Input Validation using NodeJS like a Pro
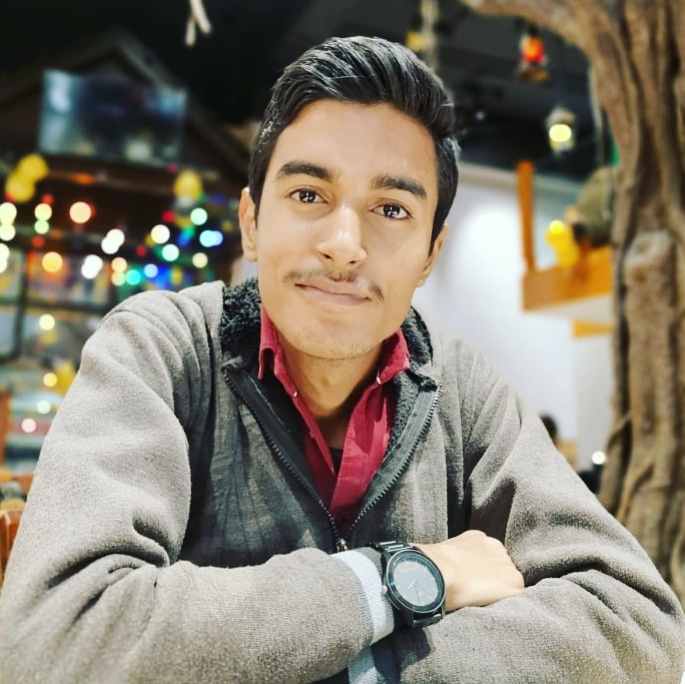
Table of contents
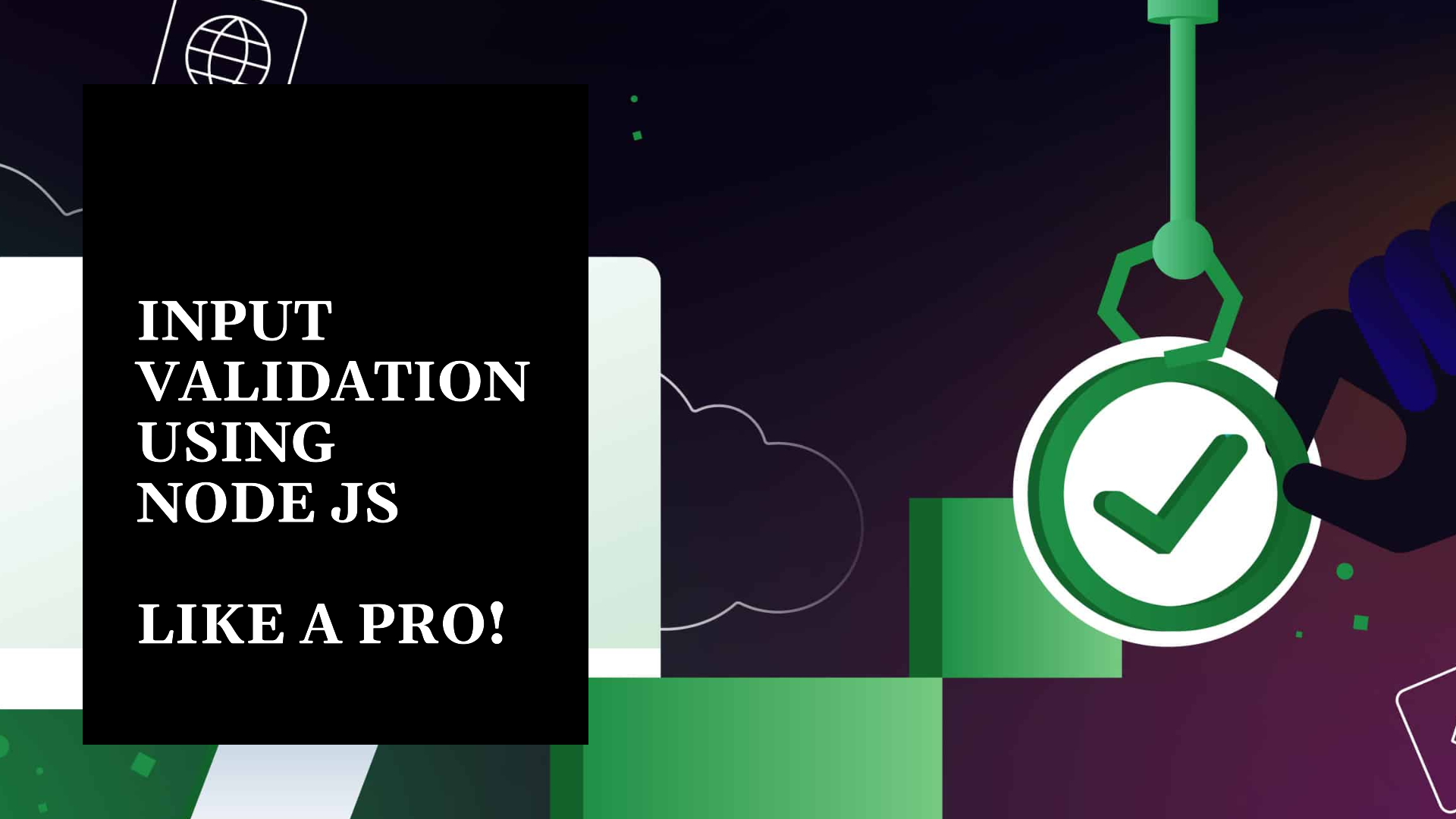
In Node.js applications, especially those handling user input, input validation is paramount. It safeguards your server from malicious attacks and ensures data consistency. This post delves into implementing input validation using the popular express-validator
library.
