Day 7: Mastering CSS Flexbox and Positioning
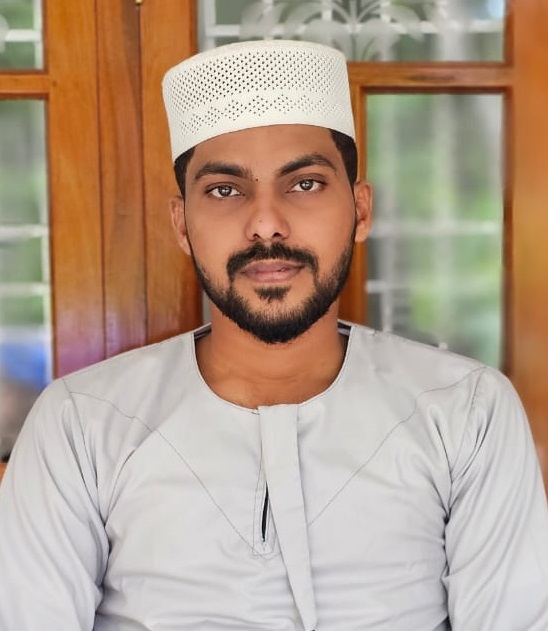
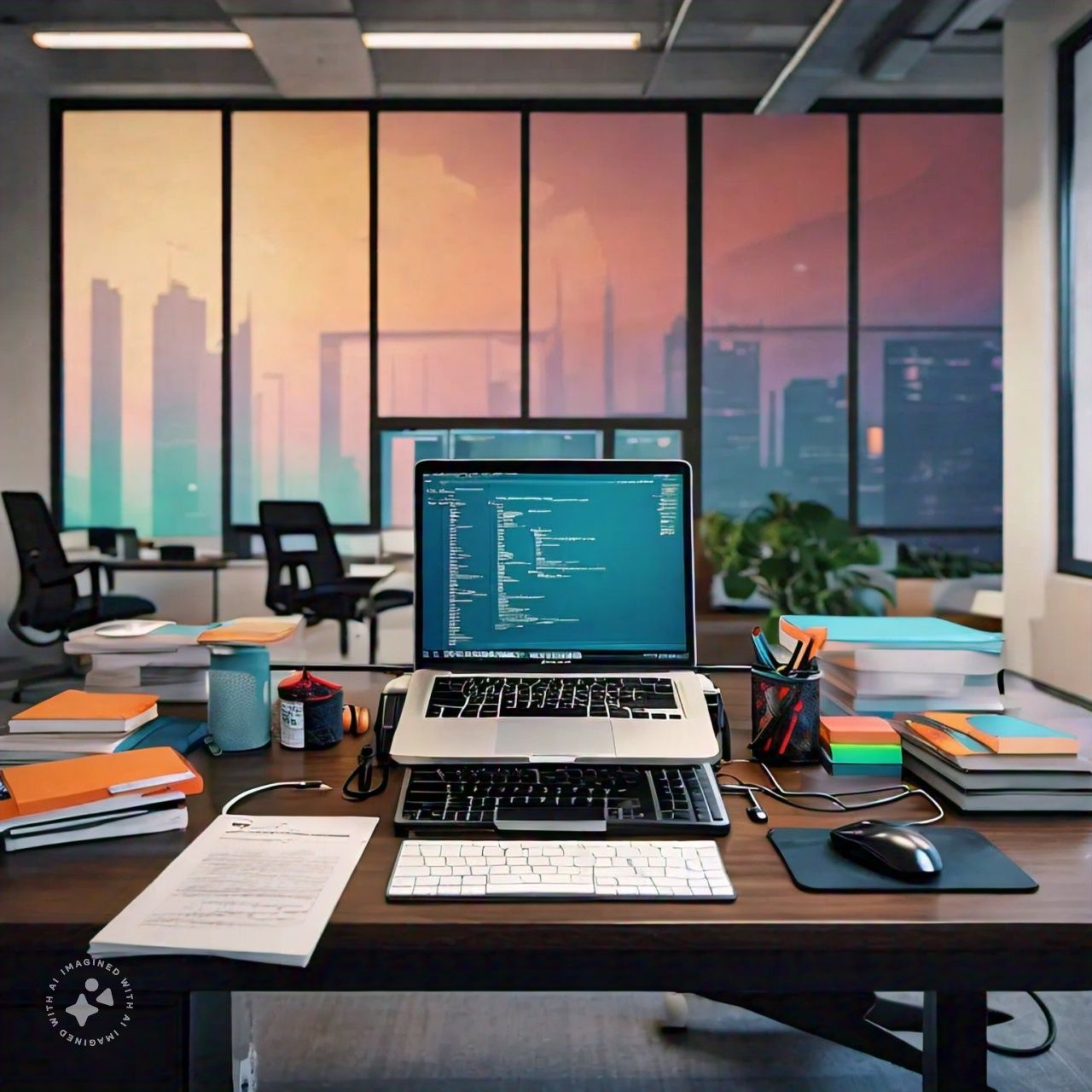
Welcome back to my frontend development journey! Today marks my seventh day, and it's all about diving deep into CSS Flexbox and positioning. These concepts are fundamental for creating responsive and well-structured web layouts, and I'm excited to share what I've learned.
