Is it a fair game: Numerical vs AI?
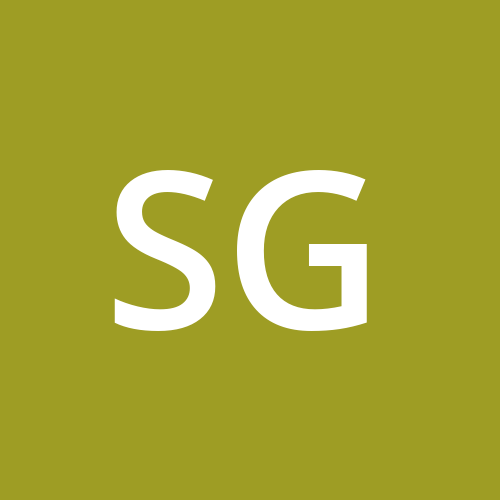
Table of contents
I was recently asked this question in an interview, which I enjoyed considering. I do not know if I could do justice to this, but here’s my attempt at answering it
Numerical methods and AI are valuable tools for solving complex problems but have different strengths and applications. Numerical methods are instrumental when dealing with mathematical models and equations, as they provide systematic and precise approaches to solving problems through various numerical techniques.
#code numerical
import numpy as np
from scipy.linalg import svd
import matplotlib.pyplot as plt
from skimage import data, img_as_float
from skimage.util import random_noise
# Load and add noise to an example image
image = img_as_float(data.camera())
noisy_image = random_noise(image, mode='gaussian', var=0.01)
# SVD Denoising function
def svd_denoise(image, rank):
U, S, Vt = svd(image, full_matrices=False)
S[rank:] = 0 # Zero out small singular values
denoised_image = np.dot(U, np.dot(np.diag(S), Vt))
return denoised_image
# Apply SVD denoising
denoised_image = svd_denoise(noisy_image, rank=50)
# Display the results
plt.figure(figsize=(10, 5))
plt.subplot(1, 3, 1)
plt.title('Original Image')
plt.imshow(image, cmap='gray')
plt.subplot(1, 3, 2)
plt.title('Noisy Image')
plt.imshow(noisy_image, cmap='gray')
plt.subplot(1, 3, 3)
plt.title('Denoised Image')
plt.imshow(denoised_image, cmap='gray')
plt.show()
On the other hand, AI excels in tasks that require pattern recognition, learning from data, and making decisions based on large amounts of information. By leveraging machine learning algorithms and neural networks, AI can analyze and recognize patterns in data that may not be easily solvable using traditional numerical methods.
#code ai (ChatGPT generated)
import numpy as np
import matplotlib.pyplot as plt
from scipy.signal import convolve2d
from skimage import data, img_as_float
from skimage.util import random_noise
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Conv2D, MaxPooling2D, UpSampling2D
# Load and add noise to an example image
image = img_as_float(data.camera())
noisy_image = random_noise(image, mode='gaussian', var=0.01)
# Prepare data for training
x_train = np.expand_dims(noisy_image, axis=0) # Single example, expand dims for batch size
x_train = np.expand_dims(x_train, axis=-1) # Expand dims for channels
# Define a simple CNN for denoising
input_img = Input(shape=(image.shape[0], image.shape[1], 1))
x = Conv2D(64, (3, 3), activation='relu', padding='same')(input_img)
x = MaxPooling2D((2, 2), padding='same')(x)
x = Conv2D(32, (3, 3), activation='relu', padding='same')(x)
encoded = MaxPooling2D((2, 2), padding='same')(x)
x = Conv2D(32, (3, 3), activation='relu', padding='same')(encoded)
x = UpSampling2D((2, 2))(x)
x = Conv2D(64, (3, 3), activation='relu', padding='same')(x)
x = UpSampling2D((2, 2))(x)
decoded = Conv2D(1, (3, 3), activation='sigmoid', padding='same')(x)
autoencoder = Model(input_img, decoded)
autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
# Train the model
autoencoder.fit(x_train, x_train, epochs=1000, batch_size=1, shuffle=True, verbose=1)
# Predict denoised image
denoised_image = autoencoder.predict(x_train)[0, :, :, 0]
# Display the results
plt.figure(figsize=(10, 5))
plt.subplot(1, 3, 1)
plt.title('Original Image')
plt.imshow(image, cmap='gray')
plt.subplot(1, 3, 2)
plt.title('Noisy Image')
plt.imshow(noisy_image, cmap='gray')
plt.subplot(1, 3, 3)
plt.title('Denoised Image')
plt.imshow(denoised_image, cmap='gray')
plt.show()
When comparing numerical methods and AI, time complexity is an important factor. Numerical methods often have well-defined time complexities, allowing for efficient analysis and optimization of algorithms, on the other hand, can have varying time complexities depending on the specific algorithms and models being used. This means that numerical methods may be more suitable for problems where time efficiency is crucial. At the same time, AI can handle tasks that require more complex pattern recognition and decision-making, even if it takes more time to process the data.
Regarding architecture, numerical methods typically use mathematical formulas and algorithms to solve problems. In contrast, AI utilizes neural networks and machine learning algorithms to learn from data and make predictions or decisions. Unlike traditional software, where the outcome is determined by a set of predefined rules and computations, AI systems rely on data-driven algorithms and neural networks to make inferences and predictions based on patterns and correlations in the data. In summary, numerical methods are primarily used to solve problems involving mathematical models and equations, providing systematic and precise approaches to obtain accurate solutions.
From a theoretic approach, numerical methods are based on theoretical concepts such as convergence, stability, and accuracy, which are crucial for ensuring the reliability and robustness of the solutions. In contrast, AI methods are more empirical, relying on data-driven approaches to learn patterns and make decisions. While numerical methods have been extensively studied and well-established, AI is a rapidly evolving field that constantly evolves new advancements and techniques. For example, consider the problem of solving a system of linear equations.
However, it is important to note that numerical methods and AI are not mutually exclusive. There may be cases where a combination of both numerical methods and AI can provide the most effective solution to a problem.
For example, numerical methods can be used in financial modelling to calculate accurate valuations and pricing models based on mathematical equations. AI can be utilized to analyze large data sets and identify patterns that may influence financial markets, leading to better forecasting and decision-making. In all honesty, this is just the tip of the iceberg waiting to be solved. Are you ready for it?
Subscribe to my newsletter
Read articles from Sreya Ghosh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
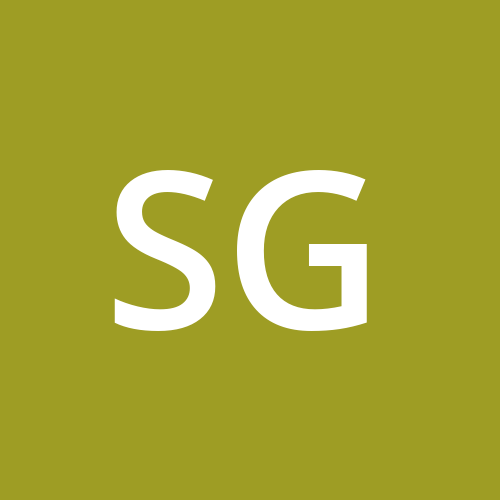