Overcoming Challenges in Backend Development: My Journey with Django Serializers
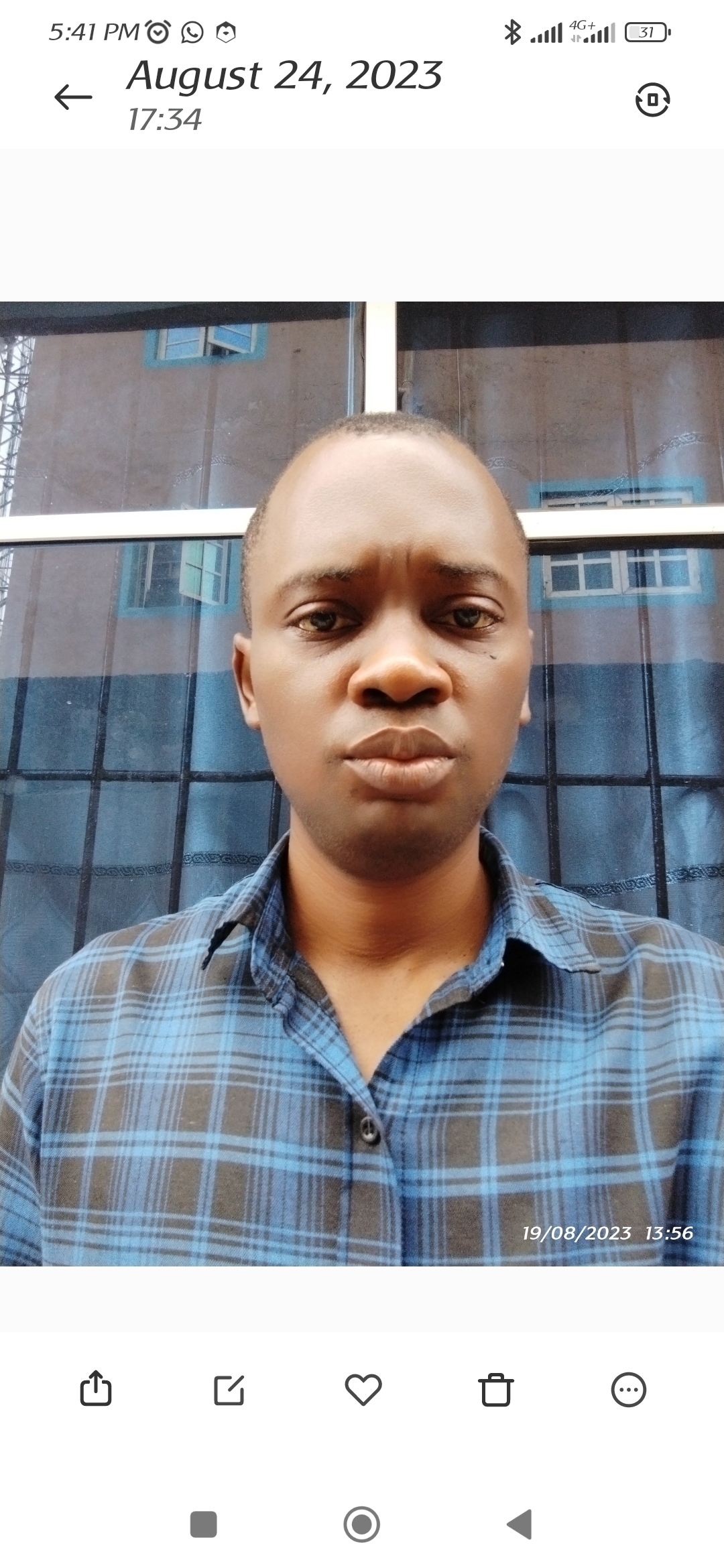
I am a not a traditional software engineer as i didn't go through the four walls of a computer science school. My knowledge and skills were gotten from watching tutorials on YouTube and coding along, joining boot-camps and collaborating with other in doing assigned tasks. Last year i joined bootcamp like HNG 10 to gain practical knowledge.
As a back-end developer, solving complex problems is part of the daily routine. Last year, I faced a particularly challenging task that not only tested my skills but also broadened my understanding of Django and APIs. This experience was a pivotal moment in my self learning and I'm excited to share how I navigated through it.
