Advanced State Management in React: Redux Toolkit vs. Recoil vs. Jotai
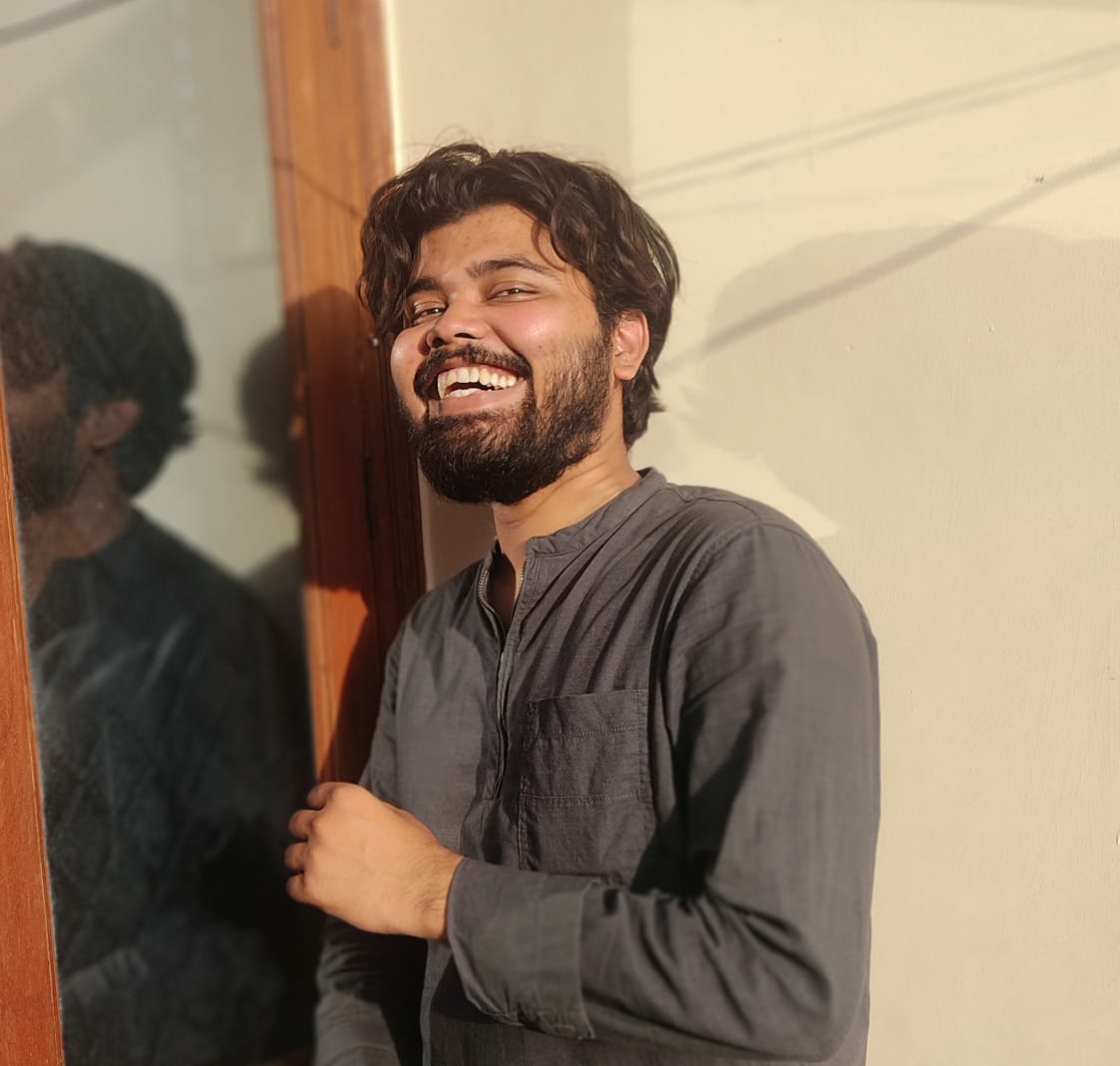
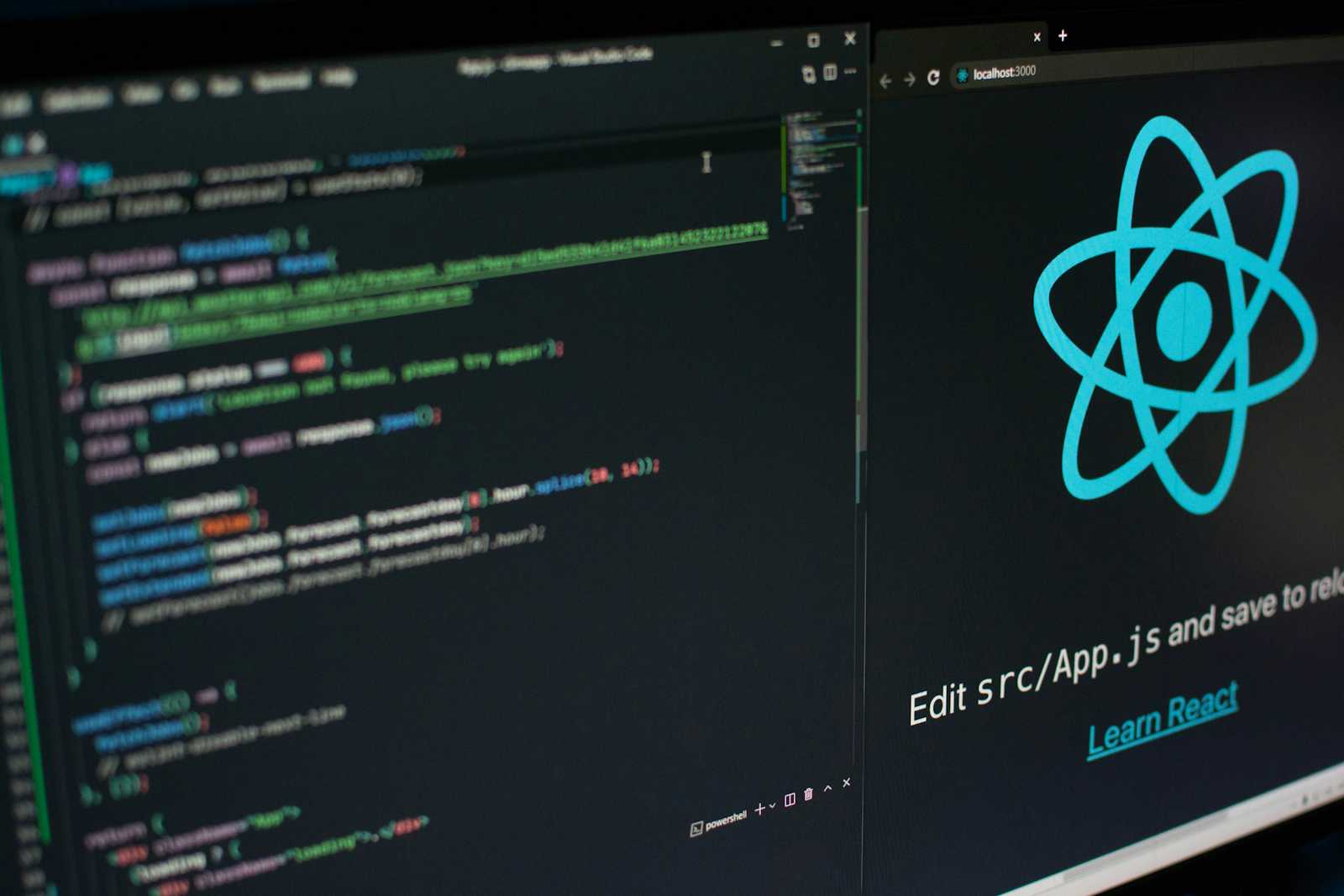
As React applications grow in complexity, managing state becomes increasingly challenging. While React's built-in useState and useContext hooks are sufficient for simpler applications, larger projects often require more robust state management solutions. In this post, we'll dive deep into three popular state management libraries: Redux Toolkit, Recoil, and Jotai, comparing their approaches, use cases, and performance implications.
