Strategies to Optimize Hierarchical Data in SQL Databases for Better Performance
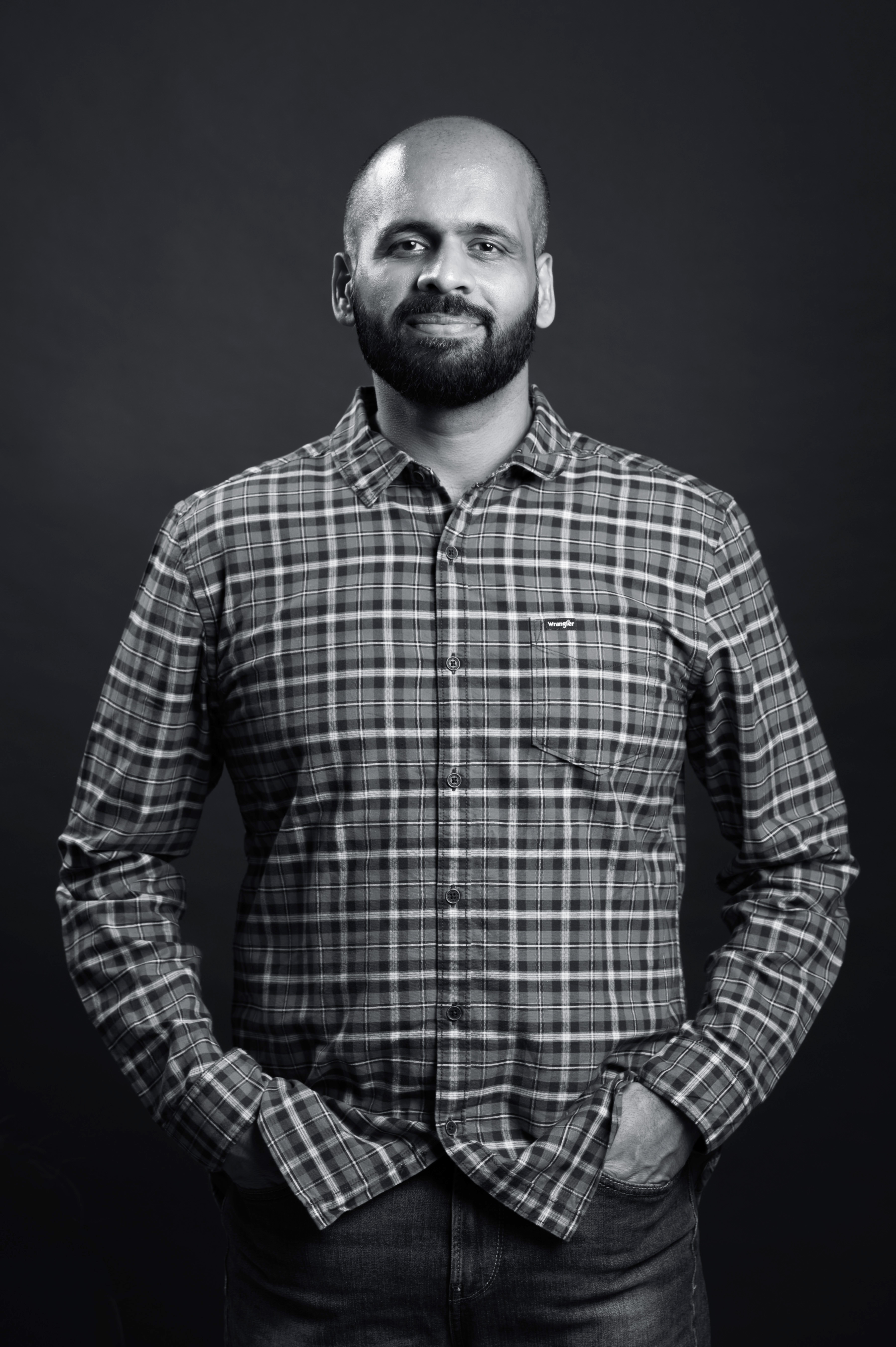
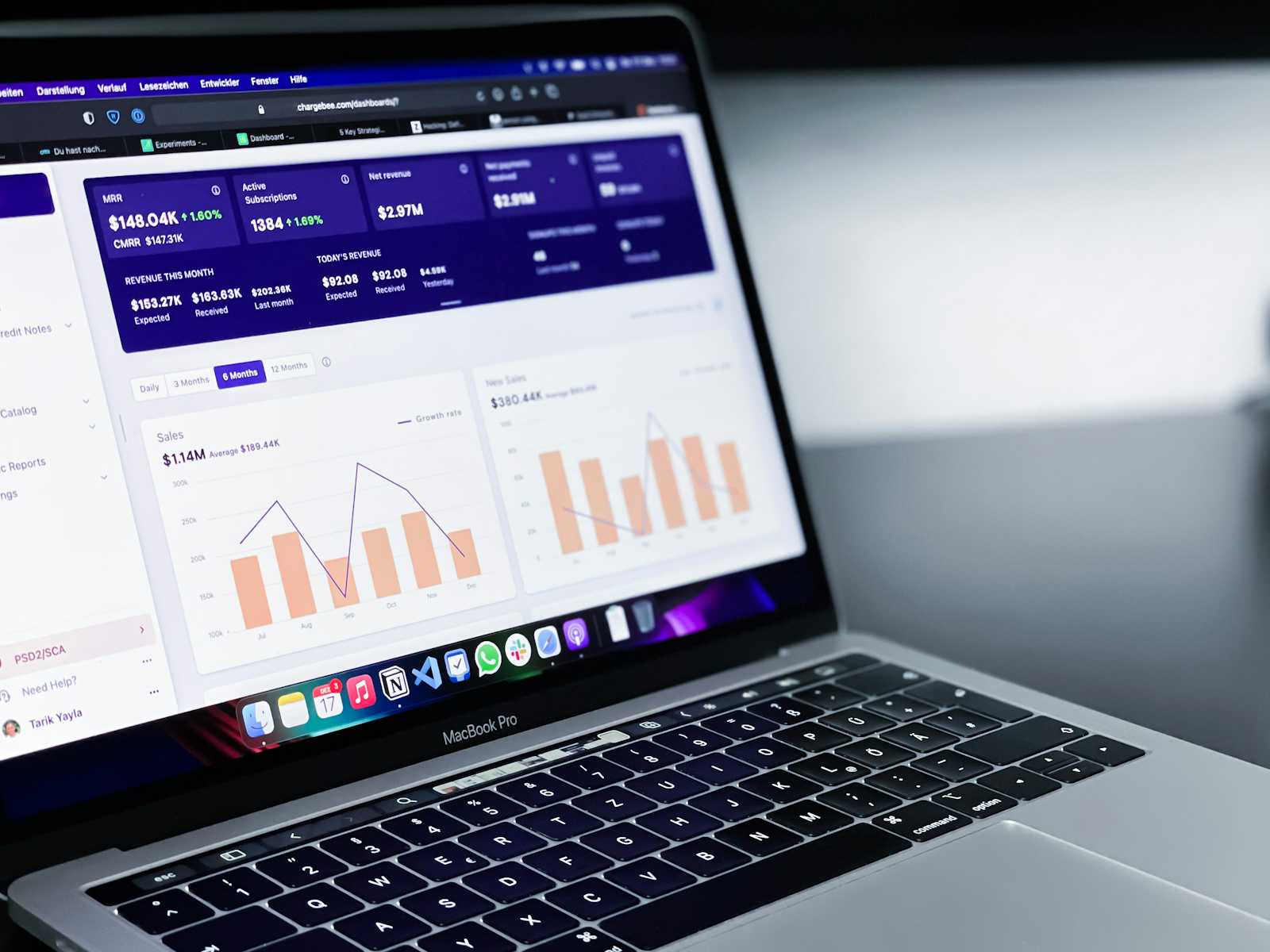
Applying hierarchical optimization to network structures in SQL-based database engineering involves organizing and optimizing hierarchical data to improve query performance, data integrity, and overall system efficiency. Here’s a detailed guide on how to approach this:
1. Understand the Hierarchical Data Model
Hierarchical data in databases typically represent parent-child relationships, such as organizational charts, file systems, or product categories. Common representations include adjacency lists, nested sets, and materialized paths.
