A Beginner's Guide to Unit Testing in C# Using xUnit
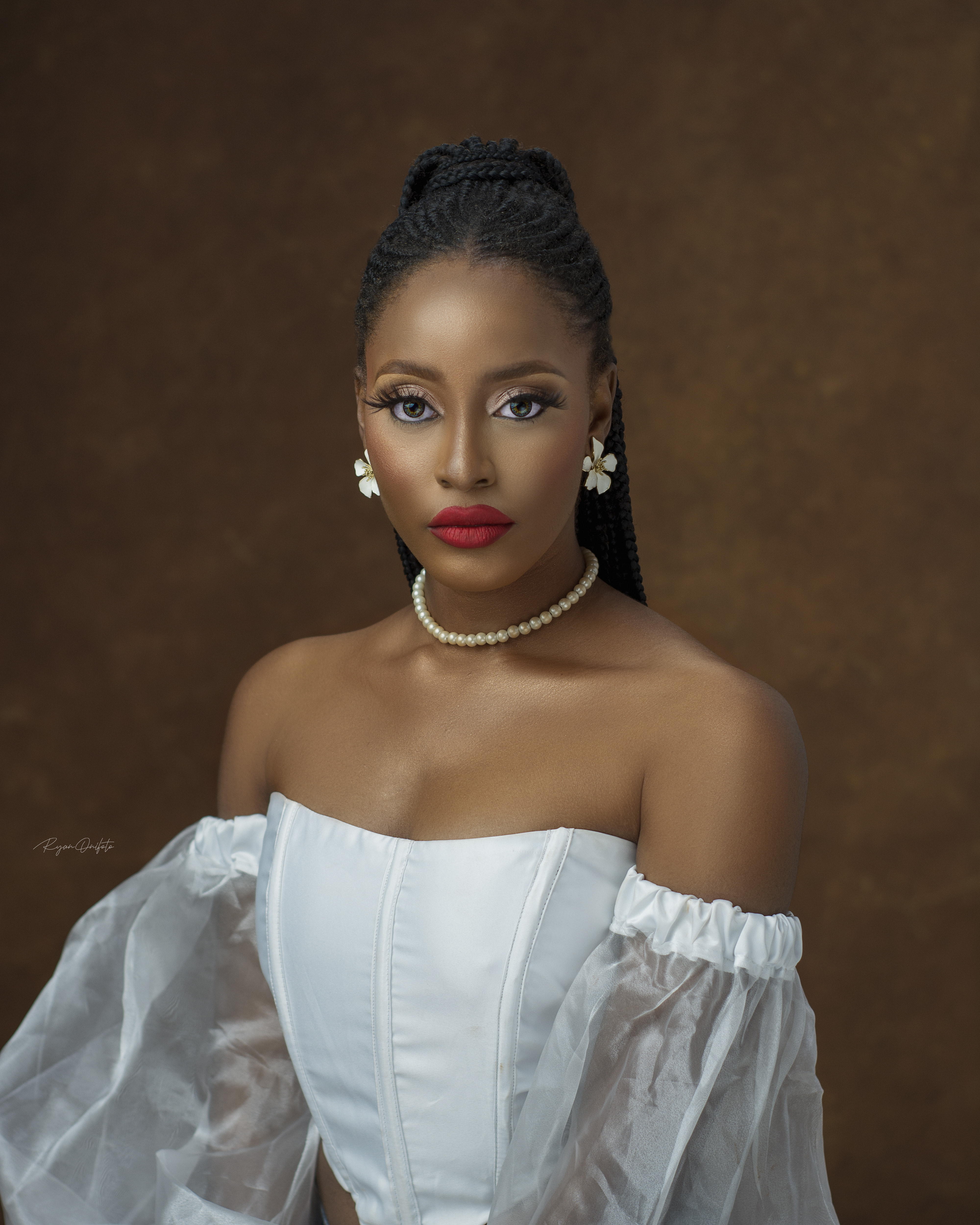
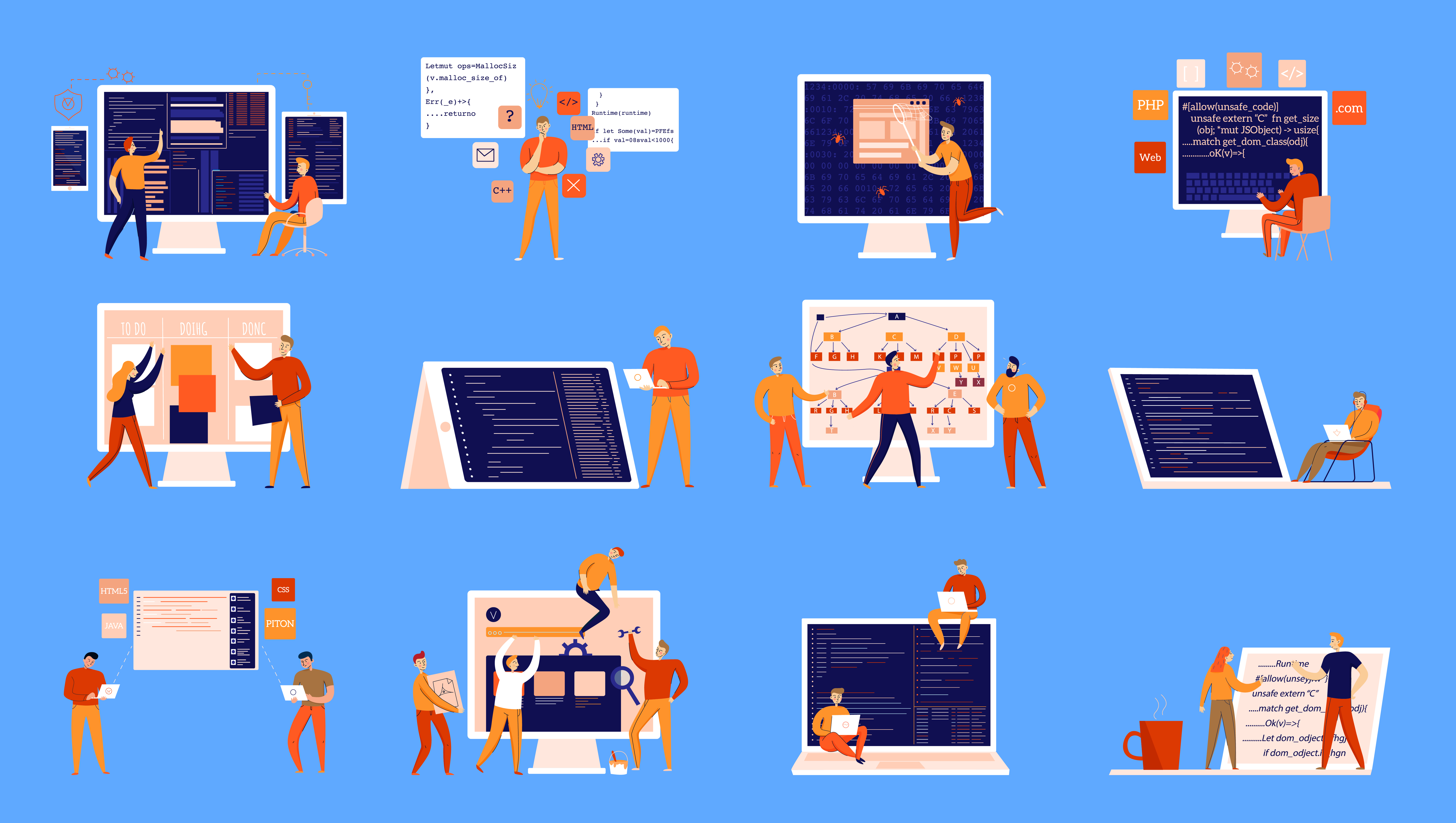
Welcome to the third article in the "Mastering C#" series. In this article, we will explore unit testing in C# using xUnit, a popular and flexible framework for .NET that simplifies writing and running tests. xUnit supports modern features like parameterized tests, asynchronous testing, and parallel test execution, making it a favorite among .NET developers due to its simplicity and versatility.