Redux Toolkit: The Evolution of a Classic
Redux has long been the go-to solution for state management in React. Redux Toolkit, introduced to simplify the Redux workflow, addresses many of the criticisms of vanilla Redux.
Key Features:
Simplified store setup with configureStore
Reduced boilerplate with createSlice
Immutable update logic with Immer
Async action creation with createAsyncThunk
Redux Toolkit shines in large applications with complex state interactions. Its centralized store approach ensures predictable state updates and makes debugging easier, especially when combined with the Redux DevTools.
import { createSlice, configureStore } from '@reduxjs/toolkit'
const counterSlice = createSlice({
name: 'counter',
initialState: { value: 0 },
reducers: {
increment: state => { state.value += 1 },
decrement: state => { state.value -= 1 }
}
})
const store = configureStore({
reducer: counterSlice.reducer
})
However, Redux Toolkit still requires a fair amount of setup and can be overkill for smaller applications.
Recoil: Facebook's Atomic Approach
Developed by Facebook, Recoil introduces a novel approach to state management with its concept of atoms and selectors.
Key Features:
Atom-based state management
Derived state with selectors
Easy integration with React Suspense
Built-in support for asynchronous state
Recoil's atom-based approach allows for more granular state management, potentially improving performance by minimizing re-renders.
import { atom, useRecoilState } from 'recoil'
const counterState = atom({
key: 'counterState',
default: 0,
})
function Counter() {
const [count, setCount] = useRecoilState(counterState)
return (
<button onClick={() => setCount(count + 1)}>
Count: {count}
</button>
)
}
Recoil is particularly useful for applications with complex, interdependent state relationships, and its integration with React Suspense makes it a strong choice for applications with asynchronous data requirements.
Jotai: Primitive and Flexible
Jotai, inspired by Recoil, aims to provide a simpler, more flexible approach to atomic state management.
Key Features:
Minimalistic API
No string keys required
Easy derivations
First-class TypeScript support
Jotai's simplicity makes it an excellent choice for projects of any size, offering an easy learning curve and high flexibility.
import { atom, useAtom } from 'jotai'
const counterAtom = atom(0)
function Counter() {
const [count, setCount] = useAtom(counterAtom)
return (
<button onClick={() => setCount(c => c + 1)}>
Count: {count}
</button>
)
}
Jotai's lightweight nature and lack of boilerplate make it particularly appealing for smaller to medium-sized applications, or as a complement to existing state management solutions in larger projects.
Choosing the Right Tool
The choice between Redux Toolkit, Recoil, and Jotai depends on your project's specific needs:
Redux Toolkit is ideal for large, complex applications with many state interactions and a need for robust debugging tools.
Recoil is well-suited for applications with complex state dependencies and those leveraging React Suspense for data fetching.
Jotai offers a simple, flexible solution that can work well in projects of any size, particularly those prioritizing simplicity and quick setup.