2. Choose an Appropriate Representation
Each representation has its own trade-offs in terms of ease of querying and updating:
Adjacency List: Each record has a pointer to its parent. This is simple but can be inefficient for certain types of queries.
Nested Set: Nodes are assigned left and right values that define their position in the hierarchy. This allows for efficient querying but can be complex to update.
Materialized Path: Each record stores the path from the root to the node. This simplifies certain queries and updates but can lead to redundancy.
3. Indexing Strategies
Proper indexing is crucial for performance:
Primary Key Indexes: Ensure that each table has a primary key for uniqueness and quick access.
Foreign Key Indexes: Index foreign keys to improve join performance.
Composite Indexes: Create composite indexes on columns frequently used together in queries.
Full-Text Indexes: For searching text within nodes, consider full-text indexing.
4. Query Optimization Techniques
Optimize queries to leverage the hierarchical structure efficiently:
Recursive Common Table Expressions (CTEs): Use CTEs to simplify and optimize hierarchical queries in SQL.
WITH RECURSIVE hierarchy AS ( SELECT id, name, parent_id FROM employees WHERE parent_id IS NULL UNION ALL SELECT e.id, e.name, e.parent_id FROM employees e INNER JOIN hierarchy h ON e.parent_id = h.id ) SELECT * FROM hierarchy;
Tree Traversal Queries: Use optimized queries for tree traversal, depending on the chosen hierarchical representation.
Batch Updates: Perform batch updates to reduce the number of transactions and improve performance.
5. Data Integrity and Constraints
Ensure data integrity through constraints and triggers:
Foreign Key Constraints: Enforce parent-child relationships.
Triggers: Use triggers to automatically update nested set values or maintain materialized paths.
6. Caching Strategies
Implement caching to reduce database load:
Materialized Views: Create materialized views for frequently accessed hierarchical data.
In-Memory Caches: Use in-memory caching solutions (e.g., Redis) to store hierarchical data.
7. Monitoring and Maintenance
Regularly monitor and maintain the database:
Query Performance Monitoring: Use tools like EXPLAIN and query logs to monitor query performance.
Index Maintenance: Regularly rebuild and reorganize indexes to maintain performance.
Data Consistency Checks: Periodically check for data consistency in the hierarchy.
Example Implementation
Here’s an example implementation using the nested set model in SQL:
Schema Definition
CREATE TABLE categories (
id INT PRIMARY KEY,
name VARCHAR(100),
lft INT,
rgt INT
);
Inserting Data
INSERT INTO categories (id, name, lft, rgt) VALUES
(1, 'Electronics', 1, 14),
(2, 'Laptops', 2, 7),
(3, 'Smartphones', 8, 13),
(4, 'Apple', 3, 4),
(5, 'Dell', 5, 6),
(6, 'Samsung', 9, 10),
(7, 'OnePlus', 11, 12);
Querying the Hierarchy
To query all descendants of 'Electronics':
SELECT * FROM categories
WHERE lft BETWEEN 1 AND 14;
Recommendations
Choose the right hierarchical model based on your specific use case and query requirements.
Optimize your queries with appropriate indexing and recursive CTEs.
Maintain data integrity with constraints and triggers.
Monitor performance regularly and adjust your indexing and caching strategies accordingly.
Additional Resources
For more detailed insights and examples, consider reviewing database optimization guides and SQL documentation specific to hierarchical data structures. Online resources such as database management forums and articles on SQL optimization can also provide valuable information.
