Part 2: Creating BFF Architecture using Kubernetes and NodeJs

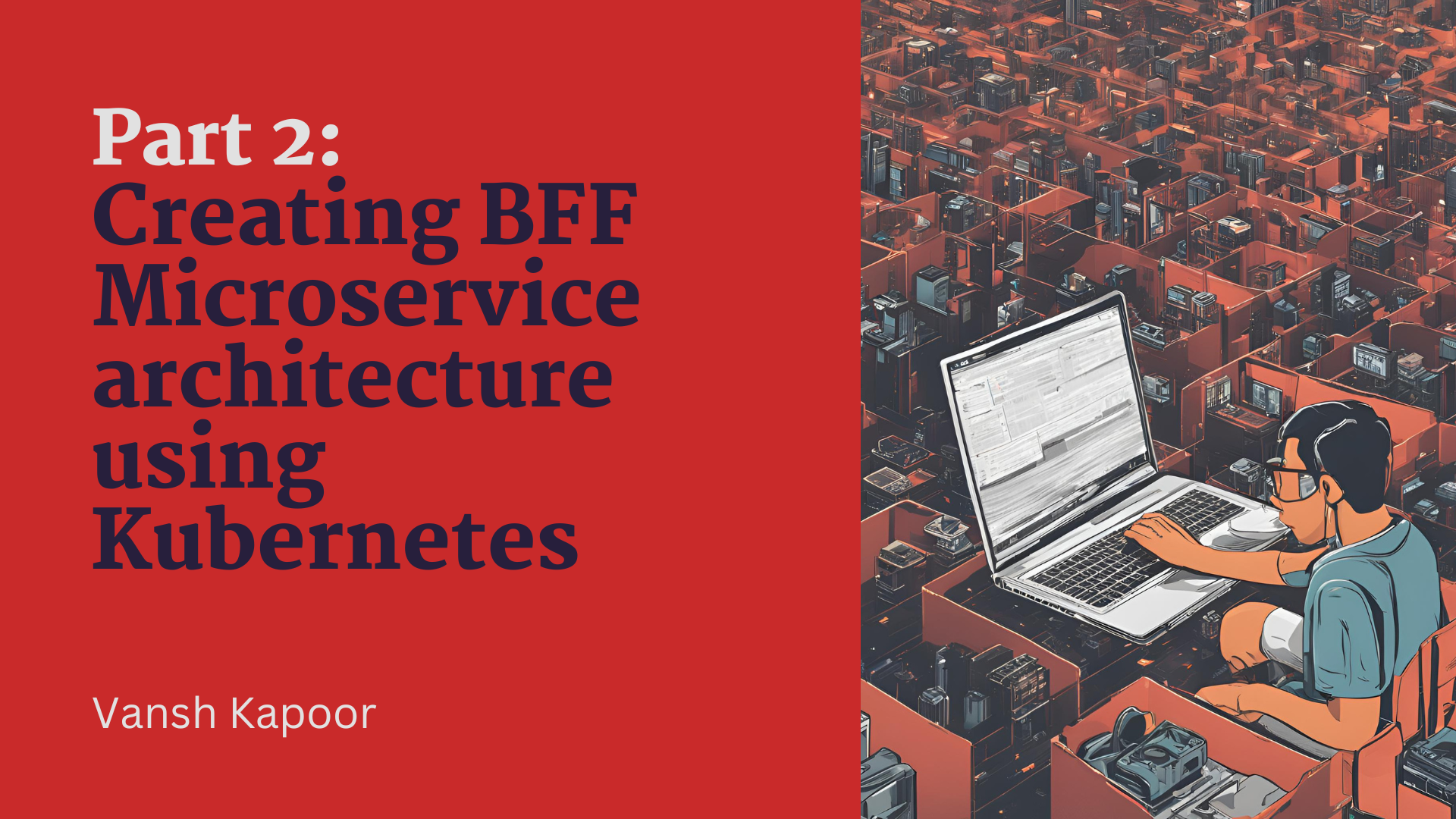
Till now we have understood about BFF pattern and we are already ready with Product SVC architecture. If you just landed here you can check previous implementation of our architecture on this link.
You can checkout the codebase and fork it from here
Our Product SVC is implemented and Now we will start with Order DB and Order Svc.
Development Time
Order DB
We first will setup Order Db in our kubernetes cluster.
