How to Use MongoDB Aggregate Functions: Step-by-Step Examples
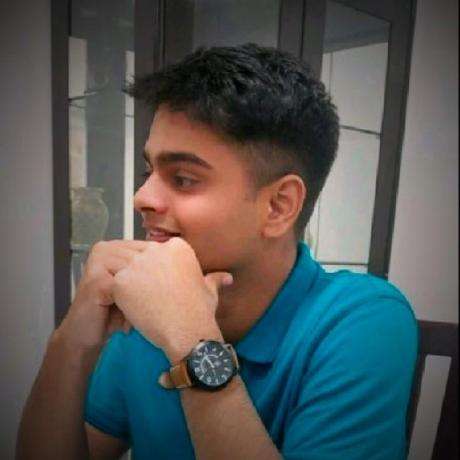
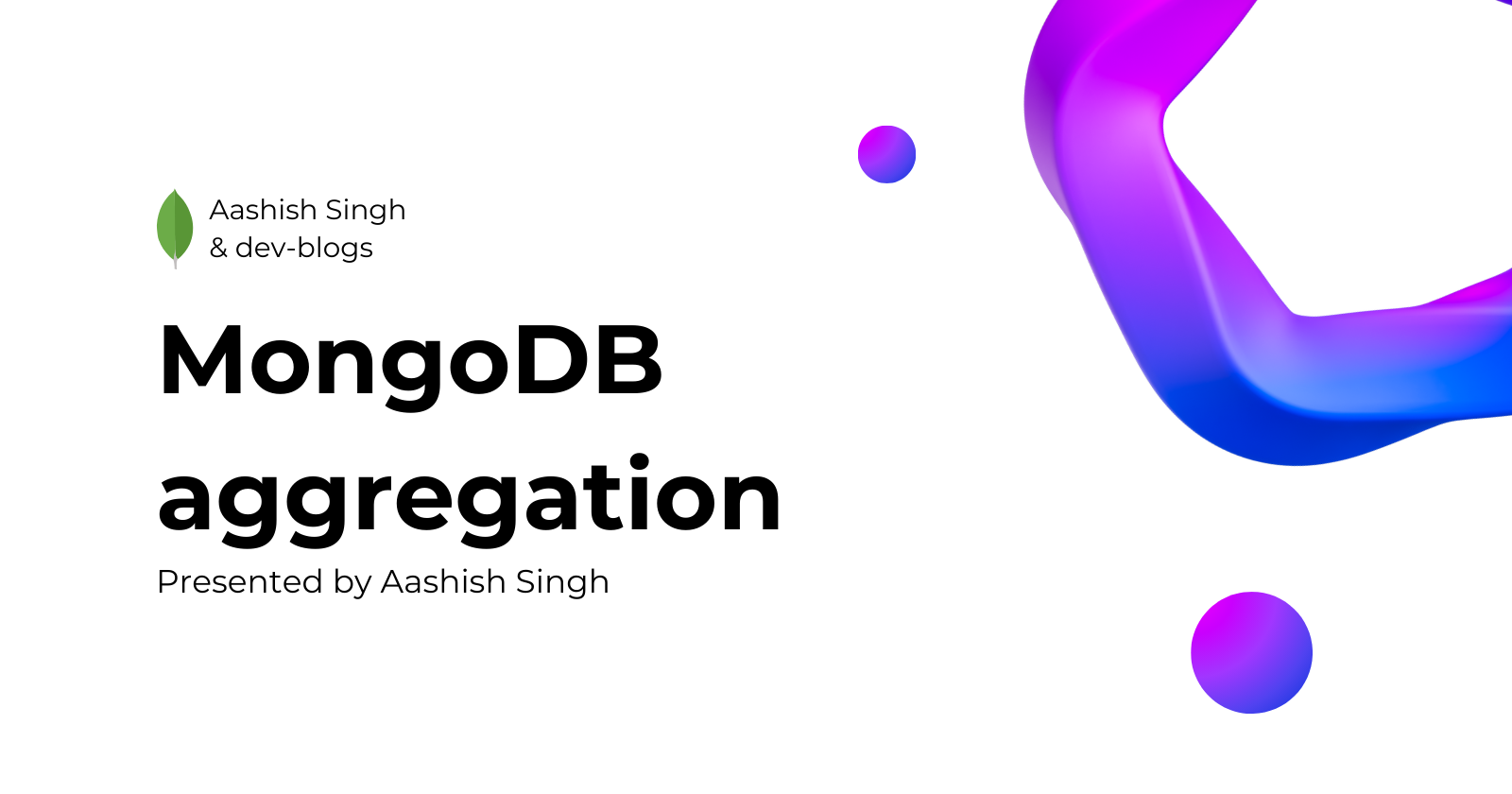
Introduction
MongoDB, a leading NoSQL database, provides powerful tools for aggregating and transforming data through its aggregation framework. Aggregate functions are crucial for performing operations like filtering, grouping, sorting, and reshaping data. In this blog, we'll explore the most commonly used aggregate functions in MongoDB, with practical examples to help you understand their usage.
