Dive Deep into JavaScript Console: A Comprehensive Guide for Beginners

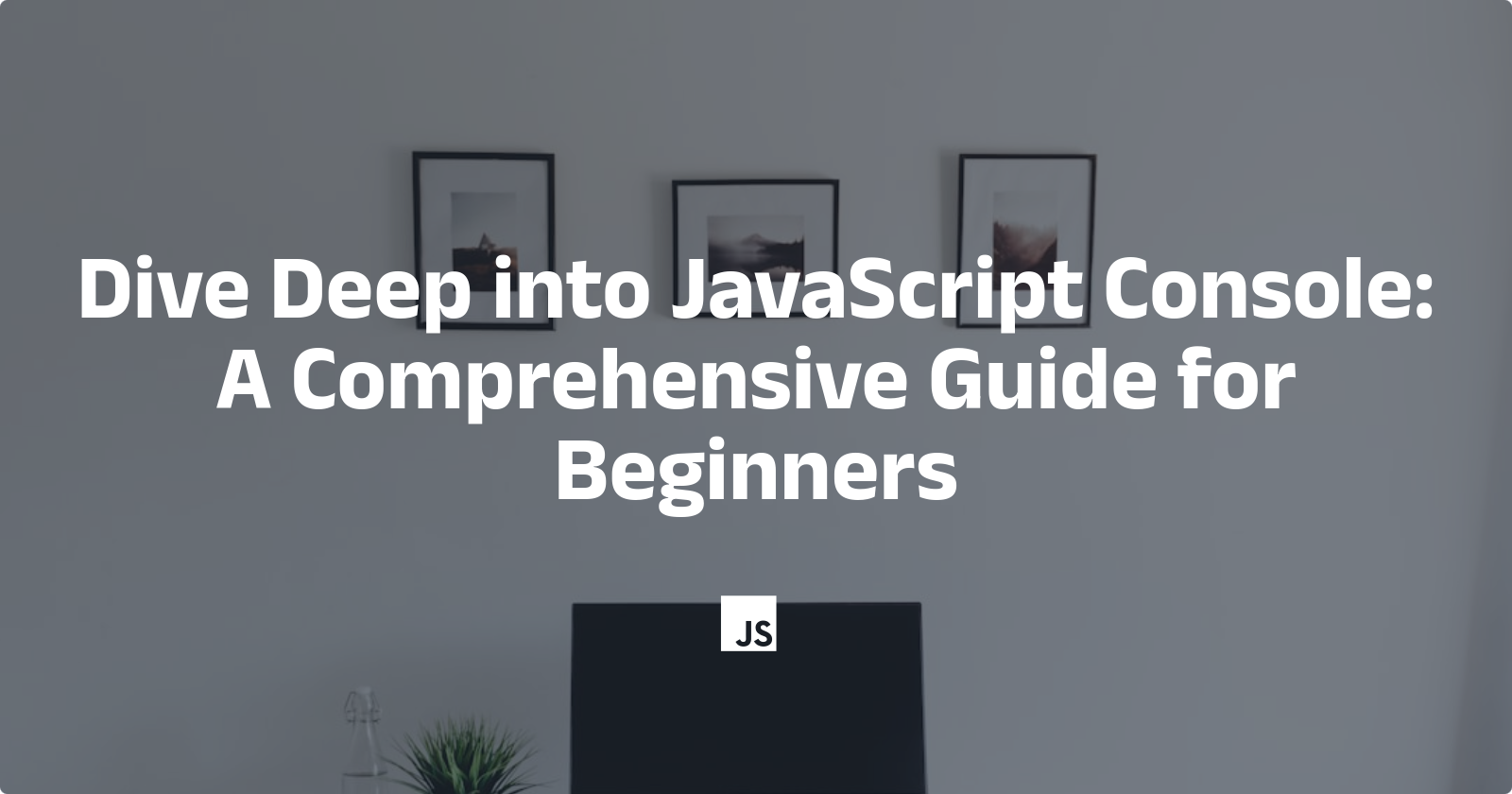
In web development, being great at finding and fixing problems in your code is like having a superpower. One of the most important tools for this is the JavaScript console
. Whether you've been coding for years or are just starting out, learning how to use the console can greatly improve how quickly and effectively you build websites and web apps.
Let's dive into everything you need to know about the JavaScript console, starting with the basics and moving on to more advanced topics.
What is the Console?
The console is a built-in tool provided by web browsers that allows developers to interact with their JavaScript code. It serves as a window into the execution of your code, providing valuable insights, debugging capabilities, and a means of communication between your code and the developer.
Getting Started
To open the console in most web browsers, simply right-click anywhere on a web page, select “Inspect” or “Inspect Element,” and navigate to the “Console” tab. Alternatively, you can use keyboard shortcuts like Ctrl + Shift + J
(Windows/Linux) or Cmd + Option + J
(Mac) to open the console directly.
Once the console is open, you can start executing JavaScript commands and accessing various features it offers.
Basic Console Methods
The console object in JavaScript provides several methods for logging messages, debugging code, and profiling performance. Here are some of the most commonly used methods:
console.log()
: Logs messages to the console.console.error()
: Logs error messages to the console.console.warn()
: Logs warning messages to the console.console.info()
: Logs informational messages to the console.console.table()
: Displays tabular data in a table format.console.group()
andconsole.groupEnd()
: Groups related messages together.console.clear()
: Clears the console.
console.log("Hello, world!");
console.error("An error occurred.");
console.warn("Warning: This action is irreversible.");
console.info("Information: Your session will expire in 5 minutes.");
const data = [
{ firstName: "John",lastName:"Doe", age: 30 },
{ firstName: "Jane",lastName:"Doe", age: 25 }
];
console.table(data);
console.group("Grouping example");
console.log("Message 1");
console.log("Message 2");
console.groupEnd();
console.clear();
Advanced Console Techniques
Beyond basic logging, the console offers several advanced features to aid in debugging and performance optimization:
- Console Interpolation: You can include dynamic values within log messages using string interpolation.
const name = "Alice";
console.log(`Hello, ${name}!`);
- Timing: You can measure the execution time of code using
console.time()
andconsole.timeEnd()
.
console.time("timer");
// Some time-consuming operation
console.timeEnd("timer");
- Assertion: You can use
console.assert()
to assert whether a condition is true and log a message if it's false.
const x = 10;
console.assert(x === 5, "Error: x is not equal to 5");
- Stack Tracing: You can trace the call stack using
console.trace()
to see the path that led to the current execution point.
function foo() {
bar();
}
function bar() {
console.trace("Trace:");
}
foo();
Browser Compatibility
While the console is a powerful tool, it's essential to be aware of differences in implementation and features across different web browsers. Always test your code in multiple browsers to ensure compatibility.
Conclusion
Mastering the console in JavaScript is a crucial skill for any web developer. By understanding its capabilities and leveraging its features effectively, you can streamline your debugging process, optimize performance, and gain deeper insights into your code's behavior. Whether you're logging messages, profiling performance, or tracing execution paths, the console is your faithful companion on the journey to JavaScript mastery. So next time you encounter a bug or want to analyze your code's performance, remember to turn to the console for assistance, it's your trusty sidekick in the world of web development.
Please feel free to provide feedback 😊. I will try my best to generate such blog in future as well 🤗.
Happy Coding!!
Subscribe to my newsletter
Read articles from Sailesh Shrestha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
