Identifying Bottlenecks in the Backend
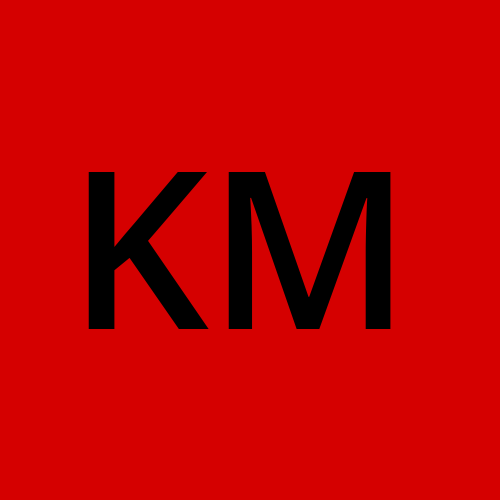
Application performance optimization has been my greatest pursuit as a Backend developer .
Understanding that perfomance is not only about writing code but understanding the factors that affect speed and latency of your backend stack. Network latency ,protocol serialization and connection establishment just to name a few are factors that greatly impact performance .
So i’d like to discuss different bottlenecks in the backend and ways to optimize application performance.
Bottlenecks essentially are points in a system where the flow of data or requests is slowed down or blocked. This may cause a lag in the system or an error.
In the backend, the server-side logic and processing of an application can affect the actual performance and scalability .For example memory leaks or concurrency issues, your application may fail to handle high volume of requests . When your application is not performing adequately under load,it's important to understand the bottlenecks in the system and address them .
Bottlenecks in the backend are commonly found in three areas:
I/O’s (i.e database queries), memory usage ,cpu usage
Database
Databases are the data storage and retrieval layer of your application,
The following are some ground rules when it comes to querying a database to reduce the number of I/O’s:
Avoid executing database queries inside loops.
Use indexed fields for joins or aggregation lookups.
Employ bulk operations when reading and writing large volumes of data.
Cache frequently accessed data.
Offload and schedule query-intensive operations. If you must execute a large number of database queries for a specific task (e.g., generating a report), run them in a separate worker process to avoid interfering with regular usage. Ideally, schedule these operations during off-peak times.
Memory usage
I think we may all agree that at one point in time we have experienced our servers running out of memory .Instead of throwing more RAM at the problem we should consider finding out if our software can be less memory hungry . Here are some rules
For operations that require a significant amount of memory or have indefinite memory usage, consider buffering data with a file or a database.
Release data that is no longer needed in memory to prevent memory leaks.
Use pagination or batch operations to avoid excessive memory consumption.
Implement memory-efficient algorithms whenever possible.
CPU usage
Computational power is the last limiting resource that frequently causes bottlenecks , While cpu usage largely depends on the nature of your application here are some ground rules.
Delegate data operations to the database whenever possible, as it can handle tasks like joins, grouping, and sorting more efficiently than code.
Cache results that are frequently computed,so that they can be retrieved faster without repeating expensive computations or queries.
Offload and schedule computationally intensive operations also splitting a large task into smaller subtasks that can be executed simultaneously by multiple threads or processes, so that they can be completed faster with more CPU utilization.
Use CPU-efficient algorithms whenever possible. Evaluate the algorithm's big-O complexity, keeping in mind the potential trade-off with memory usage.
Of Course there are many other techniques to resolve bottlenecks as i’ve highlighted only a few .
Lets keep learning and experimenting 😀
Check out HNG Internship https://hng.tech/internship, https://hng.tech/hire
Subscribe to my newsletter
Read articles from Kitts Makokha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
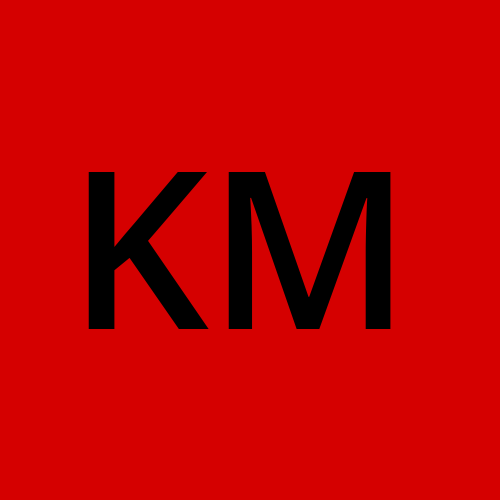