The Challenge: Learning Django Serializers
I have always been comfortable working with Django for web development. However, when my assignments started to include API cases, I realized I needed to expand my skill set. One of the first tasks I encountered was to take a name as input and return it as a parameter through an API. This required me to learn how to use Django serializers, something I had never done before.
Step-by-Step Solution
Step 1: Understanding Django Serializers
The first step was to understand what Django serializers are and how they work. Serializers in Django are used to convert complex data types, such as querysets and model instances, into native Python data types that can then be easily rendered into JSON, XML, or other content types. They are also used for deserialization, allowing parsed data to be converted back into complex types, after first validating the incoming data.
Step 2: Install Python and Pip and Set up Virtual Environment
Make sure you have Python and pip installed. You can check this by running:
python --version
pip --version
If they are not installed, download and install Python from python.org
You can create a virtual environment using the venv
module, which is included in the standard library.
On Windows:
mkdir myapi-project && cd myapi-project
python -m venv myenv
On macOS and Linux:
mkdir myapi-project && cd myapi-project
python3 -m venv myenv
You should now see (myenv)
at the beginning of your command prompt, indicating that the virtual environment is active.
Step 3: Install Packages with pip
Now you can install the packages you need using pip. For example:
pip install Django
pip install djangorestframework
Step 4: Setting Up the Project
If we have read up to this point and done the above steps, congratulation for staying with me and also for performing the task. Next, I created a new Django app within the project to keep the structure clean and modular. The command lines looked something like this:
django-admin startproject myproject
cd myproject
django-admin startapp myapp
Step 5: Creating the Serializer
Next, I created a serializer for the input data. This involved defining a new class in serializers.py
within the myapp
directory. The serializer needed to handle a single field: name
.
from rest_framework import serializers
class NameSerializer(serializers.Serializer):
name = serializers.CharField(max_length=100)
Step 6: Writing the View
With the serializer in place, I moved on to writing the view that would handle the incoming request and use the serializer to validate and process the data. This was done in views.py
.
from rest_framework.views import APIView
from rest_framework.response import Response
from rest_framework import status
from .serializers import NameSerializer
class NameView(APIView):
def post(self, request):
serializer = NameSerializer(data=request.data)
if serializer.is_valid():
name = serializer.validated_data['name']
return Response({"name": name}, status=status.HTTP_200_OK)
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
Step 7: Setting Up the URL
Finally, I needed to set up the URL routing so that the API endpoint could be accessed. This was done in urls.py
of the myapp
directory.
from django.urls import path
from .views import NameView
urlpatterns = [
path('name/', NameView.as_view(), name='name'),
]
Step 8: Configuring the Project
To ensure the project runs smoothly, we need to update the settings.py
and include necessary dependencies.
Project settings.py
In settings.py
, make sure to add rest_framework
and your app myapp
to the INSTALLED_APPS
list:
INSTALLED_APPS = [
...
'rest_framework',
'myapp',
]
Project urls.py
Update the main urls.py
file in the project directory to include the app's URLs:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('api/', include('myapp.urls')),
]
Testing the API
With everything set up, Run the Django development server. I used tools like Postman to test the API endpoint. Sending a POST request with a JSON body containing a name returned the name as a parameter, confirming that everything worked as expected.
Expanding My Skill Set
After gaining experience with Django serializers, I continued to build on my skills by working with other frameworks and tools. I explored FastAPI and Flask, both of which provided unique advantages for different types of projects. I also became proficient with tools like Postman, Swagger UI, and curling on the terminal, which have been invaluable for testing and documenting APIs. I have a great deal about authentication and authorization (JWT, OAuth 2.0), best API designs and practices (versioning, rate limiting, etc.), HTTP methods and status codes, Error handling (logging) and detail error messages, API Documentation (Postman Collections, Swagger/OpenAPI) and many more.
The HNG Internship: A New Chapter
Participating in the HNG 11 internship is an exciting new chapter in my journey. I was eliminated in stage 7 of HNG 10, but this setback has only fueled my determination to make it to the finals this time - chuckles. The internship offers a platform to learn, grow, and showcase my skills in a competitive environment. It also provides the opportunity to network with like-minded individuals and industry experts.
My goal is to leverage this experience to enhance my skills further and prove my mettle as a backend developer. The journey of learning never stops, and I am committed to pushing my limits and achieving excellence.