Pre-requisites
To fully benefit from this article, readers should have the following prerequisites:
Basic Knowledge of C#:
Understanding of C# syntax and basic programming concepts.Familiarity with .NET:
Experience with .NET development, including creating and running projects.Visual Studio or .NET SDK Installed:
Ensure you have Visual Studio or the .NET SDK installed on your machine.Understanding of Unit Testing Concepts:
Basic knowledge of what unit testing is and why it is important.NuGet Package Management:
Familiarity with adding and managing NuGet packages in your project.
Table of Contents
Introduction to xUnit
Setting Up xUnit
Writing and Running Unit Tests
Advanced Testing with xUnit
Mocking Dependencies
Testing Best Practices
Introduction to xUnit
What is xUnit?
xUnit is a widely used framework for writing and running unit tests in .NET. It helps ensure your code works correctly by running small, specific tests on different parts of your code. Designed for simplicity and efficiency, xUnit makes it easier for developers to write tests and ensure their code behaves as expected.
Why Use xUnit for Unit Testing?
Using xUnit for unit testing has several benefits:
Easy to Use:
xUnit is straightforward and user-friendly, allowing even beginners to start writing tests quickly.Modern Features:
It supports the latest testing practices, like parameterized tests, asynchronous tests, and running tests in parallel.Flexible and Extensible:
xUnit can be easily adapted to fit various testing needs and can be extended with additional tools and libraries.Reliable:
By using xUnit, you can catch bugs early, making your code more reliable and robust.
Setting Up xUnit
Setting up xUnit in your C# project involves a few steps, including installation via NuGet and configuring your project. Here’s a detailed guide to getting you started:
Installing xUnit via NuGet
Open Your Project:
Launch Visual Studio and open your C# project where you want to add unit tests.Access NuGet Package Manager:
Right-click on your project in the Solution Explorer.
Select "Manage NuGet Packages..."
Search for xUnit:
In the NuGet Package Manager, search for
"xUnit"
.Select the xUnit package from the search results.
Install xUnit
Click on the
"Install"
button next to the xUnit package.Follow the prompts to accept the installation. NuGet will download and add xUnit and its dependencies to your project.
Configuring Your Project for xUnit
Create a Test Project (if not already created):
If you don’t have a separate test project, create one in your solution. This keeps your test code separate from your application code.Add References:
Ensure your test project references the main project where your code resides. This allows xUnit to access your classes and methods for testing.Set Up Test Classes:
Create a new class or use existing ones to write your test methods. These classes will contain your unit tests that verify the behavior of your code.
Writing and Running Unit Tests
Creating Your First Test
To get started with unit testing using xUnit in C#, follow these steps to create your first test:
Create a New Test Project:
Start by creating a new C# class library project in your preferred IDE (e.g., Visual Studio Code).Install xUnit NuGet Package:
Add xUnit to your project by installing thexunit
andxunit.runner.visualstudio
packages via NuGet Package Manager or using the .NET CLI:dotnet add package xunit dotnet add package xunit.runner.visualstudio
Write Your First Test:
In your project, create a new class for your tests. Let's create a simple test that verifies basic functionality:using Xunit; public class CalculatorTests { [Fact] public void Add_TwoNumbers_ReturnsSum() { // Arrange int a = 3; int b = 5; var calculator = new Calculator(); // Act int result = calculator.Add(a, b); // Assert Assert.Equal(8, result); } } public class Calculator { public int Add(int a, int b) { return a + b; } }
This code snippet demonstrates a simple unit test for a Calculator
class using the xUnit framework in C#. Let's break it down step by step:
Code Overview
Calculator Class
The Calculator
class has a single method Add
which takes two integers as input and returns their sum.
public class Calculator
{
public int Add(int a, int b)
{
return a + b;
}
}
Purpose: This class provides basic addition functionality.
