Enhancing User Experience with a "Back to Top" Button in Astro

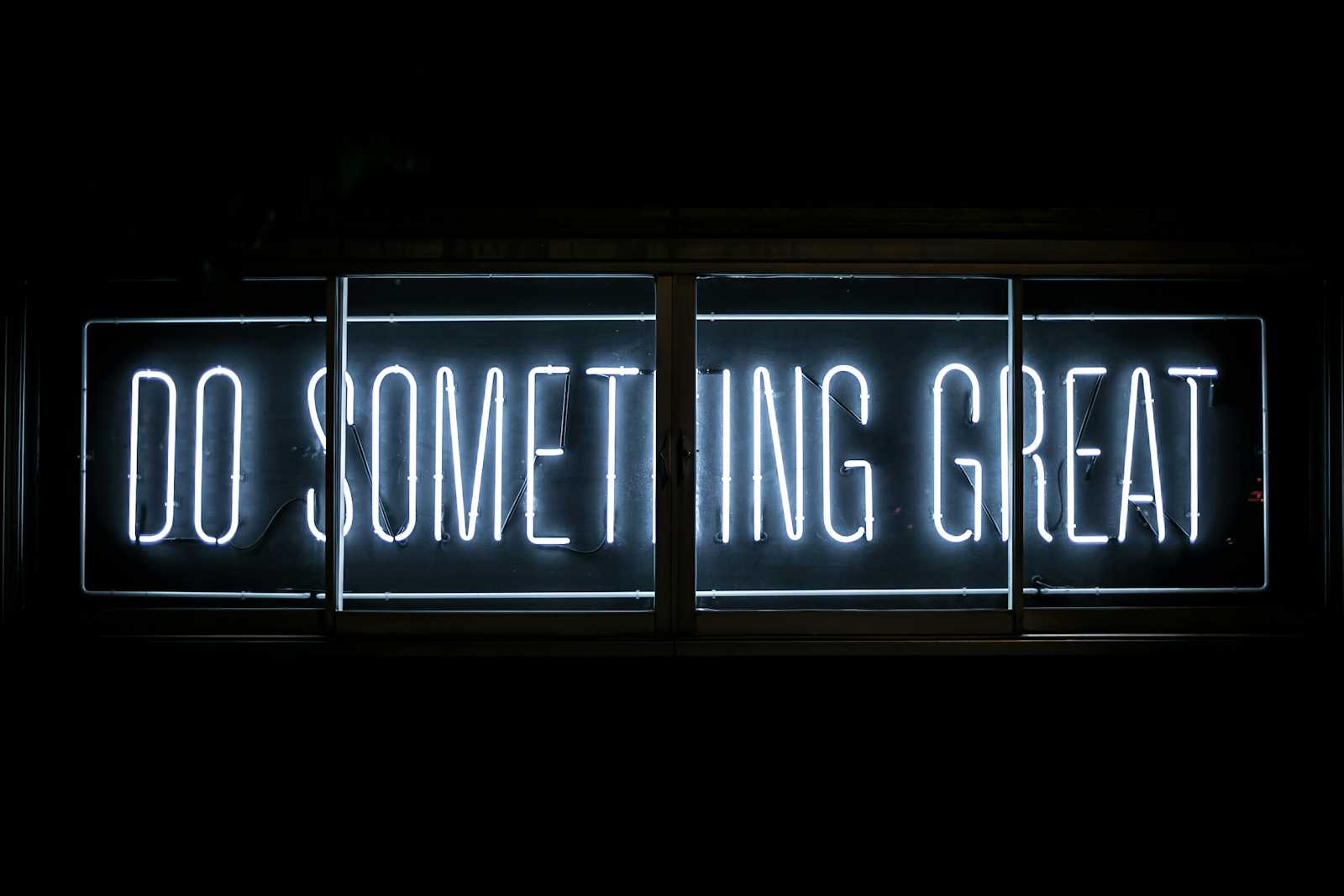
In the world of web development, small features can make a big difference in user experience. One such feature is the "Back to Top" button. This simple yet effective tool allows users to quickly return to the top of a page without tedious scrolling, especially on long, content-rich pages. In this article, we'll explore how to implement a sleek, accessible "Back to Top" button in an Astro project using Tailwind CSS and DaisyUI.
Why Implement a "Back to Top" Button?
Before diving into the implementation, let's consider why you might want to add this feature to your website:
Improved User Experience: It provides a quick and easy way for users to navigate back to the top of the page, especially on mobile devices or long-form content.
Increased Engagement: By making navigation easier, users are more likely to explore more of your content.
Accessibility: It offers an alternative method of page navigation, which can be particularly helpful for users with mobility impairments.
Modern Design: A smooth-scrolling "Back to Top" button adds a touch of interactivity and polish to your site.
Implementing the "Back to Top" Button
Let's break down the implementation of our BackToTop.astro
component:
The Button Structure
<button
id="back-to-top"
class="fixed bottom-5 right-5 bg-primary text-primary-content p-2 rounded-full shadow-lg transition-opacity duration-300 opacity-0 invisible"
aria-label="Back to top"
>
<svg xmlns="http://www.w3.org/2000/svg" class="h-6 w-6" fill="none" viewBox="0 0 24 24" stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M5 10l7-7m0 0l7 7m-7-7v18" />
</svg>
</button>
This HTML structure creates a button with the following features:
Fixed positioning in the bottom-right corner of the screen
Rounded shape with a primary background color
An SVG icon indicating upward movement
Smooth transition for appearance/disappearance
Accessibility support with an
aria-label
The JavaScript Logic
const backToTopButton = document.getElementById('back-to-top');
function toggleBackToTopButton() {
if (window.scrollY > 300) {
backToTopButton.classList.remove('opacity-0', 'invisible');
backToTopButton.classList.add('opacity-100', 'visible');
} else {
backToTopButton.classList.add('opacity-0', 'invisible');
backToTopButton.classList.remove('opacity-100', 'visible');
}
}
function scrollToTop() {
window.scrollTo({
top: 0,
behavior: 'smooth'
});
}
window.addEventListener('scroll', toggleBackToTopButton);
backToTopButton.addEventListener('click', scrollToTop);
This JavaScript code handles two main functions:
Toggling the button's visibility based on scroll position
Smooth scrolling to the top when the button is clicked
Integration with Astro and Tailwind CSS
The beauty of this implementation lies in its seamless integration with Astro and Tailwind CSS:
Astro Integration: By creating a separate
.astro
component, we encapsulate all the necessary HTML and JavaScript in one reusable file.Tailwind CSS Styling: We use Tailwind utility classes for styling, making it easy to customize the appearance and ensuring consistency with your site's design system.
DaisyUI Theming: By using DaisyUI color classes like
bg-primary
, the button automatically adapts to your site's theme, including dark mode support.
Conclusion
Implementing a "Back to Top" button is a small change that can significantly enhance your website's usability. With Astro's component-based architecture and the power of Tailwind CSS, adding this feature becomes a straightforward and maintainable task.
By following the implementation described in this article, you'll provide your users with a smooth, accessible way to navigate your content, ultimately leading to a better overall user experience.
Remember, in web development, it's often the small details that make a big difference. A well-implemented "Back to Top" button is one such detail that your users will appreciate, even if they don't consciously notice it.
Subscribe to my newsletter
Read articles from 문지웅 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
