Sentiment Analysis with R
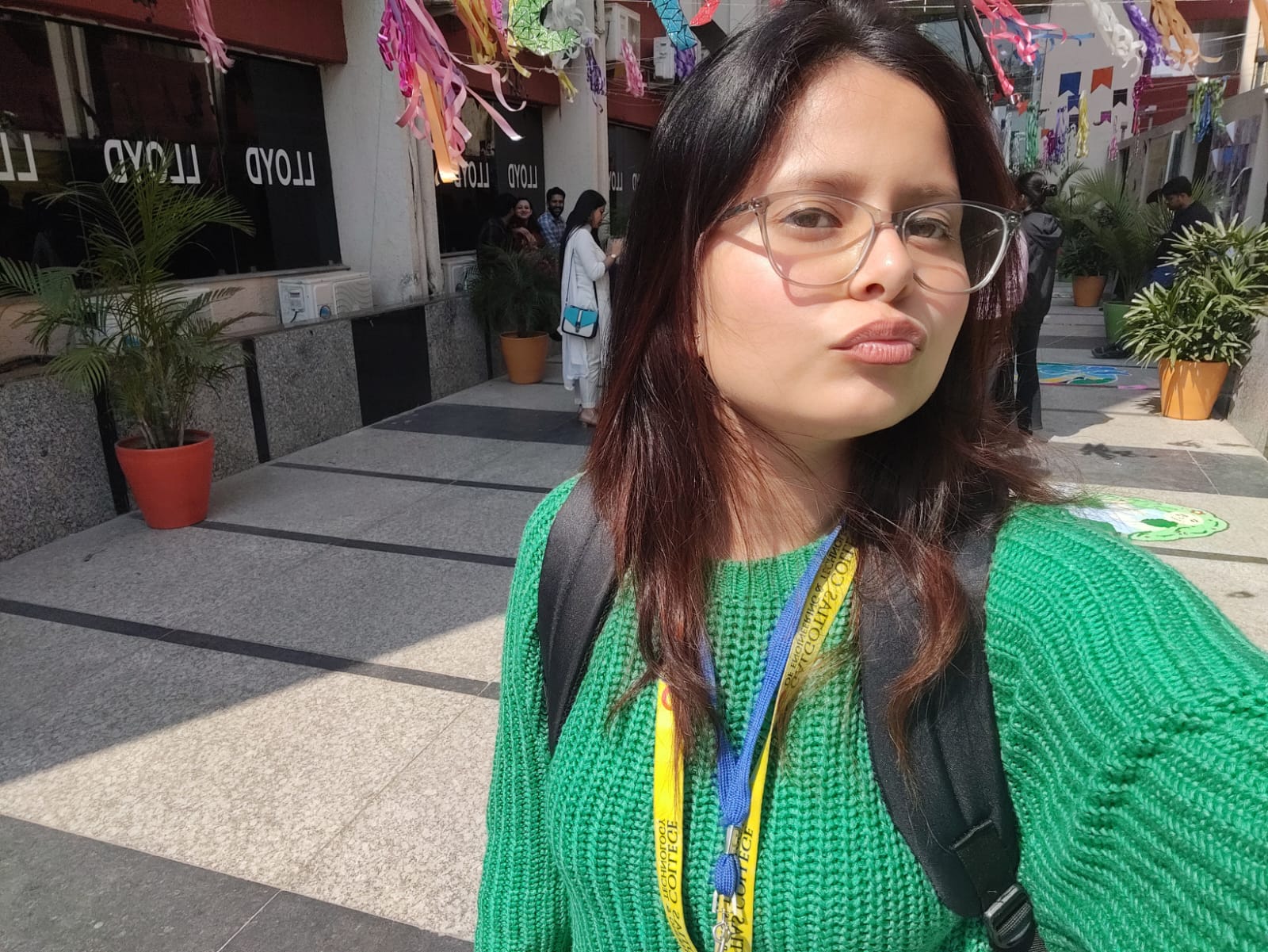
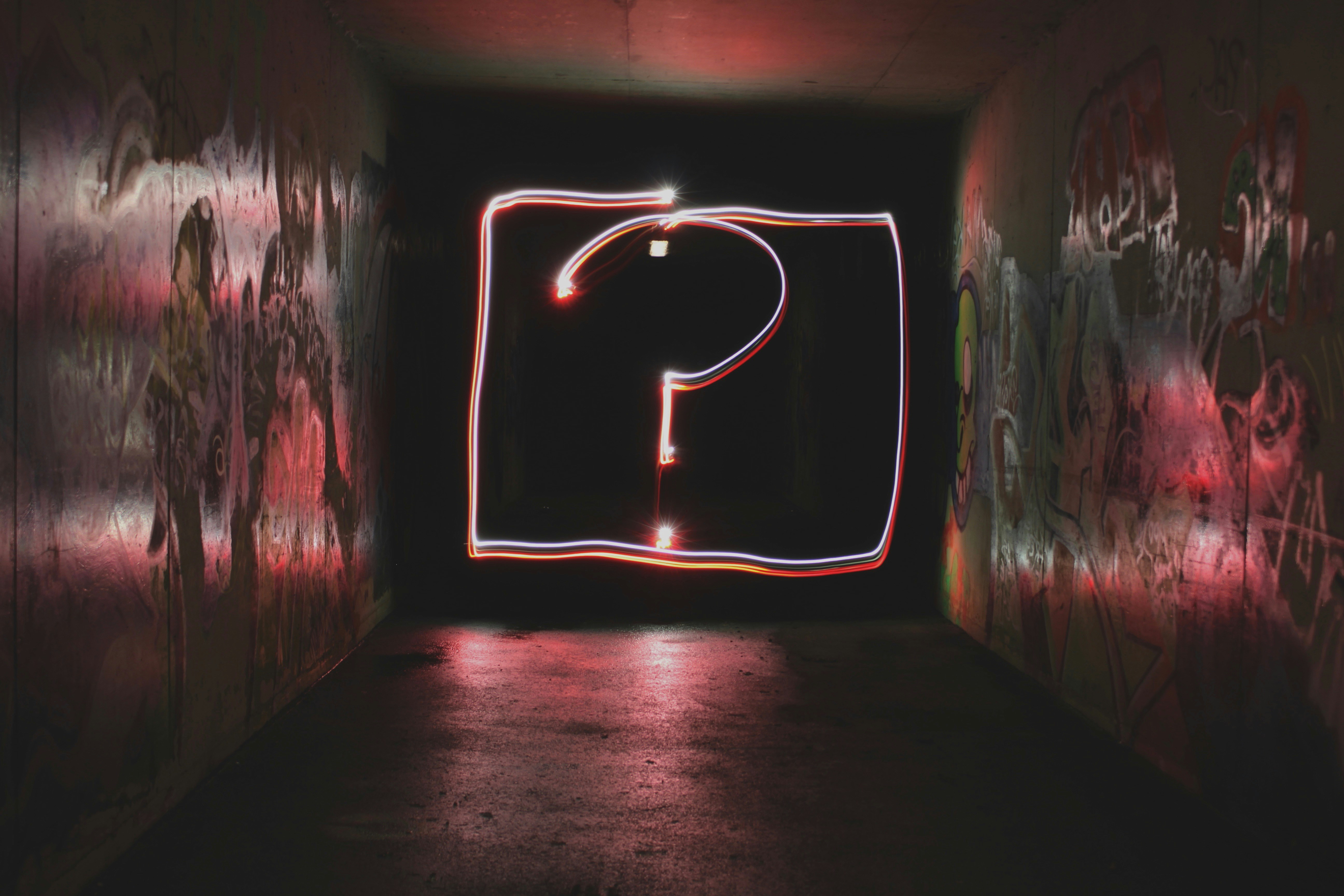
Sentiment analysis, or opinion mining, is a crucial subdomain of NLP that focuses on analyzing people’s stances or feelings toward provided textual data. This is used in many fields for different purposes, like social media monitoring, brand image and reputation analysis, customer satisfaction, and academic research on markets.