Method:
Add(int a, int b)
: Adds two integersa
andb
and returns their sum.
CalculatorTests Class
The CalculatorTests
class contains a unit test for the Calculator
class.
using Xunit;
public class CalculatorTests
{
[Fact]
public void Add_TwoNumbers_ReturnsSum()
{
// Arrange
int a = 3;
int b = 5;
var calculator = new Calculator();
// Act
int result = calculator.Add(a, b);
// Assert
Assert.Equal(8, result);
}
}
Namespace:
Xunit
is imported, which is the xUnit framework used for testing.Test Class:
CalculatorTests
is the test class that will contain all test methods for theCalculator
class.
Test Method
[Fact]
public void Add_TwoNumbers_ReturnsSum()
{
// Arrange
int a = 3;
int b = 5;
var calculator = new Calculator();
// Act
int result = calculator.Add(a, b);
// Assert
Assert.Equal(8, result);
}
[Fact] Attribute: Indicates that this method is a test method that should be run by the test runner.
Method Name:
Add_TwoNumbers_ReturnsSum
follows a naming convention that describes the test's purpose: it tests theAdd
method with two numbers and expects the result to be their sum.
Test Method Steps
Arrange:
int a = 3; int b = 5; var calculator = new Calculator();
Setup: Initialize the inputs
a
andb
with values3
and5
respectively.Create: Instantiate the
Calculator
class.
Act:
int result = calculator.Add(a, b);
Execute: Call the
Add
method of theCalculator
class with the inputsa
andb
.Capture: Store the result in the variable
result
.
Assert:
Assert.Equal(8, result);
Verify: Check if the result of the
Add
method is equal to8
(the expected sum of3
and5
).Assertion: The
Assert.Equal
method is used to compare the expected value (8
) with the actual result. If they are not equal, the test fails.
Summary
The
Calculator
class provides a simple addition method.The
CalculatorTests
class uses xUnit to test theAdd
method.The test method
Add_TwoNumbers_ReturnsSum
arranges inputs, acts by calling the method under test, and asserts the expected outcome.The
[Fact]
attribute marks the method as a test that should be executed by the xUnit test runner.
By running this test, you ensure that the Add
method of the Calculator
class correctly adds two integers and returns the expected sum.
Writing Test Cases with Assertions
In unit testing, assertions are used to validate the expected behavior of your code. Here's an example of writing test cases with assertions using xUnit:
using Xunit;
public class StringHelperTests
{
[Fact]
public void Concatenate_TwoStrings_ReturnsConcatenatedString()
{
// Arrange
string str1 = "Hello";
string str2 = "World";
var helper = new StringHelper();
// Act
string result = helper.Concatenate(str1, str2);
// Assert
Assert.Equal("HelloWorld", result);
}
[Fact]
public void IsNullOrEmpty_EmptyString_ReturnsTrue()
{
// Arrange
string str = "";
// Act
bool result = StringHelper.IsNullOrEmpty(str);
// Assert
Assert.True(result);
}
}
public class StringHelper
{
public string Concatenate(string str1, string str2)
{
return str1 + str2;
}
public bool IsNullOrEmpty(string str)
{
return string.IsNullOrEmpty(str);
}
}
The provided code is a unit test class using the xUnit framework to test the functionality of a StringHelper
class. The StringHelper
class contains two methods: Concatenate
and IsNullOrEmpty
. Below is the code breakdown:
The StringHelper
Class
This class contains two methods:
Concatenate
public string Concatenate(string str1, string str2) { return str1 + str2; }
This method takes two strings,
str1
andstr2
, as inputs and returns their concatenation.For example, if
str1
is "Hello" andstr2
is "World", the method returns "HelloWorld".
