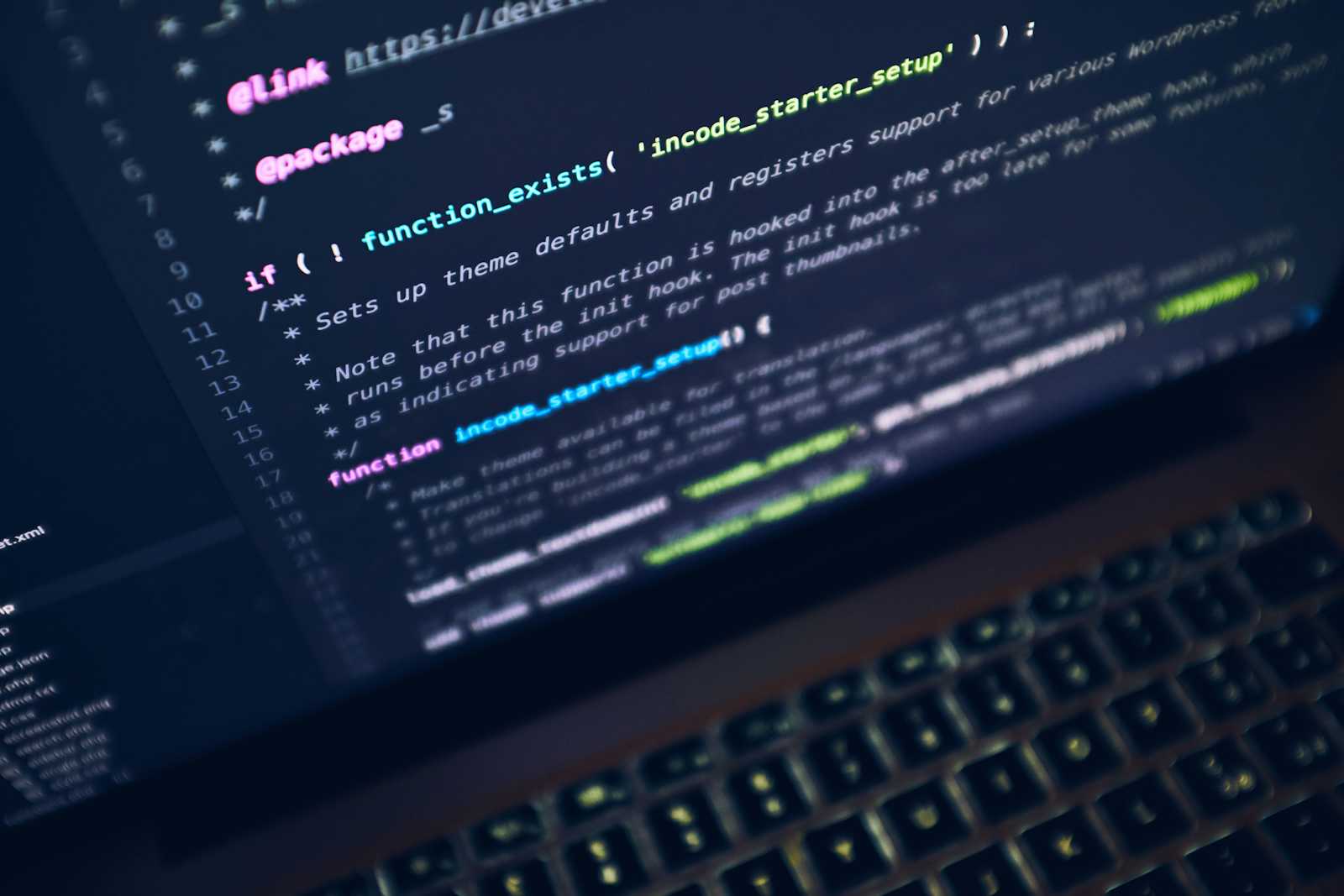
Java has made significant improvements to switch statements, making them more versatile and expressive. Starting with Java 14, you can now write switch constructs that are more robust, flexible, and easier to read. Let's explore the exciting new features available from Java 14 onwards and see how they can enhance your code.
Java 14: Switch Expressions
Java 14 introduced switch expressions, allowing you to use switch statements not just for control flow but also to return values. This means you can write concise code that evaluates an expression and returns a result based on matching cases. Here's an example of a switch expression calculating Fibonacci numbers:
int fibonacci(int i) {
return switch (i) {
case 0, 1 -> i;
default -> fibonacci(i - 1) + fibonacci(i - 2);
};
}
Pattern Matching with instanceof (Java 16)
Java 16 introduced pattern matching for instanceof
checks. This allows you to write more concise and readable conditional logic when dealing with object types. Instead of long chains of if-else
statements, you can use patterns to match the type and potentially access the object's properties directly within the condition itself.
Here's an example demonstrating pattern matching with instanceof
:
void print(Object o) {
if (o instanceof String s && s.length() > 10) {
System.out.printf("a long string: %s...", s.substring(0, 10));
} else if (o instanceof String s) {
System.out.print("a not so long string: " + s);
} else if (o instanceof Integer i) {
System.out.print("an integer whose square is: " + i * i);
}
}
Conclusion
In conclusion, the enhancements to switch statements and the introduction of pattern matching with instanceof
in Java 14 and 16 respectively bring significant improvements to the language. These features make your code more concise, readable, and expressive. By leveraging these advanced capabilities, you can write more efficient and maintainable Java programs, ultimately enhancing your overall development skills.
Subscribe to my newsletter
Read articles from Christian Lehnert directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
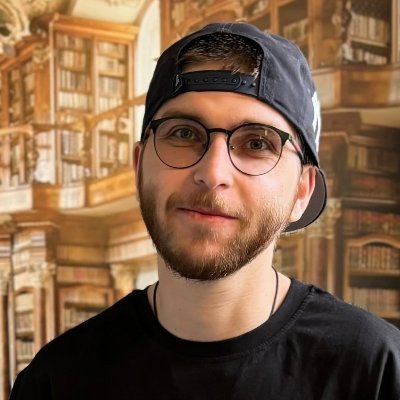
Christian Lehnert
Christian Lehnert
SR. Developer & CEO | Reduced Server Costs by 50% | 6+ Years Experience as Backend Developer | Expertise in Python, Java & Spring Boot | 30+ Certifications