Preprocessing Text Data:
Common preprocessing steps include:
Tokenization: breaking text into individual words or tokens.
Noise removal involves getting rid of unwanted characters, such as URLs, HTML tags, and special characters, from the text.
Case normalization: It is common practice to transform the text into all lower or all upper case letters.
Stop word removal: In general, after deleting the most frequently used words, which are usually common to all texts, the sentiment is distorted less. (e.g., "the," "and," "or").
Stemming/Lemmatization: The process of converting a word to its basic or primitive form.
Handling Punctuation: Delimiters can become problematic when analyzing texts because they are prone to creating difficulties. This eliminates the inclusion of useless words, thus leaving only the meaningful ones inside.
Converting Text to Lowercase: Applying full-text search principally, transforming all text to lowercase, which reduces variance and generates accurate matches during analysis.
Example Code for Preprocessing:
1. Install the packages
2. Load the required packages
3. Preprocess with 'tm' and 'tidytext'
# Using tm
library(tm)
text_data <- c("I love programming in R!", "R is a fantastic tool for data science.")
corpus <- Corpus(VectorSource(text_data))
corpus <- tm_map(corpus, content_transformer(tolower)) # Convert to lowercase
corpus <- tm_map(corpus, removePunctuation) # Remove punctuation
corpus <- tm_map(corpus, removeWords, stopwords("en")) # Remove stop words
inspect(corpus)
# Using tidytext
library(tidytext)
library(dplyr)
text_df <- data.frame(text = text_data)
text_df <- text_df %>%
mutate(text = tolower(text)) %>% # Convert to lowercase
unnest_tokens(word, text) %>% # Tokenize text
filter(!word %in% stop_words$word) # Remove stop words
print(text_df)
Sentiment Lexicons:
A sentiment lexicon is a dictionary where words or phrases are associated with sentiment values such as positive, negative, or neutral, facilitating sentiment analysis. These lexicons can be used for sentiment classification where no training data is available or as features with the use of other available data. Some popular sentiment lexicons for English include:
AFINN
Bing
NRC Word-Emotion Association Lexicon
SentiWordNet
Example Code for Using Lexicons:
Install the Packages:
install.packages("tidytext")
install.packages("dplyr")
install.packages("readr")
Load the installed packages:
library(tidytext)
library(dplyr)
library(readr)
Download the "NRC-Emotion-Lexicon-Wordlevel-v0.92.txt" file from: http://saifmohammad.com/WebPages/lexicons.html.
Replace the path with the actual path in your system.
