JavaScript Data Types Explained: A Beginner's Guide
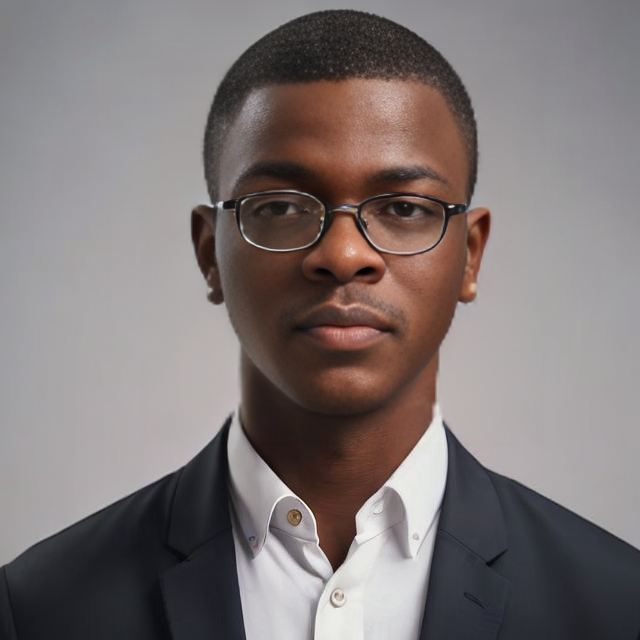
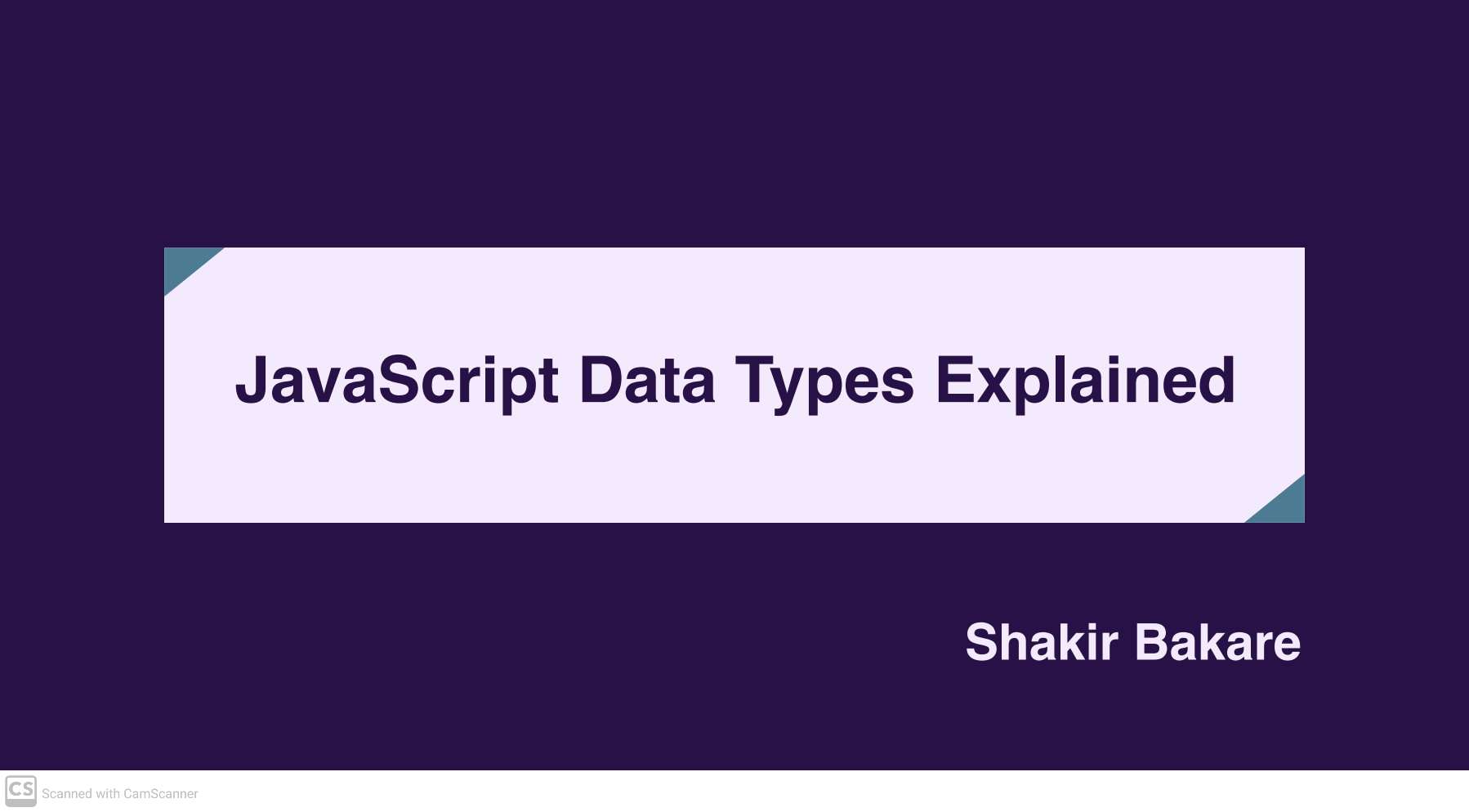
JavaScript has a number of data types to handle different forms of data. It's important to understand these data types because they define how data is stored, manipulated, and used within applications.
