Dunder in python
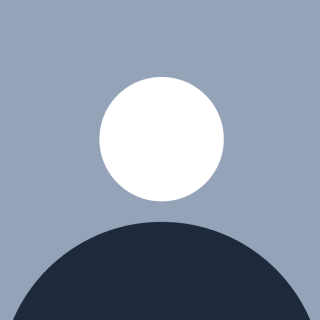
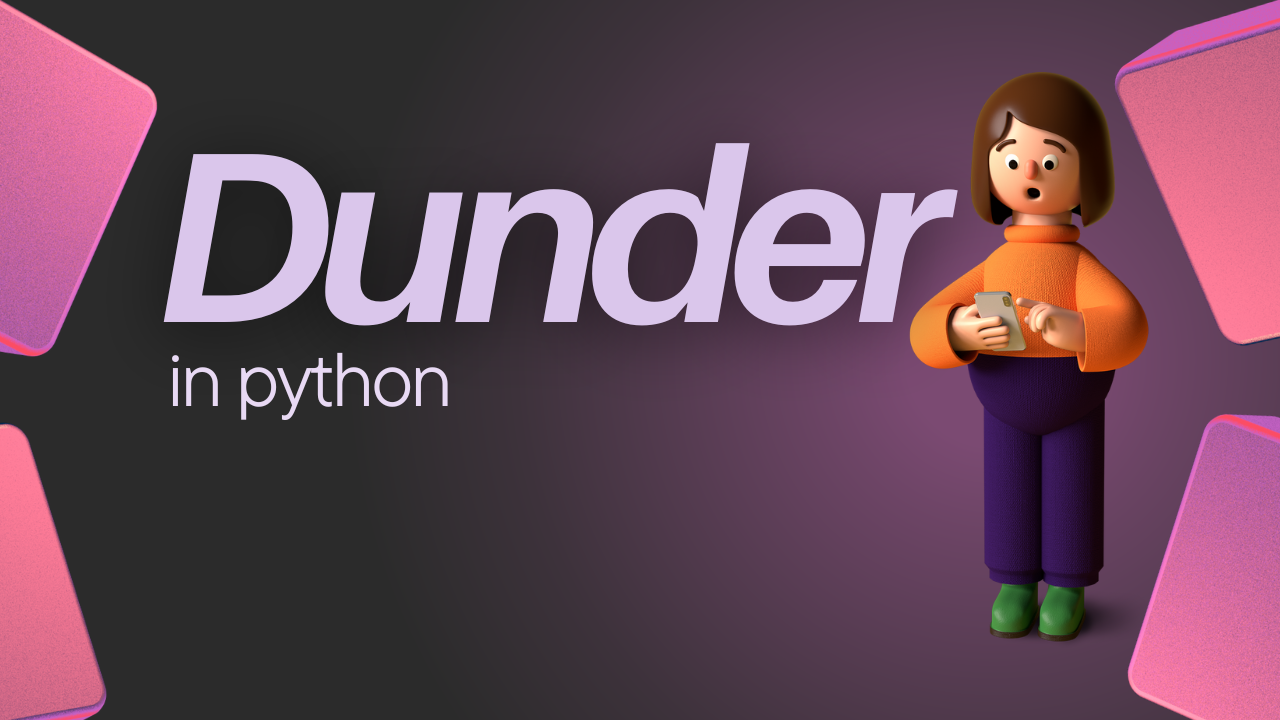
Dunder stands for "double underscore." In Python, these are special methods (or functions) that have double underscores at the beginning and end of their names. They're also called "magic methods."
Imagine you're playing with a special toy. It has buttons that do cool things when you press them. In Python, dunder methods are like these buttons—they make your code do cool and special things.
To check all the dunder methods (special methods) of a class or an object in Python, you can use the dir()
function. This function lists all the attributes and methods of the object, including the dunder methods.
Here's how you can do it:
class MyClass:
def __init__(self, name):
self.name = name
def my_method(self):
print("Hello")
# Create an instance of the class
my_instance = MyClass("Example")
# Use dir() to list all attributes and methods of the instance, including dunder methods
print(dir(my_instance))
This will print a list of all the attributes and methods of the my_instance
object, including the special dunder methods like __init__
, __str__
, __repr__
, and many others.
For example:
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'my_method', 'name']
This command works with any object or class, so you can use it to explore the dunder methods available in any part of your code.
Special Dunder Methods
__init__
:Think of this as the starting point of a toy. When you get a new toy, you have to set it up before you play with it.
In Python,
__init__
is used to set up a new object when you create it. It’s called the constructor.
class Toy:
def __init__(self, name):
self.name = name # Set up the toy with its name
my_toy = Toy("Robot")
print(my_toy.name) # Output: Robot
__main__
:Imagine you're deciding whether to play inside or outside. You check the weather first to decide.
In Python,
__main__
helps decide if your code should run as a standalone program or be used by other programs.if __name__ == "__main__": print("This code is running directly!") else: print("This code is being imported!")
When you run the file directly, __name__
is __main__
, so it prints "This code is running directly!"
Summary
Dunder Methods: Special methods with double underscores like
__init__
and__main__
that do important things in Python.__init__
: Sets up a new object with its initial values, like setting up a new toy.__main__
: Decides if the code should run directly or be used by other programs, like deciding to play inside or outside.
Subscribe to my newsletter
Read articles from Anushka Kishor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Anushka Kishor
Anushka Kishor
I'm Anushka Kishor, hailing from the culturally rich state renowned for its culinary delights in "Litti Chokha." Currently, I am fervently pursuing my Bachelor's degree at Sagar Institute of Science and Technology, Bhopal. Proficient in programming languages like HTML, CSS, JavaScript, React.js, and Tailwind CSS, along with C and C++, I also excel in Data Structures and Algorithms. I am honored to serve as the Lead for Google Developer Student Clubs, where I orchestrate initiatives aimed at fostering innovation and collaboration within our academic ecosystem. Beyond the realm of technology, I find solace and self-expression in the realms of art and sport. Acrylic painting serves as my canvas for creative exploration, while the exhilarating game of badminton keeps me grounded and energized. Committed to continuous learning, I'm passionate about leadership and self-improvement. Join me on this journey where every challenge is an opportunity for growth.