IsNullOrEmpty
public bool IsNullOrEmpty(string str) { return string.IsNullOrEmpty(str); }
This method takes a string,
str
, as input and returns a boolean indicating whether the string is null or empty.It uses the
string.IsNullOrEmpty
method provided by the .NET framework.
The StringHelperTests
Class
This class contains two unit tests that verify the behavior of the StringHelper
methods.
Concatenate_TwoStrings_ReturnsConcatenatedString
[Fact] public void Concatenate_TwoStrings_ReturnsConcatenatedString() { // Arrange string str1 = "Hello"; string str2 = "World"; var helper = new StringHelper(); // Act string result = helper.Concatenate(str1, str2); // Assert Assert.Equal("HelloWorld", result); }
Arrange:
Sets up the test by initializing two strings (str1
andstr2
) and creating an instance ofStringHelper
.Act:
Calls theConcatenate
method withstr1
andstr2
as arguments and stores the result.Assert:
Verifies that the result is "HelloWorld" usingAssert.Equal
.
IsNullOrEmpty_EmptyString_ReturnsTrue
[Fact] public void IsNullOrEmpty_EmptyString_ReturnsTrue() { // Arrange string str = ""; // Act bool result = StringHelper.IsNullOrEmpty(str); // Assert Assert.True(result); }
Arrange:
Sets up the test by initializing an empty string (str
).Act:
Calls theIsNullOrEmpty
method withstr
as the argument and stores the result.Assert:
Verifies that the result istrue
usingAssert.True
.
Key Concepts
[Fact]: This attribute indicates that the method is a unit test that should be run by the xUnit test runner.
Arrange-Act-Assert (AAA): A common pattern in unit testing:
Arrange: Set up the context and inputs for the test.
Act: Execute the method being tested.
Assert: Verify that the outcome is as expected.
Summary
The StringHelperTests
class contains unit tests for the StringHelper
class. These tests verify that the Concatenate
method correctly joins two strings and that the IsNullOrEmpty
method accurately identifies empty strings. The tests use xUnit's Fact
attribute and assertions to validate the expected behavior.
Running Tests and Interpreting Results
After writing your tests, you can run them to verify if your code behaves as expected:
Running Tests in Visual Studio:
Right-click on your test project or specific test file, and select"Run Tests"
.Running Tests Using .NET CLI:
Navigate to your test project directory and run:dotnet test
Interpreting Results:
Green checkmarks indicate passing tests.
Red marks indicate failing tests with details about the assertion that failed.
Use the test output to debug and fix any issues in your code.
Advanced Testing with xUnit
Parameterized Tests
Parameterized tests allow you to run the same test code with different inputs. This is useful for testing multiple scenarios without duplicating test code.
Example:
public class MathOperations
{
public int Add(int a, int b)
{
return a + b;
}
}
public class MathTests
{
[Theory]
[InlineData(3, 5, 8)] // Parameters: a = 3, b = 5, expected = 8
[InlineData(-2, 2, 0)] // Parameters: a = -2, b = 2, expected = 0
[InlineData(0, 0, 0)] // Parameters: a = 0, b = 0, expected = 0
public void Add_ShouldCalculateCorrectly(int a, int b, int expected)
{
// Arrange
var math = new MathOperations();
// Act
var result = math.Add(a, b);
// Assert
Assert.Equal(expected, result);
}
}
Asynchronous Tests
Asynchronous tests allow you to test code that runs asynchronously, such as methods using async and await.
Example:
public class AsyncOperations
{
public async Task<int> GetDataAsync()
{
// Simulate async operation
await Task.Delay(100);
return 42;
}
}
public class AsyncTests
{
[Fact]
public async Task GetDataAsync_ShouldReturnExpectedValue()
{
// Arrange
var asyncOperations = new AsyncOperations();
// Act
var result = await asyncOperations.GetDataAsync();
// Assert
Assert.Equal(42, result);
}
}
Running Tests in Parallel
Running tests in parallel allows multiple tests to execute concurrently, which can speed up overall test execution time.