What is Express-Validator?
Express-validator is a widely used middleware for Express.js that provides a robust set of validation rules and sanitization methods. It seamlessly integrates with Express, allowing you to define validation logic for request parameters (body, query string, params) with ease.
Installation
Begin by installing express-validator
using npm or yarn:
npm install express-validator
Let's take an example of an express app for validating username and email for registering an user.
1. Setting Up the Environment:
const express = require('express');
const { body, validationResult } = require('express-validator');
const app = express();
app.use(express.json());
Imports:
- We import the necessary modules:
express
for creating the Express application andbody
andvalidationResult
fromexpress-validator
for defining validation rules and handling validation results.
- We import the necessary modules:
Express App:
- We create an Express application instance using
express()
.
- We create an Express application instance using
Middleware:
- We use
app.use(express.json())
to parse incoming request bodies in JSON format. This ensures that the data sent by the user in the registration form is properly parsed before validation.
- We use
2. Defining the Registration Route:
app.post('/register', [
body('username')
.trim()
.notEmpty()
.withMessage('Username is required'),
body('email')
.isEmail()
.withMessage('Please enter a valid email'),
], (req, res) => {
// ... rest of the code ...
});
Route Definition:
- We define a POST route handler for
/register
usingapp.post
('/register', ...)
. This route will be triggered when a user submits a registration form with a POST request to the/r
egister
endpoint.
- We define a POST route handler for
-
- The route handler is wrapped in an array containing validation rules for the
username
andemail
fields. This ensures that the data is validated before processing the registration request.
- The route handler is wrapped in an array containing validation rules for the
body('username')
.trim()
.notEmpty()
.withMessage('Username is required'),
Validation Chain:
body('username')
starts a validation chain for theuserna
me
field in the request body.
Trim Whitespace:
.trim()
removes any leading or trailing whitespace characters from the username. This ensures that usernames with extra spaces at the beginning or end are considered invalid.
Empty Username Check:
.n
otEmpty
()
validates that the username field is not empty. This prevents users from submitting registrations without a username.
Custom Error Message:
.withMessage('Username is req
uired')
sets a custom error message to be displayed to the user if the username is empty. This provides clear feedback on what went wrong.
4. Validating Email:
body('email')
.isEmail()
.withMessage('Please enter a valid email'),
Validation Chain:
body('email')
starts a separate validation chain for theemail
field.
Email Format Check:
.isEmail()
validates that the email field contains a valid email address format. This ensures that users enter email addresses in a recognizable format (e.g., [email address removed]).
Custom Error Message:
.withMessage
('Please enter a valid email')
sets a custom error message to be displayed if the email format is invalid. This helps users understand the issue and provide a correct email address.
5. Handling Validation Results:
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
Extracting Errors:
const errors
= validation
Result(req)
extracts any validation errors that might have occurred during the validation process.
Checking for Errors:
if (!errors
.isEmpty())
{ ... }
checks if there are any errors present in theerrors
object.!errors.isEmpty()
essentially checks if theerrors
object is not empty.
Error Response:
- If there are errors, the code inside the
if
block executes. Here, we send a 400 Bad Request response with theerrors
object converted into an array using.array()
. This sends a JSON response containing the details of the validation errors back to the user.
- If there are errors, the code inside the
Benefits of Using Express-Validator
Improved Data Integrity: Ensures data received from users adheres to your defined rules, preventing unexpected behavior and potential security vulnerabilities.
Enhanced User Experience: Provides clear error messages to users when input doesn't meet requirements, guiding them towards valid submissions.
Cleaner Code: Separates validation logic from route handlers, promoting maintainability and readability.
Conclusion
Express-validator streamlines input validation in Node.js applications, saving you time and effort. By incorporating it in your development process, you create a more robust and secure backend experience. Remember to tailor validation rules to your specific application requirements.
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
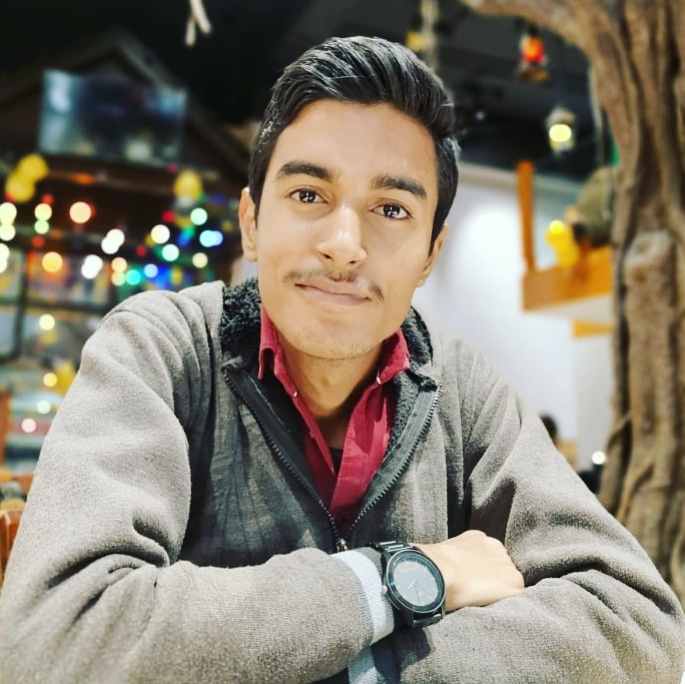
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.