This article explains the data types in JavaScript, their categories, and how you can convert them.
JavaScript’s data types are categorized into two groups: primitive types and reference types.
1. Primitive Data Types
Primitive data types are the basic forms of data in JavaScript. They are:
Number
String
Boolean
Undefined
Null
Symbol
BigInt
Number
This represents whole numbers (positive or negative), decimals, special values such as Infinity
, -Infinity
, and NaN
.
let age = 16;
// a whole number
let pi = 2.18;
// a decimal
let x = 1.797693134862315E+308;
// Infinity
let x = -1.797693134862316E+308;
// - Infinity
let largeNumber = 2e6; // 2,000,000
String
String represents texts. It is enclosed in single quotes, double quotes, or backticks.
let name = 'John Doe';
let greeting = "Hello, world!";
let templateLiteral = `Hello, ${name}`;
Boolean
It represents logical values. It can be either true
or false
.
let isActive = true;
let isAdult = false;
Undefined
This is a variable that is not assigned a value.
let notAssigned;
console.log(notAssigned);
// undefined
Null
Null is a variable with no value or an object that doesn't exist.
let emptyValue = null;
Symbol
This is a unique and immutable primitive value. It is used for object property keys.
let sym1 = Symbol('identifier');
let sym2 = Symbol('identifier');
console.log(sym1 === sym2); // false
BigInt
This represents whole numbers larger than what the number
type can handle.
let bigNumber = BigInt(123456789012345678901234567890);
2. Reference Data Types
These are complex. They include objects, arrays, and functions. They store references to the memory where data is held, not the data itself.
Object
An object is a collection of properties, where each property is a key-value pair. Objects store keyed collections and complex entities.
let person = {
name: 'Alice',
age: 30,
isStudent: false
};
Array
This is an organized collection of data. It can be any data type (numbers, strings, or more). An array is a special type of object. It can store multiple values of any type in one variable.
let numbers = [1, 2, 3, 4, 5];
let mixedArray = ['text', 42, true];
Function
A function is a block of code designed to perform a task. Functions are first class objects. That means you can store them inside variables, you can pass them as arguments, and you can return them from other functions.
function greet(name) {
return `Hello, ${name}`;
}
Date
This handles date and time. The Date object provides methods for working with dates and times.
let today = new Date();
console.log(today.toDateString());
RegExp
It stands for Regular Expression. It is used for pattern-matching within strings.
let pattern = /abc/;
let result = pattern.test('abcde'); // true
Map and Set
Maps are collections of key-value pairs. Sets are collections of unique values.
let map = new Map();
map.set('key1', 'value1');
let set = new Set();
set.add(1);
set.add(2);
set.add(1); // Duplicate, will not be added
Type Conversion
JavaScript allows you to convert variables type during execution. This conversion can be implicit (coercion) or explicit (casting).
1. Implicit Conversion
This conversion is done automatically by JavaScript.
let result = '5' - 2;
// 3, string '5' is converted to number 5
2. Explicit Conversion
This conversion is done manually by you the developer.
let num = Number('123'); // 123
let str = String(123); // '123'
Conclusion
It's important to understand data types for efficient coding. You will write easy to maintain code when you know the differences between primitive and reference types, and how type conversion works.
And when you understand and master these, you can use JavaScript full potentials to build dynamic and robust web applications.
Subscribe to my newsletter
Read articles from Shakir Bakare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
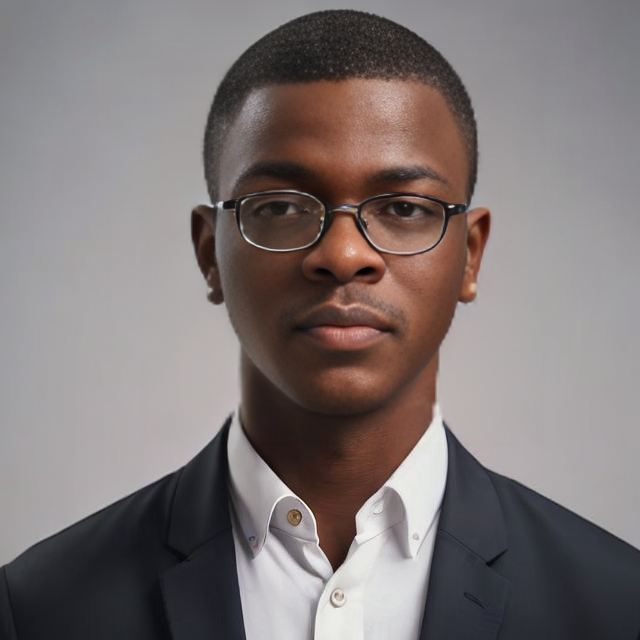
Shakir Bakare
Shakir Bakare
I'm a passionate frontend web developer with a love for creating beautiful and responsive web applications. With a strong foundation in HTML, CSS, JavaScript, and frameworks like React and Vue.js, I strive to bring innovative ideas to life. When I'm not coding, I enjoy sharing my knowledge through technical writing. I believe in the power of clear and concise documentation, and I write articles and tutorials to help others on their coding journey. Let's connect and create something amazing together!