Example:
public class ParallelTests
{
[Fact]
public void Test1()
{
// Test code
}
[Fact]
public void Test2()
{
// Test code
}
}
By default, xUnit runs tests in parallel for improved performance, unless explicitly disabled.
Mocking Dependencies
Introduction to Mocking
In unit testing, we often need to test a specific piece of code (let's call it ClassA) in isolation, without relying on its dependencies (like databases or external services). Mocking allows us to simulate these dependencies so that ClassA can be tested independently.
Using a Mocking Framework with xUnit
xUnit itself doesn't provide built-in mocking capabilities, but it works seamlessly with popular mocking frameworks like Moq. Moq is a mocking library for .NET that allows us to create mock objects dynamically.
Creating and Using Mock Objects
Let’s walk through an example using Moq to mock a dependency:
- Install Moq:
First, install the Moq NuGet package in your test project.
Install-Package Moq
Example Scenario:
Suppose we have aUserService
class that depends on anIUserRepository
interface to retrieve user data. We want to testUserService
without accessing the actual database.Create Mock Object:
Use Moq to create a mock object ofIUserRepository
.using Moq; public interface IUserRepository { User GetUserById(int userId); } public class UserService { private readonly IUserRepository _userRepository; public UserService(IUserRepository userRepository) { _userRepository = userRepository; } public string GetUserFullName(int userId) { var user = _userRepository.GetUserById(userId); return $"{user.FirstName} {user.LastName}"; } } public class User { public int UserId { get; set; } public string FirstName { get; set; } public string LastName { get; set; } }
Writing the Test:
Use Moq to mockIUserRepository
and set up behavior for the mock.using Xunit; using Moq; public class UserServiceTests { [Fact] public void GetUserFullName_ReturnsFullName() { // Arrange var mockUserRepository = new Mock<IUserRepository>(); mockUserRepository.Setup(repo => repo.GetUserById(1)) .Returns(new User { UserId = 1, FirstName = "John", LastName = "Doe" }); var userService = new UserService(mockUserRepository.Object); // Act var fullName = userService.GetUserFullName(1); // Assert Assert.Equal("John Doe", fullName); } }
In this example, mockUserRepository.Object
provides a mock implementation of IUserRepository
, allowing us to test UserService
without accessing real database data. This isolation helps in focusing on testing the logic of UserService
independently.
Testing Best Practices
Writing Effective Unit Tests
Focus on One Thing:
Each test should focus on a single behavior or function.Clear and Descriptive Names:
Use names that describe what the test is checking or verifying.Test Behavior, Not Implementation:
Tests should verify the expected behavior of your code, not how it’s implemented.Use Assertions:
Include assertions to verify expected outcomes against actual results.
Organizing Your Test Code
Separate Test Classes:
Organize tests into separate classes based on the functionality they test.Group Related Tests:
Use test suites or categories to group tests that cover similar scenarios.Arrange-Act-Assert (AAA):
Structure each test with a clear arrangement of data, action (method call), and assertion of the expected outcome.
Common Pitfalls and How to Avoid Them
Fragile Tests:
Avoid tests that break easily due to changes in unrelated code. Mock dependencies to isolate tests.Overly Complex Tests:
Keep tests simple and focused. Use parameterized tests for different scenarios instead of duplicating code.Ignoring Edge Cases:
Ensure tests cover edge cases and boundary conditions to catch unexpected behaviors.Not Updating Tests:
Update tests when code changes to reflect new behaviors or requirements.
Conclusion
Mastering unit testing with xUnit is essential for any C# developer. xUnit is easy to use, modern, and flexible, making it perfect for writing reliable tests.
By following best practices and using features like parameterized tests, asynchronous tests, and mocking, you can create strong and maintainable unit tests.
I hope you found this guide helpful and learned something new. Stay tuned for the next article in the "Mastering C#" series: "Working with JSON in C#: Serialization and Deserialization."
Happy coding!
