The Importance of Python in Data Quality Automation: A Comprehensive Guide
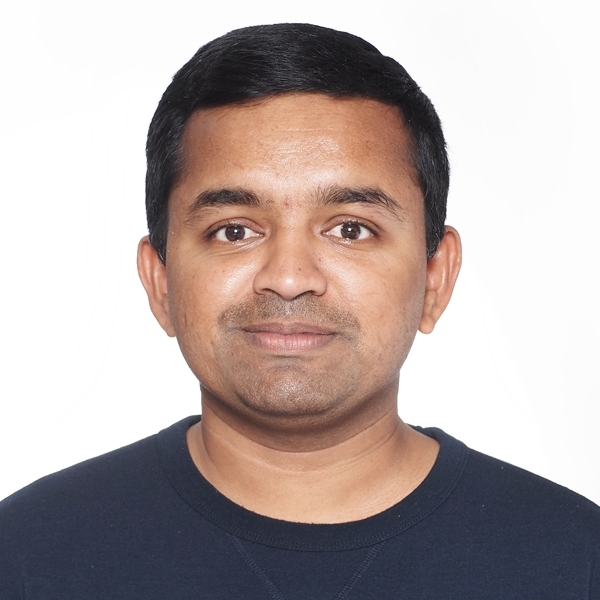

The Importance of Python in Data Quality Automation: A Comprehensive Guide
In the realm of data quality automation, ensuring that data is accurate, consistent, and reliable is paramount. As a Data Quality Test Automation Engineer, mastering Python can significantly enhance your ability to automate data quality processes, thus saving time, reducing errors, and improving overall data integrity. This blog aims to highlight the importance of Python in data quality automation and provide a comprehensive guide to mastering the language for this purpose.
Why Python?
Python has emerged as a leading language in data quality automation for several reasons:
Ease of Learning and Use: Python's simple and readable syntax makes it accessible for beginners while being powerful enough for experts.
Extensive Libraries and Frameworks: Python boasts a rich ecosystem of libraries and frameworks such as Pandas, NumPy, and Pytest, which are invaluable for data manipulation and testing.
Community Support: Python has a large and active community, providing a wealth of resources, tutorials, and forums for troubleshooting and learning.
Integration Capabilities: Python can easily integrate with various databases, data processing tools, and other programming languages, making it highly versatile.
Key Python Libraries for Data Quality Automation
Pandas: Essential for data manipulation and analysis, Pandas provides data structures and functions needed to work with structured data.
NumPy: Offers support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
Pytest: A robust framework for writing simple and scalable test cases.
Great Expectations: An open-source library for validating, documenting, and profiling your data.
Getting Started with Python for Data Quality Automation
1. Setting Up Your Environment
To begin, you'll need to set up your Python environment. This includes installing Python, setting up a virtual environment, and installing necessary libraries.
# Install Python
sudo apt-get install python3
# Install pip, the Python package installer
sudo apt-get install python3-pip
# Create a virtual environment
python3 -m venv dq_venv
# Activate the virtual environment
source dq_venv/bin/activate
# Install necessary libraries
pip install pandas numpy pytest great_expectations
2. Data Manipulation with Pandas
Pandas is a cornerstone library for data quality automation. Here’s a basic example of how to load, inspect, and clean a dataset using Pandas.
import pandas as pd
# Load a dataset
df = pd.read_csv('data.csv')
# Inspect the first few rows
print(df.head())
# Check for missing values
print(df.isnull().sum())
# Drop rows with missing values
df_cleaned = df.dropna()
# Save the cleaned dataset
df_cleaned.to_csv('data_cleaned.csv', index=False)
3. Array Operations with NumPy
NumPy is invaluable for handling large datasets and performing complex mathematical operations.
import numpy as np
# Create a NumPy array
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Perform element-wise operations
data = data * 2
print(data)
4. Writing Tests with Pytest
Pytest allows you to write simple yet powerful test cases to ensure your data meets the required quality standards.
import pytest
def test_data_quality():
df = pd.read_csv('data_cleaned.csv')
# Check if all values in a specific column are non-negative
assert (df['column_name'] >= 0).all()
# Run the tests
if __name__ == "__main__":
pytest.main()
5. Validating Data with Great Expectations
Great Expectations is an excellent tool for setting and enforcing data quality expectations.
from great_expectations.dataset import PandasDataset
# Load your dataset
df = pd.read_csv('data_cleaned.csv')
dataset = PandasDataset(df)
# Define expectations
dataset.expect_column_values_to_be_between('column_name', min_value=0, max_value=100)
dataset.expect_column_to_exist('another_column')
# Validate the dataset
results = dataset.validate()
print(results)
Best Practices for Data Quality Automation
Modular Code: Write reusable and modular code to handle different data quality checks.
Automated Testing: Integrate automated tests into your CI/CD pipeline to ensure continuous data quality.
Documentation: Document your code and data quality checks for future reference and reproducibility.
Regular Audits: Conduct regular audits of your data and automation processes to identify and rectify issues promptly.
Subscribe to my newsletter
Read articles from Vipin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
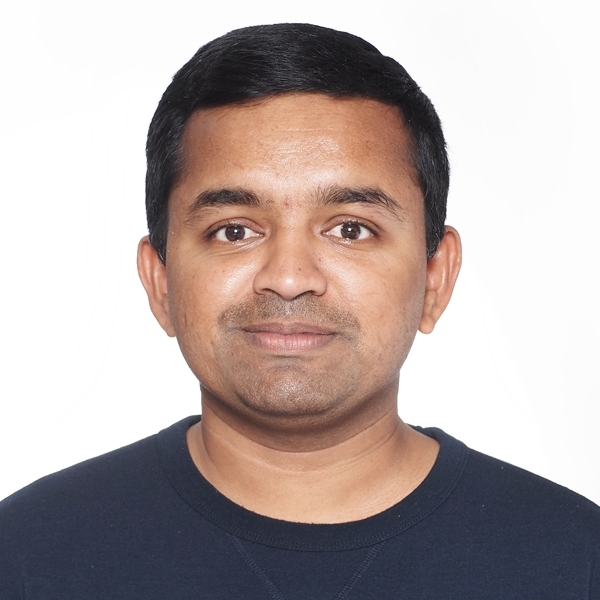
Vipin
Vipin
Highly skilled Data Test Automation professional with over 10 years of experience in data quality assurance and software testing. Proven ability to design, execute, and automate testing across the entire SDLC (Software Development Life Cycle) utilizing Agile and Waterfall methodologies. Expertise in End-to-End DWBI project testing and experience working in GCP, AWS, and Azure cloud environments. Proficient in SQL and Python scripting for data test automation.