Key React Hooks Explained: useState, useEffect, and useContext
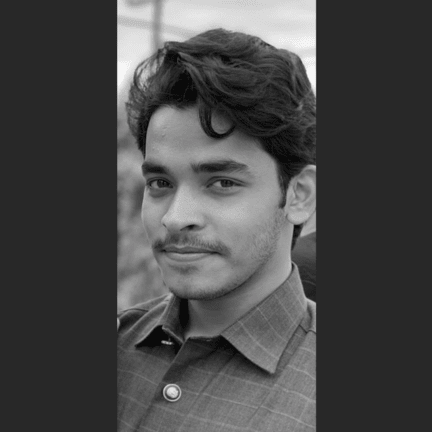
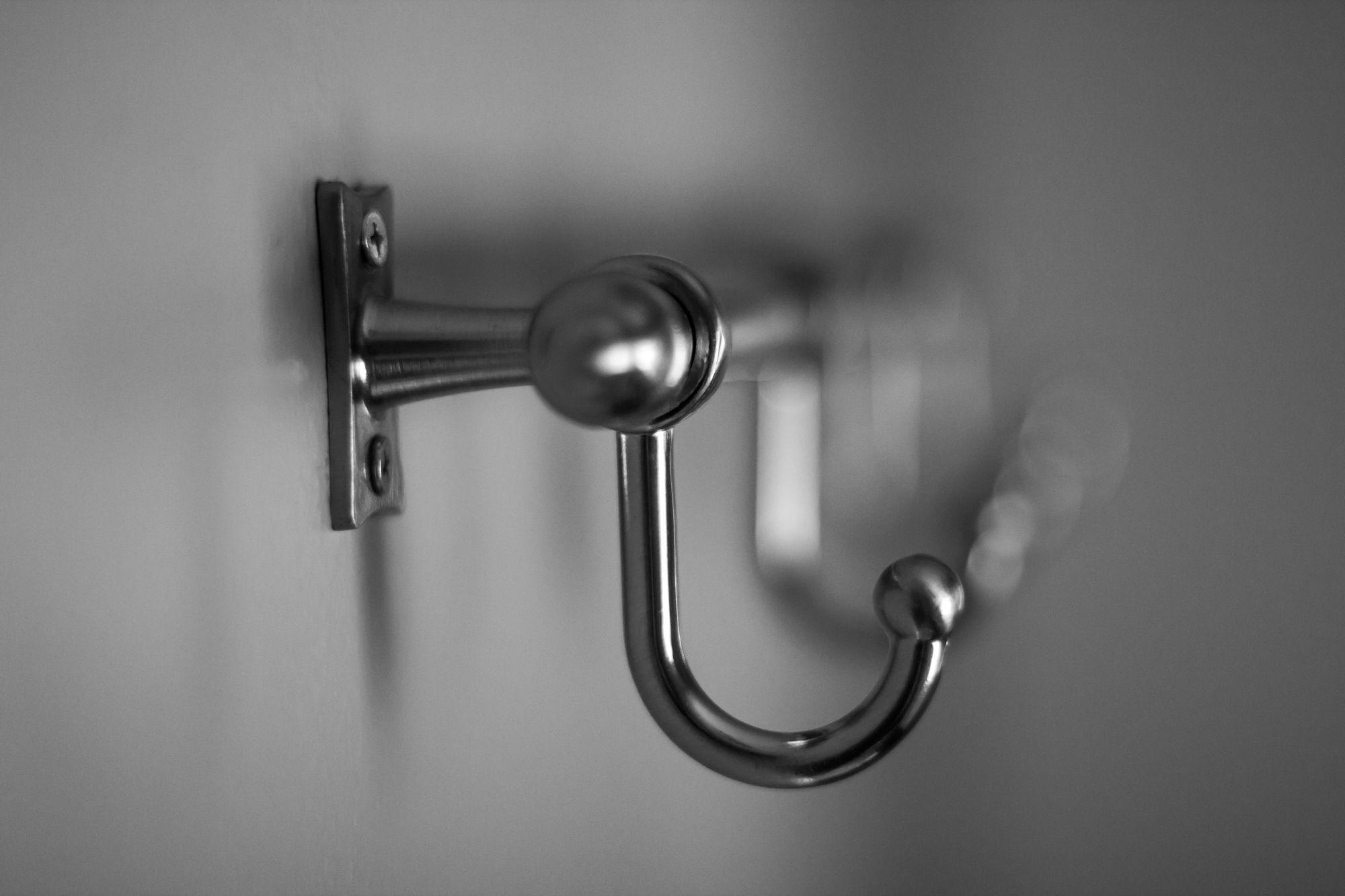
useState()
The purpose of useState
is to handle reactive data. Any data that changes in the application is called state. When the state changes you want react to update the UI, so the latest changes are reflected to the end user.