nrc_lexicon <- read_tsv("path/to/NRC-Emotion-Lexicon-Wordlevel-v0.92.txt")
Check that the lexicon was loaded correctly by printing the first few rows:
head(nrc_lexicon)
Complete code:
# Install packages (if not installed)
install.packages("tidytext")
install.packages("dplyr")
install.packages("readr")
# Load packages
library(tidytext)
library(dplyr)
library(readr)
# Load NRC lexicon
nrc_lexicon <- read_tsv("C:/Users/Vanshika/Downloads/NRC-Emotion-Lexicon-Wordlevel-v0.92.txt")
# View top rows of the lexicon
head(nrc_lexicon)
Supervised Sentiment Analysis:
Supervised sentiment analysis, on the other hand, entails the use of machine learning, and it relies on learning from training data that already comes with labels as to whether the text data is positive, negative, or neutral. Keep in mind that different adjectives can convey positivity, negativity, or neutrality. Popular algorithms for supervised sentiment analysis include:
Naive Bayes
Support Vector Machines (SVMs)
Decision Trees
Random Forests
Logistic Regression
Deep learning (e.g., recurrent neural networks, transformers)
Example code for the Naive Bayes classifier:
Install the required package:
install.packages("e1071")
install.packages("tm")
Load the library:
library(e1071)
library(tm)
Creating the "corpus" object using the "Corpus" function:
# Sample labeled data
data <- data.frame(
text = c("I love this product", "This is the worst experience"),
sentiment = factor(c("positive", "negative"))
)
# Preprocess text
corpus <- Corpus(VectorSource(data$text))
corpus <- tm_map(corpus, content_transformer(tolower))
corpus <- tm_map(corpus, removePunctuation)
corpus <- tm_map(corpus, removeWords, stopwords("en"))
corpus <- tm_map(corpus, stemDocument)
# Create Document-Term Matrix
dtm <- DocumentTermMatrix(corpus)
data_matrix <- as.matrix(dtm)
# Train Naive Bayes classifier
model <- naiveBayes(data_matrix, data$sentiment)
# Predict sentiment
predictions <- predict(model, data_matrix)
print(predictions)
Unsupervised Sentiment Analysis:
Unsupervised sentiment analysis entails assessing the sentiment of text data without the need for labeled training examples. This approach typically relies on sentiment lexicons or clustering algorithms to group similar texts based on sentiment.
Install the package:
install.packages("syuzhet")
Run this code:
library(syuzhet)
# Sample text
text <- "I am very happy with this product. It works amazingly well."
# Get sentiment scores
sentiment_scores <- get_nrc_sentiment(text)
print(sentiment_scores)
Sentiment Analysis Packages in R:
R offers several packages for sentiment analysis, including:
syuzhet: Provides access to sentiment lexicons and tools for sentiment extraction and visualization.
tidytext: An umbrella package of text mining and sentiment analysis tools that uses the various packages in the tidyverse.
quanteda: It is a software tool designed for statistical analysis of text data, with a specific focus on conducting sentiment analysis.
caret: To be specific, for predicting the mood or sentiment of the product, for example, sentiment analysis, there is a machine learning package for constructing and testing the quality of the predictive models.
tm: Text mining.
textdata: Offers raw text and dictionaries of positive/negative scores for the words.
Implementing Sentiment Analysis in R:
Here is a detailed example demonstrating how to conduct sentiment analysis in R using the syuzhet package in conjunction with the NRC Word-Emotion Association Lexicon:
# Install necessary packages if you haven't already
install.packages("tidyverse")
install.packages("tidytext")
install.packages("textclean")
install.packages("tm")
# Load the libraries
library(tidyverse)
library(tidytext)
library(textclean)
library(tm)
# Load data
tweets <- read.csv("tweets.csv")
# Preprocess text
tweets_clean <- tweets %>%
mutate(text = tolower(text), # Convert text to lowercase
text = str_replace_all(text, "[[:punct:]]", ""), # Remove punctuation
text = removeWords(text, stopwords("en"))) # Remove stopwords
# Tokenize text
tweets_tokens <- tweets_clean %>%
unnest_tokens(word, text)
# Join with sentiment lexicon
tweets_sentiment <- tweets_tokens %>%
inner_join(get_sentiments("bing"))
# Calculate sentiment scores
sentiment_scores <- tweets_sentiment %>%
count(word, sentiment) %>%
spread(sentiment, n, fill = 0) %>%
mutate(sentiment_score = positive - negative)
# Print sentiment scores
print(sentiment_scores)
Visualizing Sentiment Analysis Results:
Sentiment analysis can be visualized in several ways by R, as it offers chart types like bar charts, line charts, and word clouds.
