Android Activities and Their Lifecycle: A Developer's Guide
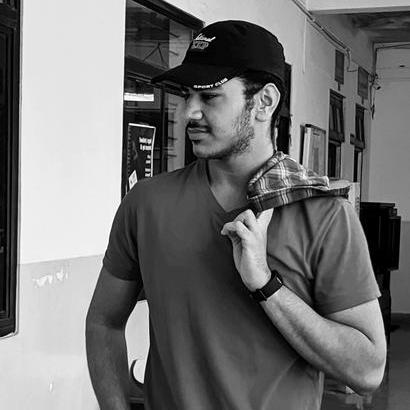
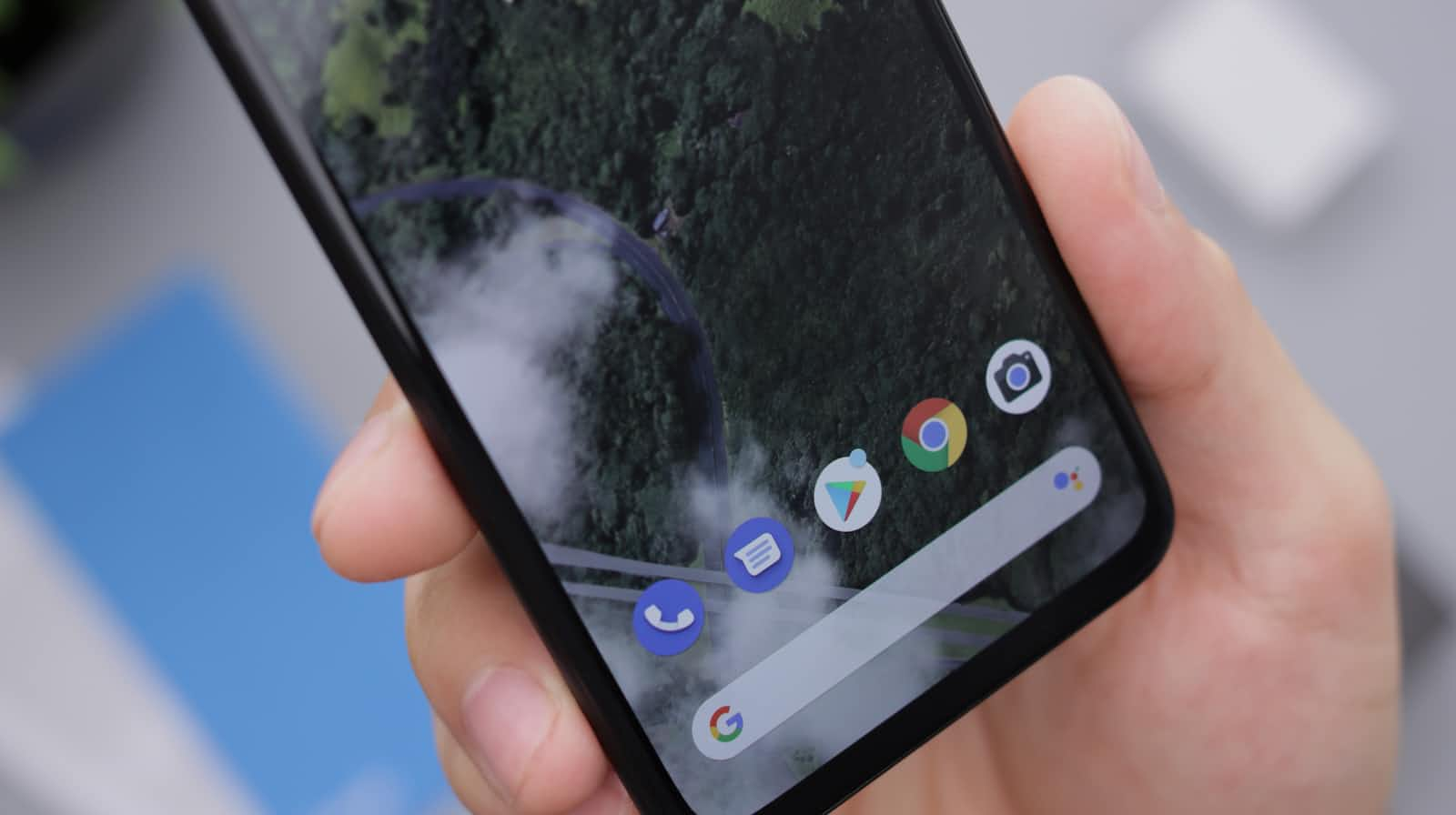
Introduction
An Activity is an application component that provides a screen for user interaction, allowing users to perform tasks like taking a photo, sending a message, or playing a video. Each activity is given a window to draw its user interface, typically filling the screen but sometimes smaller and floating over other windows.