Conclusion
Solving the challenge of setting up an API with Django serializers was a significant milestone in my backend development career. It taught me the importance of stepping out of my comfort zone and embracing new technologies. As I embark on the HNG 11 internship, I am ready to face new challenges, learn from them, and emerge stronger and more skilled. The journey is just as important as the destination, and I am excited for what lies ahead.
if you enjoyed this blog, please you can check my previous write up on Building a RESTful API for student Records with Flask and MySQL.
Subscribe to my newsletter
Read articles from Omini Okoi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
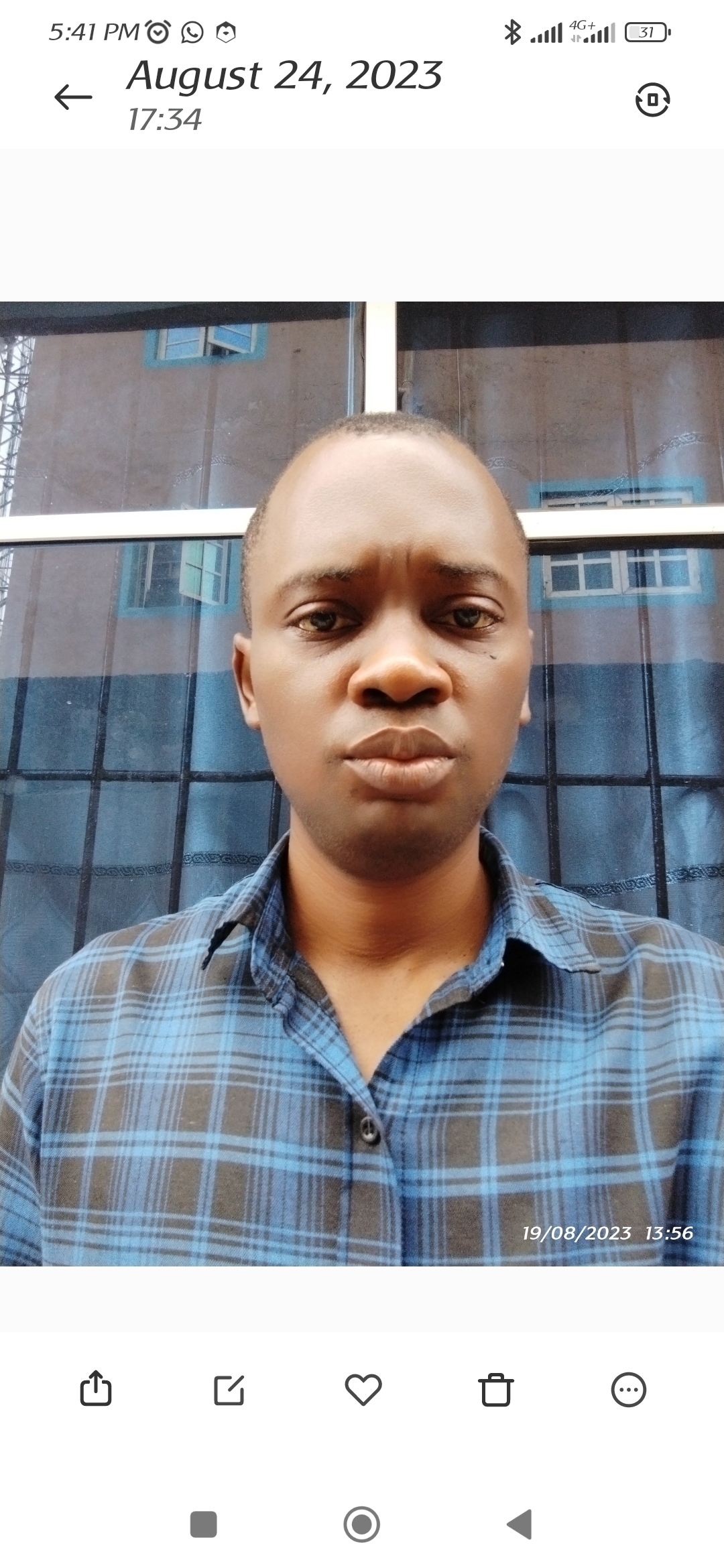
Omini Okoi
Omini Okoi
I am a web developer with experience in back-end engineering and cloud computing. I enjoy mentoring and training others in coding and technology. I’m always open to collaboration and building stuff that can make a positive impact.