Word Clouds:
library(wordcloud) wordcloud(sentiment_scores$word, sentiment_scores$sentiment_score)
- Bar Charts:
library(ggplot2)
ggplot(sentiment_scores, aes(x = word, y = sentiment_score)) +
geom_bar(stat = "identity") +
theme_minimal() +
labs(title = "Sentiment Scores", x = "Word", y = "Score")
Here's an example of visualizing sentiment scores using ggplot2:
After installing ggplot2, and loading it, run the code:
install.packages("ggplot2")
library(ggplot2)
library(ggplot2)
# Bar chart of sentiment scores
ggplot(sentiment_scores, aes(x = word, y = sentiment_score)) +
geom_bar(stat = "identity") +
theme_minimal() +
labs(title = "Sentiment Scores", x = "Word", y = "Score")
Case Studies and Examples:
Social media monitoring: brand image, product, and event discussion on the microblogging website (Tweet, Facebook, and Reddit).
Customer feedback analysis: analyzing customers’ feedback, ratings, or even the conversations during customer service to enhance the goods and services.
Political sentiment analysis: polling the people’s sentiment of the political candidates, policies, and events at a specific period [6, p. 7].
Stock market prediction: classification of positive and negative news articles or trends used for trading stocks and shares.
Practical Considerations and Best Practices:
Consider the following best practices for performing sentiment analysis:
Domain adaptation: It is understandable that the sentiment mining models may have to be fine-tuned to domains or areas of application since language, as well as sentiment, can often differ.
Handling sarcasm and irony: Sarcasm and irony are important because, more often than not, the literal meaning of the text and the implied opinion depends greatly on the context, which is more often than not illogical.
Handling negations: Negations (e.g., certain words (‘not good’) can have the reverse effect and change the polarity and therefore require attention.
Handling multi-word expressions: Some aspects are conveyed by multiword expressions (e.g., a single object in Word and Excel most often consists of two words (“not bad") is also a single object composed of two elements).
Handling multilingual data: In the case of script-defined measures, there is a possibility to apply language-specific models or lexicons.
Challenges and Limitations:
Explore the challenges and limitations that persist in sentiment analysis:While sentiment analysis has made significant progress, several challenges and limitations remain:
Subjectivity and context: This analysis underscores the relative nature of sentiment in different contexts or based on individual perceptions, which complicates identifying precise nuances.
Ambiguity and sarcasm: As mentioned earlier, detecting sarcasm and other forms of double entendre presents a significant challenge for sentiment analysis models.
Domain-Specific Vocabulary: In some instances, a specific domain may not be represented in any sentiment lexicon, posing a limitation.
Multilingual Support: It still complicates the matter when the text is written in several languages.
Future Directions:
Sentiment analysis is a wide scholarship area, and several improvements are expected to be made in the next few years. Some future directions include:
Deep learning: transformer-based models like BERT, etc. Popular transformer models such as BERT and GPT have exhibited spectacular performances in the fields of language modeling and sentiment analysis.
Transfer learning involves utilizing pre-trained language models and refining them for specific domains to improve outcomes in sentiment analysis.
Multimodal sentiment analysis involves incorporating text with additional modalities such as images, audio, and video to gain a more comprehensive understanding of the text.
Explainable AI: Towards Building Trustworthy Temporally Conditioned Sentiment Analysis for Relevance in the Real World.
Sentiment analysis at scale: designing efficient approaches to analyze vast amounts of information received from social media and other customers’ feedback tools.
Conclusion:
There are numerous libraries and functions in R for sentiment analysis, ranging from preprocessing to lexicon-based to actual supervised machine learning algorithms.
Nevertheless, there are challenges to applying the sentiment analysis methods; these include the squabbles of sarcasm, ambiguity, and linguistic differences across domains. Nevertheless, due to the trends in the development of natural language processing and machine learning techniques, sentiment analysis can be expected to become more accurate, efficient, and understandable soon.
Therefore, it is essential to follow standard recommendations, consider practical aspects, and stay informed about the advancements in sentiment analysis to implement this method in business and research effectively.