Subscribe to my newsletter
Read articles from Shiv Iyer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
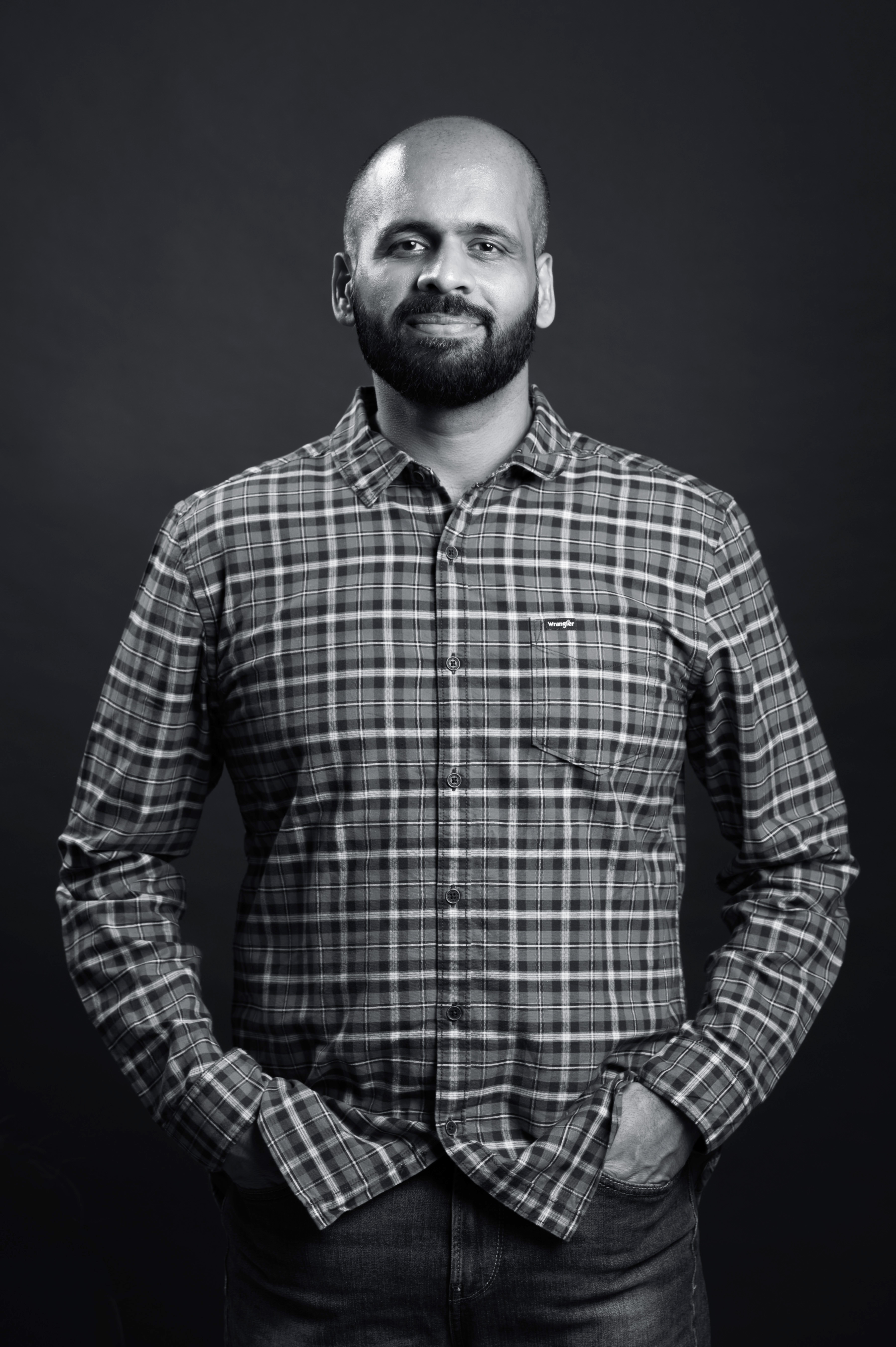
Shiv Iyer
Shiv Iyer
Over two decades of experience as a Database Architect and Database Engineer with core expertize in Database Systems Architecture/Internals, Performance Engineering, Scalability, Distributed Database Systems, SQL Tuning, Index Optimization, Cloud Database Infrastructure Optimization, Disk I/O Optimization, Data Migration and Database Security. I am the founder CEO of MinervaDB Inc. and ChistaDATA Inc.