How to use it?
const [count, setCount] = useState(0)
useState()
returns an array with 2 values:
Current state
In our example, count is the state variable which is set to 0. This initial state can be a number, boolean, string, or object
Set function to update the state.
You can update the state in two ways:
i. Directly passing the next state
setCount(2)
// Now, the updated state ofcount will be 2.
ii. Using function
setCount( prevCount => prevCount + 1)
In this function, we check the current state which is 2. The value ofprevCount
will be 2, thenwe increment it by 1.
Docs:https://react.dev/reference/react/useState#usage
useEffect()
useEffect
is a Hook in React that allows you to synchronise a component with an external system. It is a way to handle side effects, such as fetching data or subscribing to a event in a functional component.
How to use it?
useEffect(() => {
console.log('count changed')
},[count])
Here, the `count changed ` will be logged every time the count changes.
useEffect
takes two arguments:
A callback function
The callback function is called after the component has rendered.
A list of dependencies
The list of dependencies is used to determine when to re-run the effect, by comparing the current values of the dependencies to the previous values.
In the callback function, you can perform any side effects, such as fetching data or subscribing to an event. You can also return a cleanup function, which is called before the component is unmounted or the effect is re-run.
Note:
If you don’t pass dependencies the effect will re-run on every render of component.
When state variables are used in useEffect , make sure to include in dependency array.
Make sure to handle side effects, with a cleanup function.
Docs:https://react.dev/reference/react/useEffect#reference
useContext()
useContext
is a way to manage state globally without passing props down through multiple levels of the component tree.
The Problem
Prop Drilling: Prop drilling refers to the process of passing down props through multiple layers of components, even when some of those components do not directly use the props. We can solve this problem using
useContext()
How to use it?
Three things to understand:
Creating context
const EmailContext = React.createContext(null);
Provide a value for the context using ContextName.Provider
function App() { const value = { email: "in.mohsin@outlook.com" }; return ( <EmailContext.Provider value={value}> <Child /> </EmailContext.Provider> ); }
Use
useContext()
in the component that needs to consume the contextfunction Child() { const context = useContext(EmailContext); return <div>The email is: {context.email}</div>; }
When the context value is updated by the provider, the component consuming the context re-renders automatically. This allows the component to always have the latest data.
Subscribe to my newsletter
Read articles from Mohsin Khansab directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
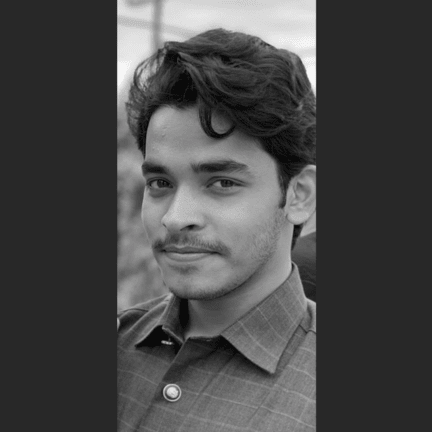