We will create deployment and a service for Order Db just like how we created Product Db.
step 1: create .\orderDbDeployment.yml
apiVersion: apps/v1
kind: Deployment
metadata:
name: orderpostgresql
spec:
replicas: 1
selector:
matchLabels:
app: orderPostgresql
template:
metadata:
labels:
app: orderPostgresql
spec:
containers:
- name: orderpostgresql-container
image: postgres:latest
env:
- name: POSTGRES_DB
value: mydatabase
- name: POSTGRES_USER
value: myuser
- name: POSTGRES_PASSWORD
value: mypassword
ports:
- containerPort: 5432
Run the Deployment by
kubectl apply -f .\orderDbDeployment.yml
we can see product postgresql pod is up
Step 2. Create Product Db service. create file orderDbService.yml.
apiVersion: v1
kind: Service
metadata:
name: order-postgresql-service
spec:
selector:
app: orderPostgresql
type: NodePort
ports:
- port: 5432
targetPort: 5432
Run the service up:
kubectl apply -f .\orderDbService.yml
and see the svc is up.
Alrighty then, we are ready with our Order Database and time to create Order service.
Order Service
For order service, we will need 3 API's
GET /:id - To get the Order by ID
POST / - To add Order Detail into Db.
DELETE /:id - To delete any Order by ID.
Here is our router file:
const express = require("express");
const { Orders } = require("../model");
const router = express.Router()
router.get("/", async ( req, res ) => {
const AllOrders = await Orders.findAll();
res.json({ data: AllOrders });
})
router.get("/:id", async ( req, res ) => {
const Order = await Orders.findByPk(req.params.id);
res.json({ data: Order });
})
router.post("/", async ( req, res ) => {
const { ProductId, UserId, Quantity, Cost } = req.body;
try {
const newOrder = await Orders.create({ ProductId, UserId, Quantity, Cost });
res.json({ data: newOrder });
} catch(error){
console.log("error", error);
res.send(400);
}
})
router.delete("/:id", async ( req, res ) => {
const { id } = req.params;
try {
await Orders.destroy({
where: {
id
}
});
res.sendStatus(204);
} catch(error){
console.log("error", error);
res.send(400);
}
})
module.exports = router;
Our Proof of concept Db schema will be like:
const { Model, DataTypes } = require('sequelize');
const sequelize = require("../db/config");
class Orders extends Model { };
Orders.init({
id: {
type: DataTypes.INTEGER,
primaryKey: true,
autoIncrement: true
},
ProductId: {
type: DataTypes.STRING,
allowNull: false,
},
UserId: {
type: DataTypes.STRING,
allowNull: false,
},
Quantity: {
type: DataTypes.INTEGER,
allowNull: false,
defaultValue: 0
},
Cost: {
type: DataTypes.INTEGER,
allowNull: false,
defaultValue: 0
},
}, {
sequelize,
timestamps: true,
modelName: 'Orders',
});
module.exports = Orders
and Db connection file :
const { Sequelize } = require("sequelize");
const db = new Sequelize({
username: 'myuser',
password: 'mypassword',
database: 'mydatabase',
host: process.env.DB_HOST || 'localhost',
port: process.env.DB_PORT || 5432,
dialect: 'postgres',
});
module.exports = db;
Just like Product SVC, we will pass the env variable from the Kubernetes Deployment.
Kubernetes Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: ordersvc-rs
spec:
replicas: 2
selector:
matchLabels:
app: ordersvc
template:
metadata:
labels:
app: ordersvc
spec:
containers:
- name: usercontainer
image: vanshk7/ordersvc
ports:
- containerPort: 3002
env:
- name: DB_HOST
value: order-postgresql-service # order Postgres DB service name
- name: DB_PORT
value: '5432'
If you notice, the DB_HOST in env is pointing to Order DB Service
Apply the deployment to create the Pod,
kubectl apply -f .\orderDeployment.yml
We will also create and run our order service as well. You can check the code here.
Let's try out our API.
Alrightyy! We are so ready with our Order service now.
We are done with 2 of our service's and now are all set to create our BFF microservice.
In the next blog where we will see how to design API's with inter microservice communication and how to ensure our API is consistent and able to rollback in case of failure.
Explore the code base for this blog here.
We will end our easy day here and will finish up our microservice in the next blog!
If you have any issues or looking forward to talk more on designing scalable backends feel free to ping me on Linkedin at Vansh Kapoor Linkedin.
For now take care and happy coding!👻
Read Further:
Part 3: creating BFF microservice Architecture using NodeJs and Kubernetes
Part 1: creating BFF microservice Architecture using NodeJs and Kubernetes
Subscribe to my newsletter
Read articles from vansh Kapoor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

vansh Kapoor
vansh Kapoor
Developing large scale application for multiple clients in Thoughtworks. Love to share my knowledge in React, Js and clean coding paractices and travel.