Subscribe to my newsletter
Read articles from Opaluwa Emidowojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
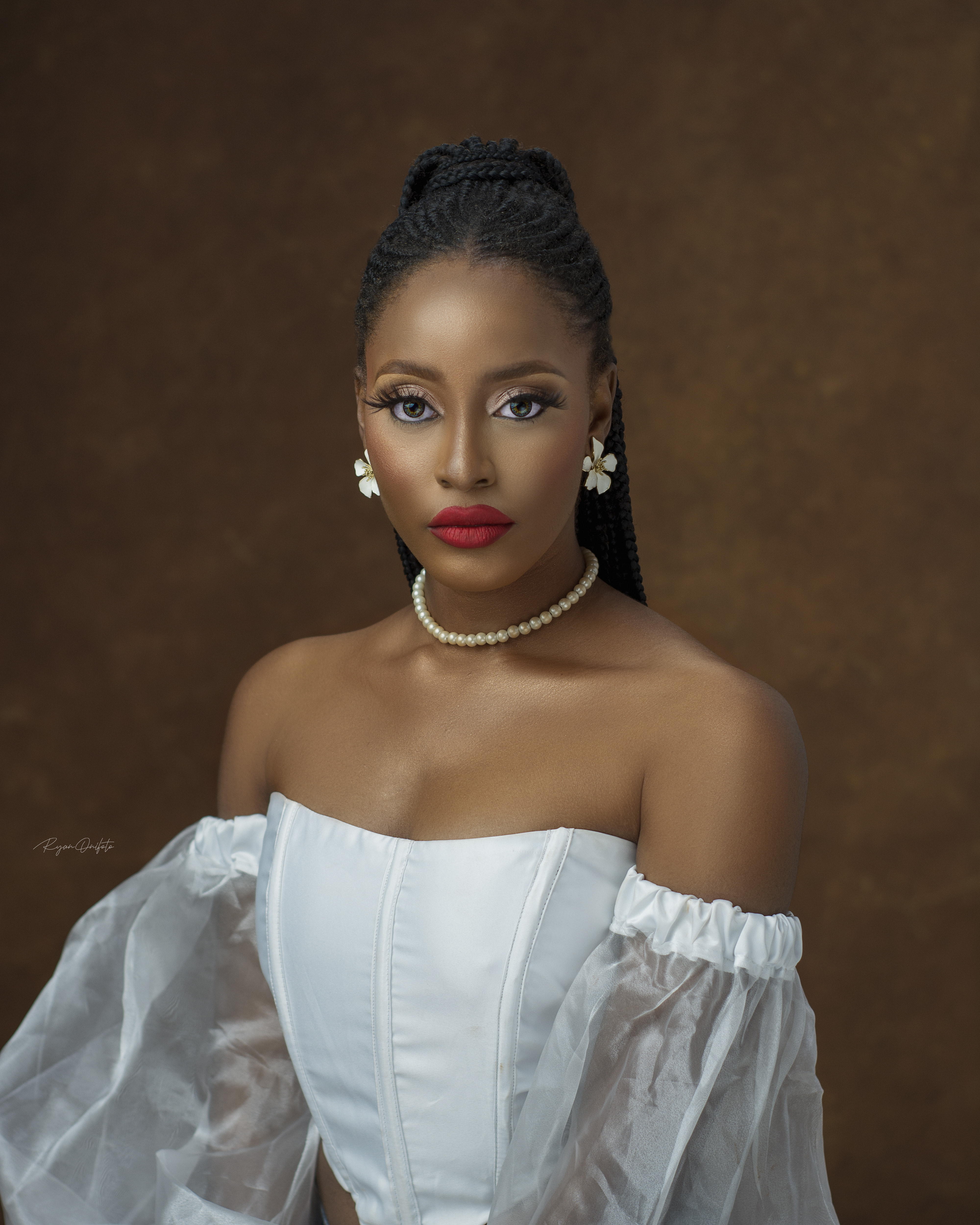