Subscribe to my newsletter
Read articles from Tejas Jaiswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
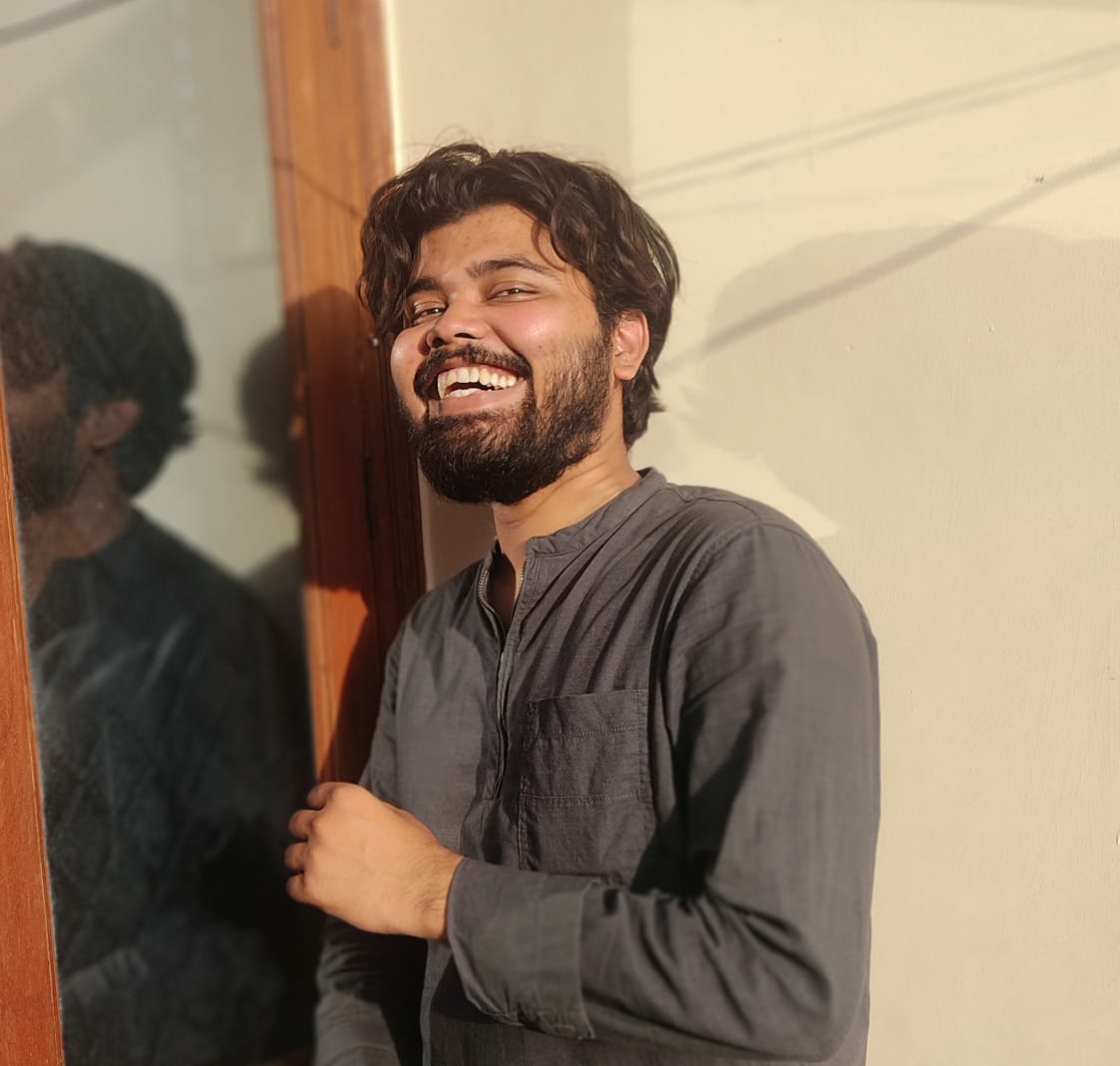
Tejas Jaiswal
Tejas Jaiswal
As a full-stack developer, I enjoy creating visually appealing and responsive websites using the MERN stack, SQL database administration, and HTTP. I have one year of experience, where I worked on various web development projects and learning new technologies and frameworks. I also completed a two-month contract as a full-stack developer at Eviox Technology Pvt Ltd, where I developed and deployed a dynamic e-commerce website for a client. In addition to web development, I am passionate about artificial intelligence and its applications. I am currently pursuing a Bachelor of Technology degree in Electronics and Communications Engineering from JSS Academy of Technical Education, Noida, where I have taken courses on machine learning, computer vision, and natural language processing. I am also a freelance video editor with one year of experience, using software such as Adobe Premiere Pro and DaVinci Resolve to create captivating visual stories. My goal is to combine my web development and AI skills to create innovative and impactful solutions for real-world problems.