1. $match
The $match
stage filters documents that meet specific criteria. It's similar to the find
query in MongoDB.
Example:
Suppose we have a sales
collection with documents like:
{ "item": "book", "quantity": 120, "price": 10 }
{ "item": "pen", "quantity": 80, "price": 2 }
{ "item": "notebook", "quantity": 200, "price": 5 }
To filter sales where the quantity is greater than 100:
db.sales.aggregate([
{ $match: { quantity: { $gt: 100 } } }
])
2. $group
The $group
stage groups documents by a specified identifier and can calculate aggregate values like sum, average, and count.
Example:
To group sales by item
and calculate the total quantity sold for each:
db.sales.aggregate([
{ $group: { _id: "$item", totalQuantity: { $sum: "$quantity" } } }
])
3. $project
The $project
stage reshapes documents, including or excluding fields and adding computed values.
Example:
To include only item
and totalPrice
(calculated as quantity * price
):
db.sales.aggregate([
{ $project: { item: 1, totalPrice: { $multiply: ["$quantity", "$price"] } } }
])
4. $sort
The $sort
stage orders documents based on specified fields.
Example:
To sort sales by quantity
in descending order:
db.sales.aggregate([
{ $sort: { quantity: -1 } }
])
5. $limit
The $limit
stage restricts the number of documents passed to the next stage.
Example:
To limit the results to the top 2 documents:
db.sales.aggregate([
{ $limit: 2 }
])
6. $skip
The $skip
stage skips a specified number of documents before passing the rest to the next stage.
Example:
To skip the first document and return the rest:
db.sales.aggregate([
{ $skip: 1 }
])
7. $unwind
The $unwind
stage deconstructs an array field from documents and outputs one document for each element of the array.
Example:
Suppose we have a sales
document with an array of tags
:
{ "item": "book", "tags": ["education", "reading"] }
To deconstruct the tags
array:
db.sales.aggregate([
{ $unwind: "$tags" }
])
8. $lookup
The $lookup
stage performs a left outer join to another collection in the same database.
Example:
Suppose we have an orders
collection and a customers
collection:
orders
:
{ "orderId": 1, "customerId": 123, "amount": 50 }
customers
:
{ "customerId": 123, "name": "John Doe" }
To join orders
with customers
on customerId
:
db.orders.aggregate([
{
$lookup: {
from: "customers",
localField: "customerId",
foreignField: "customerId",
as: "customerInfo"
}
}
])
9. $count
The $count
stage counts the number of documents that pass through it.
Example:
To count the number of sales documents:
db.sales.aggregate([
{ $count: "totalSales" }
])
10. $out
The $out
stage writes the resulting documents of the aggregation pipeline to a new collection.
Example:
To write the results to a new collection named highQuantitySales
:
db.sales.aggregate([
{ $match: { quantity: { $gt: 100 } } },
{ $out: "highQuantitySales" }
])
Conclusion
MongoDB's aggregation framework is a powerful tool for data manipulation and analysis. Understanding and effectively using aggregate functions like $match
, $group
, $project
, $sort
, $limit
, $skip
, $unwind
, $lookup
, $count
, and $out
can significantly enhance your ability to work with MongoDB data. Experiment with these functions to gain deeper insights and harness the full potential of MongoDB in your applications.
Subscribe to my newsletter
Read articles from Aashish Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
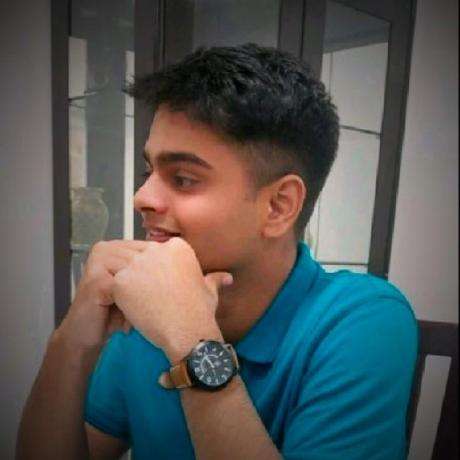
Aashish Singh
Aashish Singh
Hey there! I'm Aashish Singh, a B.Tech CSE student from Manipal passionate about all things tech. As a full-stack developer, I love diving into projects' front and back ends. My interests span the exciting realms of AI/ML and cybersecurity, always keeping me on my toes and eager to learn more. Feel free to connect with me and let's explore the tech world together! Reach out using the links given. ๐