Subscribe to my newsletter
Read articles from Vanshika Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
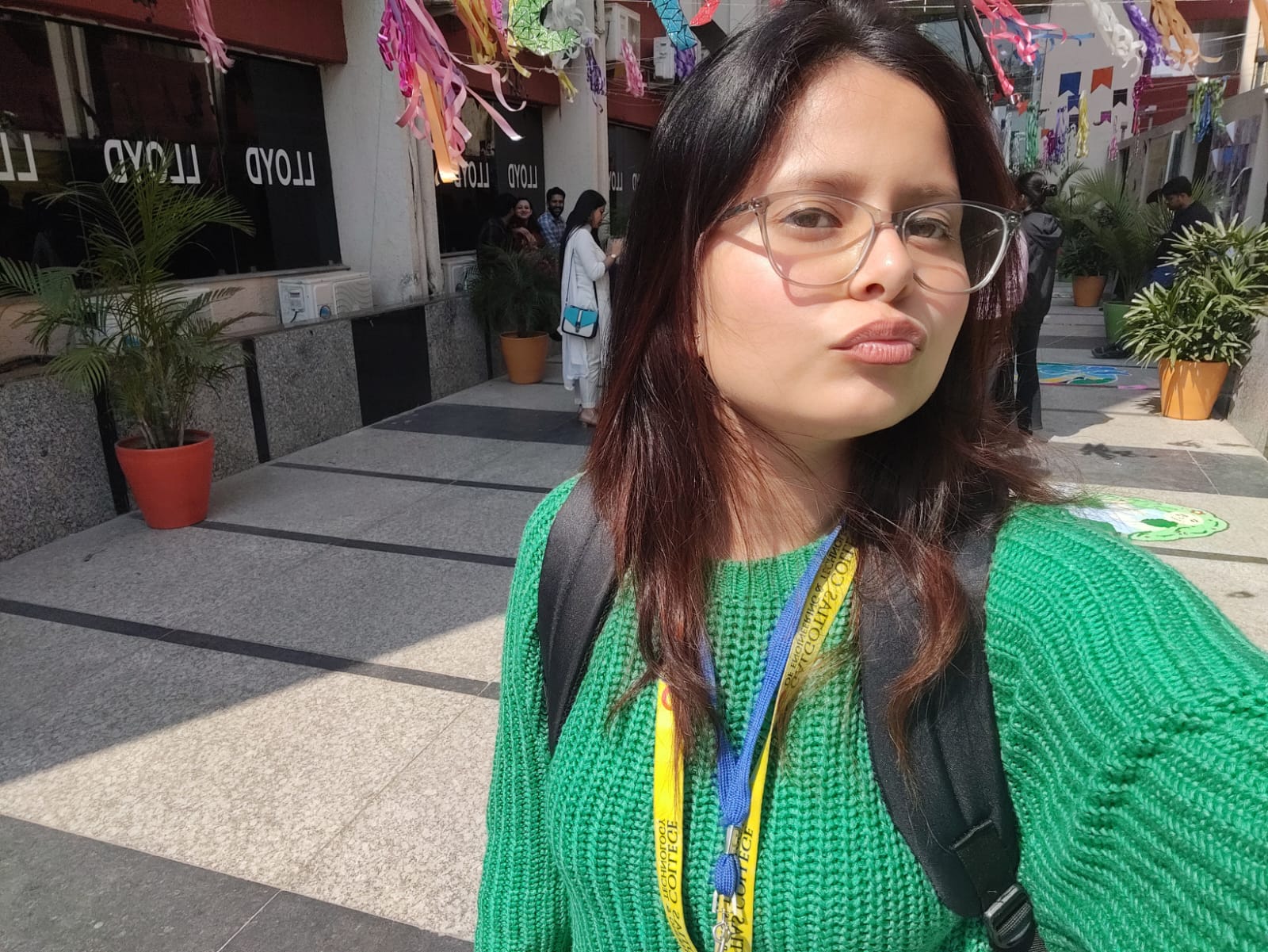
Vanshika Kumar
Vanshika Kumar
Sage Code Hooter: A dedicated "Python" and "Blockchain Enthusiast" Also, I write blogs!!!