An application can have multiple activities, loosely bound to each other, with each activity declared in the manifest file. One activity is usually designated as the "main" or "launcher" activity, presented to the user when the application is launched for the first time. Each activity can start other activities to perform different actions.
Activity Lifecycle
Activities are managed by the Android operating system and follow a lifecycle consisting of various states and callbacks. Understanding the activity lifecycle is crucial for managing resources, handling user interactions, and maintaining a smooth user experience.
Here is a diagrammatic representation of activity lifecycle:
Here's a brief overview of the different states and callbacks in the lifecycle:
onCreate():
This is the first callback called when an activity is created.
Initialize essential components, set up the user interface, and perform any one-time setup tasks.
onStart():
The activity enters the "started" state after
onCreate()
.The activity becomes visible to the user but doesn't have focus yet.
onResume():
The activity moves to the "resumed" state and comes into the foreground.
It is now fully visible and ready to receive user input.
Start animations, initialize sensors, or register broadcast receivers here.
onPause():
Triggered when the activity loses focus and goes into the background.
The activity is still partially visible but no longer has user input focus.
Release resources, pause animations, or save persistent data for a smooth transition.
onStop():
The activity enters the "stopped" state when it is no longer visible to the user.
This can happen when the user navigates away from the activity.
Perform operations that should happen when the activity is not visible, such as saving data or releasing resources that are not needed while the activity is not in the foreground.
onRestart():
Invoked if an activity was stopped and is now being restarted, before
onStart()
.Perform any necessary setup before the activity becomes visible again.
onDestroy():
The final callback in the activity lifecycle, called when the system is about to destroy the activity.
Clean up all remaining resources, unregister receivers, and ensure all necessary data is saved.
Example:
Activity Declaration:
- Define the activity class in your application. This is where you initialize components and set up the user interface.
class DemoActivity : AppCompatActivity() {
private val TAG = "Demo_Activity"
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_demo)
Log.d(TAG, "onCreate: ")
}
override fun onStart() {
super.onStart()
Log.d(TAG, "onStart: ")
}
override fun onResume() {
super.onResume()
Log.d(TAG, "onResume: ")
}
override fun onPause() {
super.onPause()
Log.d(TAG, "onPause: ")
}
override fun onStop() {
super.onStop()
Log.d(TAG, "onStop: ")
}
override fun onRestart() {
super.onRestart()
Log.d(TAG, "onRestart: ")
}
override fun onDestroy() {
super.onDestroy()
Log.d(TAG, "onDestroy: ")
}
}
Manifest File:
Ensure your activity is declared in the manifest file. This is crucial for the Android system to recognize and manage your activity.
Intent Filter:
Add an
<intent-filter>
to specify the types of intents the activity can handle.For the launcher activity, include:
<action android:name="android.intent.action.MAIN" />
to indicate the entry point of the application.<category android:name="android.intent.category.LAUNCHER" />
to specify that this activity should appear in the app launcher.
<manifest>
<application ...>
...
<activity
android:name="._04activities.DemoActivity"
android:exported="false" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Lifecycle Methods Execution in Different Scenarios
When the App is Launched:
The activity goes through the following lifecycle methods:
onCreate()
onStart()
onResume()
When There is a Configuration Change (e.g., Screen Rotation):
The activity handles the configuration change by going through these lifecycle methods:
onPause()
onStop()
onDestroy()
onCreate()
onStart()
onResume()
When the App is Closed and Removed from the Background:
When the app is moved to the background, the activity goes through:
onPause()
onStop()
When the app is removed from the background, the activity enters:
onDestroy()
Conclusion
In conclusion, Android activities are essential for creating engaging and dynamic user interfaces in apps. From initializing components in onCreate()
to managing resources and responding to user interactions throughout its lifecycle, each activity plays a key role in delivering a seamless user experience. By understanding and effectively managing the activity lifecycle, you can optimize the performance, ensure efficient resource utilization, and enhance overall app reliability.
Subscribe to my newsletter
Read articles from Yashraj Singh Jadon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
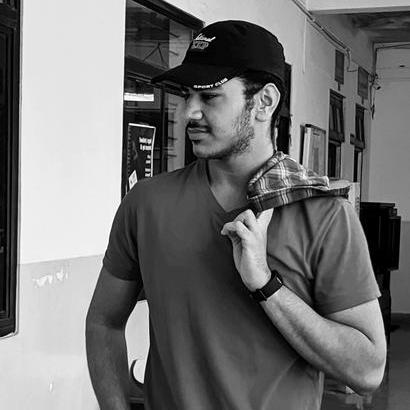
Yashraj Singh Jadon
Yashraj Singh Jadon
Hello, I'm an Android developer passionate about creating mobile apps.