Understanding CSS Flexbox
Flexbox is a powerful layout module that provides an efficient way to arrange items in a container. Unlike traditional layout methods like float and inline-block, Flexbox allows for greater control over the alignment, direction, and spacing of elements.
Key Concepts of Flexbox:
Flex Container and Flex Items:
A flex container is defined using
display: flex
ordisplay: inline-flex
.The child elements inside the flex container are called flex items.
Main Axis and Cross Axis:
The main axis is the primary axis along which flex items are placed. It can be horizontal or vertical.
The cross axis is perpendicular to the main axis.
Flex Properties:
flex-direction
: Defines the direction of the main axis (row, row-reverse, column, column-reverse).justify-content
: Aligns flex items along the main axis (flex-start, flex-end, center, space-between, space-around).align-items
: Aligns flex items along the cross axis (flex-start, flex-end, center, baseline, stretch).align-self
: Overrides the align-items value for individual flex items.flex-wrap
: Controls whether flex items should wrap onto multiple lines (nowrap, wrap, wrap-reverse).
Example: Basic Flexbox Layout
htmlCopy code<div class="flex-container">
<div class="flex-item">1</div>
<div class="flex-item">2</div>
<div class="flex-item">3</div>
</div>
<style>
.flex-container {
display: flex;
justify-content: space-around;
align-items: center;
height: 100vh;
}
.flex-item {
background-color: lightcoral;
padding: 20px;
margin: 10px;
color: white;
}
</style>
In this example, the .flex-container
is set to display: flex
, making its child elements flex items. The justify-content: space-around
distributes the flex items evenly with space around them, and align-items: center
centers them vertically.
Positioning in CSS
Positioning is another crucial concept for laying out elements on a webpage. CSS offers several positioning schemes:
Static Positioning:
The default positioning for all elements.
Elements are positioned according to the normal document flow.
Relative Positioning:
Positioned relative to its normal position.
top
,right
,bottom
, andleft
properties can adjust the element's position without affecting the surrounding elements.
Absolute Positioning:
Positioned relative to the nearest positioned ancestor (not static).
Removes the element from the normal document flow, allowing it to overlap other elements.
Fixed Positioning:
Positioned relative to the viewport, meaning it stays in place even when the page is scrolled.
Useful for creating sticky headers or footers.
Sticky Positioning:
A hybrid of relative and fixed positioning.
The element is treated as relative until it crosses a specified threshold, then it behaves as fixed.
Example: Absolute Positioning
htmlCopy code<div class="container">
<div class="box">I'm positioned absolutely!</div>
</div>
<style>
.container {
position: relative;
width: 100%;
height: 200px;
background-color: lightblue;
}
.box {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: darkblue;
color: white;
padding: 20px;
}
</style>
In this example, the .box
is positioned absolutely within the .container
. By using top: 50%
and left: 50%
, and then applying transform: translate(-50%, -50%)
, the box is perfectly centered within its container.
Conclusion
Today's exploration of CSS Flexbox and positioning has been incredibly enlightening. These tools are indispensable for any frontend developer, enabling us to create responsive, flexible, and well-structured layouts. As I continue this journey, I'm looking forward to implementing these concepts in real projects and refining my skills further.
Stay tuned for more updates as I delve deeper into the world of frontend development!
Happy coding!
Subscribe to my newsletter
Read articles from Ashfak Pr directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
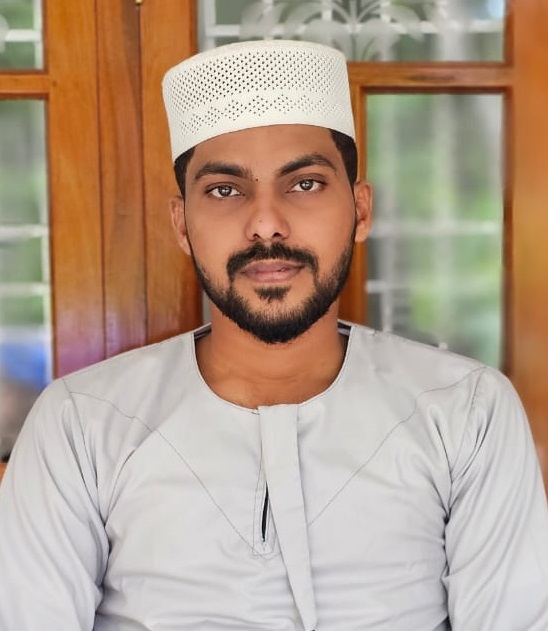